springboot整合redis及set,map,list,key-value和实体类操作
系列文章
macm1安装redis过程
springboot整合redis及set,map,list,key-value和实体类操作
redis可视工具AnotherRedisDesktopManager的使用
本文目录
添加maven依赖
与redis相关的只有spring-boot-starter-data-redis
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
</dependencies>
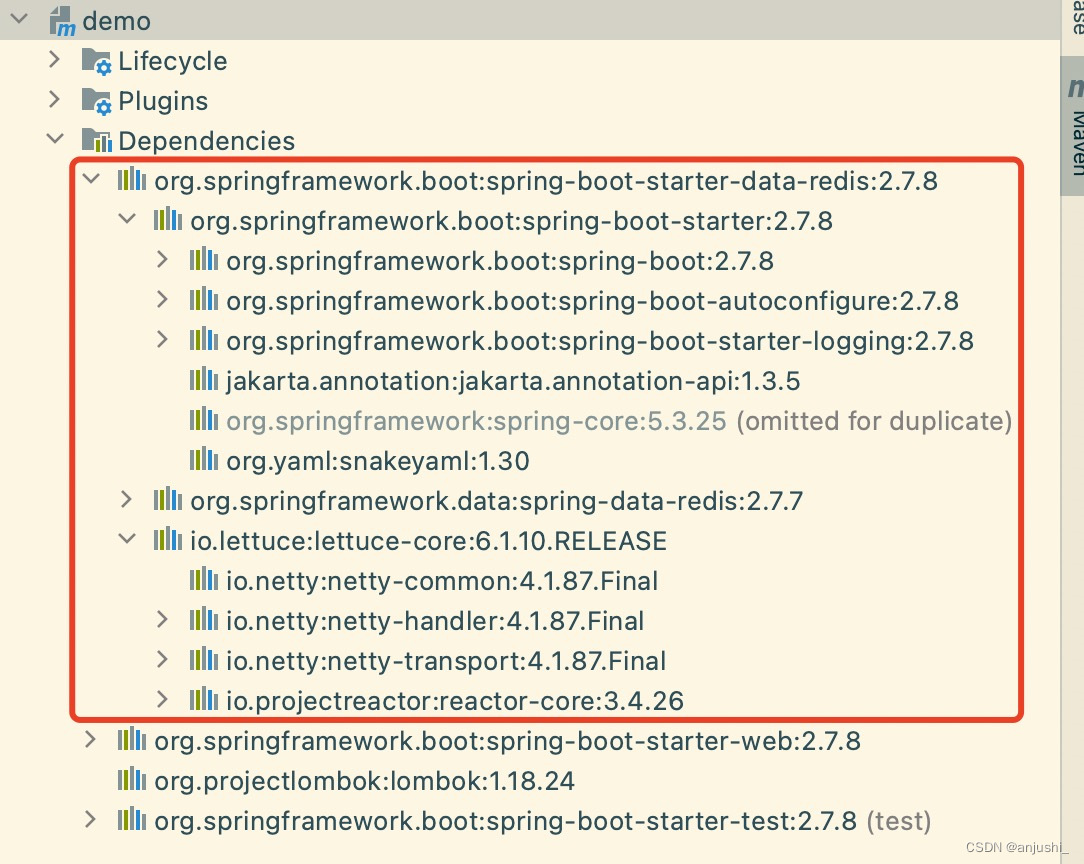
org.springframework.boot spring-boot-starter-data-redis data-redis
使用lettuce
客户端库
在程序中使用RedisTemplate
类的方法操作redis数据,实际就是调用的lettuce
客户端的中的方法
配置文件
配置application.yaml
spring:
redis:
# Redis数据库索引(默认为0)
database: 0
# Redis服务器地址
host: 127.0.0.1
# Redis服务器连接端口
port: 6379
# jedis:
# pool:
# # 连接池最大连接数(使用负值表示没有限制)
# max-active: 8
# # 连接池最大阻塞等待时间(使用负值表示没有限制)
# max-wait: 1
# # 连接池中的最大空闲连接
# max-idle: 8
# # 连接池中的最小空闲连接
# min-idle: 0
# # 连接超时时间(毫秒)
# timeout: 5000
server:
port: 8111
基础操作
