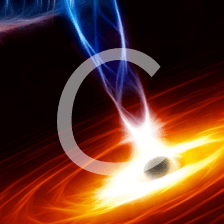
刷力扣
zjc4j
打工人
展开
-
数组中的逆序对
题目题源题目码源class Solution { public int reversePairs(int[] nums) { int len = nums.length; if (len < 2) return 0; int[] copy = new int[len]; for (int i = 0; i < len; i++) { copy[i] = nums[i];原创 2021-07-04 21:27:42 · 58 阅读 · 0 评论 -
x 的平方根
题目题源代码class Solution { public int mySqrt(int x) { // return (int)Math.sqrt(x); int left = 0, right = x, ans = -1; while(left <= right) { int mid = left + (right - left) / 2; if ((long)mid * mid <原创 2021-06-25 20:55:12 · 79 阅读 · 0 评论 -
实现 Trie (前缀树)(重要)
题目题源代码对比下图理解:码源class Trie { private Trie[] children; // isEnd用于表示该节点是否为字符串的结尾 private boolean isEnd; /** Initialize your data structure here. */ public Trie() { children = new Trie[26]; isEnd = false; }原创 2021-05-13 21:20:45 · 142 阅读 · 0 评论 -
最长回文子串
题目题源代码class Solution { public String longestPalindrome(String s) { if (s == null || s.length() < 1) return ""; int start = 0, end = 0; for (int i = 0; i < s.length(); i++) { int len1 = expandAroundCenter(s,原创 2021-05-11 22:18:45 · 69 阅读 · 0 评论 -
判断是否位2的幂
代码代码方法1,标准方法class Solution { public boolean isPowerOfTwo(int n) { // 方法1,标准方法 if(n <= 0) return false; // 判断是否为 2 的幂 return (n & (n-1)) == 0; }}方法2,暴力法class Solution { public boolean is原创 2021-05-10 22:05:58 · 67 阅读 · 0 评论 -
字典序的第K小数字
题目题源代码码源class Solution { public int findKthNumber(int n, int k) { long cur = 1; k -= 1; while(k > 0){ int nodes = getNodes(n, cur); // 当前节点的总数小于要查找的第k位,则右移 if(k >= nodes){原创 2021-05-10 21:40:31 · 109 阅读 · 0 评论 -
车队
题目代码class Solution { public int carFleet(int target, int[] position, int[] speed) { int N = position.length; Car[] cars = new Car[N]; for (int i = 0; i < N; i++) // 计算的不考虑其他车时的到达target的时间 cars[i] =原创 2021-05-08 22:45:50 · 104 阅读 · 0 评论 -
把数组排成最小的数
题目题源代码码源class Solution { public String minNumber(int[] nums) { String[] strs = new String[nums.length]; for (int i = 0; i < nums.length; i++) strs[i] = String.valueOf(nums[i]); Arrays.sort(strs,(x ,原创 2021-05-08 21:59:42 · 63 阅读 · 0 评论 -
搜索旋转排序数组
题目题源代码class Solution { // 方法1,时间复杂度O(n) public int search1(int[] nums, int target) { for (int i = 0; i < nums.length; i++) { if (target == nums[i]) { return i; } } return -1;原创 2021-05-08 21:25:59 · 83 阅读 · 0 评论 -
编辑距离
题目题源代码码源class Solution { public int minDistance(String word1, String word2) { int n = word1.length(); int m = word2.length(); // 有一个字符串为空串 if (n * m == 0) { return n + m; } // DP 数组原创 2021-05-07 22:41:55 · 88 阅读 · 0 评论 -
无重复字符的最长子串
