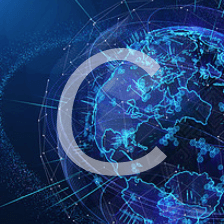
Python基础
HeatDeath
Learn by doing!
展开
-
python 内置数据结构的基本操作 —— list(2)
Lists may be constructed in several ways:Using a pair of square brackets to denote the empty list: []Using square brackets, separating items with commas: [a], [a, b, c]Using a list comprehension: [x转载 2017-05-09 10:06:28 · 720 阅读 · 0 评论 -
python 内置数据结构的基本操作 —— tuple(2)
Tuples are immutable sequences, typically used to store collections of heterogeneous data (such as the 2-tuples produced by the enumerate() built-in). Tuples are also used for cases where an immutable转载 2017-05-09 10:05:10 · 674 阅读 · 0 评论 -
python 内置数据结构的基本操作 —— Set(2)
class set([iterable])class frozenset([iterable])Return a new set or frozenset object whose elements are taken from iterable. The elements of a set must be hashable. To represent sets of sets, the inne转载 2017-05-09 10:02:43 · 519 阅读 · 0 评论 -
python 内置数据结构的基本操作 —— dict(2)
A mapping object maps hashable values to arbitrary objects. Mappings are mutable objects. There is currently only one standard mapping type, the dictionary. (For other containers see the built-in list,原创 2017-05-09 09:57:28 · 918 阅读 · 0 评论 -
python 内置数据结构的基本操作 —— dict(1)
Another useful data type built into Python is the dictionary (see Mapping Types — dict). Dictionaries are sometimes found in other languages as “associative memories” or “associative arrays”. Unlike se转载 2017-05-09 01:22:38 · 697 阅读 · 0 评论 -
python 内置数据结构的基本操作 —— Set(1)
Python also includes a data type for sets. A set is an unordered collection with no duplicate elements. Basic uses include membership testing and eliminating duplicate entries. Set objects also support转载 2017-05-09 01:21:25 · 1123 阅读 · 0 评论 -
python 内置数据结构的基本操作 —— tuple(1)
We saw that lists and strings have many common properties, such as indexing and slicing operations. They are two examples of sequence data types (see Sequence Types — list, tuple, range). Since Python转载 2017-05-09 01:19:52 · 1233 阅读 · 0 评论 -
python 内置数据结构的基本操作 —— list(2)
The list data type has some more methods. Here are all of the methods of list objects:list.append(x) Add an item to the end of the list. Equivalent to a[len(a):] = [x].list.extend(iterable) Extend th转载 2017-05-09 01:14:51 · 592 阅读 · 0 评论 -
python 函数 —— list.sort()
sort(*, key=None, reverse=None) This method sorts the list in place, using only < comparisons between items. Exceptions are not suppressed - if any comparison operations fail, the entire sort operatio原创 2017-05-09 01:04:39 · 1055 阅读 · 0 评论 -
