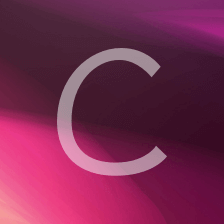
Linked List
文章平均质量分 78
豆腐脑是咸的
这个作者很懒,什么都没留下…
展开
-
Reverse Nodes in k-Group (Java)
Given a linked list, reverse the nodes of a linked list k at a time and return its modified list.If the number of nodes is not a multiple of k then left-out nodes in the end should remain as it is原创 2015-01-29 16:14:10 · 363 阅读 · 0 评论 -
Remove Duplicates from Sorted List II (Java)
Given a sorted linked list, delete all nodes that have duplicate numbers, leaving only distinct numbers from the original list.For example,Given 1->2->3->3->4->4->5, return 1->2->5.Given 1->1-原创 2015-01-01 19:50:39 · 350 阅读 · 0 评论 -
Reorder List (Java)
Given a singly linked list L: L0→L1→…→Ln-1→Ln,reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→…You must do this in-place without altering the nodes' values.For example,Given {1,2,3,4}, reorder it to原创 2015-01-01 16:55:08 · 287 阅读 · 0 评论 -
Insertion Sort List (Java)
Sort a linked list using insertion sort.写的有点乱,有时间再写一遍Source/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode(int x) { *原创 2015-01-02 20:11:29 · 330 阅读 · 0 评论 -
Rotate List (Java)
Given a list, rotate the list to the right by k places, where k is non-negative.For example:Given 1->2->3->4->5->NULL and k = 2,return 4->5->1->2->3->NULL.注意:这题是rotate 就像是把链表首尾相连,然后向右翻转,所以原创 2015-01-01 15:47:41 · 435 阅读 · 0 评论 -
Linked List Cycle II (Java)
Given a linked list, return the node where the cycle begins. If there is no cycle, return null.Follow up:Can you solve it without using extra space?先用一个指针走一步,另一个指针走两步的方法判断是否存在回路,如果存在,则将其中一个指原创 2015-01-01 21:20:35 · 334 阅读 · 0 评论 -
Sort List (Java)
Sort a linked list in O(n log n) time using constant space complexity.学习了网上的帖子,用归并排序,注意空间复杂度超了,记得重做Source/** * Definition for singly-linked list. * class ListNode { * int val; * Li原创 2015-01-01 14:30:09 · 316 阅读 · 0 评论 -
Swap Nodes in Pairs (Java)
Given a linked list, swap every two adjacent nodes and return its head.For example,Given 1->2->3->4, you should return the list as 2->1->4->3.Your algorithm should use only constant space. Y原创 2015-01-01 16:19:27 · 435 阅读 · 0 评论 -
Linked List Cycle (Java)
Given a linked list, determine if it has a cycle in it.Follow up:Can you solve it without using extra space?设两个指针,一个走两步,一个走一步,如果有环,快的一定会和慢的汇合Source/** * Definition for singly-linked原创 2015-01-01 20:46:45 · 316 阅读 · 0 评论 -
Partition List (Java)
Given a linked list and a value x, partition it such that all nodes less than x come before nodes greater than or equal to x.You should preserve the original relative order of the nodes in each of原创 2015-01-01 20:32:18 · 501 阅读 · 0 评论 -
Merge k Sorted Lists (Java)
Merge k sorted linked lists and return it as one sorted list. Analyze and describe its complexity.merge two sorted lists那道题的升级版。Source/** * Definition for singly-linked list. * public class原创 2015-02-06 18:49:26 · 388 阅读 · 0 评论 -
Merge Two Sorted Lists (Java)
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists.Source原创 2014-10-29 20:54:39 · 429 阅读 · 0 评论 -
Intersection of Two Linked Lists (Java)
Write a program to find the node at which the intersection of two singly linked lists begins.For example, the following two linked lists:A: a1 → a2 ↘原创 2014-12-22 16:43:13 · 382 阅读 · 0 评论 -
Reverse Linked List II (Java)
Reverse a linked list from position m to n. Do it in-place and in one-pass.For example:Given 1->2->3->4->5->NULL, m = 2 and n = 4,return 1->4->3->2->5->NULL.Note:Given m, n satisfy t原创 2014-12-29 22:27:15 · 330 阅读 · 0 评论 -
Remove Nth Node From End of List (Java)
Given a linked list, remove the nth node from the end of list and return its head.For example, Given linked list: 1->2->3->4->5, and n = 2. After removing the second node from the end, the原创 2014-10-27 21:36:56 · 317 阅读 · 0 评论 -
Remove Duplicates from Sorted List (Java)
Given a sorted linked list, delete all duplicates such that each element appear only once.For example,Given 1->1->2, return 1->2.Given 1->1->2->3->3, return 1->2->3.Source/** * Defini原创 2014-12-25 18:41:07 · 354 阅读 · 0 评论 -
Add Two Numbers (Java)
You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a link原创 2014-12-26 18:15:47 · 459 阅读 · 0 评论 -
Copy List with Random Pointer (Java)
A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null.Return a deep copy of the list.这道题和clone graph那道题不同的地方在于,图可以d原创 2015-01-29 19:51:33 · 451 阅读 · 0 评论 -
Convert Sorted List to Binary Search Tree
Given a singly linked list where elements are sorted in ascending order, convert it to a height balanced BST.和Convert Sorted Array to Binary Search Tree的区别是array可以用二分法,而list没法直接算mid。这时可用双指针,一个走快一个走慢原创 2015-01-21 15:55:53 · 402 阅读 · 0 评论