RedisTemplate
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class RedisController {
/**
* 注入redistemplate,名字最好保持一致,因为框架
* 会创建一个跟这个名字一样的对象,这样根据名字来找比较好
*/
@Autowired
private RedisTemplate redisTemplate;
/**
* 添加数据到redis,这里添加的是String
* */
@PostMapping("/addData")
public String addData() {
//添加String类型的数据先获取ValueOperations对象
ValueOperations valueOperations = redisTemplate.opsForValue();
valueOperations.set("name","测试demo");
return "add Success";
}
@GetMapping("/getData")
public String getData(String key) {
//添加String类型的数据先获取ValueOperations对象
ValueOperations valueOperations = redisTemplate.opsForValue();
Object data = valueOperations.get(key);
return "获取到的key为: " + data;
}
}
使用postman测试接口
可以看到有乱码
设置RedisTemplate序列化
@EnableCaching
@Configuration
public class RedisConfig extends CachingConfigurerSupport {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
RedisSerializer<String> redisSerializer = new StringRedisSerializer();
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
template.setConnectionFactory(factory);
// key序列化方式
template.setKeySerializer(redisSerializer);
// value序列化
template.setValueSerializer(jackson2JsonRedisSerializer);
// value hashmap序列化
template.setHashValueSerializer(jackson2JsonRedisSerializer);
return template;
}
@Bean
public CacheManager cacheManager(RedisConnectionFactory factory) {
RedisSerializer<String> redisSerializer = new StringRedisSerializer();
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
// 解决查询缓存转换异常的问题
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
// 配置序列化(解决乱码的问题),过期时间600秒
