目录:
Map集合
HashMap和Hashtable的区别
Collections(集合工具类)
集合练习
模拟斗地主(洗牌,发牌,看牌)
Map集合概述和特点
A: 需求: 根据学号获取学生姓名
B:Map接口概述
查看API可以知道:
将键映射到值的对象
一个映射不能包含重复的键
每个键最多只能映射到一个值
C:Map接口和Collection接口的不同
Map是双列的,Collection是单列的
Map的键唯一,Collection的子体系Set是唯一的
Map集合的数据结构针对键有效,跟值无关;Collection集合的数据结构是针对元素有效
Map集合的功能概述
A:Map集合的功能概述
a:添加功能
V put(K key,V value):添加元素。这个其实还有另一个功能?替换
如果键是第一次存储,就直接存储元素,返回null
如果键不是第一次存在,就用值把以前的值替换掉,返回以前的值
b:删除功能
void clear():移除所有的键值对元素
V remove(Object key):根据键删除键值对元素,并把值返回
c:判断功能
boolean containsKey(Object key):判断集合是否包含指定的键
boolean containsValue(Object value):判断集合是否包含指定的值
boolean isEmpty():判断集合是否为空
d:获取功能
Set<Map.Entry<K,V>> entrySet(): 返回一个键值对的Set集合
V get(Object key):根据键获取值
Set<K> keySet():获取集合中所有键的集合
Collection<V> values():获取集合中所有值的集合
e:长度功能
int size():返回集合中的键值对的对数
Map集合的基本功能测试
A:案例演示
Map集合的基本功能
V put(K key,V value)//键相同 值覆盖
V remove(Object key)
void clear()
boolean containsKey(Object key)
boolean containsValue(Object value)
boolean isEmpty()
int size()
Map集合的获取功能测试
A:案例演示
V get(Object key)
Set<K> keySet()
Collection<V> values()
HashMap<String, String> map = new HashMap<>();
map.put("文章", "马伊琍");
map.put("贾乃亮", "李小璐");
map.put("陈思成", "佟丽娅");
map.put("大郎", "金莲");
//跟判断相关 的方法
//判断有没有这个键
boolean b = map.containsKey("文章");
//判断有没有这个值
boolean b1 = map.containsValue("李小璐2");
System.out.println(b);
System.out.println(b1);
System.out.println(map);
Map集合的遍历之键找值
A:键找值思路:
获取所有键的集合
遍历键的集合,获取到每一个键
根据键找值
B:案例演示
Map集合的遍历之键找值
public static void main(String[] args) {
HashMap<String, String> map = new HashMap<>();
map.put("文章", "马伊琍");
map.put("贾乃亮", "李小璐");
map.put("陈思成", "佟丽娅");
map.put("大郎", "金莲");
键找值
//String value = map.get("文章");
//System.out.println(value);
//遍历 键找值
//获取所有键的集合
Set<String> keySet = map.keySet();
//遍历键集 键找值
for (String key : keySet) {
System.out.println(key+"=="+map.get(key));
}
System.out.println("--------------------------");
//获取所有值的集合
Collection<String> values = map.values();
System.out.println(values);
}
Map集合的遍历之键值对对象找键和值
A:键值对对象找键和值思路:
获取所有键值对对象的集合
遍历键值对对象的集合,获取到每一个键值对对象
根据键值对对象找键和值
B:案例演示
Map集合的遍历之键值对对象找键和值
public static void main(String[] args) {
//Map集合的数据结构,只跟键有关
