tf的张量排序
1. 全排序(将所有元素排序)
- sort用于全排序,argsort用于获得全排序后元素的索引
- sort(a,direction=’…’)和argsort(a,direction=’…’)
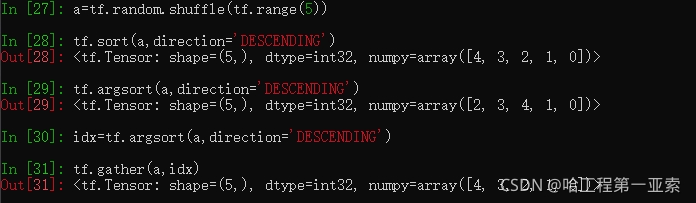
- 多维情况
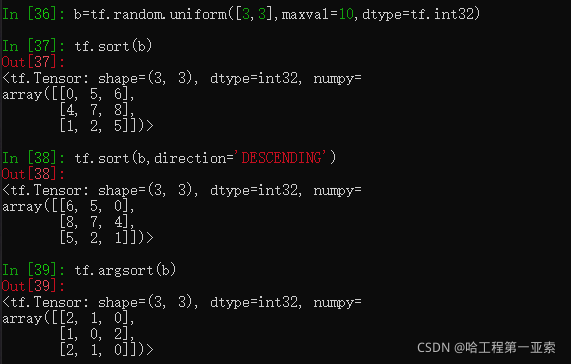
2. 部分排序(取最大的几位)
- tf.math.top_k(a,x) 取a中的最大x位,可以用indices和values去除最大x位的索引和值
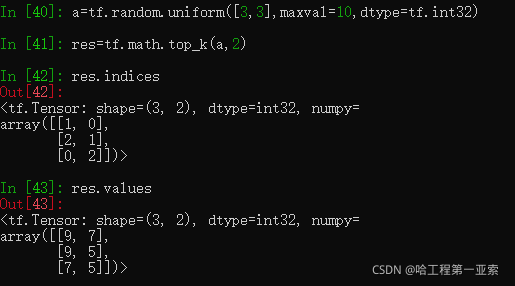
3. top_k accuracy
- 计算前几位的准确率,如最大可能的几个结果中有正确结果的概率。
- 示例:
import tensorflow as tf
import os
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2'
tf.random.set_seed(2467)
def accuracy(output, target, topk=(1,)):
maxk = max(topk)
batch_size = target.shape[0]
pred = tf.math.top_k(output, maxk).indices
pred = tf.transpose(pred, perm=[1, 0])
target_temp = tf.broadcast_to(target, pred.shape)
correct = tf.equal(pred, target_temp)
res = []
for k in topk:
correct_k = tf.cast(tf.reshape(correct[:k], [-1]), dtype=tf.float32)
correct_k = tf.reduce_sum(correct_k)
accuracy_value = float(correct_k * (100.0/batch_size))
res.append(accuracy_value)
return res
output = tf.random.normal([10, 6])
output = tf.math.softmax(output, axis=1)
target = tf.random.uniform([10], maxval=6, dtype=tf.int32)
pred = tf.argmax(output, axis=1)
accuracy_value = accuracy(output, target, topk=(1, 2, 3, 4, 5, 6))
print('原始概率:', output.numpy())
print('预测值:', pred.numpy())
print('正确值:', target.numpy())
print('取前1-6概率最大值对应的正确率:', accuracy_value)