python 函数 —— enumerate()
对于一个可迭代的(iterable)/可遍历的对象(如列表、字符串),enumerate将其组成一个索引序列,利用它可以同时获得索引和值,enumerate多用于在for循环中得到计数enumerate()使用如果对一个列表,既要遍历索引又要遍历元素时,首先可以这样写:In [69]: a=list('thie is a test')In [70]: aOut[70]: ['t', 'h',原创 2017-05-08 20:15:14 · 558 阅读 · 0 评论 -
python 函数 —— divmod()
ivmod(a,b)divmod(a,b)方法返回的是a//b(除法取整)以及a对b的余数,返回结果类型为tuple参数: a,b可以为数字(包括复数)In [49]: divmod(9,2)Out[49]: (4, 1)原创 2017-05-08 19:09:19 · 1075 阅读 · 0 评论 -
Python 函数 —— map(), reduce()
map()函数接收两个参数,一个是函数,一个是Iterable,map将传入的函数依次作用到序列的每个元素,并把结果作为新的Iterator返回。In [9]: a=[1]*10In [10]: aOut[10]: [1, 1, 1, 1, 1, 1, 1, 1, 1, 1]In [11]: def my_double(x): ...: return 2*x ...:In原创 2017-05-07 15:20:16 · 637 阅读 · 0 评论 -
Python 函数 —— ord(), chr()
chr(i)Return the string representing a character whose Unicode code point is the integer i. For example, chr(97) returns the string ‘a’, while chr(8364) returns the string ‘€’. This is the inverse of o原创 2017-05-07 14:56:29 · 559 阅读 · 0 评论 -
python 操作 set 方法 —— set.issubset()
set.issubset(other)set <= other Test whether every element in the set is in other.set < other Test whether the set is a proper subset of other, that is, set <= other and set != other.原创 2017-05-06 01:34:15 · 10084 阅读 · 0 评论 -
python 高级函数 —— filter()
filter()In [130]: a=[i for i in range(10)]In [131]: def aaa(b): ...: return b>0 ...:In [132]: list(filter(aaa,a))Out[132]: [1, 2, 3, 4, 5, 6, 7, 8, 9]原创 2017-05-06 01:24:32 · 1087 阅读 · 0 评论 -
StackoverflowAboutPython(1)—— Python中关键字yield有什么作用?
Python中关键字yield有什么作用?问题:yield有什么用?例如下面这段代码:def node._get_child_candidates(self, distance, min_dist, max_dist): if self._leftchild and distance - max_dist < self._median: yield self._leftchi原创 2017-05-03 15:02:04 · 470 阅读 · 0 评论 -
Python 中的闭包(2)—— 一个栗子
In [120]: def new_counter(): ...: i = 0 ...: def count(): ...: nonlocal i ...: i += 1 ...: return i ...: return count ...:In [121]原创 2017-05-03 12:25:20 · 345 阅读 · 0 评论 -
Python 中的闭包 —— Closure
闭包的概念 在一些语言中,在函数中可以(嵌套)定义另一个函数时,如果内部的函数引用了外部的函数的变量,则可能产生闭包。闭包可以用来在一个函数与一组“私有”变量之间创建关联关系。在给定函数被多次调用的过程中,这些私有变量能够保持其持久性。 —— 维基百科)用比较容易懂的人话说,就是当某个函数被当成对象返回时,夹带了外部变量,就形成了一个闭包。看例子。In [15]: def func(na原创 2017-05-03 09:42:53 · 807 阅读 · 0 评论 -
Python 变量作用域 —— 命名空间与 LEGB 规则
一、命名空间白话一点讲:命名空间是对变量名的分组划分。不同组的相同名称的变量视为两个独立的变量,因此隶属于不同分组(即命名空间)的变量名可以重复。命名空间可以存在多个,使用命名空间,表示在该命名空间中查找当前名称。二、 LEGB 原则 LEGB含义解释: L-Local(function);函数内的名字空间 E-Enclosing function locals;外部嵌套函数的名字原创 2017-05-03 09:12:15 · 983 阅读 · 0 评论 -
