springcloud openfeign
概念:
- openfeign是springcloud的通讯组件
- openfeign以http的形式调用rest接口,但openfeign的使用更像prc
- openfeigin内部集成了ribbon,实现负载均衡
openfeign的使用
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
- 在服务消费者应用的启动类开启openfeign配置
@EnableFeignClients
/**
* @author liouwb
*/
@EnableEurekaClient
@SpringBootApplication
// 开启openfeign客户端
@EnableFeignClients(basePackages = "com.*")
@MapperScan(basePackages = {"com.mapper"})
@ComponentScan(basePackages = {"com.*"})
public class Application extends SpringBootServletInitializer {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-qjrksSOf-1649646456841)(1A60E91967E0465A8A89802042414A6B)]](https://i-blog.csdnimg.cn/blog_migrate/63af145f559b9f04eea1ac0fb67cdd7a.png)
- 定义openfegin业务调用接口
- Feign 不支持对 SpringMVC 注解的支持,OpenFeign支持
/**
* @author liouwb
* value是接口提供者注册到eureka的名字
*/
@FeignClient(value = "server-api")
public interface UserClient {
/**
* 通过应用编码查询应用信息
*
* @param applicationCode
* @return
* @GetMapping("user/{userId}") 路径是服务提供者的接口路径,参数和方法与服务提供者一直
*@RequestParam(value = "userId") value必须指定
*/
@GetMapping("user/{userId}")
UserVo getUser(@RequestParam(value = "userId") Long userId);
/**
* @author liouwb
*/
@RestController
@Api(tags = "2.用户")
@ApiSort(2)
public class UserController {
@Autowired
private UserClient userClinet;