题目题源代码class Solution { public int lengthOfLongestSubstring(String s) { Set<Character> set = new HashSet<>(); int n = s.length(); // rk保存上一次遍历的末尾位置,这个rk指针不会回退,因为i + 1到rk这个位置一定不会重复,直接考虑rk + 1的位置即可原创 2021-05-07 22:04:55 · 67 阅读 · 0 评论 -
字符串的排列
题目题源代码码源class Solution { List<String> res = new LinkedList<>(); char[] c; public String[] permutation(String s) { c = s.toCharArray(); dfs(0); return res.toArray(new String[res.size()]); } v原创 2021-05-06 22:37:46 · 59 阅读 · 0 评论 -
最长递增子序列
题目题源代码码源class Solution { public int lengthOfLIS(int[] nums) { int len = 1, n = nums.length; if (n == 0) { return 0; } int[] d = new int[n + 1]; d[len] = nums[0]; for (int i = 1; i < n;原创 2021-05-06 21:31:02 · 59 阅读 · 0 评论 -
反转链表
题目题源代码class Solution { public ListNode reverseList(ListNode head) { ListNode pre = null; ListNode cur = head; while (cur != null) { ListNode nextNode = cur.next; cur.next = pre; pre = cur;原创 2021-05-06 00:02:27 · 73 阅读 · 0 评论 -
翻转二叉树
题目链接:https://leetcode-cn.com/problems/invert-binary-tree/代码class Solution { public TreeNode invertTree(TreeNode root) { if (root == null) return null; TreeNode right = invertTree(root.right); TreeNode left = inve原创 2021-05-05 23:53:53 · 64 阅读 · 0 评论 -
爬楼梯(动态规划)
题目代码class Solution { public int climbStairs(int n) { if (n == 1) return 1; int[] dp = new int[n + 1]; dp[1] = 1; dp[2] = 2; for (int i = 3; i <= n; i++) { dp[i] = dp[i - 1] + dp[i -原创 2021-05-05 23:41:17 · 87 阅读 · 0 评论 -
三数之和
题目代码先排序 + 前后双指针class Solution { public List<List<Integer>> threeSum(int[] nums) { int n = nums.length; Arrays.sort(nums); List<List<Integer>> ans = new ArrayList<List<Integer>>();原创 2021-05-03 22:28:57 · 80 阅读 · 0 评论 -
乘积最大子数组
题目代码码源class Solution { public int maxProduct(int[] nums) { int maxF = nums[0], minF = nums[0], ans = nums[0]; int length = nums.length; for (int i = 1; i < length; ++i) { int mx = maxF, mn = minF;原创 2021-05-03 22:00:47 · 72 阅读 · 0 评论 -
缺失的第一个正数
题目代码方法1,进阶法,hash:码源class Solution { //方法1 public int firstMissingPositive(int[] nums) { int n = nums.length; for (int i = 0; i < n; i++) { if (nums[i] <= 0) { nums[i] = n + 1; }原创 2021-04-23 09:55:38 · 78 阅读 · 0 评论 -
最大子序和
题目代码beforeNumMax 表示前一个数的最大连续子数组,当前num的最大连续子数组仅仅跟当前数的前一个数的最大连续子数组相关。class Solution { public int maxSubArray(int[] nums) { int maxSum = nums[0]; int beforeNumMax = 0; for (int num : nums) { beforeNumMax = Math.max(原创 2021-04-21 22:30:07 · 91 阅读 · 0 评论 -
组合总和(可重复取)
题目代码码源class Solution { public List<List<Integer>> combinationSum(int[] candidates, int target) { int len = candidates.length; List<List<Integer>> la = new ArrayList<>(); if (len == 0)原创 2021-04-21 22:00:27 · 198 阅读 · 0 评论 -
