原栈
class Stack{
int top;
int[] elem;
public Stack(){
this(10);
}
public Stack(int size){
this.elem = new int[size];
this.top = 0;
}
/**
* 栈是否已满
*/
public boolean isFull(){
if(this.top == this.elem.length){
return true;
}
return false;
}
/**
* 入栈
*/
public boolean push(int val){
if(isFull()){
return false;
}
this.elem[top++] = val;
return true;
}
/**
* 是否为空
*/
public boolean isEmpty(){
if(this.top == 0){
return true;
}
return false;
}
/**
* 出栈
*/
public int pop(){
if(isEmpty()){
return -1;
}
return elem[--this.top];
}
/**
* 得到栈的元素
*/
public int getTop(){
if(isEmpty()){
return -1;
}
return this.elem[this.top-1];
}
/**
* 打印栈内元素
*/
public void show(){
for(int i =0;i<this.top;i++){
System.out.println(this.elem[i]+" ");
}
System.out.println();
}
}
/**
*
* @author jhl
*
*/
public class Test3stack {
public static void main(String[] args) {
Stack s1 = new Stack();
int length = 10;
for(int i = 0;i<length;i++){
s1.push(i);
}
s1.show();
}
}
泛型栈
class Stack<T>{
private int top;
private T[] elem = null;
public Stack(){
this(10);
}
public Stack(int size){
this.elem = (T[])new Object[size];
this.top = 0;
}
/**
* 栈是否已满
*/
public boolean isFull(){
if(this.top == this.elem.length){
return true;
}
return false;
}
/**
* 入栈
*/
public boolean push(T val){
if(isFull()){
return false;
}
this.elem[top++] = val;
return true;
}
/**
* 是否为空
*/
public boolean isEmpty(){
if(this.top == 0){
return true;
}
return false;
}
/**
* 出栈
*/
public T pop(){
if(isEmpty()){
return null;
}
return elem[--this.top];
}
/**
* 得到栈的元素
*/
public T getTop(){
if(isEmpty()){
return null;
}
return this.elem[this.top-1];
}
/**
* 打印栈内元素
*/
public void show(){
for(int i =0;i<this.top;i++){
System.out.println(this.elem[i]+" ");
}
System.out.println();
}
}
public class Tstack {
public static void main(String[] args) {
Stack<Integer> s1 = new Stack<Integer>();
int length = 10;
for (int i = 0; i < length; i++) {
s1.push(i);
}
s1.show();
}
}
运行结果
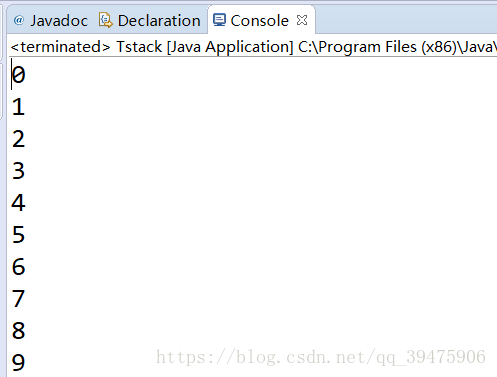