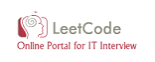
LeetCode全题解
LeetCode全题解
CheeRok
这个作者很懒,什么都没留下…
展开
-
LeetCode题解:Convert Sorted Array to Binary Search Tree
Given an array where elements are sorted in ascending order, convert it to a height balanced BST.题意:给定一个升序数组,将它转换为二叉搜索树解决思路:二分……代码:public class Solution { public TreeNode sortedArrayToBST(int[] num原创 2015-10-02 20:43:08 · 573 阅读 · 0 评论 -
LeetCode题解:Sum Root to Leaf Numbers
Given a binary tree containing digits from 0-9 only, each root-to-leaf path could represent a number.An example is the root-to-leaf path 1->2->3 which represents the number 123.Find the total sum of al原创 2015-10-02 21:14:31 · 832 阅读 · 1 评论 -
LeetCode题解:Word Ladder
Given two words (beginWord and endWord), and a dictionary’s word list, find the length of shortest transformation sequence from beginWord to endWord, such that:Only one letter can be changed at a time原创 2015-10-02 21:13:10 · 1146 阅读 · 0 评论 -
LeetCode题解:Best Time to Buy and Sell Stock II
Say you have an array for which the ith element is the price of a given stock on day i.Design an algorithm to find the maximum profit. You may complete as many transactions as you like (ie, buy one and原创 2015-10-02 21:07:59 · 940 阅读 · 0 评论 -
LeetCode题解:Best Time to Buy and Sell Stock
Say you have an array for which the ith element is the price of a given stock on day i.If you were only permitted to complete at most one transaction (ie, buy one and sell one share of the stock), desi原创 2015-10-02 21:06:16 · 1075 阅读 · 0 评论 -
LeetCode题解:Triangle
Given a triangle, find the minimum path sum from top to bottom. Each step you may move to adjacent numbers on the row below.For example, given the following triangle [ [2], [3,4], [6,5,原创 2015-10-02 21:04:26 · 902 阅读 · 0 评论 -
LeetCode题解:Populating Next Right Pointers in Each Node
Given a binary treestruct TreeLinkNode { TreeLinkNode *left; TreeLinkNode *right; TreeLinkNode *next;}Populate each next pointer to point to its next right node. If there is no next right node,原创 2015-10-02 21:01:47 · 952 阅读 · 0 评论 -
LeetCode题解:Flatten Binary Tree to Linked List
Given a binary tree, flatten it to a linked list in-place.For example, Given 1 / \ 2 5 / \ \ 3 4 6The flattened tree should look like: 1 \ 2 \ 3原创 2015-10-02 20:59:45 · 809 阅读 · 0 评论 -
LeetCode题解:Path Sum II
Given a binary tree and a sum, find all root-to-leaf paths where each path’s sum equals the given sum.For example: Given the below binary tree and sum = 22, 5 / \原创 2015-10-02 20:50:41 · 736 阅读 · 0 评论 -
LeetCode题解:Convert Sorted List to Binary Search Tree
Given a singly linked list where elements are sorted in ascending order, convert it to a height balanced BST.题意:给定一个升序排列的单链表,转换为二叉搜索树解决思路:同样是二分……代码:public class Solution { private ListNode node;原创 2015-10-02 20:45:44 · 1004 阅读 · 0 评论 -
LeetCode题解:Construct Binary Tree from Inorder and Postorder Traversal
Given inorder and postorder traversal of a tree, construct the binary tree.Note: You may assume that duplicates do not exist in the tree.题解:通过中序遍历和后序遍历还原二叉树解决思路:首先要明确一点,对于后序遍历的结果,如果一个元素所在的位置为i,若在中序遍历的原创 2015-10-02 20:35:46 · 796 阅读 · 0 评论 -
LeetCode题解:Construct Binary Tree from Preorder and Inorder Traversal
Given preorder and inorder traversal of a tree, construct the binary tree.Note: You may assume that duplicates do not exist in the tree.题意:给定一棵树的前序遍历和中序遍历,还原二叉树解决思路:我们可以知道的是,前序遍历的第一个结点就是根节点,而且对于中序遍历,每原创 2015-10-02 20:16:29 · 510 阅读 · 0 评论 -
LeetCode题解:Binary Tree Zigzag Level Order Traversal
Given a binary tree, return the zigzag level order traversal of its nodes’ values. (ie, from left to right, then right to left for the next level and alternate between).For example: Given binary tree原创 2015-10-02 20:10:01 · 811 阅读 · 0 评论 -