两个正序数组的中位数
题目代码方法1,简单易想法:有序的合并成一个数组,取其中位数即可。class Solution { public double findMedianSortedArrays(int[] nums1, int[] nums2) { int[] nums; int m = nums1.length; int n = nums2.length; nums = new int[m + n]; if原创 2021-04-20 22:22:40 · 152 阅读 · 0 评论 -
零钱兑换(完全背包问题)
题目代码码源动态规划法class Solution { public int coinChange(int[] coins, int amount) { int max = amount + 1; int[] dp = new int[amount + 1]; Arrays.fill(dp,max); dp[0] = 0; for (int i = 1; i <= amount; i++)原创 2021-04-17 14:23:14 · 216 阅读 · 0 评论 -
接雨水
题目代码双指针法:左边,以左指针遍历过的最大高度记为left_max,然后左指针向右走下一格,如果高度大于left_max则更新left_max,否则计算接水量,计算方式为left_max - 左指针所在当前位置的高度即为这个位置接到的水量;右侧,同理;class Solution { public int trap(int[] height) { int left = 0; int right = height.length-1; int原创 2021-04-17 13:06:18 · 81 阅读 · 0 评论 -
数据流的中位数
题目代码使用两个堆,一个是大顶堆,一个是小顶堆。大顶堆中最大的数小于等于小顶堆中最小的数;保证这两个堆中的元素个数的差不超过 1。若数据总数为偶数,当这两个堆建好之后,中位数就是这两个堆顶元素的平均值。当数据总数为奇数时,根据两个堆的大小,中位数一定在数据多的堆的堆顶。class MedianFinder { private PriorityQueue<Integer> maxHeap; private PriorityQueue<Integer> min原创 2021-04-14 22:31:16 · 100 阅读 · 0 评论 -
两数之和(力扣第一题)
题目代码class Solution { // 方法1 暴力法 public int[] twoSum(int[] nums, int target) { for (int i = 0; i < nums.length; i++) { for (int j = i+1; j < nums.length; j++) { if (target - nums[i] == nums[j])原创 2021-04-07 22:20:39 · 86 阅读 · 0 评论 -
找数组中重复的数字(如果多个只返回其中一个就可以)
题目代码class Solution { public int findRepeatNumber(int[] nums) { Set<Integer> set = new HashSet<>(); int repeat = -1; for (int num: nums) { if (!set.add(num)) { repeat = num;原创 2021-04-04 21:44:30 · 344 阅读 · 0 评论 -
一个数组,只出现一次的数字(且只有一个出现一次的数字)
题目解析码源代码class Solution { public int singleNumber(int[] nums) { int single = 0; for (int num : nums) { single ^= num; } return single; }}原创 2021-03-30 22:40:19 · 171 阅读 · 0 评论 -
求数组中最小的k个数
题目代码方法1,实现最简单的方法// 方法1,思路最简单排序public int[] getLeastNumbers(int[] arr, int k) { Arrays.sort(arr); int[] result = new int[k]; for (int i = 0; i< k; i++) { result[i] = arr[i]; } return result;}方法2,堆排序码源// 方法2,堆排序,Prior原创 2021-03-26 22:34:59 · 205 阅读 · 0 评论 -
二叉树的所有路径
题目代码方法1,广度优先法: // 方法1,广度优先遍历class Solution { public List<String> binaryTreePaths(TreeNode root) { List<String> paths = new ArrayList<>(); if (root == null) return paths; Queue<原创 2021-03-24 22:40:11 · 70 阅读 · 0 评论 -
二叉树的直径(任意两个结点路径长度中的最大值)
题目代码class Solution { int ans = 1; public int diameterOfBinaryTree(TreeNode root) { depth(root); return ans-1; } public int depth(TreeNode node) { if (node == null) return 0; // 获取左孩子为根的树的深度 int le原创 2021-03-23 22:36:41 · 389 阅读 · 0 评论 -
