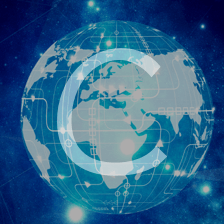
数据结构
yuccess
这个作者很懒,什么都没留下…
展开
-
124. Binary Tree Maximum Path Sum
题目:Given a binary tree, find the maximum path sum.For this problem, a path is defined as any sequence of nodes from some starting node to any node in the tree along the parent-child connecti原创 2016-12-11 21:39:34 · 270 阅读 · 0 评论 -
133. Clone Graph :一个典型的DFS
题目:Clone an undirected graph. Each node in the graph contains a label and a list of its neighbors.代码:/** * Definition for undirected graph. * struct UndirectedGraphNode { * int label;原创 2016-12-13 23:23:59 · 337 阅读 · 0 评论 -
105. Construct Binary Tree from Preorder and Inorder Traversal,前序+中序 构建 树
观察: A / \ B C / \ D Epreorder: A B D E Cinorder: D B E A C 规律:对于inorder序列,最左边的一定是整个树的leftmost。这样,从左☞右遍历pre,直到pre[i] == in[0], 便找到了le原创 2016-12-07 21:00:02 · 415 阅读 · 0 评论 -
110. Balanced Binary Tree 判定是否平衡
题目:Given a binary tree, determine if it is height-balanced.For this problem, a height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node neve原创 2016-12-09 15:19:57 · 497 阅读 · 0 评论 -
113. Path Sum II 这里要注意 引用 和 传值的区别,见注释
题目:Given a binary tree and a sum, find all root-to-leaf paths where each path's sum equals the given sum.For example:Given the below binary tree and sum = 22, 5原创 2016-12-09 16:47:18 · 286 阅读 · 0 评论 -
114. Flatten Binary Tree to Linked List, pre节点的妙用
题目:Given a binary tree, flatten it to a linked list in-place.For example,Given 1 / \ 2 5 / \ \ 3 4 6The flattened tree should look like:原创 2016-12-09 20:13:57 · 190 阅读 · 0 评论 -
116. Populating Next Right Pointers in Each Node
题目:Given a binary tree struct TreeLinkNode { TreeLinkNode *left; TreeLinkNode *right; TreeLinkNode *next; }Populate each next pointer to point to its next rig原创 2016-12-09 21:17:04 · 188 阅读 · 0 评论 -
117. Populating Next Right Pointers in Each Node II 非常精炼的代码,值得好好体会
题目:Follow up for problem "Populating Next Right Pointers in Each Node".What if the given tree could be any binary tree? Would your previous solution still work?Note:You may only use原创 2016-12-09 22:41:27 · 210 阅读 · 0 评论 -
20. Valid Parentheses
Given a string containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.The brackets must close in the correct order, "()" and "()[]{}" are all va原创 2017-01-05 09:15:48 · 208 阅读 · 0 评论 -
21. Merge Two Sorted Lists 一道基础题的两种精炼解法
方法一:递归/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; */class Solution {public:原创 2017-01-05 09:53:11 · 991 阅读 · 0 评论 -