RedisCacheConfiguration config = RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofSeconds(600))
.serializeKeysWith(RedisSerializationContext.SerializationPair.fromSerializer(redisSerializer))
.serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer(jackson2JsonRedisSerializer))
.disableCachingNullValues();
RedisCacheManager cacheManager = RedisCacheManager.builder(factory)
.cacheDefaults(config)
.build();
return cacheManager;
}
}
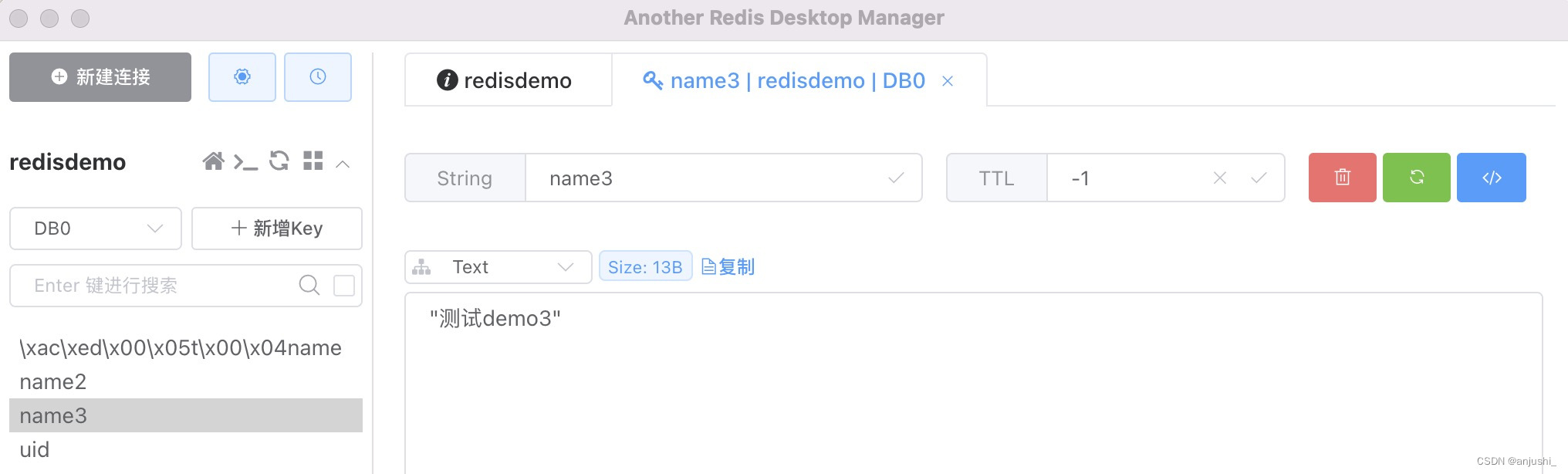
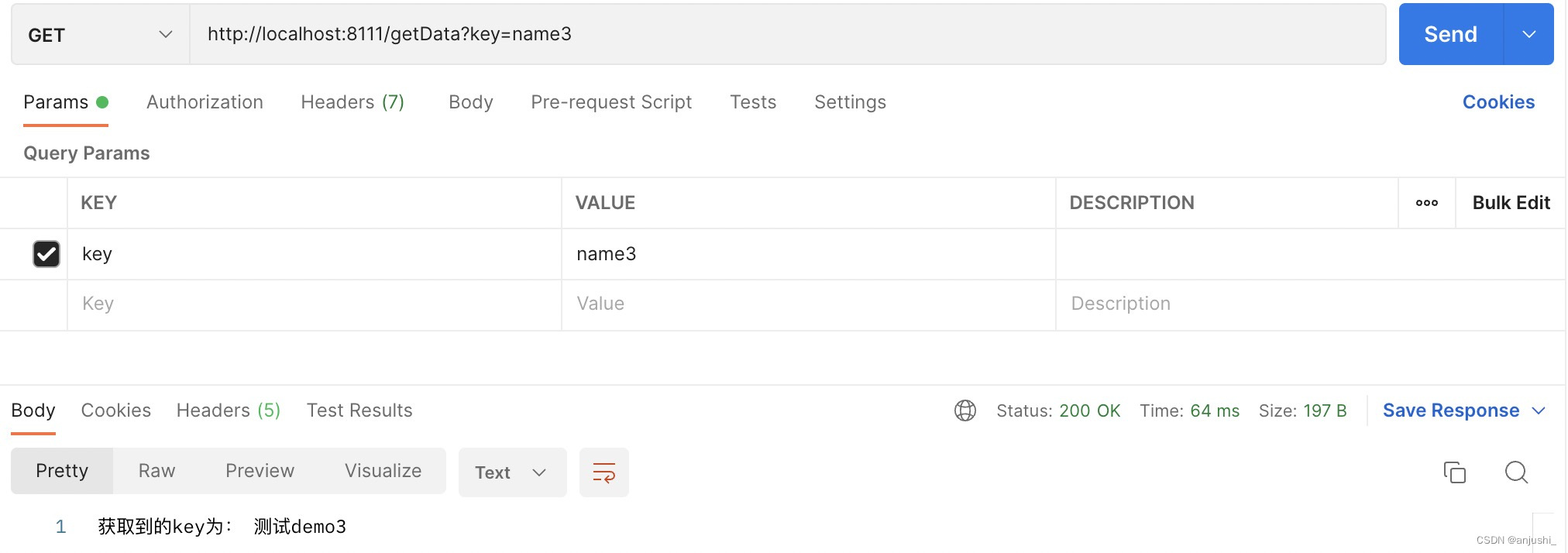
StringRedisTemplate
@RestController
public class RedisController {
@Autowired
private StringRedisTemplate stringRedisTemplate;
@PostMapping("/addSData")
public String addSData() {
//添加String类型的数据先获取ValueOperations对象
ValueOperations valueOperations = stringRedisTemplate.opsForValue();
valueOperations.set("name2","测试demo2");
