#include<iostream>
using namespace std;
#define MAX 100
#define OK 1
typedef int KeyType;
typedef struct {
KeyType key;
}RedType;
typedef struct {
RedType r[MAX + 1];
int length;
}SqList;
int InitList_Sq(SqList& L);
int Partition(SqList& L, int low, int high);
void QSort(SqList& L, int low, int high);
void print(SqList L);
int main()
{
SqList L;
InitList_Sq(L);
QSort(L, 1, L.length);
print(L);
return 0;
}
int InitList_Sq(SqList& L)
{
int n, k = 1;
L.length = 0;
while (1)
{
cin >> n;
if (n == -1)
break;
L.r[k++].key = n;
L.length++;
}
return OK;
}
int Partition(SqList& L, int low, int high)
{
L.r[0] = L.r[low];
int pivotkey = L.r[0].key;
while (low < high)
{
while (low < high && L.r[high].key >= pivotkey)
--high;
L.r[low] = L.r[high];
while (low < high && L.r[low].key < pivotkey)
++low;
L.r[high] = L.r[low];
}
L.r[low] = L.r[0];
return low;
}
void QSort(SqList& L, int low, int high)
{
if (low < high)
{
int pivotloc = Partition(L, low, high);
QSort(L, low, pivotloc - 1);
QSort(L, pivotloc + 1, high);
}
}
void print(SqList L)
{
for (int i = 1; i <= L.length; i++)
cout << L.r[i].key << ' ';
cout << endl;
}
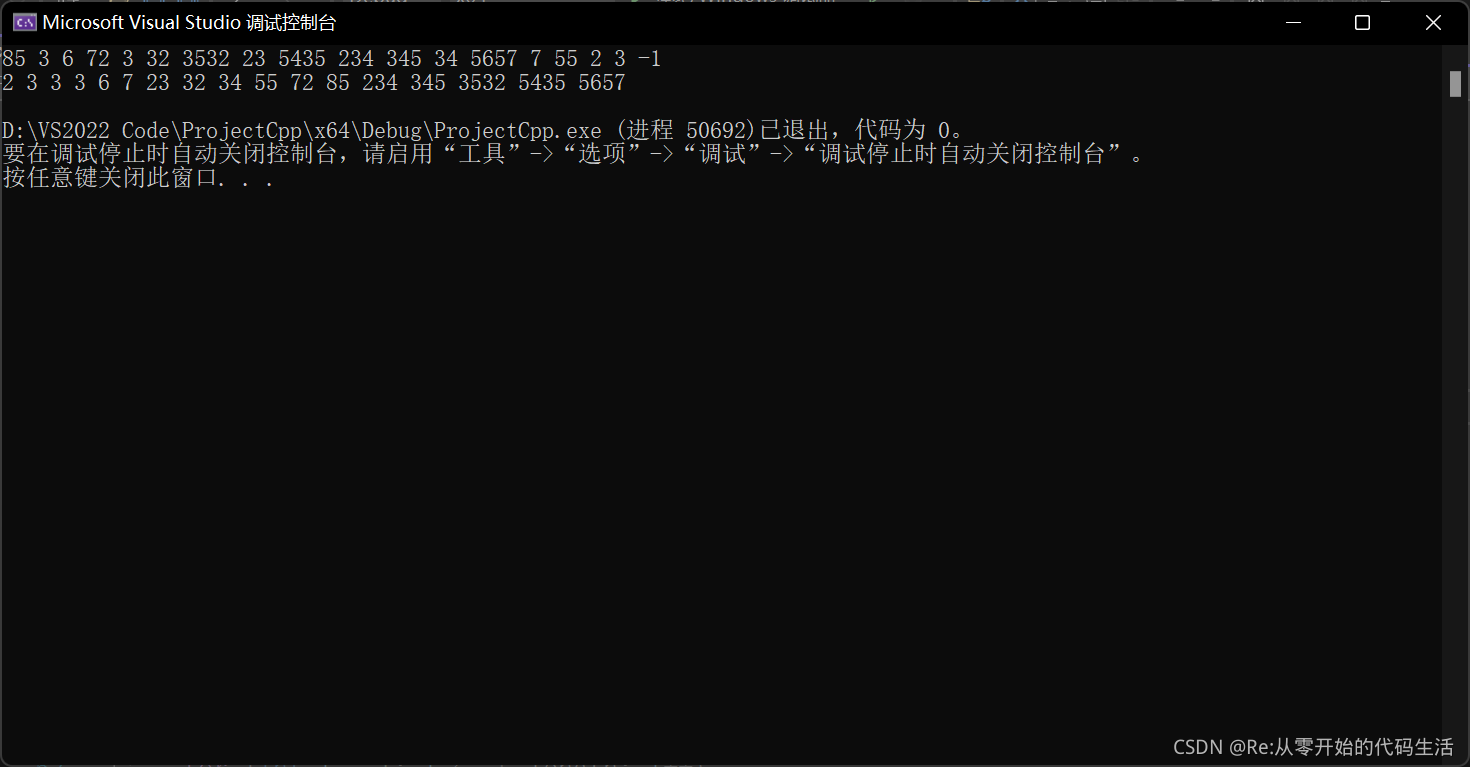