25. Reverse Nodes in k-Group 怎样不使用额外空间翻转一个单向链表?
Given a linked list, reverse the nodes of a linked list k at a time and return its modified list.If the number of nodes is not a multiple of k then left-out nodes in the end should remain as it is原创 2017-01-05 12:20:19 · 461 阅读 · 0 评论 -
56. Merge Intervals 好代码
Given a collection of intervals, merge all overlapping intervals.For example,Given [1,3],[2,6],[8,10],[15,18],return [1,6],[8,10],[15,18]./** * Definition for an interval. * struct I原创 2017-01-07 02:00:28 · 317 阅读 · 0 评论 -
红黑树
平衡查找树在一颗含有N个结点的树中,我们希望树高为~lgN,这样我们就能保证所有查找都能在~lgN此比较内结束,就和二分查找一样。不幸的是,在动态插入中保证树的完美平衡的代价太高了。我们放松对完美平衡的要求,使符号表API中所有操作均能够在对数时间内完成。 2-3查找树为了保证查找树的平衡性,我们需要一些灵活性,因此在这里我们允许树中的一个结点保原创 2016-11-24 22:04:37 · 502 阅读 · 0 评论 -
map,hash_map, unordered_map
1.结论运行效率方面:unordered_map最高,hash_map其次,而map效率最低单提供了有序的序列。占用内存方面:hash_map内存占用最低,unordered_map其次(数量少时优于hash_map),而map占用最高。需要无序容器时候用unordered_map,有序容器时候用map。2.原理map的内部实现是二叉平衡原创 2016-11-24 21:37:12 · 217 阅读 · 0 评论 -
144. Binary Tree Preorder Traversal 二叉树的前序遍历
/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */clas原创 2016-12-11 22:38:09 · 279 阅读 · 0 评论 -
145. Binary Tree Postorder Traversal 树后续遍历 flag方法和reverse方法
/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */clas原创 2016-12-11 23:37:03 · 349 阅读 · 0 评论 -
94. Binary Tree Inorder Traversal
题目:Given a binary tree, return the inorder traversal of its nodes' values.For example:Given binary tree [1,null,2,3], 1 \ 2 / 3return [1,3,2].解法:Hi,原创 2016-12-04 21:14:21 · 198 阅读 · 0 评论 -
138. Copy List with Random Pointer
题目复制带有随机指针的链表:一个单链表除了next指针外还有一个random指针随机指向任何一个元素(可能为空) 《剑指offer》上的面试题26分析方法一: map,先按普通方法复制链表,再两个链表同时走复制random(旧节点a,新节点a’)a'->random=map[a->random](空指针单独处理) 方法二: 插入:每个节点后面插入一个自身的“复本转载 2016-12-04 23:14:02 · 237 阅读 · 0 评论 -
98. Validate Binary Search Tree
Given a binary tree, determine if it is a valid binary search tree (BST).class Solution {public: bool isValidBST(TreeNode* root) { TreeNode* prev = NULL; return validate(r原创 2016-12-05 15:24:25 · 179 阅读 · 0 评论 -
彻底理解线索二叉树
一、线索二叉树的原理 通过考察各种二叉链表,不管儿叉树的形态如何,空链域的个数总是多过非空链域的个数。准确的说,n各结点的二叉链表共有2n个链域,非空链域为n-1个,但其中的空链域却有n+1个。如下图所示。 因此,提出了一种方法,利用原来的空链域存放指针,指向树中其他结点。这种指针称为线索。 记ptr指向二叉链表中的一个结点,以下是建立线索的规转载 2016-12-05 17:18:42 · 675 阅读 · 0 评论 -
99. Recover Binary Search Tree
题目:基础题,代码里给出了三种解答方法,其中Morris法是O(1)space。Two elements of a binary search tree (BST) are swapped by mistake.Recover the tree without changing its structure.Note:A solution using O(n) spa原创 2016-12-05 21:28:58 · 597 阅读 · 0 评论 -
101. Symmetric Tree
题目:Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).For example, this binary tree [1,2,2,3,4,4,3] is symmetric: 1 / \ 2 2 / \ / \3原创 2016-12-06 17:48:34 · 196 阅读 · 0 评论 -
102. Binary Tree Level Order Traversal 树的层序遍历 递归 和 队列方法
题目:Given a binary tree, return the level order traversal of its nodes' values. (ie, from left to right, level by level).For example:Given binary tree [3,9,20,null,null,15,7], 3 /原创 2016-12-06 18:58:01 · 265 阅读 · 0 评论 -
103. Binary Tree Zigzag Level Order Traversal
vectorvectorint> > zigzagLevelOrder(TreeNode* root) { if (root == NULL) { return vectorvectorint> > (); } vectorvectorint> > result; queue nodesQueue; nodesQueue.push(root原创 2016-12-06 22:44:52 · 195 阅读 · 0 评论 -
104. Maximum Depth of Binary Tree 求树的最大深度
1,my solution/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {}原创 2016-12-06 23:03:58 · 287 阅读 · 0 评论 -
57. Insert Interval , STL的使用
Given a set of non-overlapping intervals, insert a new interval into the intervals (merge if necessary).You may assume that the intervals were initially sorted according to their start times.E原创 2017-01-07 03:45:34 · 326 阅读 · 0 评论