插入
插入语句 string insertSte="insert into 表名 values(数据);";
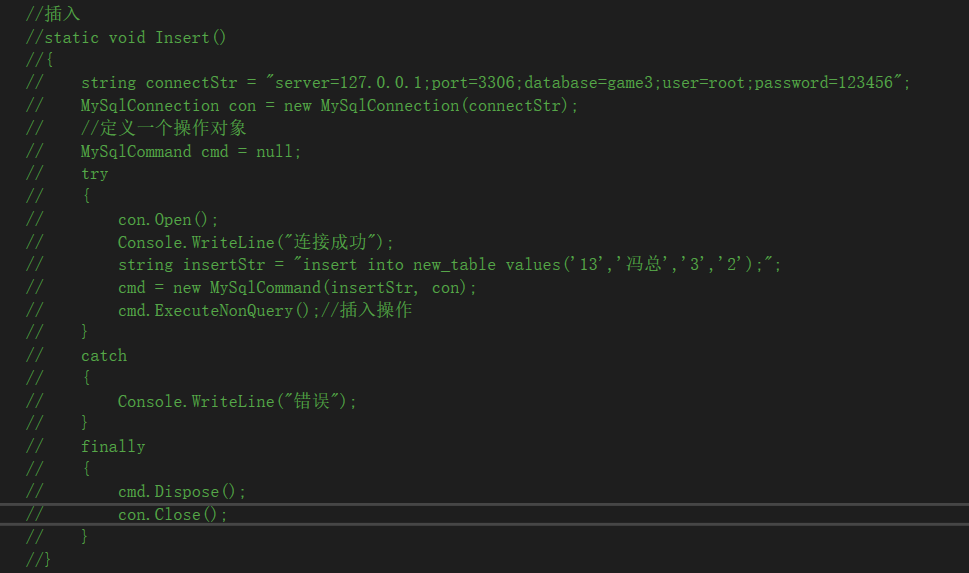
static void Insert()
{
string connectStr = "server=127.0.0.1;port=3306;database=game3;user=root;password=123456";
MySqlConnection con = new MySqlConnection(connectStr);
//定义一个操作对象
MySqlCommand cmd = null;
try
{
con.Open();
Console.WriteLine("连接成功");
string insertStr = "insert into new_table values('13','冯总','3','2');";
cmd = new MySqlCommand(insertStr, con);
cmd.ExecuteNonQuery();//插入操作
}
catch
{
Console.WriteLine("错误");
}
finally
{
cmd.Dispose();
con.Close();
}
}
删除
删除语句 string deleteStr="delete from 表名 where ××=××;";
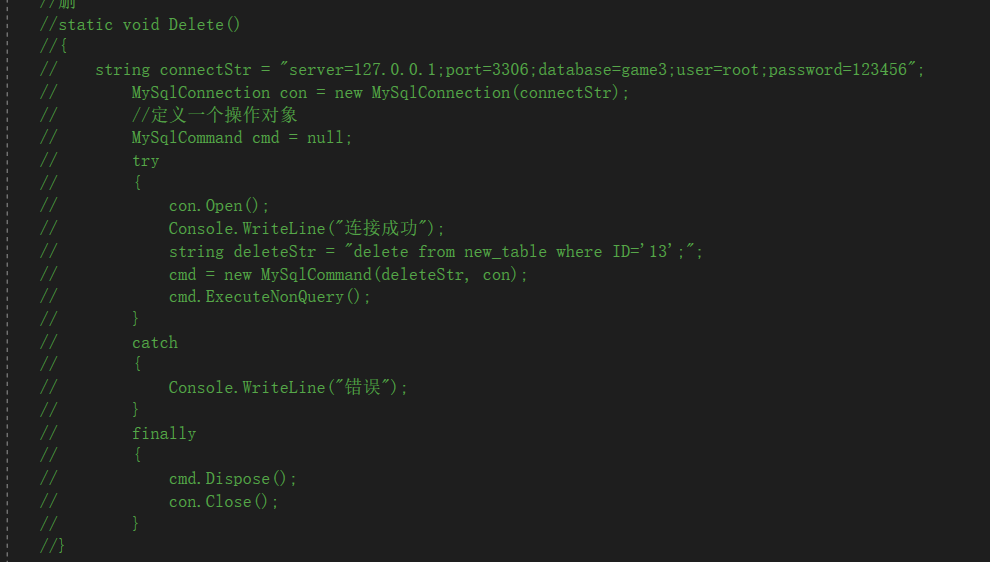
static void Delete()
{
string connectStr = "server=127.0.0.1;port=3306;database=game3;user=root;password=123456";
MySqlConnection con = new MySqlConnection(connectStr);