return "add Success";
}
}
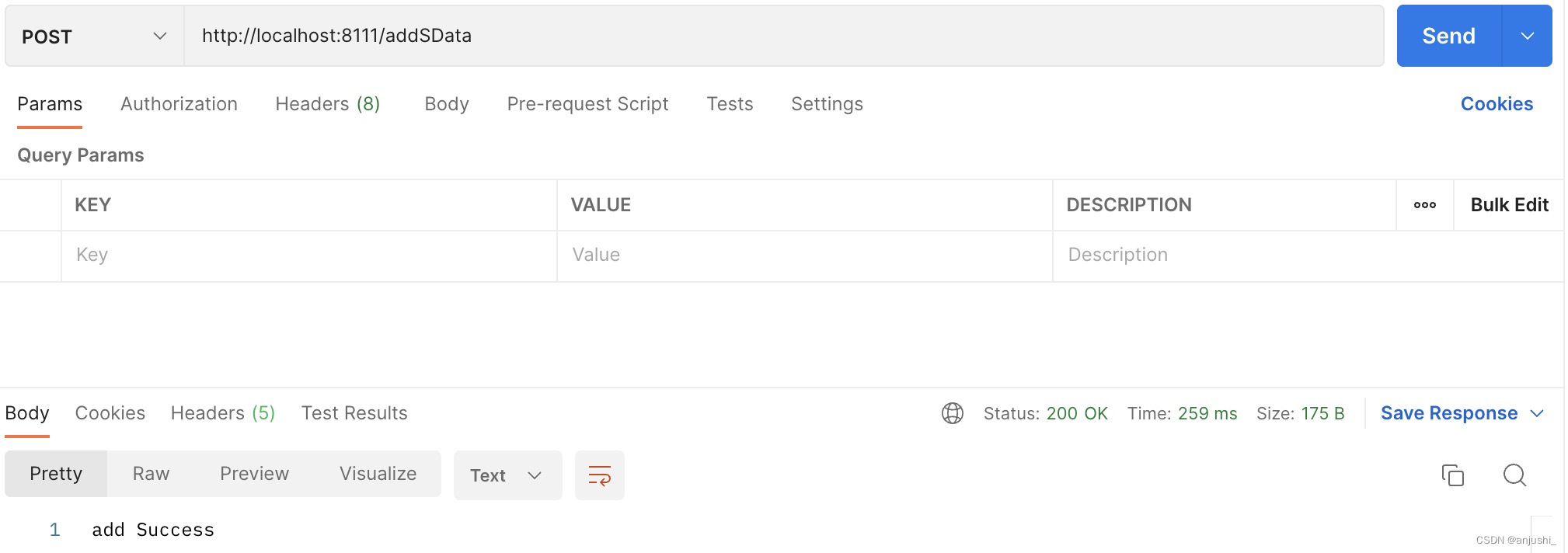
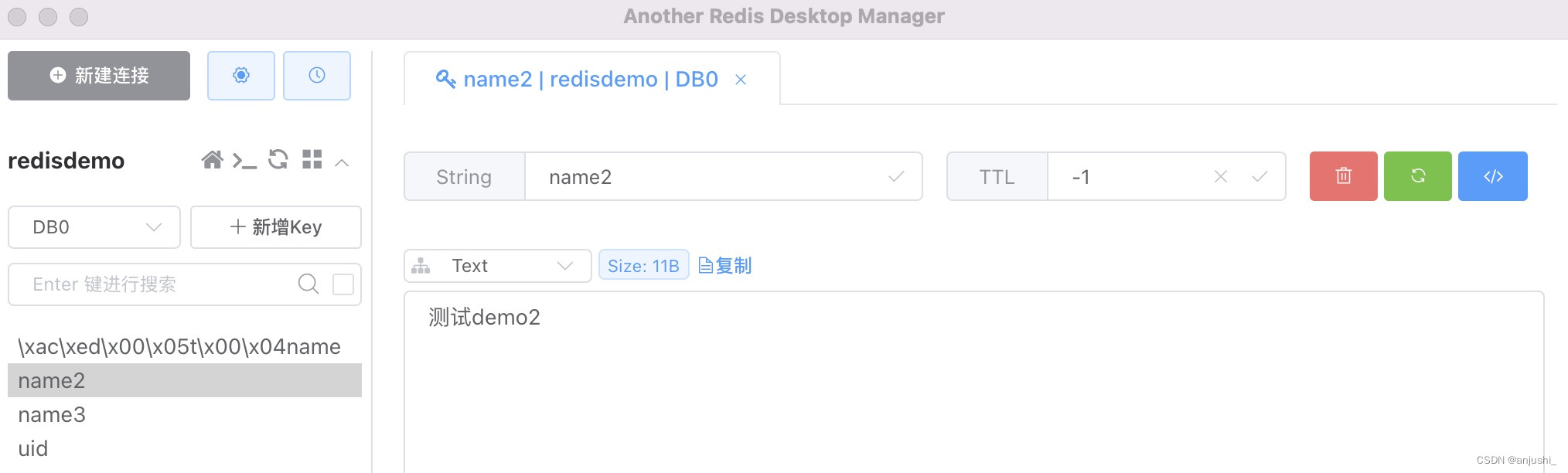
redis的set,map,list,key-value和实体类操作
set
@GetMapping("/addSet")
public String addSet() {
Set<String> set1 = new HashSet<String>();
set1.add("set1");
set1.add("set2");
set1.add("set3");
redisTemplate.opsForSet().add("set1", set1);
Set<String> resultSet = redisTemplate.opsForSet().members("set1");
System.out.println("resultSet:" + resultSet);
return "add set Success";
}
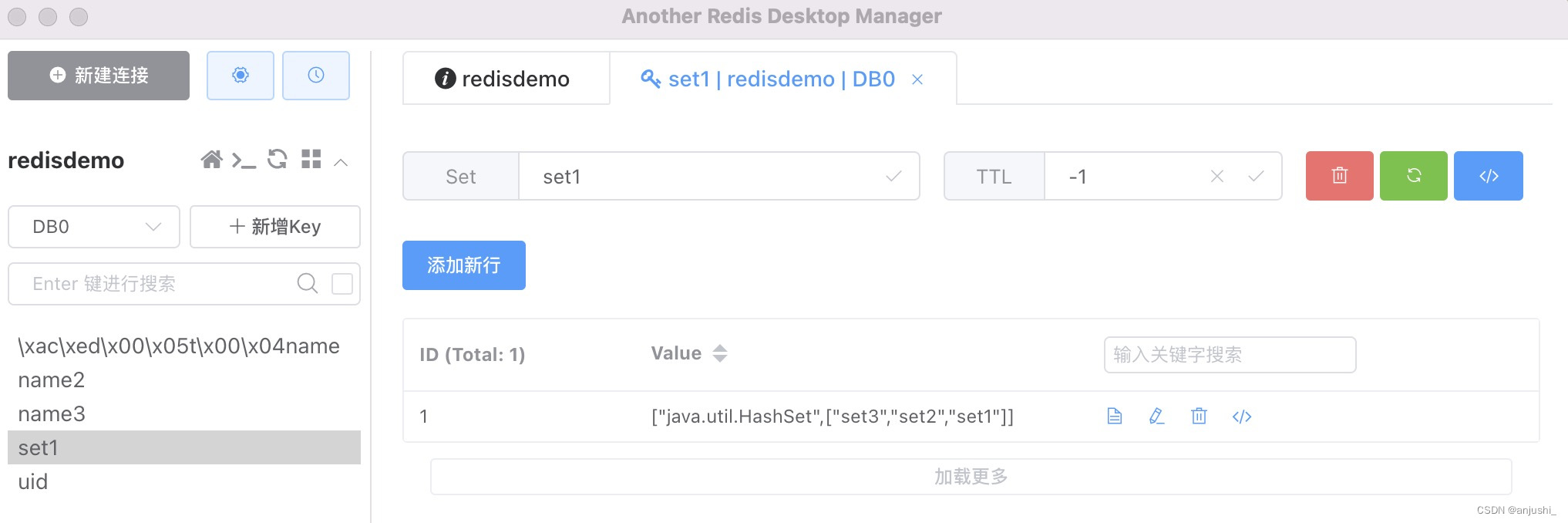
map
@GetMapping("/addMap")
public String addMap() {
Map<String, String> map = new HashMap<String, String>();
map.put("key1", "value1");
map.put("key2", "value2");
map.put("key3", "value3");
map.put("key4", "value4");
map.put("key5", "value5");
redisTemplate.opsForHash().putAll("map1", map);