TreeMap<Student, Integer> treeMap = new TreeMap<>();
treeMap.put(new Student("zhangsan",1),1);
treeMap.put(new Student("zhangsan", 10), 1);
treeMap.put(new Student("zhangsan", 12), 1);
treeMap.put(new Student("zhangsan", 122), 1);
treeMap.put(new Student("zhangsan", 144), 1);
treeMap.put(new Student("zhangsan", 109), 1);
treeMap.put(new Student("zhangsan", 156), 1);
treeMap.put(new Student("zhangsan", 124), 1);
treeMap.put(new Student("zhangsan", 124), 1);
Set<Map.Entry<Student, Integer>> entries = treeMap.entrySet();
for (Map.Entry<Student, Integer> en : entries) {
Student key = en.getKey();
Integer value = en.getValue();
System.out.println(key.getName()+"=="+key.getAge()+"======="+value);
}
}
Map集合遍历的两种方式比较图解
A:画图演示: Map集合遍历的两种方式比较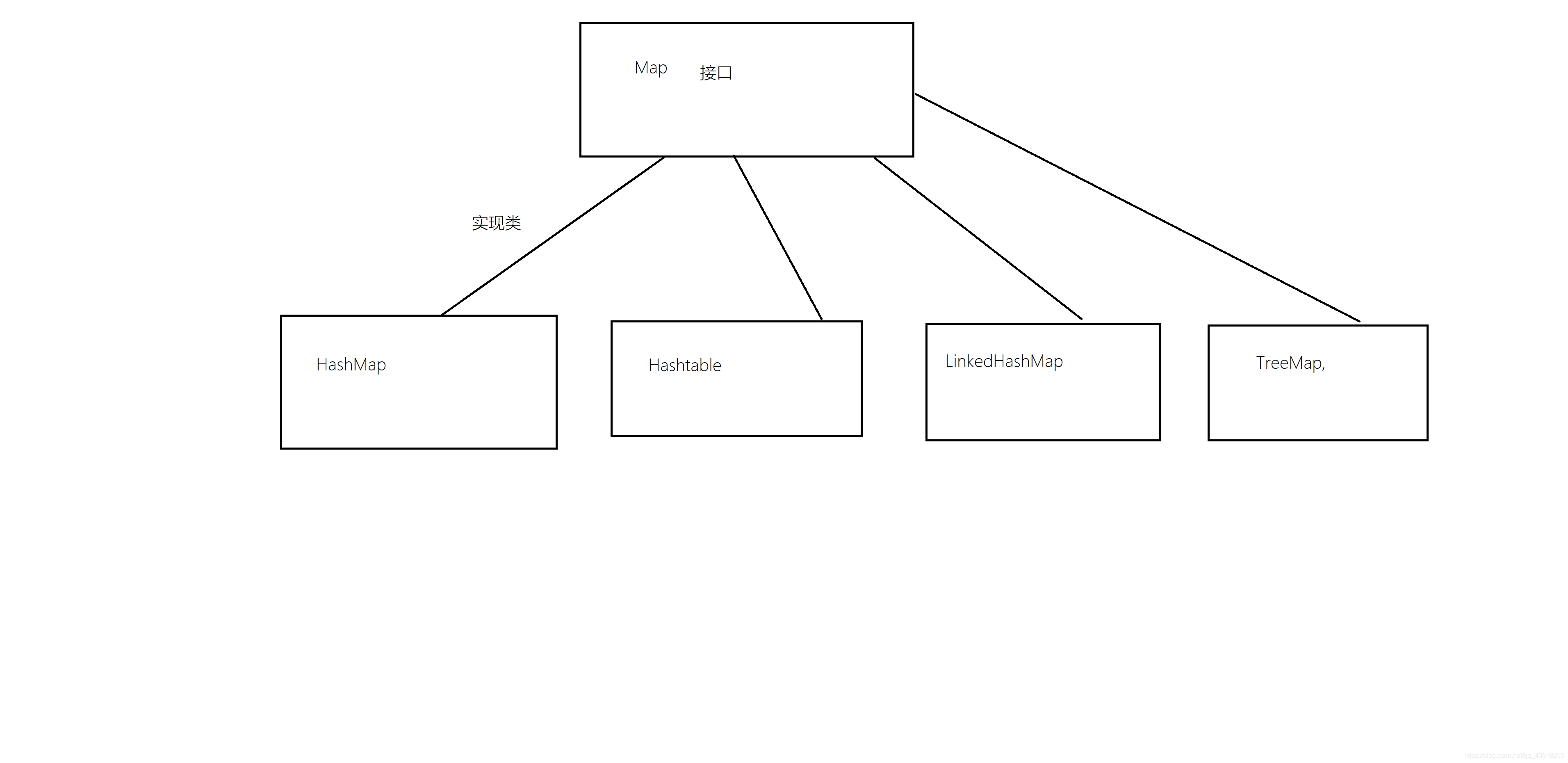
HashMap集合键是Stirng值是String的案例
HashMap 允许插入null键 null值
A:案例演示: HashMap集合键是Stirng值是String的案例
public static void main(String[] args) {
//所有Map集合的数据结构,只跟键有关,跟值没关系,一个键只能映射一个值,键相同,值覆盖
HashMap<String, String> map = new HashMap<>();
//当我们第一次存储键值对 数据时,返回的时null
//当我们再次存储一个键相同的数据时,会覆盖掉旧值,返回值,上一次这个键所对应的旧值
String str = map.put("文章", "马伊琍");
//键相同,值覆盖
String str2 = map.put("文章", "姚笛");
map.put("贾乃亮","李小璐");
map.put("陈思成","佟丽娅");
map.put("大郎","金莲");
System.out.println(str);
System.out.println(str2);
System.out.println(map);
}
HashMap集合键是String值是Student的案例
A:案例演示: HashMap集合键是String值是Student的案例
public static void main(String[] args) {
//存储键是String 类型 值Student类型
HashMap<String, Student> hashMap = new HashMap<>();
hashMap.put("s001",new Student("张三",23));
hashMap.put("s001", new Student("李四", 24));
hashMap.put("s002", new Student("王五", 25));
hashMap.put("s003", new Student("赵六", 26));
System.out.println(hashMap);
}
HashMap集合键是Student值是String的案例
键唯一 注意重写hashCode方法 和 equals 方法
A:案例演示: HashMap集合键是Student值是String的案例
public static void main(String[] args) {
//存键是Student 类型值 String 类型
//Map集合的数据结构,只跟键有关,跟值没关系
// new HashSet().add("abc");
HashMap<Student, String> hashMap = new HashMap<>();
hashMap.put(new Student("张三", 23),"s001");
hashMap.put(new Student("张三", 23), "s002");
hashMap.put(new Student("李四", 23), "s003");