LeetCode题解:Validate Binary Search Tree
Given a binary tree, determine if it is a valid binary search tree (BST).Assume a BST is defined as follows:The left subtree of a node contains only nodes with keys less than the node’s key. The right原创 2015-10-02 20:06:23 · 701 阅读 · 0 评论 -
LeetCode题解:Unique Binary Search Trees II
Given n, generate all structurally unique BST’s (binary search trees) that store values 1…n.For example, Given n = 3, your program should return all 5 unique BST’s shown below.1 3 3 2原创 2015-10-02 20:01:33 · 711 阅读 · 0 评论 -
LeetCode题解:Unique Binary Search Trees
Given n, how many structurally unique BST’s (binary search trees) that store values 1…n?For example, Given n = 3, there are a total of 5 unique BST’s.1 3 3 2 1 \ /原创 2015-10-02 19:57:15 · 542 阅读 · 0 评论 -
LeetCode题解:Binary Tree Inorder Traversal
Given a binary tree, return the inorder traversal of its nodes’ values.For example: Given binary tree {1,#,2,3}, 1 \ 2 / 3 return [1,3,2].题意:中序遍历二叉树解决思路:”左根右“的递归或者通过栈完成的迭代代码:p原创 2015-10-02 19:29:46 · 665 阅读 · 0 评论 -
LeetCode题解:Restore IP Addresses
Given a string containing only digits, restore it by returning all possible valid IP address combinations.For example: Given “25525511135”,return [“255.255.11.135”, “255.255.111.35”]. (Order does not原创 2015-10-02 19:27:50 · 909 阅读 · 0 评论 -
LeetCode题解:Reverse Linked List II
Reverse a linked list from position m to n. Do it in-place and in one-pass.For example: Given 1->2->3->4->5->NULL, m = 2 and n = 4,return 1->4->3->2->5->NULL.Note: Given m, n satisfy the following co原创 2015-10-02 19:26:17 · 812 阅读 · 0 评论 -
LeetCode题解:Decode Ways
A message containing letters from A-Z is being encoded to numbers using the following mapping:‘A’ -> 1 ‘B’ -> 2 … ‘Z’ -> 26 Given an encoded message containing digits, determine the total number of原创 2015-10-02 19:19:42 · 1761 阅读 · 0 评论 -
LeetCode题解:Reverse Bits
Reverse bits of a given 32 bits unsigned integer.For example, given input 43261596 (represented in binary as 00000010100101000001111010011100), return 964176192 (represented in binary as 00111001011110原创 2015-08-26 20:35:16 · 453 阅读 · 0 评论 -
LeetCode题解:Implement Stack using Queues
Implement the following operations of a stack using queues.push(x) – Push element x onto stack.pop() – Removes the element on top of the stack.top() – Get the top element.empty() – Return whether t原创 2015-08-12 11:02:13 · 484 阅读 · 0 评论 -
LeetCode题解:Pascal's Triangle
Given numRows, generate the first numRows of Pascal’s triangle.For example, given numRows = 5, Return [ [1], [1,1], [1,2,1], [1,3,3,1], [1,4,6,4,1] ]题意是:给定一个正整数numR原创 2015-08-12 10:08:25 · 594 阅读 · 0 评论 -
LeetCode题解:Subsets II
Given a collection of integers that might contain duplicates, nums, return all possible subsets.Note: Elements in a subset must be in non-descending order. The solution set must not contain duplicate原创 2015-09-06 21:32:54 · 670 阅读 · 0 评论 -
LeetCode题解:Subsets
Given a set of distinct integers, nums, return all possible subsets.Note: Elements in a subset must be in non-descending order. The solution set must not contain duplicate subsets. For example, If原创 2015-09-06 21:30:11 · 511 阅读 · 0 评论 -
LeetCode题解:Search Insert Position
Given a sorted array and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.You may assume no duplicates in the array.Here原创 2015-09-06 21:28:13 · 463 阅读 · 0 评论 -
LeetCode题解:Group Anagrams
