1、首先安装vue-router
npm install vue-router@4
2、配置路由:在src下新建router文件夹,再建index.js其内容如下:
import Home from "../components/Home.vue"
import Item from "../components/Item.vue"
import Outer from "../components/Outer.vue"
import Center from "../components/Center.vue"
import OuterItem from "../components/OuterItem.vue"
import {createRouter, createWebHashHistory} from "vue-router";
const routes = [
{
path: '/',
component: Home,
children:[
{path:"/:id", component: Item},
{
path:"/outer",
component: Outer,
children:[
{path:"/outer/:index", component: OuterItem},
]
},
{path:"/center", component: Center},
]
},
]
const router = createRouter({
history: createWebHashHistory(),
routes,
})
export default router
3、在main.js中导入并挂载路由
import { createApp } from 'vue'
import App from './App.vue'
import router from "@/router";
createApp(App).use(Vant).use(UI).use(router).mount('#app')
4、依次创建
4.1、App.vue,其内容为
<template>
<RouterView />
</template>
<script setup>
</script>
<style scoped lang="less">
</style>
4.2、Center.vue,其内容为
<template>
<div>
<div class="outer">Center</div>
</div>
</template>
<script>
export default {
name: "Item"
}
</script>
<style scoped>
.outer{
text-align: center;
font-size: 40px;
border-bottom: 1px solid grey;
}
.outer-detail{
text-align: center;
}
</style>
4.3、OuterItem.vue,其内容为
<template>
<div class="outer">OuterItem</div>
</template>
<script>
export default {
name: "Item"
}
</script>
<style scoped>
.outer{
text-align: center;
font-size: 30px;
border-bottom: 1px solid grey;
}
</style>
4.4、Outer.vue,其内容为
<template>
<div>
<div class="outer">Outer</div>
<div class="outer-detail">
<router-link to="/outer/1">到自己的详情目录</router-link>
</div>
<RouterView></RouterView>
</div>
</template>
<script>
export default {
name: "Item"
}
</script>
<style scoped>
.outer{
text-align: center;
font-size: 40px;
border-bottom: 1px solid grey;
}
.outer-detail{