hashMap.put(new Student("王五", 23), "s004");
System.out.println(hashMap);
}
LinkedHashMap的概述和使用
A:LinkedHashMap的概述: Map 接口的哈希表和链接列表实现,具有可预知的迭代顺序
B:LinkedHashMap的特点: 底层的数据结构是链表和哈希表 元素有序 并且唯一
元素的有序性由链表数据结构保证 唯一性由 哈希表数据结构保证
Map集合的数据结构只和键有关
C:案例演示: LinkedHashMap的特点
public static void main(String[] args) {
//键唯一且有序,链表保证了键有序,哈希表保证了键唯一
LinkedHashMap<Integer, String> linkedHashMap = new LinkedHashMap<>();
linkedHashMap.put(10,"abc");
linkedHashMap.put(20, "abc");
linkedHashMap.put(30, "abc");
linkedHashMap.put(40, "abc");
linkedHashMap.put(50, "abc");
linkedHashMap.put(60, "abc");
linkedHashMap.put(70, "abc");
linkedHashMap.put(70, "abcdddd");
System.out.println(linkedHashMap);
}
TreeMap集合键是String值是String的案例
A: TreeMap: 键的数据结构是红黑树,可保证键的排序和唯一性
排序分为自然排序和比较器排序
线程是不安全的效率比较高
TreeMap 键不允许插入null
B:案例演示: TreeMap集合键是Integer值是String的案例
TreeMap集合键是Student值是String的案例
A:案例演示: TreeMap集合键是Student值是String的案例
按照年龄大小进行排序
注意键要实现Comparable 接口
统计字符串中每个字符出现的次数
A:案例演示: 需求:统计字符串中每个字符出现的次数
"aababcabcdabcde",获取字符串中每一个字母出现的次数要求结果:a(5)b(4)c(3)d(2)e(1)
public static void main(String[] args) {
//A:
//案例演示:
//需求:统计字符串中每个字符出现的次数
//"aababcabcdabcde", 获取字符串中每一个字母出现的次数要求结果:a(5) b(4) c(3) d(2) e(1)
Scanner scanner = new Scanner(System.in);
System.out.println("请随便输入一行字符");
String line = scanner.nextLine();
HashMap<Character, Integer> hashMap = new HashMap<>();
for (int i = 0; i < line.length(); i++) {
//截取字符,作为键,存到集合中
char ch = line.charAt(i);
if(!hashMap.containsKey(ch)){
hashMap.put(ch,1);
}else{
Integer integer = hashMap.get(ch);
integer++;
hashMap.put(ch,integer); //键相同,值覆盖
}
}
StringBuilder sb = new StringBuilder();
Set<Character> keySet = hashMap.keySet();
for (Character key : keySet) {
sb.append(key).append("(").append(hashMap.get(key)).append(")");
}
String s = sb.toString();
System.out.println(s);
}
集合嵌套之HashMap嵌套HashMap
A:案例演示
集合嵌套之HashMap嵌套HashMap
基础班
张三 20
李四 22
就业班
王五 21
赵六 23
public static void main(String[] args) {
HashMap<String, Integer> jcMap = new HashMap<>();
jcMap.put("张三",20);
jcMap.put("李四",22);
HashMap<String, Integer> jyMap = new HashMap<>();
jyMap.put("王五", 21);
jyMap.put("赵六", 23);