Given an array of strings, group anagrams together.For example, given: [“eat”, “tea”, “tan”, “ate”, “nat”, “bat”], Return:[ [“ate”, “eat”,”tea”], [“nat”,”tan”], [“bat”] ] Note: For the re原创 2015-09-06 21:24:27 · 1327 阅读 · 0 评论 -
LeetCode题解:Permutations
Given a collection of numbers, return all possible permutations.For example, [1,2,3] have the following permutations: [1,2,3], [1,3,2], [2,1,3], [2,3,1], [3,1,2], and [3,2,1].题意:求出给定数字的所有组合可能解决思路:先加入原创 2015-09-06 21:14:25 · 453 阅读 · 0 评论 -
LeetCode题解:Search for a Range
Given a sorted array of integers, find the starting and ending position of a given target value.Your algorithm’s runtime complexity must be in the order of O(log n).If the target is not found in the ar原创 2015-09-06 21:07:40 · 445 阅读 · 0 评论 -
LeetCode题解:Next Permutation
Implement next permutation, which rearranges numbers into the lexicographically next greater permutation of numbers.If such arrangement is not possible, it must rearrange it as the lowest possible orde原创 2015-09-06 21:03:15 · 565 阅读 · 0 评论 -
LeetCode题解:Divide Two Integers
Divide two integers without using multiplication, division and mod operator.If it is overflow, return MAX_INT.题意:不使用乘,除,模符号作除法运算解决思路:每一个数都可以用2进制表示,利用这一点通过位运算完成代码:public class Solution { public int原创 2015-09-06 20:58:18 · 1025 阅读 · 0 评论 -
LeetCode题解:Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head.For example, Given 1->2->3->4, you should return the list as 2->1->4->3.Your algorithm should use only constant space. You may no原创 2015-09-06 20:55:30 · 510 阅读 · 0 评论 -
LeetCode题解:Construct Binary Tree from Preorder and Inorder Traversal
Given preorder and inorder traversal of a tree, construct the binary tree.Note: You may assume that duplicates do not exist in the tree.题意:给定一棵树的先序遍历和中序遍历,还原该树解决思路:在中序遍历中,每一个根节点的左方为其左子树,右方为右子树;而在先序遍历中原创 2015-09-06 20:52:08 · 523 阅读 · 0 评论 -
LeetCode题解:Generate Parentheses
Given n pairs of parentheses, write a function to generate all combinations of well-formed parentheses.For example, given n = 3, a solution set is:“((()))”, “(()())”, “(())()”, “()(())”, “()()()”题意:求给定原创 2015-08-28 11:01:34 · 482 阅读 · 0 评论 -
LeetCode题解:4Sum
Given an array S of n integers, are there elements a, b, c, and d in S such that a + b + c + d = target? Find all unique quadruplets in the array which gives the sum of target.Note: Elements in a quad原创 2015-08-28 10:59:44 · 476 阅读 · 0 评论 -
LeetCode题解:Letter Combinations of a Phone Number
Given a digit string, return all possible letter combinations that the number could represent.A mapping of digit to letters (just like on the telephone buttons) is given below.Input:Digit string “23”原创 2015-08-28 10:58:34 · 479 阅读 · 0 评论 -
LeetCode题解:3Sum Closest
Given an array S of n integers, find three integers in S such that the sum is closest to a given number, target. Return the sum of the three integers. You may assume that each input would have exactly原创 2015-08-28 10:57:06 · 461 阅读 · 0 评论 -
LeetCode题解:3Sum
Given an array S of n integers, are there elements a, b, c in S such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.Note: Elements in a triplet (a,b,c) must be i原创 2015-08-28 10:55:52 · 408 阅读 · 0 评论 -
LeetCode题解:Integer to Roman
Given an integer, convert it to a roman numeral.Input is guaranteed to be within the range from 1 to 3999.题意:给定一个整数,将其转换为罗马数解题思路:按照转换关系换代码:import java.util.LinkedHashMap;public class Solution { pri原创 2015-08-28 10:54:26 · 456 阅读 · 0 评论 -
LeetCode题解:Container With Most Water
Given n non-negative integers a1, a2, …, an, where each represents a point at coordinate (i, ai). n vertical lines are drawn such that the two endpoints of line i is at (i, ai) and (i, 0). Find two lin原创 2015-08-28 10:53:18 · 477 阅读 · 0 评论