Ubuntu16.04 下同时使用 Python2 和 Python3 及对应的 pip 的方法
在终端命令行输入,分别启动 python2 、 python3 用以下命令分别安装pip 、 pip3 分别显示各自的 pip list原创 2017-04-22 18:55:16 · 10278 阅读 · 0 评论 -
不曾见过的Python函数——any(),all()
any(iterable) Return True if any element of the iterable is true. If the iterable is empty, return False. Equivalent to:def any(iterable): for element in iterable: if element:原创 2017-04-15 00:52:01 · 1073 阅读 · 0 评论 -
不曾见过的Python函数——join()
语法: ‘sep’.join(seq)参数说明 sep:分隔符。可以为空 seq:要连接的元素序列、字符串、元组、字典 上面的语法即:以sep作为分隔符,将seq所有的元素合并成一个新的字符串 返回值:返回一个以分隔符sep连接各个元素后生成的字符串In [29]: ''.join(str(i) for i in range(10))Out[29]: '0123456789'In [3原创 2017-04-15 00:02:07 · 562 阅读 · 0 评论 -
使用Python将MongoDB中的数据转存到MySQL中的一次尝试
打算把整个项目上传到 github 上,但是数据啥的都保存在 MongoDB 中,因为没找到把 MongoDB 导出到 sql 脚本的方式,遂决定将数据从 Mongo 中提取出来,再放到 MySQL 中,这样就可以导出 sql 脚本文件,或者是 Excel 文件啦!#coding=utf-8import pymongoimport MySQLdb#------------------------原创 2017-04-08 16:28:31 · 3282 阅读 · 0 评论 -
安装PIL报错Could not find a version that satisfies the requirement PIL (from versions: )的解决办法
使用 pip 安装报错使用 Pycharm 报错找到的一些解决办法:安装PIL的坑pip install PIL dont install into virtualenv以上两种方式均无法解决有效的方案:python 用 python -m pip install –upgrade pip 更新 pip 后 还是不行该怎么办安装 Pillow 就好了…看了一下 Pillow 的官方文档Pillow文原创 2017-04-08 02:27:36 · 44880 阅读 · 3 评论 -
关于Python操作txt文件的一点尝试
读取文件,没有该名称文件的时候会创建该名称的文件with open('/path/to/file', 'r') as f: print(f.read())写文件,写入文件是会覆盖掉原来的内容with open('/Users/michael/test.txt', 'w') as f: f.write('Hello, world!')在文件中追加内容,追加的内容在文件的最后一行原创 2017-04-08 01:51:16 · 774 阅读 · 0 评论 -
关于Python中dic的一点尝试
In [9]: a={}In [10]: b='assa'In [11]: a['{}'.format(b)]=666In [12]: aOut[12]: {'assa': 666}原创 2017-04-07 12:17:11 · 540 阅读 · 0 评论 -
关于Python中None的一点尝试
In [68]: a=NoneIn [69]: aIn [70]: type(a)Out[70]: NoneTypeIn [71]: if a == None: ...: print("a is None") ...:a is NoneIn [72]: if type(a) == 'NoneType': ...: print('type of a is N原创 2017-04-07 01:23:44 · 1148 阅读 · 0 评论 -
不曾见过的Python函数——startswith(),endswith()
In [4]: a='我是'In [5]: aOut[5]: '我是'In [6]: a.endswith('是')Out[6]: TrueIn [7]: a.startswith('我')Out[7]: True原创 2017-04-06 01:32:39 · 1107 阅读 · 0 评论 -
不曾见过的Python函数——map()
map()函数接收两个参数,一个是函数,一个是Iterable,map将传入的函数依次作用到序列的每个元素,并把结果作为新的Iterator返回。In [1]: def f(x): ...: return x*2 ...:In [2]: r = map(f,[i for i in range(10)])In [3]: rOut[3]: <map at 0x18081362eb原创 2017-04-05 18:33:17 · 476 阅读 · 0 评论 -
不曾见过的Python函数——extend()