@PostMapping("user/{userId}")
@ApiOperationSupport(order = 1, author = "liouwb")
@ApiOperation(value = "根据用户id获取用户新奇")
public UserVo getUserInfo(@PathVariable(value = "userId") Long userId) {
return userClinet.getUser(userId);
}
openfeign配置方式
- 使用openfeign client的配置
- 使用ribbon的配置
- feign配置是在ribbon配置的基础上做了扩展
- 配置优先级Feign局部配置 > Feign全局配置 > Ribbon局部配置 > Ribbon全局配置
openfeign feign配置方式
- 在服务消费服务配置openfeign
application.yml
- 可以在
FeignClientProperties
类里面FeignClientConfiguration
类查看配置项和默认值
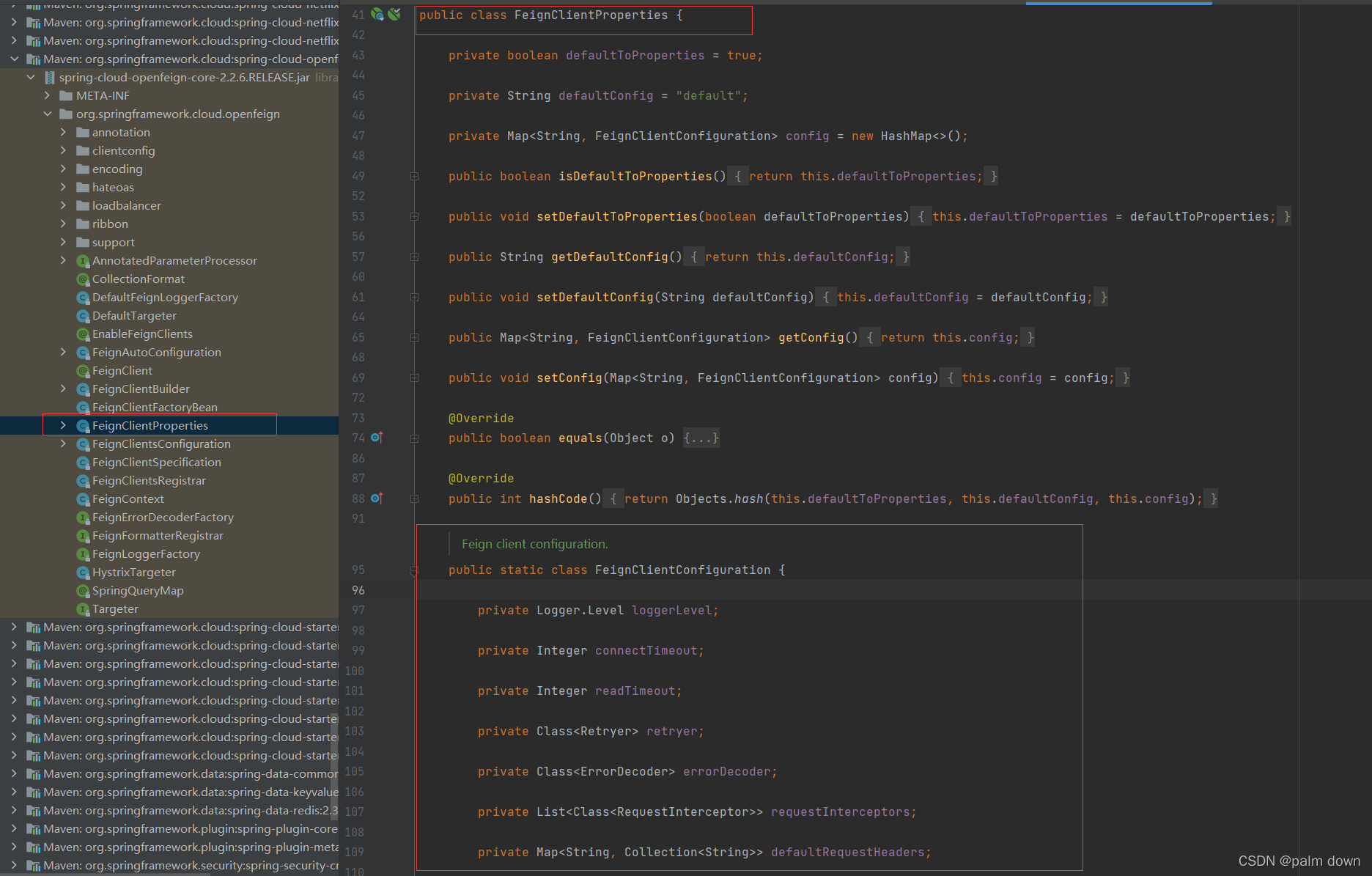
feign:
client:
config:
feignName:
connectTimeout: 5000
readTimeout: 5000
loggerLevel: full
errorDecoder: com.example.SimpleErrorDecoder
retryer: com.example.SimpleRetryer
defaultQueryParameters:
query: queryValue
defaultRequestHeaders:
header: headerValue
requestInterceptors:
- com.example.FooRequestInterceptor
- com.example.BarRequestInterceptor
decode404: false
encoder: com.example.SimpleEncoder
decoder: com.example.SimpleDecoder
contract: com.example.SimpleContract
capabilities:
- com.example.FooCapability
- com.example.BarCapability
queryMapEncoder: com.example.SimpleQueryMapEncoder
metrics.enabled: false
openfeign ribbon配置方式
- 在服务消费服务配置openfeign
application.yml
- 可以在
RibbonClientConfiguration
类里面查看配置项和默认值
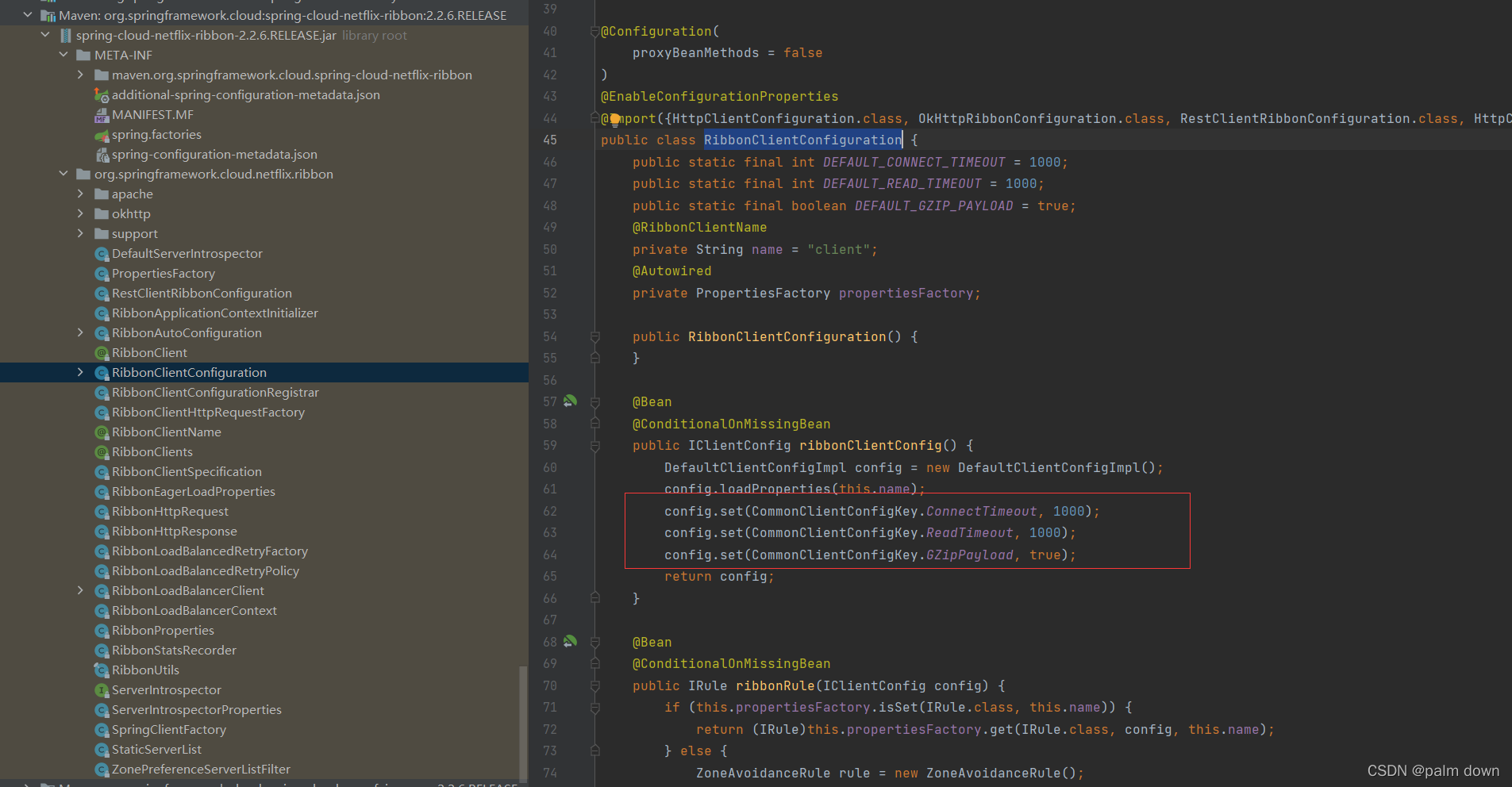
ribbon:
