题目描述
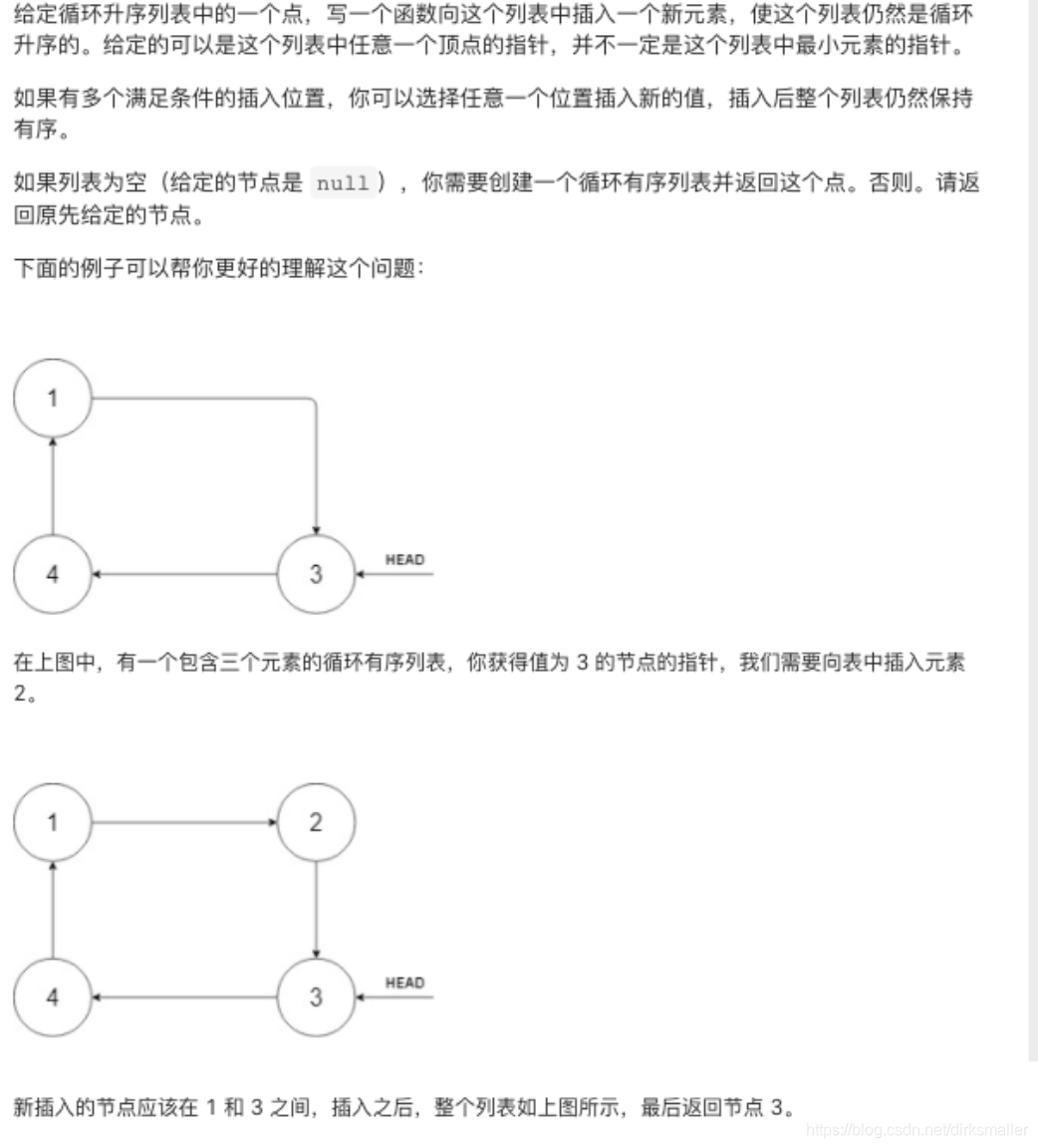
解题思路
- 由于是单向循环列表;能够保证每个元素都能遍历到
- 要插入的元素,其插入的位置有两种情况
a. 插入的位置 ,其前驱的值不大于 要插入的值 ,并且其后继的值要不小于当前值
b.插入的位置, 不小于最大值或者不大于最小值
#include "linked.hpp"
void insert(Node * circle , int value){
if(circle == nullptr){
return;
}
Node* insert = new Node();
insert->value = value;
if(circle->next == nullptr){
circle->next = insert;
insert->next = circle;
return;
}
Node* cur = circle;
Node* next = circle->next;
while (true) {
if(cur->value>next->next->value){
if(cur->value<= insert->value ||next->value < insert->value){
cur->next = insert;
insert->next = next;
next = insert;
break;
}
}else{
if(cur->value <= insert->value && insert->value <= next->value){
cur->next = insert;
insert->next = next;
next = insert;
break;
}
}
cur = cur->next;
next = next->next;
}
}
测试代码
int main(int argc, const char * argv[]) {
Node * node = new Node();
node->value = 10;
insert(node, 1);
insert(node, 2);
insert(node,11);
return 0;
}