//定义一个操作对象
MySqlCommand cmd = null;
try
{
con.Open();
Console.WriteLine("连接成功");
string deleteStr = "delete from new_table where ID='13';";
cmd = new MySqlCommand(deleteStr, con);
cmd.ExecuteNonQuery();
}
catch
{
Console.WriteLine("错误");
}
finally
{
cmd.Dispose();
con.Close();
}
}
3.修改
修改语句 string updateStr="update 表名 set ××=×× where ××=××"
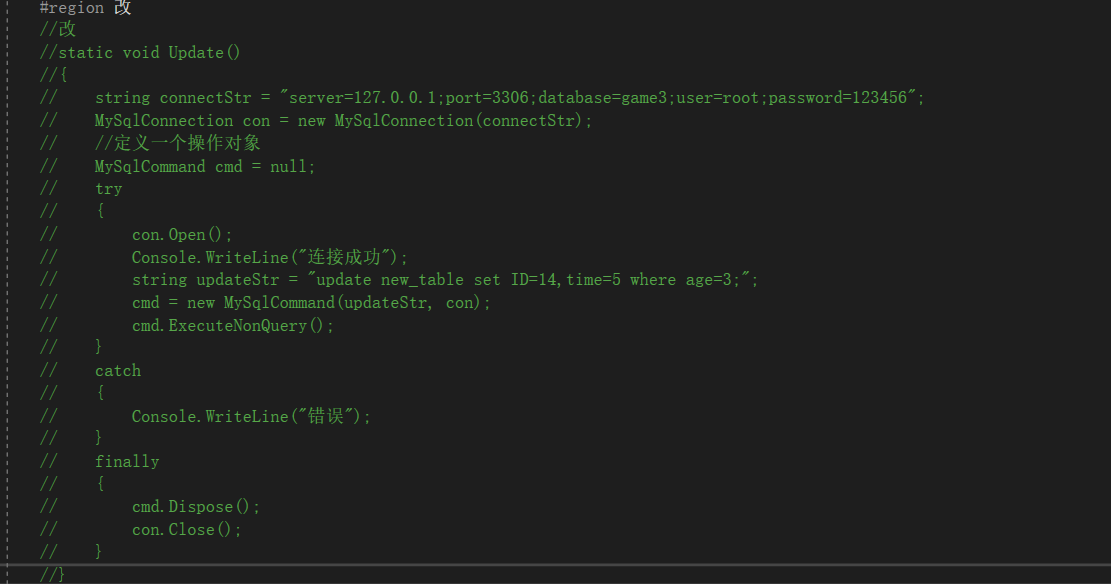
static void Update()
{
string connectStr = "server=127.0.0.1;port=3306;database=game3;user=root;password=123456";
MySqlConnection con = new MySqlConnection(connectStr);
//定义一个操作对象
MySqlCommand cmd = null;
try
{
con.Open();
Console.WriteLine("连接成功");
string updateStr = "update new_table set ID=14,time=5 where age=3;";
cmd = new MySqlCommand(updateStr, con);
cmd.ExecuteNonQuery();
}
catch
{
Console.WriteLine("错误");
}
finally
{
cmd.Dispose();