Map<String, String> resultMap = redisTemplate.opsForHash().entries("map1");
List<String> reslutMapList = redisTemplate.opsForHash().values("map1");
Set<String> resultMapSet = redisTemplate.opsForHash().keys("map1");
String value = (String) redisTemplate.opsForHash().get("map1", "key1");
System.out.println("value:" + value);
System.out.println("resultMapSet:" + resultMapSet);
System.out.println("resultMap:" + resultMap);
System.out.println("resulreslutMapListtMap:" + reslutMapList);
return "add map Success";
}
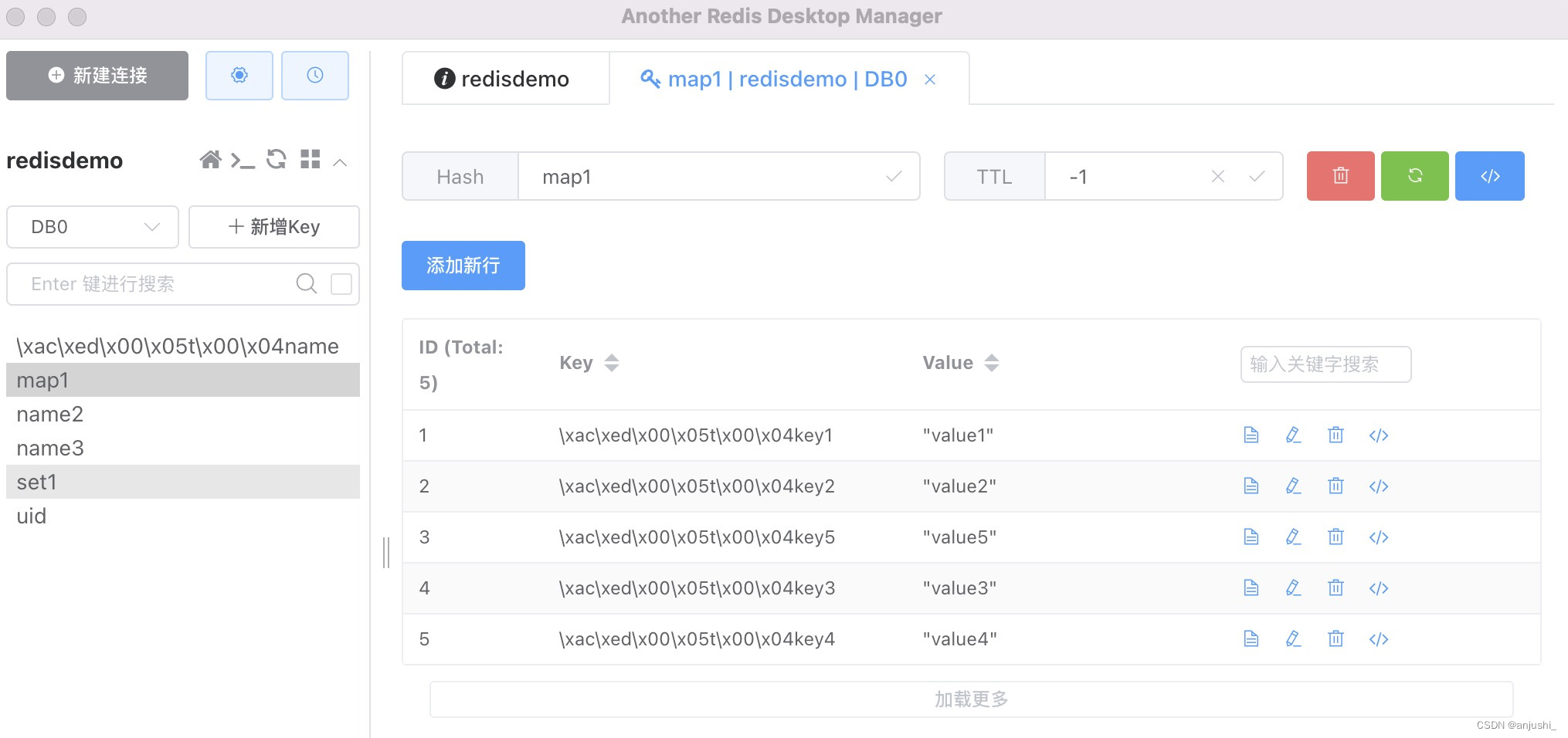
list
@GetMapping("/addList")
public String addList() {
List<String> list1 = new ArrayList<>();
list1.add("a1");
list1.add("a2");
list1.add("a3");
List<String> list2 = new ArrayList<>();
list2.add("b1");
list2.add("b2");
list2.add("b3");