# 默认是1000毫秒,1分钟
ReadTimeout: 10000
# 最大重试次数
MaxAutoRetries: 1
# 刷新ribbonServerList缓存的时间 默认是30秒
ServerListRefreshInterval: 2000
- serverList刷新在
PollingServerListUpdater
类
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-kFrRzGaW-1649646456843)(8DFB2D396D8B4C2092811AD13C1C980B)]
openfeign文件上传
@ApiOperationSupport(order = 1, author = "刘斌")
@ApiOperation("上传文件")
@PostMapping("upload/file")
@ApiImplicitParam(name = "files", value = "上传的文件", dataType = "java.io.File", required = true)
public String uploadFile(@RequestPart("files") MultipartFile[] file) {
return "上传成功");
}
/**
* @author liouwb
*/
@FeignClient(value = "file-server")
public interface FileClient {
/**
* 上传登录用户头像
*
* @param file 头像文件
* @return
*/
@PostMapping(value = "upload/file", consumes = MediaType.MULTIPART_FORM_DATA_VALUE)
String uploadFile(@RequestPart("files") MultipartFile[] file);
/**
*
* @author liouwb
*/
@RestController
@ApiSort(1)
@Api(tags = "公共组件")
public class CommonController {
@Autowired
private FileClient fileClient;
@ApiOperationSupport(order = 1, author = "刘斌")
@ApiOperation("文件上传")
@PostMapping("upload")
@ApiImplicitParam(name = "files", value = "上传的文件", dataType = "java.io.File", required = true)
public String upload(@RequestPart("files") MultipartFile[] file) {
return fileClient.uploadFile(file);
}