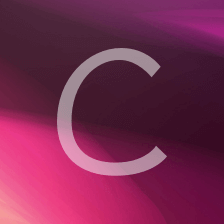
cc-6th
SharonMoMo
Every thing will be okay in the end....
展开
-
10.5 Sparse Search
The basic idea is binary search. But we have to modify it a little bit: when str[mid] is “”, we have to find a non empty string near to original one as the new str[mid]! int search(vector<string>& s原创 2015-11-02 07:09:21 · 398 阅读 · 0 评论 -
Design Pattern/ OOD/ System Design
可以看一些design pattern的书比如Design Patterns: Elements of Reusable Object-Oriented Software(我们上课用的这本) 关于系统设计,这个其实很看积累的,system本来就是考功底的地方,如果没时间上一些system的课(os, distributed system什么的),可以抽空去看下这些课的slides 另外读一些现在转载 2015-10-30 03:57:23 · 1467 阅读 · 0 评论 -
5.3 Flip Bit to Win
The first method to solve this problem is using O(k) time w/ O(k) memory where k indicates length of input in binary form. //java code: public class program { public static int longestSequen原创 2015-10-29 08:09:13 · 457 阅读 · 0 评论 -
5.4 Next Number
Be clear about how to clear or update or reset bits!!! public class program { public static int getNext(int n){ int c = n, c0 = 0, c1 = 0; while((c & 1) == 0 && c != 0){原创 2015-10-29 12:43:45 · 305 阅读 · 0 评论 -
5.2 Binary to String
Be clear about how to convert decimal w/o fraction to binary and decimal w/ fraction to binary.A similar but a little bit more complex problem can be found from lintCode: Binary Representation. Code is原创 2015-10-29 06:10:40 · 464 阅读 · 0 评论 -
5.6 Conversion
Note: c & (c - 1)c & (c - 1)will clear the least significant bit in c. if we need to call this equation n times to let c becomes 0, there are n 1s in c! int bitSwapRequired(int a, int b) { /原创 2015-10-29 05:23:48 · 200 阅读 · 0 评论 -
5.1 Insertion
We need a mask for this problem which helps us to clear the bits we need to insert. The left part of 1s can shift left for j+1 bits. For right part, we can get it by set the initial value of right as原创 2015-10-29 05:15:34 · 250 阅读 · 0 评论 -
5.7 Pairwise Swap
Using mask1 = 101010 to find odd bits, mask2 = 010101 to find even bits. Then mask1 >>> 1 to even pos, and mask2 << 1 to odd pos. The swap res will be then or result. public static int swaPair(int n原创 2015-10-29 12:57:09 · 499 阅读 · 0 评论 -
3.2 Stack min
Using extra stack to track minimum element. class MinStack {public: stack<int> s1; stack<int> s2; void push(int x) { s1.push(x); if(s2.empty() || x<= getMin()) s2.push(x原创 2015-10-27 12:17:29 · 241 阅读 · 0 评论 -
2.5 Sum List
Q: numbers are stored in reverse order. L1: 7->1->6 (716) L2:5->9->2 (295) L1 + L2 = 716 + 295 = 912 L3: 2->1->9. ListNode* addTwoNumbers(ListNode* l1, ListNode* l2{ ListNode* newHead =原创 2015-10-27 05:30:08 · 280 阅读 · 0 评论 -
8.1 Triple Stair
Dynamic Programming: For ith stair there are three ways to jump here: a. From (i-1)th stair and jump one step; b. From(i-2) th stiar and jump two steps; c. For(i-3)th stair and jump three steps;原创 2015-10-30 06:50:43 · 271 阅读 · 0 评论 -
8.2 Robot in a Grid
Simple dynamic problem, we can reduce the memory space we used by only using O(n) space instead of O(mn) space.O(mn) space code: int uniquePathsWithObstacles(vector<vector<int> > &obstacleGrid) {原创 2015-10-30 07:13:39 · 297 阅读 · 0 评论 -
8.3 Magic Index
If elements in the sorted array are distinct, then we could simply find the index by binary search. public static int findMagic(int[] array){ int lo = 0, hi = array.length; while(lo<原创 2015-10-30 08:22:21 · 248 阅读 · 0 评论 -
