-计算机网络(自顶向下的方法)python实现多线程web服务器
-先编写两个本地的html文件,success.html和fail.html用来测试
-success.html
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta http-equiv="content-type" content="text/html"; charset="utf-8"/>
</head>
<style type="text/css">
div {
text-align: center;
font-family: 楷体;
}
a{
color: red;
}
</style>
<body>
</br></br>
<div>
<img src="http://pic.gerenjianli.com/xiaohui2046/b57.jpg"/>
</br></br></br>
<h1><a href="https://www.szu.edu.cn/">欢迎来到深圳大学!</a></h1>
</div>
</body>
</html>
-fail.html
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta http-equiv="content-type" content="text/html"; charset="utf-8"/>
</head>
<style type="text/css">
div{
font-size: x-large;
text-align: center;
font-family: 楷体;
color: red;
}
</style>
<body>
<div>
<br><br>
<img src="http://pic.gerenjianli.com/xiaohui2046/b57.jpg" />
<br><br>
<h1 >404! 访问的路径不存在!</h1>
</div>
</body>
</html>
-web server
from socket import *
import threading
import os
'''自定义线程函数'''
def Server(tcpClisock, addr):
BUFSIZE = 1024
print('Waiting for the connection:', addr)
data = tcpClisock.recv(BUFSIZE).decode()
filename = data.split()[1]
filename = filename[1:]
'''当网络质量差没有收到浏览器的访问数据时执行'''
if filename == "":
tcpClisock.close()
print("请输入要访问的文件")
base_dir = os.getcwd()
file_dir = os.path.join(base_dir,filename)
'''当访问的文件在本地服务器存在时执行'''
if os.path.exists(file_dir):
f = open(file_dir,encoding = 'utf-8')
SUCCESS_PAGE = "HTTP/1.1 200 OK\r\n\r\n" + f.read()
print(SUCCESS_PAGE)
tcpClisock.sendall(SUCCESS_PAGE.encode())
tcpClisock.close()
else:
FAIL_PAGE = "HTTP/1.1 404 NotFound\r\n\r\n" + open(os.path.join(base_dir, "fail.html"), encoding="utf-8").read()
print(FAIL_PAGE)
tcpClisock.sendall(FAIL_PAGE.encode())
tcpClisock.close()
'''主函数'''
if __name__ == '__main__':
'''分配IP、端口、创建套接字对象'''
ADDR = ("", 8080)
tcpSersock = socket(AF_INET, SOCK_STREAM)
tcpSersock.bind(ADDR)
tcpSersock.listen(5)
print("waiting for connection......\n")
while True:
tcpClisock, addr = tcpSersock.accept()
thread = threading.Thread(target=Server, args=(tcpClisock, addr))
thread.start()
tcpSersock.close()
-项目文件目录
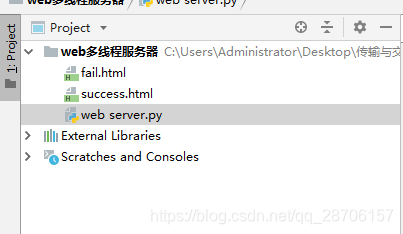
-下面开始测试
-在浏览器中输入http://localhost:8080/success.html 因为success.html文件在本地服务器存在所以可以返回success.html页面
-
-在浏览器中输入http://localhost:8080/index.html 因为index.html文件在本地服务器不存在所以可以返回fail.html页面
-控制台的打印信息
D:\anaconda\python.exe "C:/Users/Administrator/Desktop/传输与交换技术作业/web多线程服务器/web server.py"
waiting for connection......
Waiting for the connection: ('127.0.0.1', 54613)
HTTP/1.1 200 OK
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta http-equiv="content-type" content="text/html"; charset="utf-8"/>
</head>
<style type="text/css">
div {
text-align: center;
font-family: 楷体;
}
a{
color: red;
}
</style>
<body>
</br></br>
<div>
<img src="http://pic.gerenjianli.com/xiaohui2046/b57.jpg"/>
</br></br></br>
<h1><a href="https://www.szu.edu.cn/">欢迎来到深圳大学!</a></h1>
</div>
</body>
</html>
Waiting for the connection: ('127.0.0.1', 55265)
Waiting for the connection: ('127.0.0.1', 55266)
HTTP/1.1 404 NotFound
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta http-equiv="content-type" content="text/html"; charset="utf-8"/>
</head>
<style type="text/css">
div{
font-size: x-large;
text-align: center;
font-family: 楷体;
color: red;
}
</style>
<body>
<div>
<br><br>
<img src="http://pic.gerenjianli.com/xiaohui2046/b57.jpg" />
<br><br>
<h1 >404! 访问的路径不存在!</h1>
</div>
</body>
</html>