redisTemplate.opsForList().leftPush("listkey1", list1);
redisTemplate.opsForList().rightPush("listkey2", list2);
// List<String> resultList1 = (List<String>) redisTemplate.opsForList().leftPop("listkey1");
// List<String> resultList2 = (List<String>) redisTemplate.opsForList().rightPop("listkey2");
return "add list Success";
}
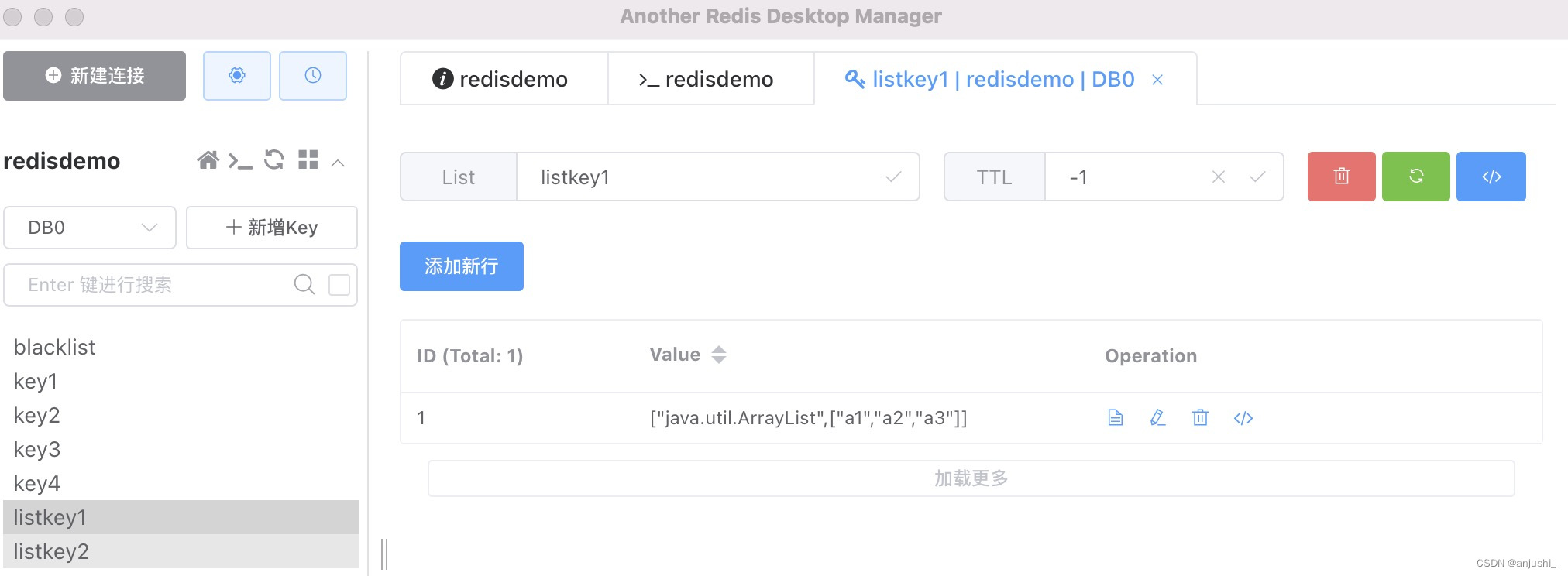
key-value
@GetMapping("/addKeyValue")
public String addKeyValue() {
System.out.println("缓存正在设置。。。。。。。。。");
redisTemplate.opsForValue().set("key1","value1");
redisTemplate.opsForValue().set("key2","value2");
redisTemplate.opsForValue().set("key3","value3");
redisTemplate.opsForValue().set("key4","value4");
System.out.println("缓存已经设置完毕。。。。。。。");
String result1=redisTemplate.opsForValue().get("key1").toString();
