我们在项目中经常会遇到这样一个应用场景:执行某个耗时操作时,为了安抚用户等待的烦躁心情我们一般会使用进度条之类的空间,在android中让大家最 容易想到的就是progressbar或者progressDialog,区别在于前者是一个控件,后者是对话框。由于一些需求在弹出进度条时不希望用户 能够操作其他控件,所以只能使用progressDialog,这个时候有遇到了一个问题,我不想要progressDialog的黑色框框,感觉这样跟 应用的整体风格不协调,这个时候就考虑了写一个自定义的progressDialog。
在网上搜过很多自定义progressDialog的例子,对着写了下,但是没有任何效果,不知道是自己使用的方法不对还是什么地方出错了。通过不断的查找资料,写了一个简单的自定义progressDialog。先上图看下效果:
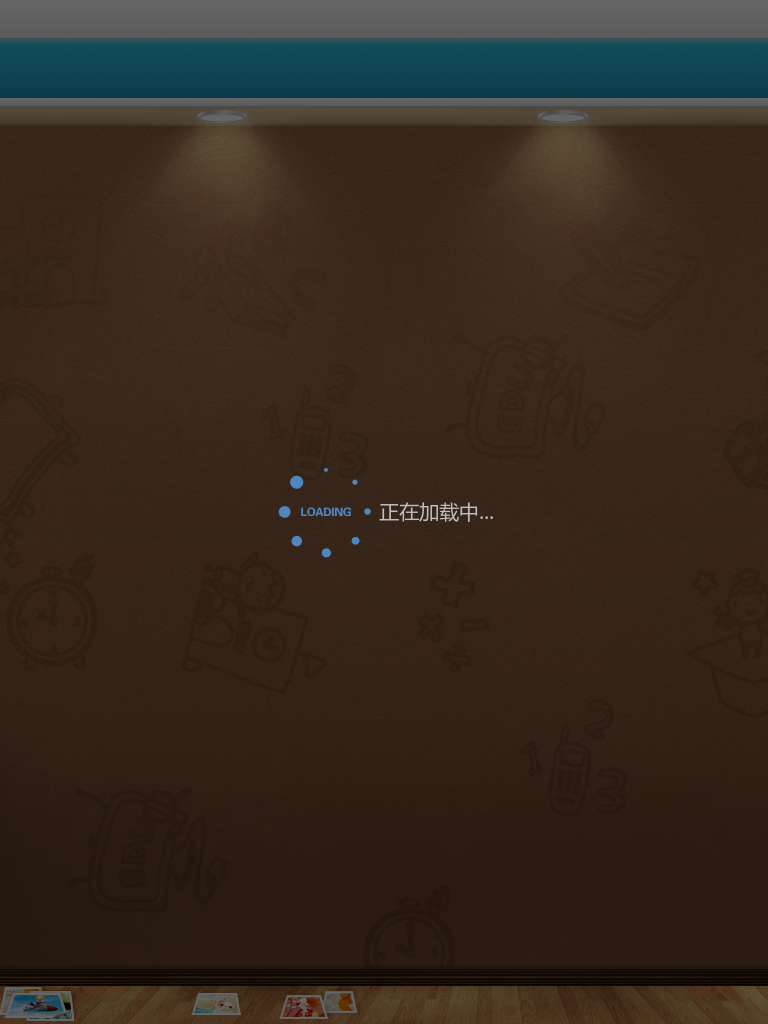
1.String.xml 文件,progressDialog是继承与Dialog,先设置一下progressDialog的风格,设置背景透明色。
01 |
< style name = "CustomDialog" parent = "@android:style/Theme.Dialog" >
|
02 |
< item name = "android:windowFrame" >@null</ item >
|
03 |
< item name = "android:windowIsFloating" >true</ item >
|
04 |
< item name = "android:windowContentOverlay" >@null</ item >
|
05 |
< item name = "android:windowAnimationStyle" >@android:style/Animation.Dialog</ item >
|
06 |
< item name = "android:windowSoftInputMode" >stateUnspecified|adjustPan</ item >
|
09 |
< style name = "CustomProgressDialog" parent = "@style/CustomDialog" >
|
10 |
< item name = "android:windowBackground" >@android:color/transparent</ item >
|
11 |
< item name = "android:windowNoTitle" >true</ item >
|
2.customprogressdialog.xml文件,定义自己的布局,由于我的需求只需要一个进度条以及一串显示的内容,所以布局比较接单
01 |
<? xml version = "1.0" encoding = "utf-8" ?>
|
03 |
xmlns:android = "http://schemas.android.com/apk/res/android" |
04 |
android:layout_width = "fill_parent" |
05 |
android:layout_height = "fill_parent" |
06 |
android:orientation = "horizontal" >
|
08 |
android:id = "@+id/loadingImageView" |
09 |
android:layout_width = "wrap_content" |
10 |
android:layout_height = "wrap_content" |
11 |
android:background = "@anim/progress_round" />
|
13 |
android:id = "@+id/id_tv_loadingmsg" |
14 |
android:layout_width = "wrap_content" |
15 |
android:layout_height = "wrap_content" |
16 |
android:layout_gravity = "center_vertical" |
17 |
android:textSize = "20dp" />
|
3.progress_round.xml文件.这个文件为了实现转动的效果,循环显示这些图片。
01 |
<? xml version = "1.0" encoding = "utf-8" ?>
|
03 |
xmlns:android = "http://schemas.android.com/apk/res/android" |
04 |
android:oneshot = "false" >
|
05 |
< item android:drawable = "@drawable/progress_1" android:duration = "200" />
|
06 |
< item android:drawable = "@drawable/progress_2" android:duration = "200" />
|
07 |
< item android:drawable = "@drawable/progress_3" android:duration = "200" />
|
08 |
< item android:drawable = "@drawable/progress_4" android:duration = "200" />
|
09 |
< item android:drawable = "@drawable/progress_5" android:duration = "200" />
|
10 |
< item android:drawable = "@drawable/progress_6" android:duration = "200" />
|
11 |
< item android:drawable = "@drawable/progress_7" android:duration = "200" />
|
12 |
< item android:drawable = "@drawable/progress_8" android:duration = "200" />
|
4.CustomProgressDialog.java文件,这个是就是我们最终需要使用的progressDialog了。
01 | /************************************************************************************** |
07 | * CustomProgressDialog.java |
09 | * Copyright 2012 LXD All Rights Reserved. |
11 | * Version Date Author Record |
12 | *-------------------------------------------------------------------------------------- |
13 | * 1.0.0 2012-4-27 lxd (rohsuton@gmail.com) Create |
14 | **************************************************************************************/ |
16 |
package com.lxd.widgets;
|
19 |
import com.lxd.activity.R;
|
21 |
import android.app.Dialog;
|
22 |
import android.content.Context;
|
23 |
import android.graphics.drawable.AnimationDrawable;
|
24 |
import android.view.Gravity;
|
25 |
import android.widget.ImageView;
|
26 |
import android.widget.TextView;
|
29 | /******************************************************************** |
31 |
* TODO 请在此处简要描述此类所实现的功能。因为这项注释主要是为了在IDE环境中生成tip帮助,务必简明扼要
|
33 |
* TODO 请在此处详细描述类的功能、调用方法、注意事项、以及与其它类的关系.
