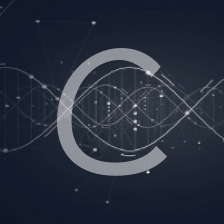
树
数据结构部分-二叉树
zjc4j
打工人
展开
-
层次遍历,偶数层从右向左打印,奇数层从左向右打印。
题目 层次遍历,偶数层从右向左打印,奇数层从左向右打印。 代码 package com.zjc.test.tree; import java.util.*; class TreeNode { int val; TreeNode left; TreeNode right; TreeNode(){} TreeNode(int val) {this.val = val;} TreeNode(int val,TreeNode left, TreeNode righ原创 2021-08-30 00:55:30 · 389 阅读 · 0 评论 -
键值的映射
题目 题源 代码 码源 class MapSum { /** Initialize your data structure here. */ private class Node { Node[] child = new Node[26]; int val; } private Node root; public MapSum() { root = new Node(); } public原创 2021-07-30 01:37:07 · 219 阅读 · 0 评论 -
二叉搜索树中的众数
题目 题源 代码 class Solution { private TreeNode pre; private int curSameNum = 0; private int maxSameNum = 0; public int[] findMode(TreeNode root) { List<TreeNode> list = new ArrayList<TreeNode>(); helper(root, list);原创 2021-07-29 23:01:04 · 77 阅读 · 0 评论 -
二叉搜索树的最小绝对差
题目 码源 代码 class Solution { private int minNum = Integer.MAX_VALUE; private TreeNode pre = null; public int getMinimumDifference(TreeNode root) { minimumDifference(root); return minNum; } private void minimumDifference(Tr原创 2021-07-29 21:34:18 · 65 阅读 · 0 评论 -
两数之和 IV - 输入 BST
题目 题源 代码 class Solution { List<Integer> al = new ArrayList<>(); public boolean findTarget(TreeNode root, int k) { if (root == null) return false; TreeNode2ArrayList(root); int i = 0, j = al.size() - 1;原创 2021-07-28 23:56:36 · 69 阅读 · 0 评论 -
有序链表转换二叉搜索树
题目 题源 代码 class Solution { public TreeNode sortedListToBST(ListNode head) { if (head == null) return null; if (head.next == null) return new TreeNode(head.val); ListNode preMid = MidNode(head); ListNode MidNode = preMid.原创 2021-07-28 22:48:01 · 93 阅读 · 0 评论 -
从有序数组中构造二叉查找树
题目 题源 代码 class Solution { public TreeNode sortedArrayToBST(int[] nums) { TreeNode tn = toBST(nums, 0, nums.length - 1); return tn; } private TreeNode toBST(int[] nums, int left, int right) { if (left > right) return原创 2021-07-17 23:07:56 · 209 阅读 · 0 评论 -
二叉树的最近公共祖先
目前 题源 代码 码源 class Solution { public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) { if (root == null || (root == p || root == q)) return root; TreeNode left = lowestCommonAncestor(root.left, p, q); TreeNo原创 2021-07-16 21:21:56 · 95 阅读 · 0 评论 -
二叉搜索树的最近公共祖先(注意是二叉搜索树)
题目 题源 代码 class Solution { public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) { if (root.val > p.val && root.val > q.val) return lowestCommonAncestor(root.left, p, q); if (root.val < p.val &原创 2021-07-15 22:48:57 · 79 阅读 · 0 评论 -
把二叉搜索树转换为累加树
题目 题源 代码 右中左的方式递归遍历: class Solution { int sum = 0; public TreeNode convertBST(TreeNode root) { if (root != null) { convertBST(root.right); sum += root.val; root.val = sum; convertBST(root.lef原创 2021-07-15 20:46:39 · 80 阅读 · 0 评论 -
二叉搜索树中第K小的元素
题目 题源 代码 码源 解法1: class Solution { public int kthSmallest(TreeNode root, int k) { LinkedList<TreeNode> stack = new LinkedList<TreeNode>(); while (true) { while (root != null) { stack.add(root);原创 2021-07-15 20:14:02 · 68 阅读 · 0 评论 -
修剪二叉搜索树
题目 题源 代码 码源 class Solution { public TreeNode trimBST(TreeNode root, int low, int high) { if(root == null) return null; if (root.val < low) { //因为是二叉搜索树,节点.left < 节点 < 节点.right //节点数字比low小,就把左节点全部裁掉.原创 2021-07-13 23:23:34 · 88 阅读 · 0 评论 -
找树左下角的值
题目 题源 代码 class Solution { public int findBottomLeftValue(TreeNode root) { Queue<TreeNode> queue = new LinkedList<>(); queue.offer(root); while (!queue.isEmpty()) { root = queue.poll(); if (ro原创 2021-07-13 21:52:22 · 59 阅读 · 0 评论 -
二叉树的层平均值
题目 题源 代码 class Solution { public List<Double> averageOfLevels(TreeNode root) { List result = new ArrayList<>(); Queue<TreeNode> queue = new LinkedList<>(); queue.offer(root); while (!queue.isEmpt原创 2021-07-13 21:41:44 · 87 阅读 · 0 评论 -
二叉树中第二小的节点
题目 题源 代码 码源 class Solution { public int findSecondMinimumValue(TreeNode root) { if (root == null) return -1; if (root.left == null && root.right == null) return -1; int leftVal = root.left.val; int rightVal = ro原创 2021-07-13 21:17:39 · 79 阅读 · 0 评论 -
