一、CountDownLatch
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CountDownLatch;
/**
* CountDownLatch 用于文本文件中多行数字求和
* @author superman
*
*/
public class Demo2 {
private int[] nums;
public Demo2(int line) {
nums = new int[line];
}
public void calc(String line, int index, CountDownLatch latch) {
String[] nus = line.split(",");
int total = 0;
for (String num : nus) {
total += Integer.parseInt(num);
}
nums[index] = total;
System.out.println(Thread.currentThread().getName() + " 执行计算任务... " + line + " 结果为:" + total);
latch.countDown();
}
public void sum() {
System.out.println("汇总线程开始执行... ");
int total = 0;
for (int i = 0; i < nums.length; i++) {
total += nums[i];
}
System.out.println("最终的结果为:" + total);
}
public static void main(String[] args) {
List<String> contents = readFile();
int lineCount = contents.size();
CountDownLatch latch = new CountDownLatch(lineCount);
Demo2 d = new Demo2(lineCount);
for (int i = 0; i < lineCount; i++) {
final int j = i;
new Thread(new Runnable() {
@Override
public void run() {
d.calc(contents.get(j), j, latch);
}
}).start();
}
try {
latch.await();
} catch (InterruptedException e) {
e.printStackTrace();
}
d.sum();
}
private static List<String> readFile() {
List<String> contents = new ArrayList<>();
String line = null;
BufferedReader br = null;
try {
br = new BufferedReader(new FileReader("nums.txt"));
while ((line = br.readLine()) != null) {
contents.add(line);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return contents;
}
}
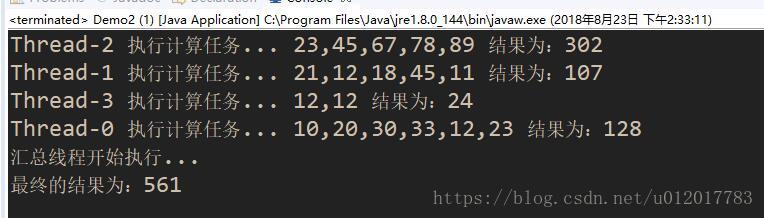
二、CyclicBarrier
import java.util.Random;
import java.util.concurrent.CyclicBarrier;
/**
* 控制多个线程同一时刻访问
* @author superman
*
*/
public class Demo {
Random random = new Random();
public void meeting(CyclicBarrier barrier) {
try {
Thread.sleep(random.nextInt(4000));
} catch (InterruptedException e1) {
e1.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + " 到达会议室,等待开会..");
if(Thread.currentThread().getName().equals("Thread-7")) {
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
e.printStackTrace();
}
barrier.reset();
}
try {
barrier.await();
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
Demo demo = new Demo();
CyclicBarrier barrier = new CyclicBarrier(10, new Runnable() {
@Override
public void run() {
System.out.println("好!我们开始开会...");
}
});
for (int i = 0; i < 10; i++) {
new Thread(new Runnable() {
@Override
public void run() {
demo.meeting(barrier);
}
}).start();
}
new Thread(new Runnable() {
@Override
public void run() {
while(true) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("等待的线程数 " + barrier.getNumberWaiting());
System.out.println("is broken " + barrier.isBroken());
}
}
}).start();
}
}
三、Semaphore
import java.util.concurrent.Semaphore;
/**
* 同一时间段访问资源的数量
* @author superman
*
*/
public class Demo {
public void method (Semaphore semaphore) {
try {
semaphore.acquire();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + " is run ...");
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
semaphore.release();
}
public static void main(String[] args) {
Demo d = new Demo();
Semaphore semaphore = new Semaphore(10);
while(true) {
new Thread(new Runnable() {
@Override
public void run() {
d.method(semaphore);
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}).start();
}
}
}
四、Exchanger
import java.util.concurrent.Exchanger;
/**
* 用于线程间信息的交换
* @author superman
*
*/
public class Demo {
public void a (Exchanger<String> exch) {
System.out.println("a 方法执行...");
try {
System.out.println("a 线程正在抓取数据...");
Thread.sleep(2000);
System.out.println("a 线程抓取到数据...");
} catch (InterruptedException e) {
e.printStackTrace();
}
String res = "12345";
try {
System.out.println("a 等待对比结果...");
exch.exchange(res);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public void b (Exchanger<String> exch) {
System.out.println("b 方法开始执行...");
try {
System.out.println("b 方法开始抓取数据...");
Thread.sleep(4000);
System.out.println("b 方法抓取数据结束...");
} catch (InterruptedException e) {
e.printStackTrace();
}
String res = "12345";
try {
String value = exch.exchange(res);
System.out.println("开始进行比对...");
System.out.println("比对结果为:" + value.equals(res));
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
Demo d = new Demo();
Exchanger<String> exch = new Exchanger<>();
new Thread(new Runnable() {
@Override
public void run() {
d.a(exch);
}
}).start();
new Thread(new Runnable() {
@Override
public void run() {
d.b(exch);
}
}).start();
}
}