String result2=redisTemplate.opsForValue().get("key2").toString();
String result3=redisTemplate.opsForValue().get("key3").toString();
System.out.println("缓存结果为:result:"+result1+" "+result2+" "+result3);
return "add key value Success";
}
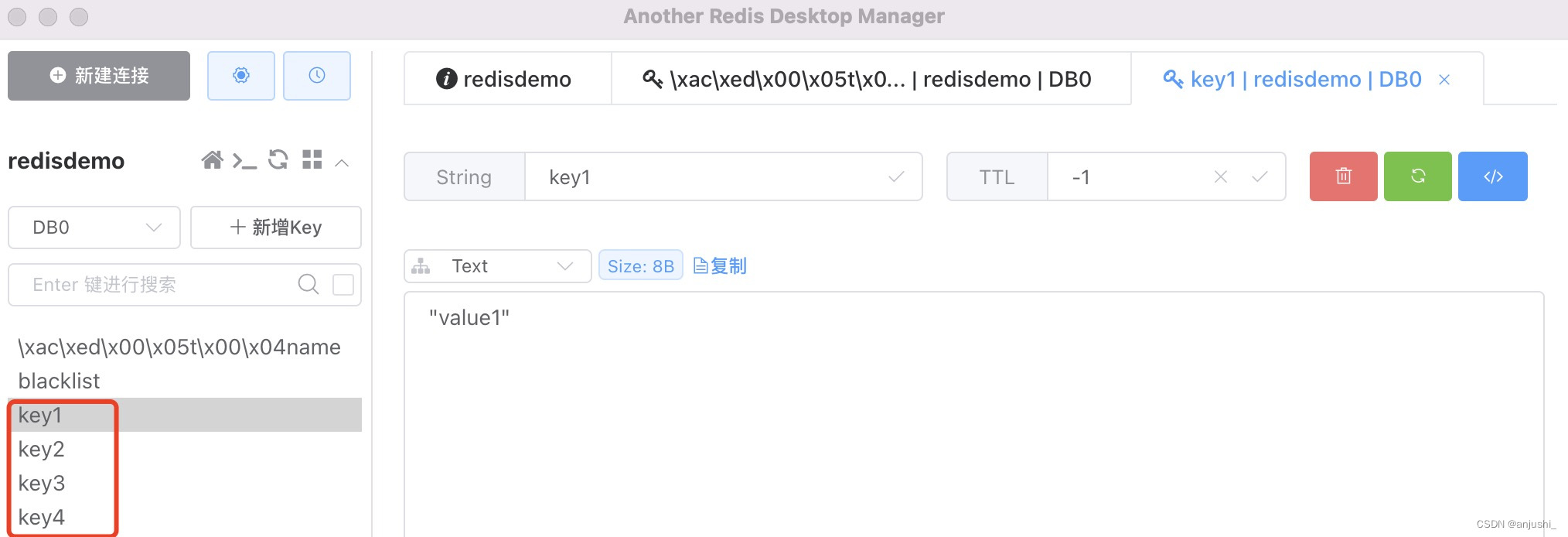
实体类
@GetMapping("/addEntity")
public String addEntity() {
List<Blacklist> blackList = new LinkedList<>();
Blacklist black = new Blacklist();
black.setId(1);
black.setIp("111.111.111.111");
black.setIptime(new Date());
blackList.add(black);
redisTemplate.opsForValue().set("blacklist",blackList);
List<Blacklist> resultBlackList= (List<Blacklist>) redisTemplate.opsForValue().get("blacklist");
for(Blacklist blacklist:resultBlackList){
System.out.println("ip:"+blacklist.getIp());
}
return "add entity Success";
}
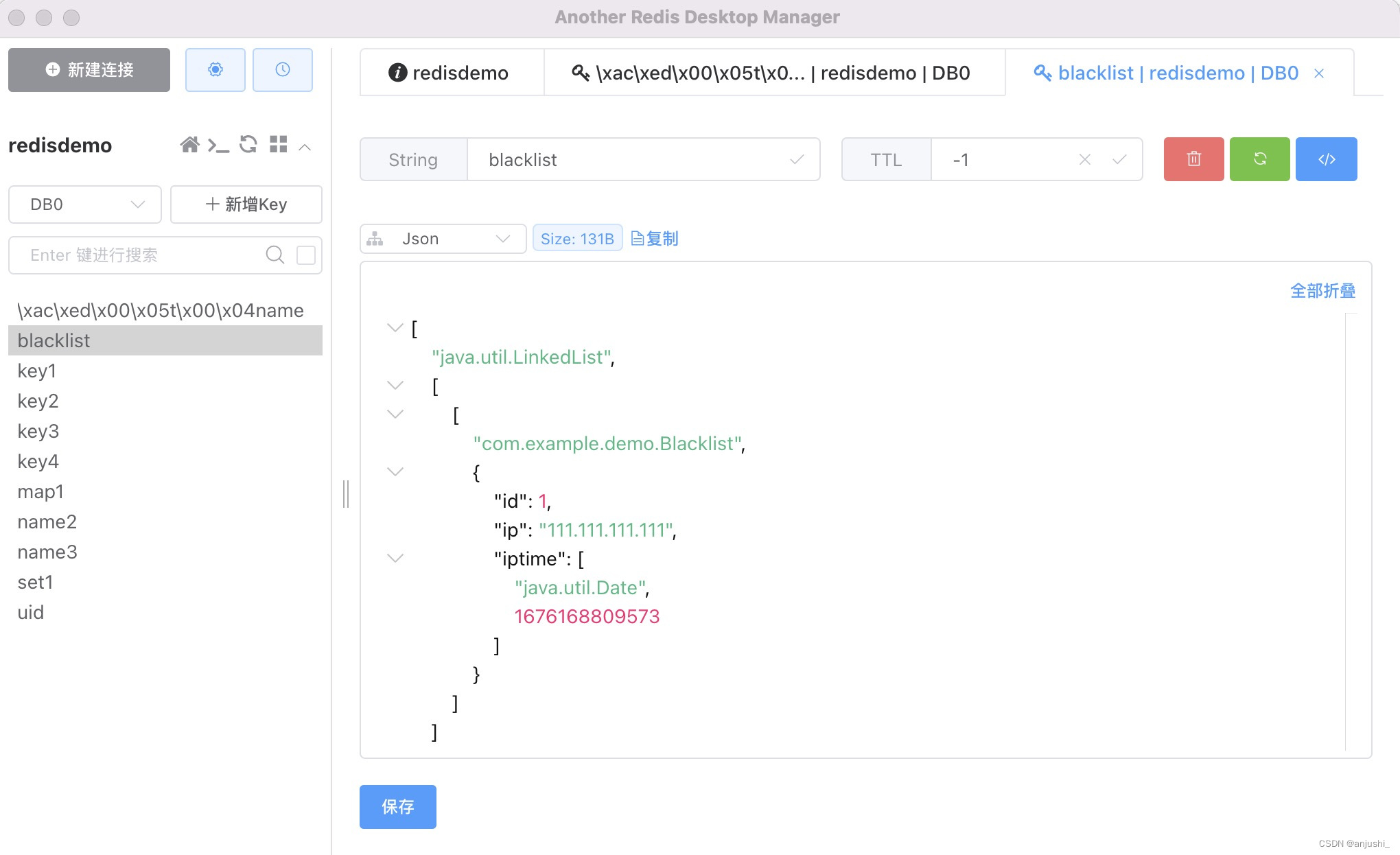