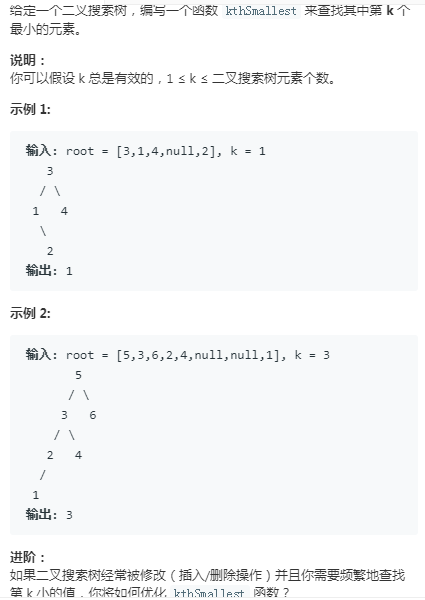
int count = 0;
public int kthSmallest(TreeNode root, int k) {
if (root == null) {
return Integer.MIN_VALUE;
}
int left = kthSmallest(root.left, k);
if (left!=Integer.MIN_VALUE){
return left;
}
count++;
if (count==k){
return root.val;
}
int right = kthSmallest(root.right, k);
if (right!=Integer.MIN_VALUE){
return right;
}
return Integer.MIN_VALUE;
}
public int kthSmallest2(TreeNode root, int k) {
if (root==null){
throw new IllegalArgumentException();
}
Stack<TreeNode> stack = new Stack<>();
while (!stack.isEmpty() || root!=null) {
while (root!=null){
stack.push(root);
root = root.left;
}
root = stack.pop();
k--;
if (k==0){
return root.val;
}
root = root.right;
}
throw new IllegalArgumentException();
}