打家劫舍 III
题目 码源 代码 简化一下这个问题:一棵二叉树,树上的每个点都有对应的权值,每个点有两种状态(选中和不选中),问在不能同时选中有父子关系的点的情况下,能选中的点的最大权值和是多少。 码源 class Solution { Map<TreeNode, Integer> f = new HashMap<TreeNode, Integer>(); Map<TreeNode, Integer> g = new HashMap<TreeNode, Integ原创 2021-07-13 20:59:40 · 82 阅读 · 0 评论 -
最长同值路径
题目 题源 代码 class Solution { private int path = 0; public int longestUnivaluePath(TreeNode root) { dfs(root); return path; } private int dfs(TreeNode root) { if (root == null) return 0; int left = dfs(root.left原创 2021-07-13 20:14:37 · 67 阅读 · 0 评论 -
左叶子之和
题目 题源 代码 深度优先: class Solution { public int sumOfLeftLeaves(TreeNode root) { return root != null ? dfs(root) : 0; } private int dfs(TreeNode node) { int ans = 0; if (node.left != null) { ans += isLeafNode(no原创 2021-07-06 22:40:15 · 63 阅读 · 0 评论 -
二叉树的最小深度
题目 题源 代码 码源 class Solution { public int minDepth(TreeNode root) { if (root == null) { return 0; } if (root.left == null && root.right == null) { return 1; } int min_depth = Inte原创 2021-07-05 22:17:29 · 57 阅读 · 0 评论 -
另一个树的子树
题目 题源 代码 class Solution { public boolean isSubtree(TreeNode root, TreeNode subRoot) { return (root != null) && (subRoot != null) &&((helper(root, subRoot) || isSubtree(root.left, subRoot) || isSubtree(root.right, subRoot)));原创 2021-07-05 21:43:36 · 62 阅读 · 0 评论 -
路径总和 III
题目 题源 代码 码源 class Solution { public int pathSum(TreeNode root, int targetSum) { if (root == null) return 0; int result = pathSumStartWithRoot(root, targetSum) + pathSum(root.left,targetSum) + pathSum(root.right,targetSum); retu原创 2021-07-05 21:19:17 · 73 阅读 · 0 评论 -
合并二叉树
题目 题源 代码 class Solution { public TreeNode mergeTrees(TreeNode root1, TreeNode root2) { if (root1 == null || root2 == null) { return root1 == null ? root2 : root1; } TreeNode root = new TreeNode(root1.val + root2.val原创 2021-07-05 20:52:02 · 56 阅读 · 0 评论 -
平衡二叉树
题目 题源 代码 码源 自顶向下的递归: class Solution { public boolean isBalanced(TreeNode root) { if (root == null) { return true; } else { return Math.abs(height(root.left) - height(root.right)) <= 1 && isBalanced(ro原创 2021-07-05 20:35:24 · 58 阅读 · 0 评论 -
二叉树的最大深度
题目 题源 代码 class Solution { public int maxDepth(TreeNode root) { if (root == null) return 0; return Math.max(maxDepth(root.left), maxDepth(root.right) )+ 1; } }原创 2021-07-05 20:13:14 · 46 阅读 · 0 评论 -
路径总和 II
题目 题源 代码 深度优先: class Solution { List<List<Integer>> ret = new LinkedList<List<Integer>>(); Deque<Integer> path = new LinkedList<Integer>(); public List<List<Integer>> pathSum(TreeNode root, int原创 2021-07-03 21:43:30 · 61 阅读 · 0 评论 -
二叉树的序列化与反序列化
题目 题源 代码 官方代码 public class Codec { // Encodes a tree to a single string. public String serialize(TreeNode root) { return rserialize(root,""); } // Decodes your encoded data to tree. public TreeNode deserialize(String dat原创 2021-07-01 21:43:12 · 72 阅读 · 0 评论 -
路径总和(深度优先与广度优先解决此问题)
问题 码源 代码 深度优先法: class Solution { public boolean hasPathSum(TreeNode root, int targetSum) { if (root == null) { return false; } return dfsPathSum (root,targetSum); } private boolean dfsPathSum (TreeNod原创 2021-06-30 21:10:34 · 137 阅读 · 0 评论 -
二叉树的中序遍历(递归与非递归)
题目 题源 略 代码 递归法: class Solution { List<Integer> list = new LinkedList<>(); public List<Integer> inorderTraversal(TreeNode root) { infixOrder(root); return list; } private void infixOrder(TreeNode root) {原创 2021-06-06 22:16:35 · 57 阅读 · 0 评论 -
二叉树的锯齿形层序遍历
题目 题源 代码 用广度优先思想解决此问题: class Solution { public List<List<Integer>> zigzagLevelOrder(TreeNode root) { List<List<Integer>> ans = new LinkedList<List<Integer>>(); if (root == null) return an原创 2021-06-05 21:57:51 · 65 阅读 · 0 评论 -
对称二叉树
代码 // 迭代法 class Solution { public boolean isSymmetric(TreeNode root) { return check(root, root); } public boolean check(TreeNode p, TreeNode q) { Queue<TreeNode> queue = new LinkedList<TreeNode>(); queue.off原创 2021-05-31 00:22:50 · 111 阅读 · 0 评论