一、车间
/**
* 车间(电商平台)
* @author superman
*
*/
public class Tmall {
private int count;
public final int max=10;
public synchronized void produce() {
while(count>=max) {
try {
System.out.println(Thread.currentThread().getName()+":"+"库存数量达到上限,生产停止");
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
count++;
System.out.println(Thread.currentThread().getName()+":"+"生产者生产,"+"当前库存的数量为"+count);
notifyAll();
}
public synchronized void consume() {
while(count<=0) {
try {
System.out.println(Thread.currentThread().getName()+":"+"库存数量为0,停止消费");
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
count--;
System.out.println(Thread.currentThread().getName()+":"+"消费者消费,当前库存为"+count);
notifyAll();
}
}
二、生产者
/***
* 生产者
* @author superman
*
*/
public class Producer implements Runnable {
private Tmall tmall;
public Producer(Tmall tmall) {
this.tmall=tmall;
}
@Override
public void run() {
while(true) {
tmall.produce();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
三、消费者
/**
* 消费者
* @author superman
*
*/
public class Consumer implements Runnable{
private Tmall tmall;
public Consumer(Tmall tmall) {
this.tmall=tmall;
}
@Override
public void run() {
while(true) {
tmall.consume();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
四、主调用类
/**
* 主函数实现生产者,消费者模拟过程
* @author superman
*
*/
public class Main {
public static void main(String[] args) {
Tmall mall=new Tmall();
Producer p=new Producer(mall);
Consumer c=new Consumer(mall);
new Thread(p).start();
new Thread(p).start();
new Thread(p).start();
new Thread(p).start();
new Thread(p).start();
new Thread(c).start();
new Thread(c).start();
new Thread(c).start();
new Thread(c).start();
}
}
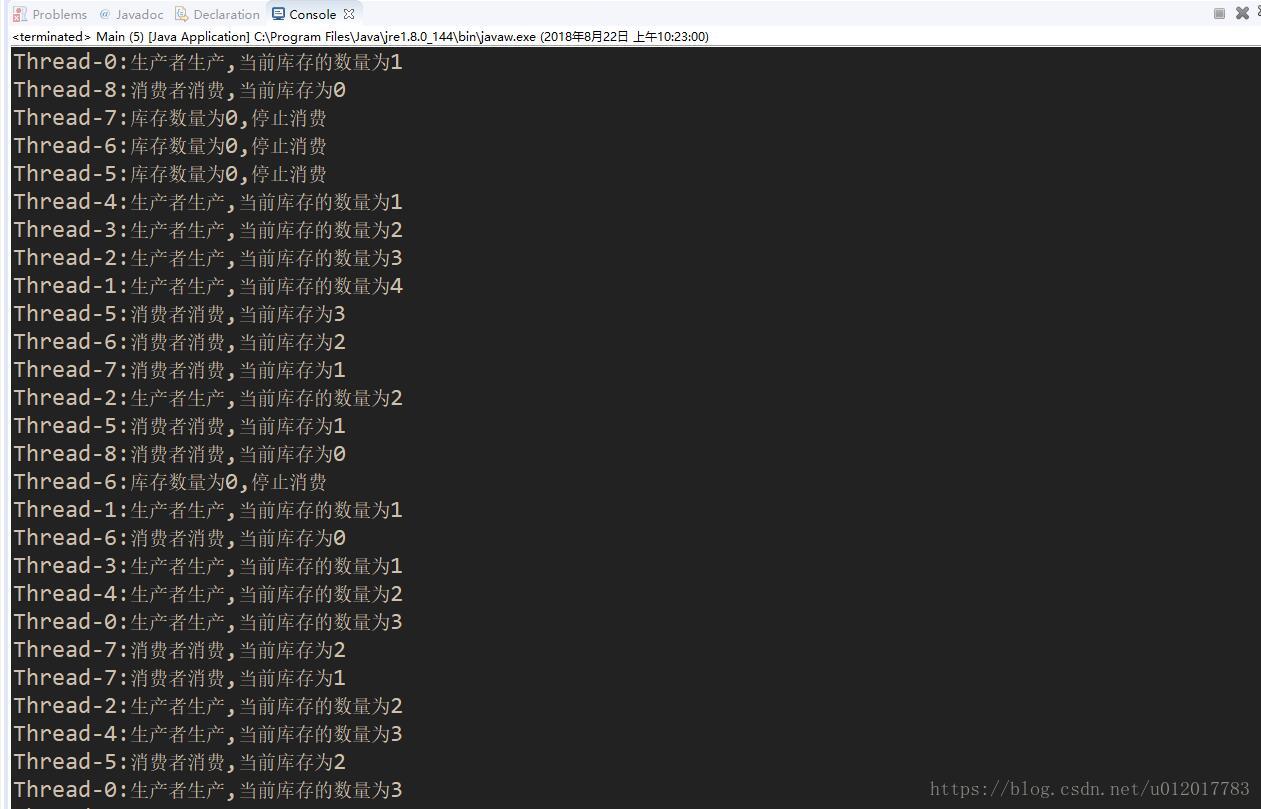