10.3 Search in Rotated Array
Without duplicates the code will run O(logn), however, if there are lots of duplicates it will run in O(n). bool search(vector<int> &A, int target) { // write your code here int lo =原创 2015-10-31 13:24:13 · 256 阅读 · 0 评论 -
8.10 Paint Fill
If given PaintFill(screen, 2, 2, Color.White), we should have: Basically we do DFS, the code is shown as following: public enum Colors{ WHITE, BLACK, GREEN, RED, YELLOW}; public static boolean P原创 2015-10-31 01:04:06 · 294 阅读 · 0 评论 -
10.2 Group Anagram
Simple string question. void groupAnagrams(vector<string>& strs, vector<string>& res) { unordered_map<string, multiset<string>> mp; for (string s : strs) { string t = s; sort原创 2015-10-31 12:31:11 · 314 阅读 · 0 评论 -
8.14 Boolean Evaluation
Assume the input string always valid(which means odd position always be operator). We solve problem recursively by check the operator each time: “|”: leftTrue*rightTrue + leftTrue* rightFalse + leftFa原创 2015-10-31 05:12:16 · 343 阅读 · 0 评论 -
8.13 Stack of Boxes
First we have to sort the boxes by their height (descending), then we try to find each the max height for each box i as the bottom one and store their max height in a int[]. public static int create原创 2015-10-31 03:40:12 · 305 阅读 · 0 评论 -
8.11 Coins
For example, if we have n = 100 cents: makeChange(100) = makeChange(100 w/ 0 quater) + makeChange(100 w/ 1 quater) + makeChange(100 w/ 2 quater) + makeChange(100 w/ 3 quater) + makeChange(100 w/ 4 qu原创 2015-10-31 02:31:15 · 232 阅读 · 0 评论 -
8.4 Power Set
Simple backtracking question. void getSubsets(vector<vector<int>>& result, vector<int>& nums, vector<int>& sub,int pos){ result.push_back(sub); for (int i=pos; i<nums.size(); ++i) {原创 2015-10-30 08:38:05 · 272 阅读 · 0 评论 -
8.7 8.8 Permutation
Following code can handle both unique or duplicates string! void getPermutation(string& s, int pos, vector<string>& res){ if(pos == s.size()){ res.push_back(s); retur原创 2015-10-30 13:17:47 · 203 阅读 · 0 评论 -
8.5 Recursive Multiply
Nothing special… public static int helper(int small, int large){ if(small == 0) return 0; if(small == 1) return large; int s = small/2; int tmpProduct = helper(s,larg原创 2015-10-30 08:59:26 · 342 阅读 · 0 评论 -
2.4 Partition
Build two linked list to track small element and large element. ListNode* partition(ListNode* head, int k){ if(!head ) return head; ListNode* newHeadS = new ListNode(-1); Lis原创 2015-10-27 04:24:25 · 268 阅读 · 0 评论 -
4.6 Successor
For this problem, first we need to know the in-order traverse: left -> root ->right. Then we can analyze the problem by simply draw a BT: Therefore, we can find there are total three different原创 2015-10-28 04:07:48 · 318 阅读 · 0 评论 -
4.4 Check Balanced
Balanced Binary Tree: 1. The left and right subtree’s height differ by at most one, AND 2. The left subtree is balanced, AND 3. The right subtree is balance.According to above definition, we have原创 2015-10-28 02:46:42 · 265 阅读 · 0 评论 -
1.8 Rotate Matrix
Clock-wise rotate: matrix upside down then swap number in pairs which are symmetric by diagonal; Anti-clock rotate: reverse matrix right->left; then do swap; void rotate(vector<vector<int>>& matrix原创 2015-10-26 14:54:57 · 341 阅读 · 0 评论 -
