Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 46827 | Accepted: 20042 |
Description
- Choose any one of the 16 pieces.
- Flip the chosen piece and also all adjacent pieces to the left, to the right, to the top, and to the bottom of the chosen piece (if there are any).
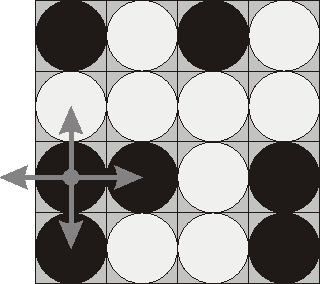
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
Input
Output
Sample Input
bwwb bbwb bwwb bwww
Sample Output
4
题意:变化颜色使所有的颜色全为黑色或白色。
思路:扣了几天才做出来,最近还是要刷基础题和简单算法题把基础做好啊。
枚举所有情况,翻转一个的情况,两个的情况,三个。。。
代码:
#include<stdio.h>
char map[5][5];
int mp[5][5];
int vis[4][4];
int mark;
int temp;
int flag = 0;
void fz(int x,int y)
{
mp[x][y] = !mp[x][y];
if (x - 1 >= 0)
mp[x - 1][y] = !mp[x - 1][y];
if (x + 1 < 4)
mp[x + 1][y] = !mp[x + 1][y];
if (y - 1 >= 0)
mp[x][y - 1] = !mp[x][y - 1];
if (y + 1 < 4)
mp[x][y + 1] = !mp[x][y + 1];
}
bool judge()
{
int t = mp[0][0];
for (int i = 0; i < 4; i++)
for (int j = 0; j < 4; j++)
{
if (mp[i][j] != t)return 0;
}
return 1;
}
void dfs(int x, int y,int num)
{
if (judge())flag = 1;
for (int i = x; i < 4; i++)
for (int j = y; j < 4; j++)
{
if (flag)return;
fz(i, j);
if (judge())flag = 1;
temp = num + 1;
if (temp < mark)
{
if (j < 3)
dfs(i, j + 1, temp);
else if (i < 3)
dfs(i + 1, 0, temp);
}
fz(i, j);
}
}
int main()
{
for (int i = 0; i < 4; i++)
{
scanf("%s", map[i]);
for (int j = 0; j < 4; j++)
{
if (map[i][j] == 'b')
mp[i][j] = 0;
if (map[i][j] == 'w')
mp[i][j] = 1;
}
}
mark = 1;
for (int i = 1; i <= 16; i++)
{
dfs(0, 0, 0);
mark++;
if (flag)break;
}
if(flag)printf("%d\n", temp);
else printf("Impossible\n");
}