1.dao层实现
public interface StudentDaoInte {
List<Student> selectCondition(Map<String, String> whereMap);
}
@Override
//条件查询
public List<Student> selectCondition(Map<String, String> whereMap) {
//用来 存储 合法 的 参数的值
List<String> list = new ArrayList<>();
//用来 拼接sql 语句的where条件
StringBuilder where = new StringBuilder();
//遍历迭代 whereMap
Set<String> keySet = whereMap.keySet();
Iterator<String> its = keySet.iterator();
while(its.hasNext()){
String key = its.next();//键:字段
String val = whereMap.get(key);//值: 字段值
//判断是否为空,空字符串和 0
if(val != null && !"".equals(val.trim()) && !"0".equals(val.trim())){
//对 name 进行模糊查询
if("name".equals(key)){
where.append(" and "+key+" like ?");
list.add("%"+val+"%");
}else{
//拼接条件
where.append(" and "+key+"=?");
list.add(val);
}
}
}
//为了 让 拼接 sql 从and 开始,加 1=1 恒成立条件
String sql = "select * from students where 1=1"+where;
BeanListHandler<Student> handler = new BeanListHandler<Student>(Student.class);
//将 list 合法参数 转换 成 对象数组
Object[] args = list.toArray();
return DBUtil.select(sql,handler,args);
}
2.entity层实现
3.service层实现
// 按条件查询
List<Student> queryCondition(Map<String, String> whereMap);
@Override
public List<Student> queryCondition(Map<String, String> whereMap) {
return sdi.selectCondition(whereMap);
}
4.servlet层实现
case "search":
String sno = req.getParameter("sno");
String name = req.getParameter("name");
String class_id = req.getParameter("class_id");
Map<String ,String> whereMap = new HashMap<>();
whereMap.put("id",sno);
whereMap.put("name",name);
whereMap.put("class_id",class_id);
List<Student> list = ssi.queryCondition(whereMap);
req.setAttribute("list",list);
List<Classes> clz_list = csi.queryAll();
req.setAttribute("clz_list",clz_list);
req.setAttribute("where",whereMap);
req.getRequestDispatcher("/WEB-INF/sms/stu/list.jsp").forward(req,resp);
break;
default:break;
}
private Map<String, String> getParameterMap(HttpServletRequest req) {
DiskFileItemFactory diskFileItemFactory = new DiskFileItemFactory();
FileUpload fileUpload = new FileUpload(diskFileItemFactory);
Map<String,String> map = new HashMap<>();
try {
List<FileItem> list = fileUpload.parseRequest(req);
for (FileItem fileItem : list) {
if(fileItem.isFormField()){//判断是否是 普通的表单项
map.put(fileItem.getFieldName(),new String(fileItem.getString().getBytes("ISO8859-1"),"utf-8"));
map.put(fileItem.getFieldName(),fileItem.getString());
}else{//说明是 文件上传的表单项,处理 上传文件,将上传文件 保存到服务器的硬盘中
//拼接路径
String path = this.getServletContext().getRealPath("/")+"upload"+ File.separator;
String fileName = UUID.randomUUID().toString();
String suffix = fileItem.getName().substring(fileItem.getName().lastIndexOf('.'));
//TODO 验证 大小、类型 等是否符合要求
// System.out.println(path+fileName+suffix);
File file = new File(path+fileName+suffix);
fileItem.write(file);
//将 路径 存储到 map ,以再便存到数据库里
map.put(fileItem.getFieldName(),"/upload/"+fileName+suffix);
}
}
} catch (FileUploadException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
return map;
}
5.utils层实现
public static <T> List<T> select(String sql, BeanListHandler<T> beanListHandler, Object... args){
List<T> list = null;
try {
list = runner.query(sql,beanListHandler,args);
} catch (SQLException e) {
e.printStackTrace();
}
return list;
}
6.效果图
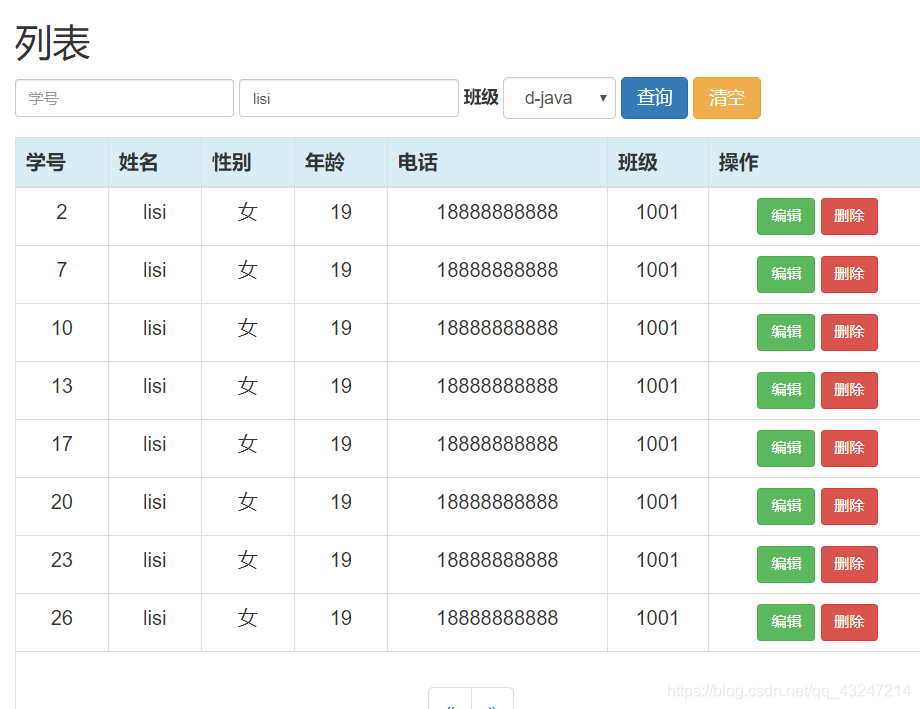