text-align: center;
}
</style>
4.5、Item.vue,其内容为
<template>
<div>item{{$route.params.id}}</div>
</template>
<script>
export default {
name: "Item"
}
</script>
<style scoped>
</style>
4.6、Home.vue,其内容为
<template>
<div class="container">
<div class="left">
<div><router-link active-class="active" to="/outer">内裹RouterView</router-link></div>
<div><router-link exact-active-class="active" to="/1">Outer</router-link></div>
<div><router-link exact-active-class="active" to="/center">each-item</router-link></div>
<div><router-link to="">each-item</router-link></div>
<div><router-link to="">each-item</router-link></div>
<div><router-link to="">each-item</router-link></div>
<div><router-link to="">each-item</router-link></div>
<div><router-link to="">each-item</router-link></div>
<div><router-link to="">each-item</router-link></div>
<div><router-link to="">each-item</router-link></div>
</div>
<div class="right">
<RouterView></RouterView>
</div>
</div>
</template>
<script>
export default {
name: "Home"
}
</script>
<style scoped lang="less">
.container{
width: 100%;
//background-color: pink;
display: flex;
color: black;
//flex-wrap: wrap;
.left{
width: 200px;
&>div{
//width: 200px;
width: 100%;
height: 100px;
line-height: 100px;
text-align: center;
//background-color: pink;
border-bottom: 1px solid;
border-right: 1px solid pink;
box-sizing: border-box;
a{
font-size: 20px;
color: black;
position: relative;
&.active{
color: #27BA9B;
&:before {
display: block;
}
}
&:before {
content: "";
display: none;
width: 6px;
height: 6px;
border-radius: 50%;
position: absolute;
top: 10px;
left: -10px;
background-color: #27BA9B;
}
}
&:last-child {
border-bottom: none;
}
}
}
.right{
flex: 1;
//width: 100px;
background-color: pink;
}
}
</style>
5、页面展示的效果如下:
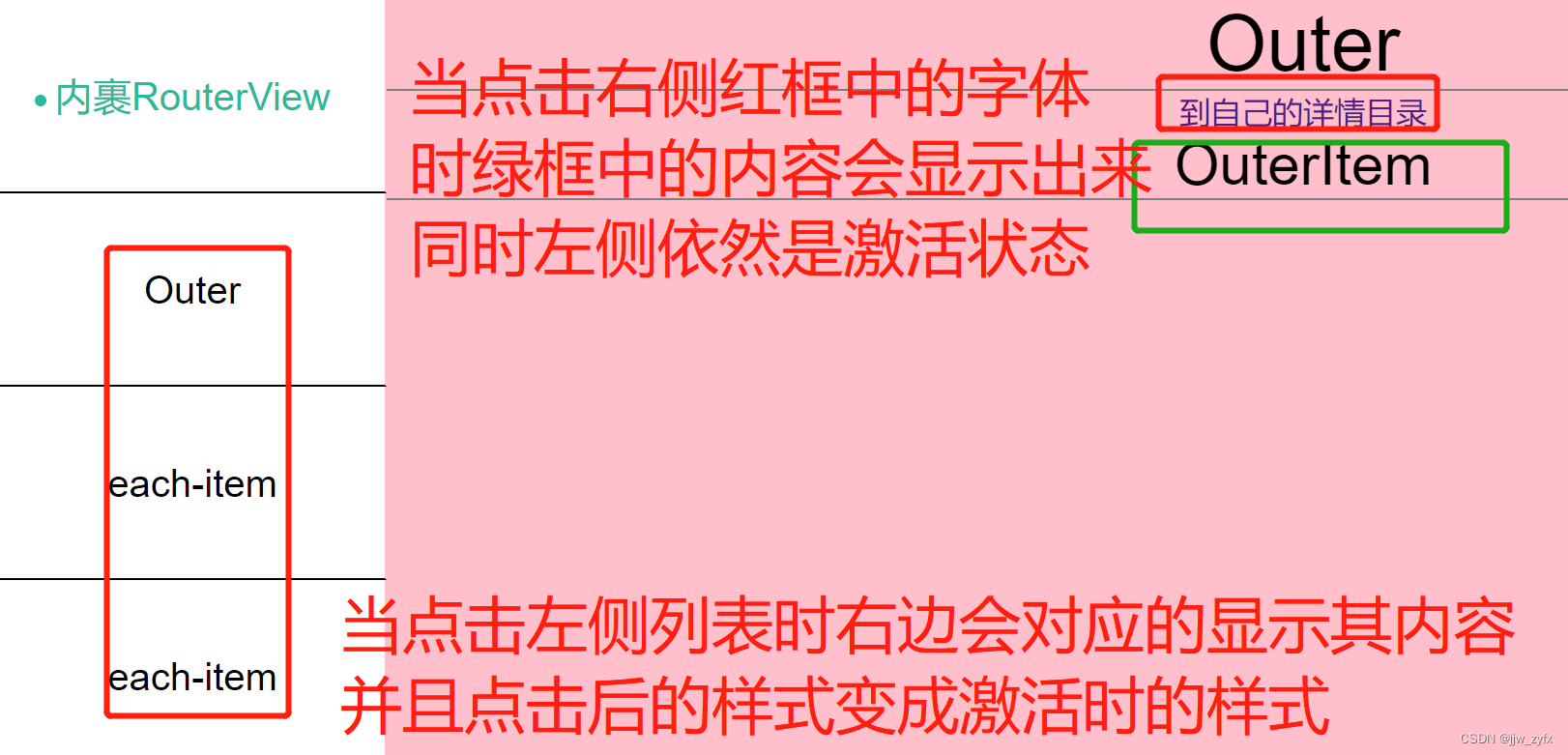
点击其他按钮右侧内容会改变
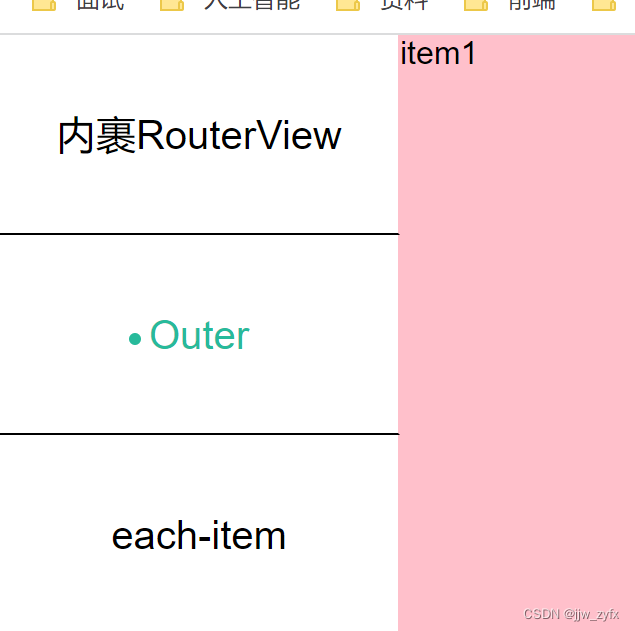