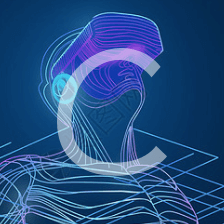
Leetcode
OString2024
个人公众号:OString2024
zhihu:Ostring
展开
-
Leetcode summary: Binary search
34. Find First and Last Position of Element in Sorted Arrayclass Solution(object): def searchRange(self, nums, target): """ :type nums: List[int] :type target: int ...原创 2020-07-07 11:26:58 · 149 阅读 · 0 评论 -
Leetcode sumary: Math problem
29. Divide Two Integersclass Solution(object): def divide(self, dividend, divisor): """ :type dividend: int :type divisor: int :rtype: int """ if (...原创 2019-11-18 11:50:15 · 166 阅读 · 0 评论 -
Leetcode sumary: minmax usage
486. Predict the Winner[375]. Guess Number Higherclass Solution(object): def getMoneyAmount(self, n): """ :type n: int :rtype: int """ dp=[[0 for i in r...原创 2019-11-18 11:49:44 · 136 阅读 · 0 评论 -
Leetcode summary: prime number problem
204. Count Primesclass Solution(object): def countPrimes(self, n): """ :type n: int :rtype: int """ if n<3: return 0 prime...原创 2019-11-18 11:48:34 · 158 阅读 · 0 评论 -
Leetcode summary: longest increase subsequece or substring or subarray
300. Longest Increasing SubsequenceGiven an unsorted array of integers, find the length of longest increasing subsequence.Example:Input: [10,9,2,5,3,7,101,18]Output: 4Explanation: The longest inc...原创 2019-11-18 11:44:45 · 116 阅读 · 0 评论 -
[算法] 二叉排序树查找中位数
比较tricky的解法,不用额外的内存首先考虑将二叉树转换成双向链表[LeetCode] 426. Convert Binary Search Tree to Sorted Doubly Linked List将二叉搜索树转为有序双向链表然后通过查找链表中间节点的方法找到中位数def doubleconvert(root): if not root: return None ...原创 2019-11-14 19:44:01 · 1133 阅读 · 0 评论 -
单链表的快速排序和归并排序
# -*- coding: UTF-8 -*-class TreeNode(object): def __init__(self,val,nex): self.val=val self.next=nexdef linksort(s,e): if s==e: return key=s.val p=s ...原创 2019-11-14 16:23:13 · 138 阅读 · 0 评论 -
Leetcode summary: wiggle problem
775. Global and Local InversionsWe have some permutation A of [0, 1, …, N - 1], where N is the length of A.The number of (global) inversions is the number of i < j with 0 <= i < j < N an...原创 2019-10-23 20:43:16 · 124 阅读 · 0 评论 -
Leetcode summary: array number in the range of array length
这类题一般有个前提:Given an array of integers, 1 ≤ a[i] ≤ n (n = size of array)442. Find All Duplicates in an Arrayclass Solution(object): def findDuplicates(self, nums): """ :type num...原创 2019-07-24 15:03:39 · 123 阅读 · 0 评论 -
Leetcode Summary: Union Find
721. Accounts Mergeclass Solution(object): def accountsMerge(self, accounts): def find(a): if ds[a] < 0: return a ds[a] = find(ds[a]) ...原创 2019-07-30 09:49:08 · 118 阅读 · 0 评论 -
LeetCode[24] Swap Nodes in Pairs
My solution:mark: not work# Definition for singly-linked list.# class ListNode(object):# def __init__(self, x):# self.val = x# self.next = Noneclass Solution(object): d...原创 2019-08-08 15:33:29 · 99 阅读 · 0 评论 -
Leetcode summary: backstrack problem
permutation77. Combinationsclass Solution(object): def combine(self, n, k): """ :type n: int :type k: int :rtype: List[List[int]] """ def backtra...原创 2019-08-14 20:39:54 · 144 阅读 · 0 评论 -
Leetcode[234] Palindrome Linked List
234. Palindrome Linked Listrecursion not work, stack will be overflowclass Solution(object): def isPalindrome(self, head): """ :type head: ListNode :rtype: bool "...原创 2019-08-10 23:16:28 · 106 阅读 · 0 评论 -
Leetcode [91]: Decode ways
91.Decode Waysdfs way:class Solution(object): def numDecodings(self, s): """ :type s: str :rtype: int """ out=[] self.ret=0 def h...原创 2019-08-15 20:20:13 · 125 阅读 · 0 评论 -
Leetcode summary: some trick problem
781. Rabbit in the forrest## @lc app=leetcode id=781 lang=python## [781] Rabbits in Forest#class Solution(object): def numRabbits(self, answers): """ :type answers: List[int]...原创 2019-08-13 09:35:25 · 204 阅读 · 0 评论 -
leetcode summary: link list problem solved by extra space
143. Reorder Listlink list ⇒ listclass Solution(object): def reorderList(self, head): """ :type head: ListNode :rtype: None Do not return anything, modify head in-place i...原创 2019-08-13 09:36:09 · 140 阅读 · 0 评论 -
Leetcode Binary Tree recursive and iterative summary
[572] Subtree of Another Treeclass Solution(object): def isSubtree(self, s, t): """ :type s: TreeNode :type t: TreeNode :rtype: bool """ if not s ...原创 2019-07-12 11:49:45 · 147 阅读 · 0 评论 -
Leetcode Summary: Graph