1.8 Zero Matrix
Using the first row and column to store that row and col’s state: if there is a ‘0’ element matrix[i][0] = matrix[0][j] = 0. And using a tmp variable (at here it’s called col0) to indicate the first原创 2015-10-26 15:20:49 · 435 阅读 · 0 评论 -
careercup sumarry
http://www.foreverpostcard.com/2014/08/summary-of-ctci.html转载 2015-10-26 14:19:00 · 370 阅读 · 0 评论 -
1.6 String Compression
Just a simple string problem. string compress(string str){ string res = ""; int cnt = 1; for (int i = 0; i<str.size(); ++i) { if (res.size() >= str.size()) return原创 2015-10-26 14:30:56 · 428 阅读 · 0 评论 -
1.3 URLify
First, we count the number of spaces between string. Because we are going to replace those spaces with “%20”, which means the new length of s will become length + spaceCnt * 2.void replaceSpaces(char*原创 2015-10-24 15:01:15 · 642 阅读 · 0 评论 -
1.2 Check Permutation
bool permutation(string s, string t){ if(s.size() != t.size()) return false; char mp[128] = {0}; for(char c : s) ++mp[c]; for(char c : t){ --mp[c]; if(mp[c]<0) return fa原创 2015-10-24 14:11:58 · 369 阅读 · 0 评论 -
1.5 One Array
If the difference between string s and string t ‘s size larger than one, return false; bool oneEditWay(string s, string t){ if( abs((int)s.size() - (int)t.size()) > 1) return false;原创 2015-10-24 17:16:39 · 309 阅读 · 0 评论 -
1.1 IS Unique
“`C++ bool isUnique(string s){ if(s.size() > 128) return false; char mp[128] = {0}; for(char c : s){ if(mp[c] > 0) return false; ++mp[c];原创 2015-10-24 14:03:32 · 379 阅读 · 0 评论 -
1.9 String Rotation
By observing the relationship between s1 and s2, we find that if s2 is substring of s1s1 we return true and we only use one call of isSubString(). bool isRotation(string s1, string s2){ int原创 2015-10-27 02:04:15 · 293 阅读 · 0 评论 -
2.6 Palindrome
1. Reverse and Compare: ListNode* reverseList(ListNode* head){ if(!head) return head; ListNode* pre = NULL; while (head) { ListNode* tmp = head->next;原创 2015-10-27 06:46:34 · 281 阅读 · 0 评论 -
2.7 Intersection
Simple linked list problem. ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) { // write your code here if(!headA || !headB) return NULL; ListNode* p = headA;原创 2015-10-27 07:03:32 · 263 阅读 · 0 评论 -
4.2 Minimal Tree
Binary search tree with minimal height means: we want the root to be the middle of the array. TreeNode* builder (vector<int>& nums, int start, int end){ if( start > end) return nullptr;原创 2015-10-27 14:29:33 · 248 阅读 · 0 评论 -
2.8 Loop Detection
For this problem, we have to do a little math deduction: let L1 indicates the distance between start point and head; L2 indicates the distance between start point and meet point; C indicates the lengt原创 2015-10-27 07:34:20 · 326 阅读 · 0 评论 -
3.5 Sort Stack
Using extra stack to sort old stack s, let its smallest element on top. For this problem we use a temporary variable cur to store s.top(), and try to find a right place for cur in stack r. stack<in原创 2015-10-27 13:17:49 · 273 阅读 · 0 评论 -
2.2 Return Kth to Last
Eg: 1–>2–>3–>4–>5–>6–>7–>NULL 4th node to last should be: 4.Just a simple two pointer problem. int kthNodeToLast(ListNode* head, int k){ if(!head || !k) return -1; ListNode* fast =原创 2015-10-27 03:40:54 · 295 阅读 · 0 评论