con.Close();
}
}
4.查询
查询语句 string querStr="select ×× ×× ×× ×× from 表名;";
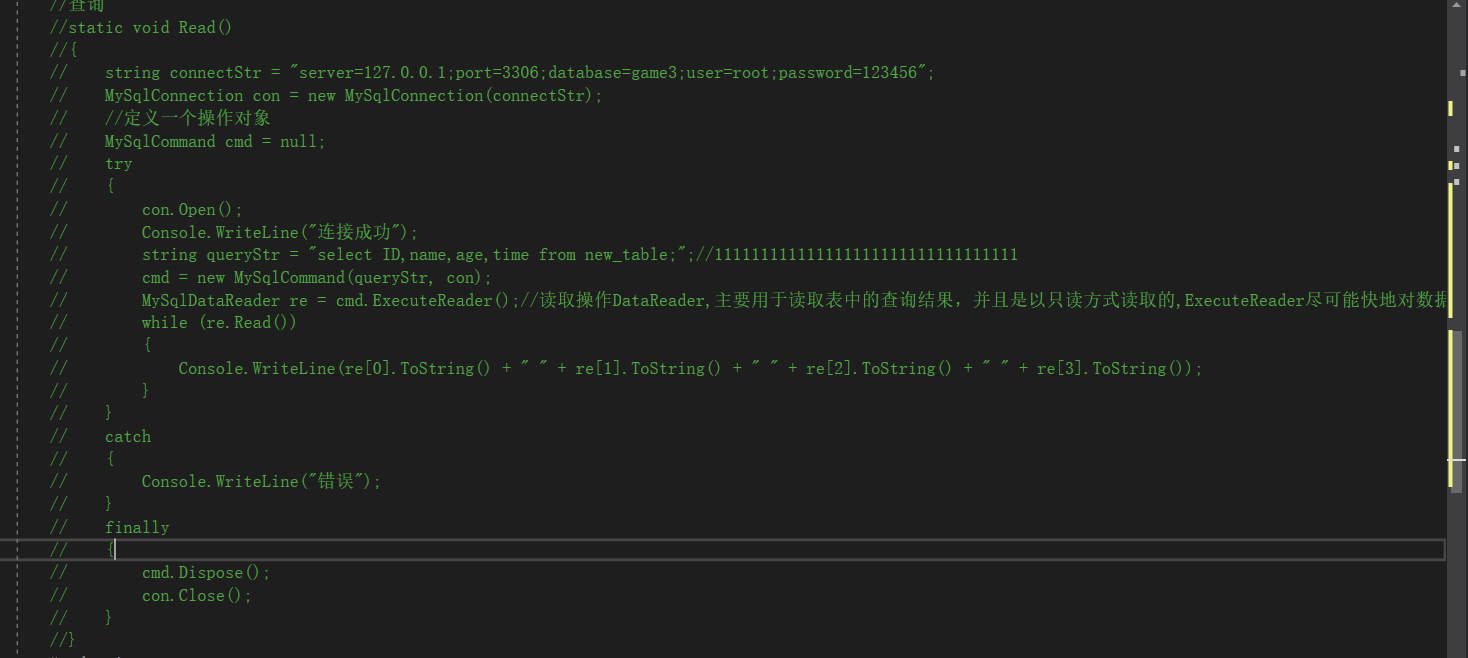
static void Read()
{
string connectStr = "server=127.0.0.1;port=3306;database=game3;user=root;password=123456";
MySqlConnection con = new MySqlConnection(connectStr);
//定义一个操作对象
MySqlCommand cmd = null;
try
{
con.Open();
Console.WriteLine("连接成功");
string queryStr = "select ID,name,age,time from new_table;";//111111111111111111111111111111111
cmd = new MySqlCommand(queryStr, con);
MySqlDataReader re = cmd.ExecuteReader();//读取操作DataReader,主要用于读取表中的查询结果,并且是以只读方式读取的,ExecuteReader尽可能快地对数据库进行查询并得到结果
while (re.Read())
{
Console.WriteLine(re[0].ToString() + " " + re[1].ToString() + " " + re[2].ToString() + " " + re[3].ToString());
}
}
catch
{
Console.WriteLine("错误");
}
finally
{
cmd.Dispose();
con.Close();
}
}