743. Network Delay TimeInput: times = [[2,1,1],[2,3,1],[3,4,1]], N = 4, K = 2Output: 2class Solution(object): def networkDelayTime(self, times, N, K): """ :type times: List[Lis...原创 2019-07-05 11:49:17 · 212 阅读 · 0 评论 -
Leetcode Palindrome problem summary
class Solution: def helper(self,s,l,r): while l>=0 and r<len(s) and s[l]==s[r]: l=l-1 r=r+1 return s[l+1:r] def longestPalindrome(self, s...原创 2019-05-05 16:07:49 · 131 阅读 · 0 评论 -
Leetcode Journey
数组按出现频次从大到小排序http://www.geeksforgeeks.org/amazon-interview-set-21/#include &amp;amp;amp;amp;amp;amp;amp;lt;stdio.h&amp;amp;amp;amp;amp;amp;amp;gt;typedef struct{int id;int count;}item;void sort(int a[], int n){item temp[n];原创 2018-05-23 10:42:14 · 221 阅读 · 0 评论 -
Leetcode[451] Sort Characters By Frequency Medium
Given a string, sort it in decreasing order based on the frequency of characters.Example 1:Input:“tree”Output:“eert”Explanation:‘e’ appears twice while ‘r’ and ‘t’ both appear once.So ‘e’ must...原创 2019-03-29 13:55:57 · 252 阅读 · 0 评论 -
Leetcode[136] Single Number
Given an array of integers, every element appears twice except for one. Find that single one.Note:Your algorithm should have a linear runtime complexity. Could you implement it without using extra m...原创 2019-03-29 17:59:08 · 107 阅读 · 0 评论 -
Leetcode[389] Find the Difference
Given two strings s and t which consist of only lowercase letters.String t is generated by random shuffling string s and then add one more letter at a random position.Find the letter that was added ...原创 2019-03-29 18:30:17 · 114 阅读 · 0 评论 -
Leetcode[344] Reverse String
Write a function that takes a string as input and returns the string reversed.Example:Given s = “hello”, return “olleh”.void inverse(int start, int end, char *p){ char temp; if(p) { temp=p[st...原创 2019-03-29 18:52:19 · 200 阅读 · 0 评论 -
Leetcode[25] Reverse Nodes in k-Group
增加一個pre 和next 節點進行鏈表内部的翻轉# Definition for singly-linked list.# class ListNode(object):# def __init__(self, x):# self.val = x# self.next = Noneclass Solution(object): def...原创 2019-04-01 08:57:35 · 102 阅读 · 0 评论 -
Leetcode summary: array element sum problem
Tag: Dictclass Solution(object): def twoSum(self, nums, target): """ :type nums: List[int] :type target: int :rtype: List[int] """ dic={} r...原创 2019-04-30 16:34:20 · 110 阅读 · 0 评论 -
Leetcode[2] Add Two Numbers and Leetcode[445] Add Two Numbers II
Leetcode[2] Add Two Numbersclass Solution(object): def addTwoNumbers(self, l1, l2): """ :type l1: ListNode :type l2: ListNode :rtype: ListNode """ ...原创 2019-04-30 17:16:05 · 196 阅读 · 0 评论 -
Leetcode[692] and[347] Heapq problem
本质上是一样的解决方案,利用python的heapq 进行解题[347] Top K Frequent Elementsclass Solution(object): def topKFrequent(self, nums, k): """ :type nums: List[int] :type k: int :rtype...原创 2019-06-12 18:09:11 · 280 阅读 · 0 评论 -
Leetcode keyboard problem [650] and [651]
4 keyboardclass Solution {public: int maxA(int N) { vector<int> dp(N + 1, 0); for (int i = 0; i <= N; ++i) { dp[i] = i; for (int j = 1; j < i ...原创 2019-06-04 15:44:09 · 127 阅读 · 0 评论 -
Leetcode summary: dynamic programing, [322] coin change & [474] ones and zeros
[322] coin changeclass Solution(object): def coinChange(self, coins, amount): """ :type coins: List[int] :type amount: int :rtype: int """ #init as...原创 2019-06-26 14:38:18 · 127 阅读 · 0 评论 -
Leetcode [740]. Delete and Earn & [213]. House Robber II
Take or Skip problem740. Delete and Earnclass Solution(object): def deleteAndEarn(self, nums): """ :type nums: List[int] :rtype: int """ dp=[0 for i in ra...原创 2019-06-28 16:35:10 · 105 阅读 · 0 评论 -
Leetcode summary: Monostone Stack problem
42. Trapping Rain Waterclass Solution(object): def trap(self, height): """ :type height: List[int] :rtype: int """ st=[] #use decrease stack here ...原创 2019-07-04 09:42:54 · 229 阅读 · 0 评论 -
Leetcode Summary: Ugly number
[264] Ugly Number IIclass Solution(object): def nthUglyNumber(self, n): """ :type n: int :rtype: int """ if n<=0: return 0 if n==1:...原创 2019-07-04 11:50:09 · 100 阅读 · 0 评论 -
Leetcode[138] Copy List with Random Pointer
an error:keyerror,class Solution(object): def copyRandomList(self, head): """ :type head: Node :rtype: Node """ if head==None: return None ...原创 2019-04-13 22:38:46 · 114 阅读 · 0 评论