题目
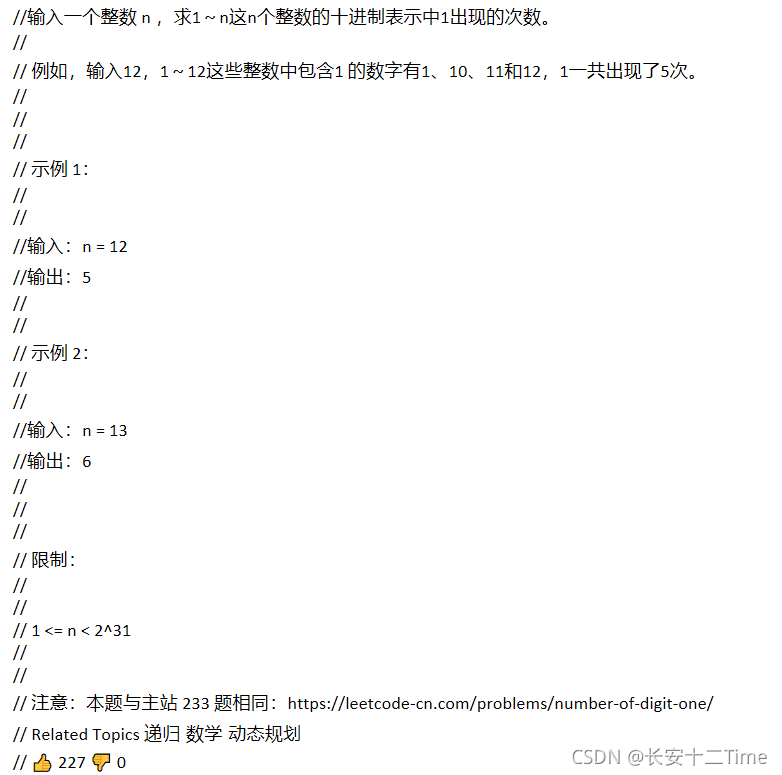
思路
1.最高位1出现的次数
如果最高位为1,那么出现次数就是后4位的值 + 1
不为1,那么出现次数就是10^(10的后4位)
2.后几位出现的1的次数,最高位的值 * 后几位的排列组合(位数 * 10^位数-1 )
题解
class Solution {
public int countDigitOne(int n) {
return getCount(String.valueOf(n), 0);
}
private int getCount(String str, int count) {
if (str == null || Integer.valueOf(str) == 0) {
return count;
}
if (Integer.valueOf(str) < 10) {
return count + 1;
}
int length = str.length();
int first = str.charAt(0) - '0';
int highCount = 0;
int lowCount = 0;
if (first == 0) {
return getCount(str.substring(1), count);
} else if (first == 1) {
highCount = Integer.valueOf(str.substring(1)) + 1;
} else {
highCount = Double.valueOf(Math.pow(10, length - 1)).intValue();
}
lowCount = first * (length - 1) * Double.valueOf(Math.pow(10, length - 2)).intValue();
count += (highCount + lowCount);
return getCount(str.substring(1), count);
}
}