array.extend(iterable) 追加从可迭代项到array的末尾In [3]: a=[1,2,3,45]In [4]: aOut[4]: [1, 2, 3, 45]In [5]: c=['a','b']In [6]: cOut[6]: ['a', 'b']In [7]: c.extend(a)In [8]: cOut[8]: ['a', 'b', 1, 2, 3, 45]原创 2017-04-05 12:39:54 · 1038 阅读 · 0 评论 -
不曾见过的Python函数——pprint.pprint()
pprint模块提供了打印出任何Python数据结构类和方法。 pprint 包含一个“美观打印机”,用于生成数据结构的一个美观视图。格式化工具会生成数据结构的一些表示,不仅可以由解释器正确地解析,而且便于人类阅读。输出尽可能放在一行上,分解为多行时则需要缩进。>>>import pprint>>>data = ( "this is a string", [1, 2, 3, 4], ("m原创 2017-03-30 00:55:55 · 2351 阅读 · 0 评论 -
Python操作文件的基本方法
读取文本文件path为要读取的文本的路径with open(path,'r') as f: print(f.read())调用read()会一次性读取文件的全部内容,如果文件有10G,内存就爆了,所以,要保险起见,可以反复调用read(size)方法,每次最多读取size个字节的内容。另外,调用readline()可以每次读取一行内容,调用readlines()一次读取所有内容并按行返回li原创 2017-03-29 23:56:47 · 837 阅读 · 0 评论 -
关于Python中以字母r/R,或字母u/U 开头的字符串
1、以r或R开头的python中的字符串表示(非转义的)原始字符串python里面的字符,如果开头处有个r,比如:(r’^time/plus/\d{1,2}/$’, hours_ahead)说明字符串r”XXX”中的XXX是普通字符。有普通字符相比,其他相对特殊的字符,其中可能包含转义字符,即那些,反斜杠加上对应字母,表示对应的特殊含义的,比如最常见的”\n”表示换行,”\t”表示Tab等。而如果是转载 2017-03-27 11:04:34 · 834 阅读 · 0 评论 -
Python异常处理的学习(1)
参考虫师的:python异常处理(基础)原创 2017-03-24 19:57:00 · 458 阅读 · 0 评论 -
使用python向MySQL数据库中插入数据时报错UnicodeEncodeError的解决办法
版本说明:Python3.5MySQL5.7mysqlclient应该是最新的>>> ================== RESTART: C:\Users\rHotD\Desktop\test.py ==================Traceback (most recent call last): File "C:\Users\rHotD\Desktop\test.py",原创 2017-03-24 19:54:17 · 2539 阅读 · 0 评论 -
通过mysqlclient操作MySQL数据库
我使用的是 Python3.5,所以选择 mysqlclient 来操作 MySQL安装mysqlclient要想使 python 可以操作 mysql 就需要 MySQLdb 驱动,它是 python 操作 mysql 必不可少的模块。使用pip安装pip install mysqlclient测试测试非常简单,检查 MySQLdb 模块是否可以正常导入。>>> import MySQLdb没有报转载 2017-03-24 17:23:35 · 20226 阅读 · 1 评论 -
使用Pyinstaller将python脚本打包成exe文件
Pyinstaller giuhub地址Pyinstaller官方文档只保留该文件即可,其余文件皆可删除原创 2017-03-24 16:58:17 · 1065 阅读 · 0 评论 -
不曾见过的Python函数——zip()
zip()是Python的一个内建函数,它接受一系列可迭代的对象作为参数,将对象中对应的元素打包成一个个tuple(元组)。若传入参数的长度不等,则返回list的长度和参数中长度最短的对象相同。利用*号操作符,可以将list unzip(解压)>>> x=[1,2,3]>>> y=[4,5,6]>>> zipped=zip(x,y)>>> zipped<zip object at 0x0000原创 2017-03-22 17:04:59 · 654 阅读 · 0 评论 -
使用pip管理Python的packages
您可以使用pip程序安装,升级和删除软件包 。pip search 可以使用有限的搜索功能 >>pip search pymysql >> pip install 可以通过指定package的名称来安装其最新版本 >>pip install scrapy >> 您还可以通过提供packageName==Version来安装特定版本的包: >>pip install django=原创 2017-03-21 19:14:33 · 1073 阅读 · 1 评论 -
关于python类和实例的一些尝试
Python 3.6.0 (v3.6.0:41df79263a11, Dec 23 2016, 08:06:12) [MSC v.1900 64 bit (AMD64)] on win32Type "copyright", "credits" or "license()" for more information.>>> a=5>>> b=5>>> deTraceback (most re原创 2017-03-11 13:34:20 · 1143 阅读 · 0 评论