最近最少使用(LRU)缓存策略设计一个数据结构
题目题源样例1:输入:LRUCache(2)set(2, 1)set(1, 1)get(2)set(4, 1)get(1)get(2)输出:[1,-1,1]解释:cache上限为2,set(2,1),set(1, 1),get(2) 然后返回 1,set(4,1) 然后 delete (1,1),因为 (1,1)最少使用,get(1) 然后返回 -1,get(2) 然后返回 1样例2:输入:LRUCache(1)set(2, 1)get(2)set(3, 2)ge原创 2021-03-22 22:38:16 · 228 阅读 · 0 评论 -
二叉树的前序、中序、后序(递归和非递归)、层次遍历
代码public class Solution { /** * @param root: A Tree * @return: Preorder in ArrayList which contains node values. */ private List<Integer> list = new ArrayList<Integer>(); public List<Integer> preorderTraversa.原创 2021-03-21 22:01:40 · 126 阅读 · 1 评论 -
验证二叉搜索树
题目代码 //方法1,递归方法public boolean isValidBST(TreeNode root) { if (root == null || (root.left == null && root.right == null)) return true; return helper(root,Long.MIN_VALUE,Long.MAX_VALUE);}public boolean helper(TreeNode node, long minVa原创 2021-03-14 22:45:42 · 69 阅读 · 0 评论 -
判断二叉树B是否是二叉树A的子结构
题目代码思路如果二叉树B是二叉树A的子树,A树中必然存在B树的根节点。所以,先比较A树的根节点与B树的根节点,如果相同,则采用递归方法比较A树的左子树和B树的左子树以及比较A树的右子树和B树的右子树。如果不相同,则用A树根节点的左孩子与B树的跟节点比较,这里调用的是isSubStructure方法而不是helper方法是因为A树根节点的左孩子不一定存在。如果还不相同,同理用A树根节点的右孩子与B树的跟节点比较。class Solution { public boolean isSubSt原创 2021-03-14 22:13:25 · 799 阅读 · 1 评论 -
每K 个一组进行翻转链表
题目代码官方代码public ListNode reverseKGroup(ListNode head, int k) { ListNode hair = new ListNode(0); hair.next = head; ListNode pre = hair; while (head != null) { ListNode tail = pre; // 查看剩余部分长度是否大于等于 k for (int i原创 2021-03-12 16:33:58 · 80 阅读 · 0 评论 -
求栈的最小值
题目代码思路维护两个栈,一个是主栈,一个是最小栈。1、每次push操作,将元素插入主栈栈顶时,同步判断是否小于等于最小栈的栈顶元素,如果是则同步插入最小栈。2、每次pop操作,主栈栈顶元素如果等于最小栈栈顶元素,则同步出栈。class MinStack { private Stack<Integer> stack; private Stack<Integer> miniStack; /** initialize your data struct原创 2021-03-12 15:24:03 · 233 阅读 · 0 评论 -
逆波兰表达式
题目代码思路从左至右扫描表达式,遇到数字时,将数字压入堆栈,遇到运算符时,弹出栈顶的两个数,用运算符对它们做相应的计算(次顶元素和栈顶元素),并将结果入栈;重复上述过程直到表达式最右端,最后运算得出的值即为表达式的结果。注意:弹出的第一个数是操作的第二个数,弹出的第二个数是操作的第一个数。 public int evalRPN(String[] tokens) { Stack<String> stack = new Stack<>(); for原创 2021-03-12 13:57:42 · 101 阅读 · 0 评论 -
两个栈实现一个队列
题目代码思路双栈实现,一个只负责入,另一个只负责出。class CQueue { Stack<Integer> stackIn = null; Stack<Integer> stackOut =null; public CQueue() { stackIn = new Stack<>(); stackOut = new Stack<>(); } publ原创 2021-03-12 12:07:37 · 106 阅读 · 0 评论 -
括号匹配算法
题目代码思路 遇到右括号一定要在栈顶中取到对应的左括号,否则返回false。 public boolean isValid(String s) { int n = s.length(); // 奇数个括号直接返回false if (n % 2 == 1) { return false; } // 将各种括号存入HashMap中,右括号为key左括号为value Map<Character, Character>原创 2021-03-12 11:44:59 · 463 阅读 · 0 评论