HashMap<String, HashMap<String, Integer>> maxMap = new HashMap<>();
maxMap.put("基础班",jcMap);
maxMap.put("就业班",jyMap);
//遍历集合
Set<Map.Entry<String, HashMap<String, Integer>>> entries = maxMap.entrySet();
for (Map.Entry<String, HashMap<String, Integer>> en : entries) {
String key = en.getKey();
System.out.println(key);
HashMap<String, Integer> value = en.getValue();
Set<Map.Entry<String, Integer>> entries1 = value.entrySet();
for (Map.Entry<String, Integer> entry : entries1) {
String key1 = entry.getKey();
Integer value1 = entry.getValue();
System.out.println("\t"+key1+"\t"+value1);
}
System.out.println();
}
}
集合嵌套之HashMap嵌套ArrayList
A:案例演示
集合嵌套之HashMap嵌套ArrayList
假设HashMap集合的元素是ArrayList。有3个。
每一个ArrayList集合的值是字符串。
三国演义
吕布
周瑜
笑傲江湖
令狐冲
林平之
神雕侠侣
郭靖
杨过
public static void main(String[] args) {
ArrayList<String> sgList = new ArrayList<>();
sgList.add("吕布");
sgList.add("周瑜");
ArrayList<String> xaList = new ArrayList<>();
xaList.add("令狐冲");
xaList.add("林平之");
ArrayList<String> sdList = new ArrayList<>();
sdList.add("郭靖");
sdList.add("杨过");
HashMap<String, ArrayList<String>> listHashMap = new HashMap<>();
listHashMap.put("三国演义",sgList);
listHashMap.put("笑傲江湖", xaList);
listHashMap.put("神雕侠侣", sdList);
//遍历集合
Set<Map.Entry<String, ArrayList<String>>> entries = listHashMap.entrySet();
for (Map.Entry<String, ArrayList<String>> entry : entries) {
String key = entry.getKey();
System.out.println(key);
ArrayList<String> value = entry.getValue();
for (String s : value) {
System.out.println("\t"+s);
}
System.out.println();
}
集合嵌套之ArrayList嵌套HashMap
A:案例演示
集合嵌套之ArrayList嵌套HashMap
假设ArrayList集合的元素是HashMap。有3个。
每一个HashMap集合的键和值都是字符串。
周瑜---小乔
吕布---貂蝉
郭靖---黄蓉
杨过---小龙女
令狐冲---任盈盈
林平之---岳灵珊
public static void main(String[] args) {
HashMap<String, String> sgMap = new HashMap<>();
sgMap.put("周瑜","小乔");
sgMap.put("吕布","貂蝉");
HashMap<String, String> sdMap = new HashMap<>();
sdMap.put("郭靖", "黄蓉");
sdMap.put("杨过", "小龙女");
HashMap<String, String> xaMap = new HashMap<>();
xaMap.put("令狐冲", "任盈盈");
xaMap.put("林平之", "岳灵珊");
ArrayList<HashMap<String, String>> maxList = new ArrayList<>();
maxList.add(sgMap);
maxList.add(sdMap);
maxList.add(xaMap);
