这里写自定义目录标题
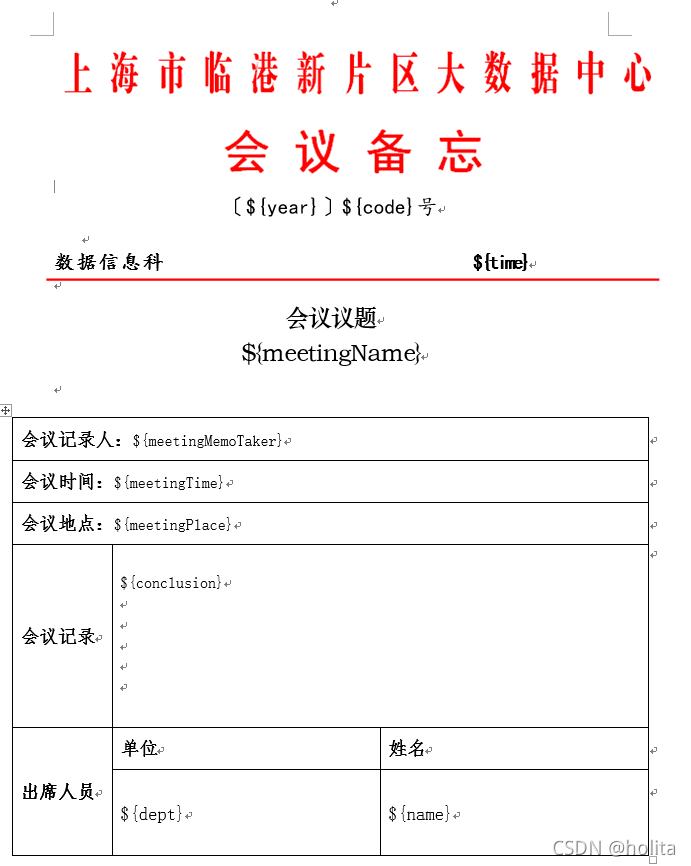
@Override
public String exportWordMemo(Long id) throws Exception {
String tmpPath = ResourceUtils.getURL("classpath:").getPath() + "static" + "/" + "doc" + "/" + "memo.doc";
String tmpPath2 = ResourceUtils.getURL("classpath:").getPath() + "static" + "/" + "doc" + "/" + "memo2.doc";
Map<String, Object> memo = memoMapper.selectMemoForWord(id);
List<MeetingMemoTaker> takers = meetingMemoTakerMapper.getMemoTaker(id);
Map<String, String> data = new HashMap<>();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy.MM.dd HH:mm");
SimpleDateFormat sdf1 = new SimpleDateFormat("yyyy年MM月dd日");
String date = null;
String date1 = null;
if (memo.get("MEETING_TIME") != null && !"".equals(memo.get("MEETING_TIME"))) {
date = sdf.format(memo.get("MEETING_TIME"));
data.put("${meetingTime}", date);
date1 = sdf1.format(memo.get("MEETING_TIME"));
data.put("${time}", date1);
} else {
data.put("${meetingTime}", "");
}
if (memo.get("MEETING_NAME") != null && !"".equals(memo.get("MEETING_NAME"))) {
data.put("${meetingName}", memo.get("MEETING_NAME").toString());
} else {
data.put("${meetingName}", "");
}
if (memo.get("MEETING_MEMO_TAKER") != null && !"".equals(memo.get("MEETING_MEMO_TAKER"))) {
data.put("${meetingMemoTaker}", memo.get("MEETING_MEMO_TAKER").toString());
} else {
data.put("${meetingMemoTaker}", "");
}
if (memo.get("MEETING_PLACE") != null && !"".equals(memo.get("MEETING_PLACE"))) {
data.put("${meetingPlace}", memo.get("MEETING_PLACE").toString());
} else {
data.put("${meetingPlace}", "");
}
if (memo.get("REMAINING_PROBLEMS") != null && !"".equals(memo.get("REMAINING_PROBLEMS"))) {
data.put("${remainingProblems}", memo.get("REMAINING_PROBLEMS").toString());
} else {
data.put("${remainingProblems}", "");
}
if (memo.get("CONCLUSION") != null && !"".equals(memo.get("CONCLUSION"))) {
String conclusion="";
Document document=Jsoup.parse(memo.get("CONCLUSION").toString());
conclusion=document.text();
data.put("${conclusion}", conclusion);
} else {
data.put("${conclusion}", "");
}
if (memo.get("MEETING_MEMO_NUM") != null && !"".equals(memo.get("MEETING_MEMO_NUM"))) {
data.put("${code}", memo.get("MEETING_MEMO_NUM").toString());
} else {
data.put("${code}", "");
}
MeetingMemoTaker[] memoTakers = new MeetingMemoTaker[takers.size()];
takers.toArray(memoTakers);
String nameList = "";
String deptList = "";
for (int i = 0; i < memoTakers.length; i++) {
nameList = nameList + memoTakers[i].getName() + (char) 11;
deptList = deptList + memoTakers[i].getDeptName() + (char) 11;
}
data.put("${name}", nameList);
data.put("${dept}", deptList);
data.put("${year}", String.valueOf(Calendar.getInstance().get(Calendar.YEAR)));
Set<Map.Entry<String, String>> entrySet = data.entrySet();
for (Map.Entry<String, String> entry : entrySet) {
System.out.println(entry.getKey() + "===" + entry.getValue());
}
File file = new File(tmpPath);
System.out.println(file.getAbsolutePath() + "\t" + file.exists());
HWPFDocument changWord2003 = WordToNewWordUtil.changWord2003(tmpPath, data);
FileOutputStream fileOutputStream = new FileOutputStream(tmpPath2);
changWord2003.write(fileOutputStream);
fileOutputStream.close();
return "success";
}
@Override
public String fileDownload(String type, HttpServletResponse response) throws Exception {
String wordName = "";
String fileName = "";
if ("1".equals(type)) {
wordName = "meeting2.doc";
fileName = "会议通知.doc";
} else if ("2".equals(type)) {
wordName = "memo2.doc";
fileName = "备忘录.doc";
} else if ("3".equals(type)) {
wordName = "weekly2.doc";
fileName = "周报.doc ";
}
String filePath = ResourceUtils.getURL("classpath:").getPath() + "static" + "/" + "doc" + "/" + wordName;
try {
File file = new File(filePath);
InputStream is = new BufferedInputStream(new FileInputStream(file));
byte[] buffer = new byte[is.available()];
is.read(buffer);
is.close();
response.reset();
response.addHeader("Content-Disposition", "attachment;filename=" + new String(fileName.getBytes(StandardCharsets.UTF_8), StandardCharsets.ISO_8859_1));
response.addHeader("Content-Length", "" + file.length());
OutputStream toClient = new BufferedOutputStream(response.getOutputStream());
response.setContentType("application/octet-stream");
toClient.write(buffer);
toClient.flush();
toClient.close();
return "";
} catch (Exception e) {
e.printStackTrace();
return "文件下载出错";
}
}
@RequestMapping("/exportWordMemo")
@ResponseBody
public String exportWordMemo(Long id){
String exportWordMemo = null;
try {
exportWordMemo = projectMeetingService.exportWordMemo(id);
} catch (Exception e) {
e.printStackTrace();
}
return exportWordMemo;
}
@RequestMapping("/fileDownload")
@ResponseBody
public String fileDownload(String type,HttpServletResponse response){
String str= null;
try {
str = projectMeetingService.fileDownload(type,response);
} catch (Exception e) {
e.printStackTrace();
}
return str;
}