1:Array
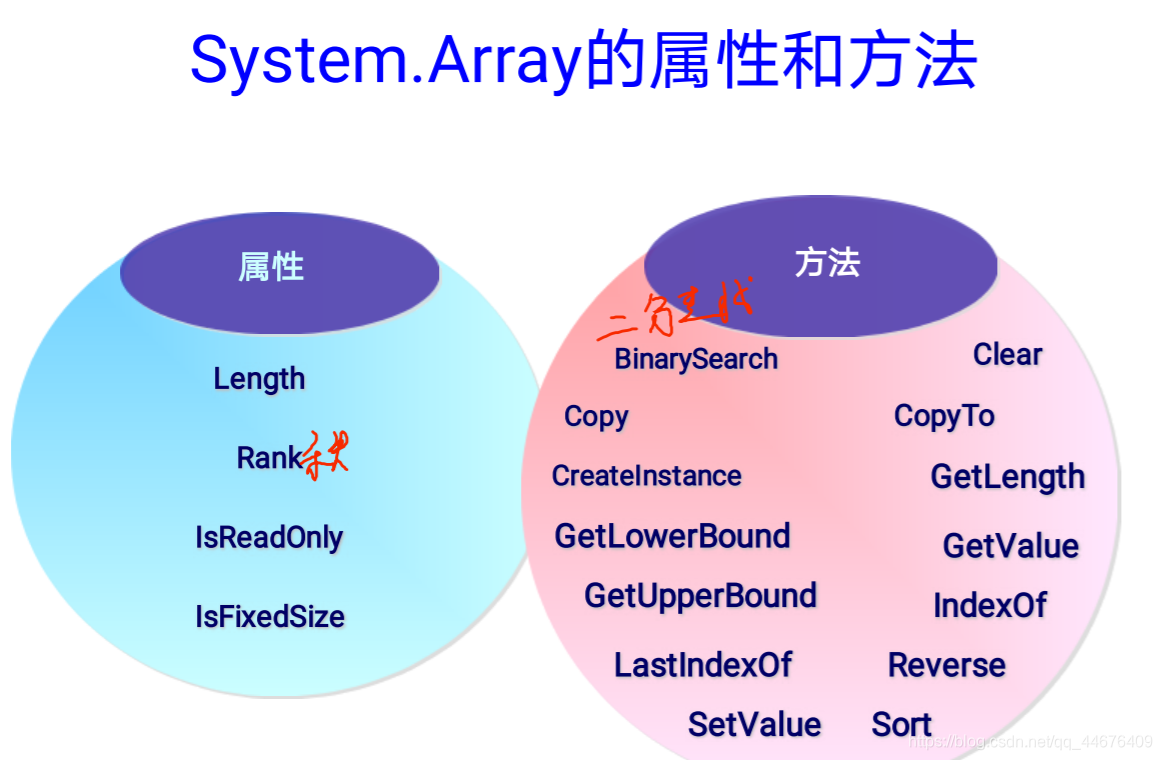
using System;
namespace test
{
public class test
{
public static void Main(string[] args)
{
Array objNames = Array.CreateInstance(typeof(string), 5);
objNames.SetValue("A",0);
objNames.SetValue("B",1);
objNames.SetValue("C",2);
objNames.SetValue("D",3);
objNames.SetValue("E",4);
Console.WriteLine("数组值");
for (int ctr = 0; ctr < 5; ctr++)
{
Console.WriteLine("元素{0}:{1}",ctr + 1,objNames.GetValue(ctr));
}
Console.WriteLine(objNames.Rank);
Console.WriteLine(objNames.IsReadOnly);
Console.WriteLine(objNames.IsFixedSize);
Console.WriteLine(Array.BinarySearch(objNames,"A"));
Array.Reverse(objNames);
Array.Sort(objNames);
for (int ctr = 0; ctr < 5; ctr++)
{
Console.WriteLine("元素{0}:{1}", ctr + 1, objNames.GetValue(ctr));
}
Console.WriteLine("Array.IndexOf(objNames,A)"+ Array.IndexOf(objNames, "A"));
Console.ReadLine();
}
}
}
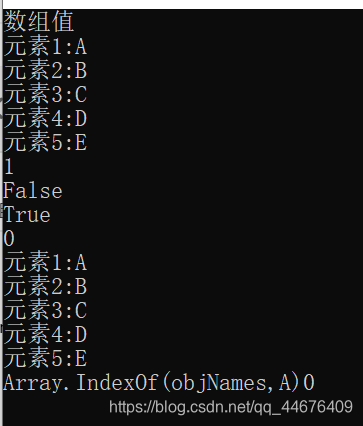
using System;
using System.Collections;
namespace test
{
public class Chair
{
public double myPrice;
public string myVendor, myID;
public Chair() { }
public Chair(double price, string vendor, string sku)
{
myPrice = price;
myVendor = vendor;
myID = sku;
}
}
public class MyCompareClass : IComparer
{
public int Compare(Object x, Object y)
{
if (x is Chair && y is Chair)
{
Chair castObjX = (Chair)x;
Chair castObjY = (Chair)y;
if (castObjX.myPrice > castObjY.myPrice) return 1;
else if (castObjX.myPrice < castObjY.myPrice) return -1;
else return 0;
}
return 666;
}
}
public class Test
{
public static void Main(string[] args)
{
Chair[] chairs = new Chair[4];
chairs[0] = new Chair(150.0, "Lane", "99-88");
chairs[1] = new Chair(250.0, "Lane", "99-00");
chairs[2] = new Chair(100.0, "Lane", "98-88");
chairs[3] = new Chair(120.0, "Harris", "93-9");
Array.Sort(chairs, new MyCompareClass());
foreach (Chair c in chairs)
{
Console.WriteLine(c.myPrice + " " + c.myVendor + " " + c.myID);
}
Console.ReadLine();
}
}
}
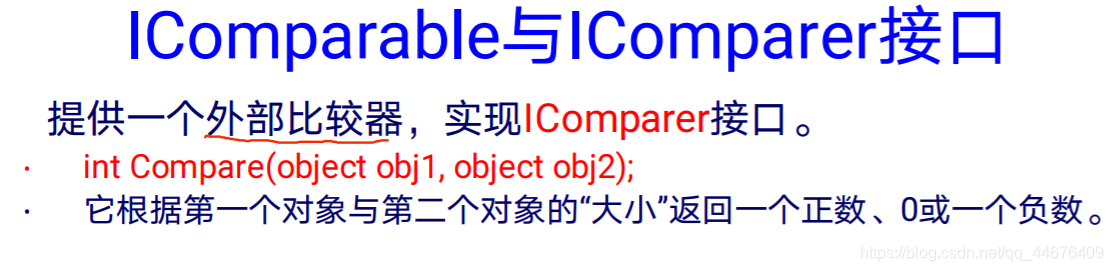