//遍历
for (HashMap<String, String> hm : maxList) {
Set<Map.Entry<String, String>> entries = hm.entrySet();
for (Map.Entry<String, String> entry : entries) {
String key = entry.getKey();
String value = entry.getValue();
System.out.println(key+"-----"+value);
}
System.out.println();
}
}
HashMap和Hashtable的区别
A:面试题
HashMap和Hashtable的区别: 查看API可以知道
HashMap: 线程不安全,效率高.允许null值和null键
Hashtable: 线程安全 , 效率低.不允许null值和null键
B:案例演示
HashMap和Hashtable的区别
Collections工具类的概述和常见方法讲解
A:Collections类概述: 针对集合操作 的工具类
B:Collections成员方法
public static <T> void sort(List<T> list): 排序,默认按照自然顺序
public static <T> int binarySearch(List<?> list,T key): 二分查找
public static <T> T max(Collection<?> coll): 获取最大值
public static void reverse(List<?> list): 反转
public static void shuffle(List<?> list): 随机置换
C:案例演示: Collections工具类的常见方法讲解
模拟斗地主洗牌和发牌
A:案例演示: 模拟斗地主洗牌和发牌,牌没有排序
###18.21_集合框架(模拟斗地主洗牌和发牌并对牌进行排序的原理图解)(理解)
A:画图演示: 画图说明排序原理
###18.22_集合框架(模拟斗地主洗牌和发牌并对牌进行排序的代码实现)(理解)
A:案例演示: 模拟斗地主洗牌和发牌并对牌进行排序的代码实现
public static void main(String[] args) {
HashMap<Integer, String> map = new HashMap<>();
String[] colos = {"♥", "♠", "♦", "♣"};
String[] nums = {"A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"};
int i=0;
for (String color : colos) {
for (String num : nums) {
String poker = color.concat(num);
map.put(i,poker);
i++;
}
}
map.put(52,"?");
map.put(53,"☀");
ArrayList<Integer> list = new ArrayList<>();
for (int j = 0; j < 54; j++) {
list.add(j);
}
Collections.shuffle(list);
Collections.shuffle(list);
Collections.shuffle(list);
TreeSet<String> 高进 = new TreeSet<>();
TreeSet<String> 星仔 = new TreeSet<>();
TreeSet<String> 刀仔 = new TreeSet<>();
TreeSet<String> 底牌 = new TreeSet<>();
for (int k = 0; k < list.size(); k++) {
if(k>=list.size()-3){
底牌.add(map.get(k));
}else if(k%3==0){
高进.add(map.get(k));
} else if (k % 3 == 1) {
星仔.add(map.get(k));
}else {
刀仔.add(map.get(k));
}
}
lookpoker("高进",高进);
lookpoker("星仔",星仔);
lookpoker("刀仔",刀仔);
lookpoker("底牌",底牌);
}
private static void lookpoker(String name, TreeSet<String> list) {
System.out.println(name);
for (String poker : list) {
System.out.print(poker+"\t");
}
System.out.println();
}