- 函数式接口:只包含一个抽象方法的接口,Lambda表达式实际上就是一个函数式接口,在底层使用匿名类来实现这个接口
以下都是笔记总结,详细内容都发布在资源当中
0. 关键字和基础知识大全
- 函数和方法的术语通常一样。不过偶尔会有不同,成为函数的代码块和对象无关,成为方法的代码块一般是与对象相关联的。
1. Kotlin入门知识
- 入门知识基础较多,之后会写的新博客进行总结,主要是Kotlin的一些基本规则
//1(声明一个可以为空的字符串类型的变量,并将其初始化为null)
val nullableString : String ? = null
2. 集合、函数式API(Lambda表达式)
Thread{
println("Thread is running")
}.start()
button.setOnClickListener{
}
3. 标准函数、静态方法
//4
val textview = TextView(context).apply{
text = "Hello"
textSize = 16dp
setTextColor(Color.BLACK)
}
//5
Object Util{
fun doAction(){
println("...")
}
}
//6、7
class Util{
fun doAction(){
println("...")
}
//companion object是一个伴生类,只有这个方法可以 Util.doAction2 (Kotlin保证只有一个伴生类对象)
companion object{
//@JvmStatic
fun doAction2(){
println("...")
}
}
}
//9
repeat(2){
data.add("Apple")
data.add("Grape")
data.add("Banana")
}
4. 延迟初始化和密封类
//1
private lateinit var adapter : StrAdapter
5. 拓展函数和运算符重载
//1
fun String.letterCount() : Int {
var count = 0
for( char in this ){
if( char.isLetter() ){
count++
}
}
return count
}
//2
class Obj{
...
operator fun plus(obj : Obj) : Obj{
//处理相加逻辑
}
}
val obj3 = obj1 + obj2
//5
//times是乘法的指定函数
//设置成String的拓展函数
//通过operator操作符进行重载
//repeat可以将字符串重复n次
operator fun String.times(n : Int) = repeat(n)
str * 20 就可以写了
6. 高阶函数详解
//2
fun Num1AndNum2 ( num1 : Int , num2 : Int , operation : (Int,Int)->Int ) : Int{
val result = operation(num1 , num2)
return result
}
fun plus(num1 : Int , num2 : Int) : Int{
return num1 + num2
}
fun minus(num1 : Int , num2 : Int) : Int{
return num1 - num2
}
//4
val result1 = Num1AndNum2( num1 , num2 , ::plus)
//9
val result1 = Num1AndNum2( num1 , num2 ){ n1 , n2 ->
n1 + n2
}
//11
inline fun Num1AndNum2( num1 : Int , num2 : Int , operation : (Int , Int) -> Int) : Int{
val result = operation(num1 , num2)
return result
}
//13
inline fun inlineTest(block1 : () -> Unit , noinline block2 : () -> Unit){
//只对block1进行了内联
}
7. 高阶函数的应用
- 高阶函数简化SharedPreferences
- 这部分代码在资源文件中
8. 泛型和委托
//3
class MyClass<T> {
fun method(param : T) : T{
return param
}
}
val myclass = MyClass<Int>
val result = myclass.method(123)
//4
//只定义泛型方法
class MyClass{
fun <T>method(param : T) : T{
return param
}
}
val result = myClass.method<Int>(123)
//9
//传入一个辅助对象HashSet,通过HashSet实现 接口Set 中的方法
class MySet<T>(val helpSet : HashSet<T>) : Set<T>{
}
//10
//by关键字让我们不需要实现所有的方法
class MySet<T>(val helpSet : HashSet<T>) : Set<T> by helperSet{
}
//11
class Example{
val p by Delegate()
}
class Delegate{
operator fun getValue(thisRef : Any? , property : KProperty<*>) : String{
}
operator fun setValue(thisRef : Any? , property : KProperty<*> , value : String){
}
}
9. 使用infix函数构建可读性语法
//2
infix fun String.beginWith(prefix : String) = startsWith(prefix) //startsWith是标准函数
if("Hello 123".startsWith("Hello")){
}
"Hello 123" beginWith "Hello"//infix函数的语法糖形式
10. 泛型的高级特性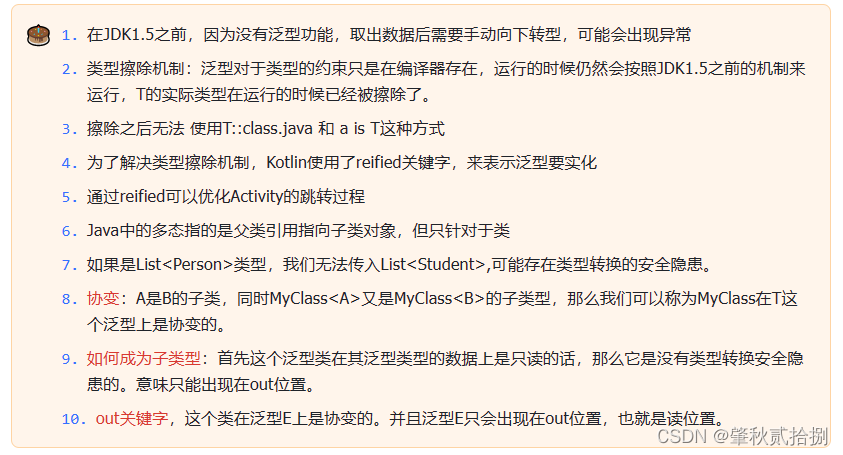
inline fun <reified T> startActivity(context :Context){
val intent = Intent(context , T::class.java)
context.startActivity(intent)
}
public interface MyList<out E> : Collection<E>{}
public operator fun get(index : Int) : E 这里泛型E出现在返回值的地方,所以是out位置
override fun contain(element : @UnsafeVariance : E ) : Boolean 这里泛型E出现在in位置,所以会报错
11. 使用协程编写高效的并发程序
//2
GlobalScope.launch{
println("codes run in courtines scope")
delay(1500)
}
//4
runBlocking{
println("....")
delay(1500)
printlb("...")
}
//5
runBlocking{
launch{
println("...")
delay(1000)
println("launch1 finished")
}
launch{
println("...")
delay(1000)
println("launch2 finished")
}
}
//8
suspend fun printDot(){
println(".")
delay(1000)
}
//10
suspend fun printDot() = coroutineScope{
launch{
println(".")
delay(1000)
}
}
//14
val job = GlobalScope.launch{
//处理具体的逻辑
}
job.cancel()
//15
val job = Job()
val scope = GlobalScope(job)
scope.launch{
//处理具体逻辑
}
job.cancel //可以将同一个作用域内的所有协程全部取消
//17
val deferred1 = async{
delay(1000)
5 + 5
}
val deferred2 = async{
delay(1000)
4 + 6
}
deferred1.await() + deferred2.await() 并行输出结果
//18
val result = withContext(Dispatchers.IO){
makeNetwork() //在IO线程中执行网络请求等耗时操作
}