测试数据AB^CD^^^E^^
这是建立的树
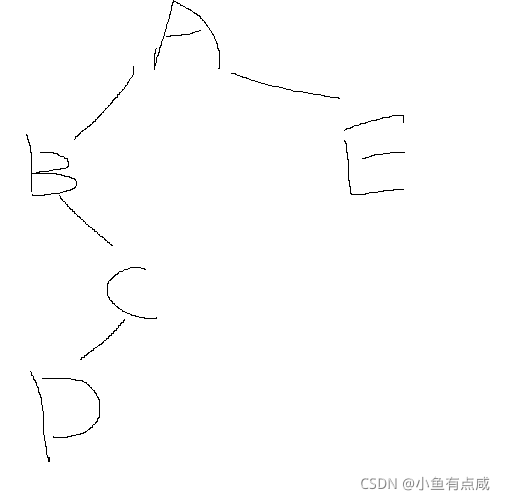
代码:
#include <stdio.h>
#include <iostream>
#include <stack>
#include <queue>
#include <malloc.h>
using namespace std;
#define MAXSIZE 100
typedef struct Node
{
char date;
struct Node* Lchild;
struct Node* Rchild;
}BiTNode,*BiTree;
void CreatBiTree(BiTree* root);
void PostOrder(BiTree root);
void PreOrder(BiTree root);
void LeveOrder(BiTree root);
void POrder(BiTree root);
void Inrder(BiTree root);
int top = -1;
int main()
{
BiTree tree;
cout << "扩展先序序列建立二叉树,^代表空" << endl;
CreatBiTree(&tree);
cout << "创建成功" << endl;
cout << "递归先序" << endl;
POrder(tree);
cout << endl;
cout << "递归中序" << endl;
Inrder(tree);
cout << endl;
cout << "递归后序" << endl;
PostOrder(tree);
cout << endl;
cout << "非递归先序" << endl;
PreOrder(tree);
cout << endl;
cout << "层次遍历" << endl;
LeveOrder(tree);
return 0;
}
void CreatBiTree(BiTree* root) //扩展先序序列创建二叉树
{
char ch;
scanf("%c", &ch);
if (ch == '^')
{
*root = NULL;
}
else
{
*root = (BiTree)malloc(sizeof(BiTNode));
(*root)->date = ch;
CreatBiTree(&((*root)->Lchild));
CreatBiTree(&((*root)->Rchild));
}
}
void PostOrder(BiTree root)//后续递归遍历
{
if (root)
{
PostOrder(root->Lchild);
PostOrder(root->Rchild);
printf("%c", root->date);
}
}
void POrder(BiTree root)//先续递归遍历
{
if (root)
{
printf("%c", root->date);
PostOrder(root->Lchild);
PostOrder(root->Rchild);
}
}
void Inrder(BiTree root)//中续递归遍历
{
if (root)
{
PostOrder(root->Lchild);
printf("%c", root->date);
PostOrder(root->Rchild);
}
}
//非递归先序
void PreOrder(BiTree root)
{
stack<BiTNode*>S;
BiTree p;
p = root;
while (p != NULL || !S.empty())
{
while (p)
{
S.push(p);
//Push(S, p);
p = p->Lchild;//一直找到左子树,将根节点和左子树入栈
}
if (!S.empty())
{
BiTree top1; //栈顶即为最后的左子树
top1 = S.top();
p = top1;//保存p指针,防止出栈后指针丢失
printf("%c",*top1);
S.pop();//出栈
//Pop(S, p);
p = p->Rchild;//找右孩子,与左子树入栈同理
}
}
}
//层次遍历
void LeveOrder(BiTree root)
{
queue<BiTNode*>Q;
BiTree p;
p = root;
Q.push(root);
while (!Q.empty())
{
BiTree root1;
root1 = Q.front();//从根节点开始入队
printf("%c",root1->date);
p = root1;//记住该根节点
Q.pop();
if (p->Lchild != NULL)//依次找该根节点的左右孩子
{
Q.push(p->Lchild);
}
if (p->Rchild != NULL)
{
Q.push(p->Rchild);
}
}
}
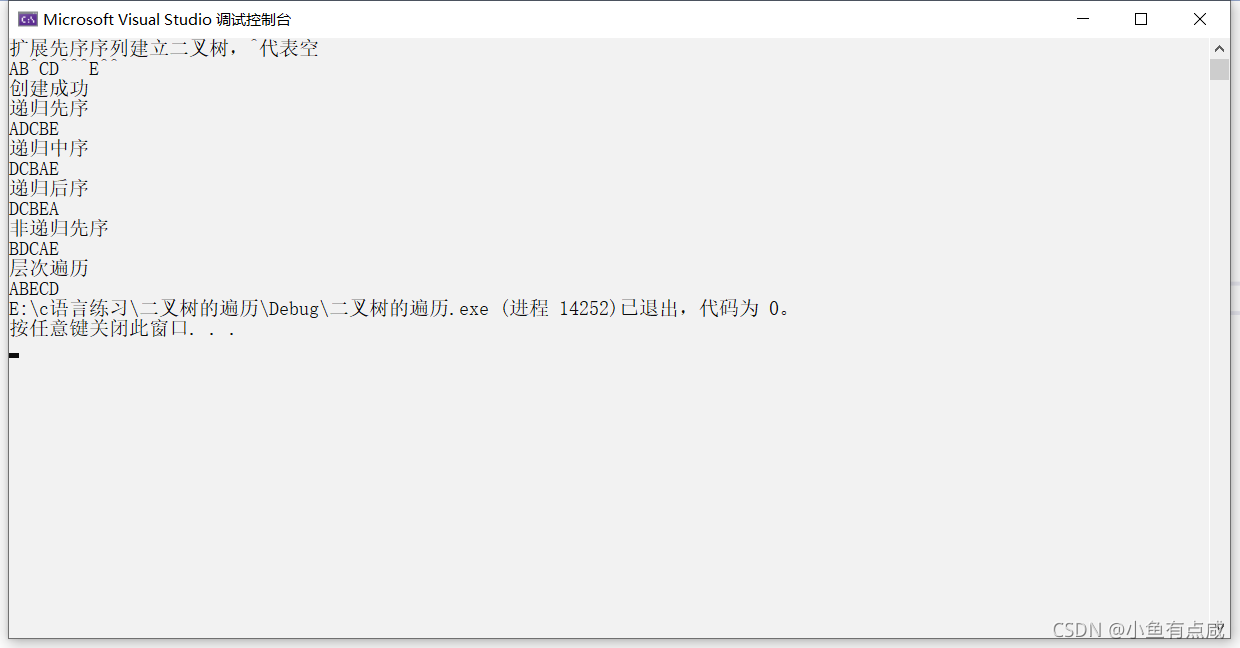