|
34 |
*******************************************************************/
|
36 |
public class CustomProgressDialog extends Dialog {
|
37 |
private Context context = null ;
|
38 |
private static CustomProgressDialog customProgressDialog = null ;
|
40 |
public CustomProgressDialog(Context context){
|
42 |
this .context = context;
|
45 |
public CustomProgressDialog(Context context, int theme) {
|
46 |
super (context, theme);
|
49 |
public static CustomProgressDialog createDialog(Context context){
|
50 |
customProgressDialog = new CustomProgressDialog(context,R.style.CustomProgressDialog);
|
51 |
customProgressDialog.setContentView(R.layout.customprogressdialog);
|
52 |
customProgressDialog.getWindow().getAttributes().gravity = Gravity.CENTER;
|
54 |
return customProgressDialog;
|
57 |
public void onWindowFocusChanged( boolean hasFocus){
|
59 |
if (customProgressDialog == null ){
|
63 |
ImageView imageView = (ImageView) customProgressDialog.findViewById(R.id.loadingImageView);
|
64 |
AnimationDrawable animationDrawable = (AnimationDrawable) imageView.getBackground();
|
65 |
animationDrawable.start();
|
76 |
public CustomProgressDialog setTitile(String strTitle){
|
77 |
return customProgressDialog;
|
88 |
public CustomProgressDialog setMessage(String strMessage){
|
89 |
TextView tvMsg = (TextView)customProgressDialog.findViewById(R.id.id_tv_loadingmsg);
|
92 |
tvMsg.setText(strMessage);
|
95 |
return customProgressDialog;
|
5.接下来就是写一个测试activity调用我们的progressDialog了。
01 |
package com.lxd.activity;
|
03 |
import com.lxd.widgets.CustomProgressDialog;
|
05 |
import android.app.Activity;
|
06 |
import android.os.AsyncTask;
|
07 |
import android.os.Bundle;
|
08 |
import android.view.Window;
|
09 |
import android.view.WindowManager;
|
11 |
public class MainFrame extends Activity {
|
12 |
private MainFrameTask mMainFrameTask = null ;
|
13 |
private CustomProgressDialog progressDialog = null ;
|
16 |
public void onCreate(Bundle savedInstanceState) {
|
17 |
super .onCreate(savedInstanceState);
|
18 |
this .requestWindowFeature(Window.FEATURE_NO_TITLE);
|
19 |
this .getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN,
|
20 |
WindowManager.LayoutParams.FLAG_FULLSCREEN);
|
22 |
setContentView(R.layout.main);
|
24 |
mMainFrameTask = new MainFrameTask( this );
|
25 |
mMainFrameTask.execute();
|
29 |
protected void onDestroy() {
|
32 |
if (mMainFrameTask != null && !mMainFrameTask.isCancelled()){
|
33 |
mMainFrameTask.cancel( true );
|
39 |
private void startProgressDialog(){
|
40 |
if (progressDialog == null ){
|
41 |
progressDialog = CustomProgressDialog.createDialog( this );
|
42 |
progressDialog.setMessage( "正在加载中..." );
|
45 |
progressDialog.show();
|
48 |
private void stopProgressDialog(){
|
49 |
if (progressDialog != null ){
|
50 |
progressDialog.dismiss();
|
51 |
progressDialog = null ;
|
55 |
public class MainFrameTask extends AsyncTask<Integer, String, Integer>{
|
56 |
private MainFrame mainFrame = null ;
|
58 |
public MainFrameTask(MainFrame mainFrame){
|
59 |
this .mainFrame = mainFrame;
|
63 |
protected void onCancelled() {
|
69 |
protected Integer doInBackground(Integer... params) {
|
72 |
Thread.sleep( 10 * 1000 );
|
73 |
} catch (InterruptedException e) {
|
80 |
protected void onPreExecute() {
|
81 |
startProgressDialog();
|
85 |
protected void onPostExecute(Integer result) {
|
这样我们需要的progressDialog效果就出来了