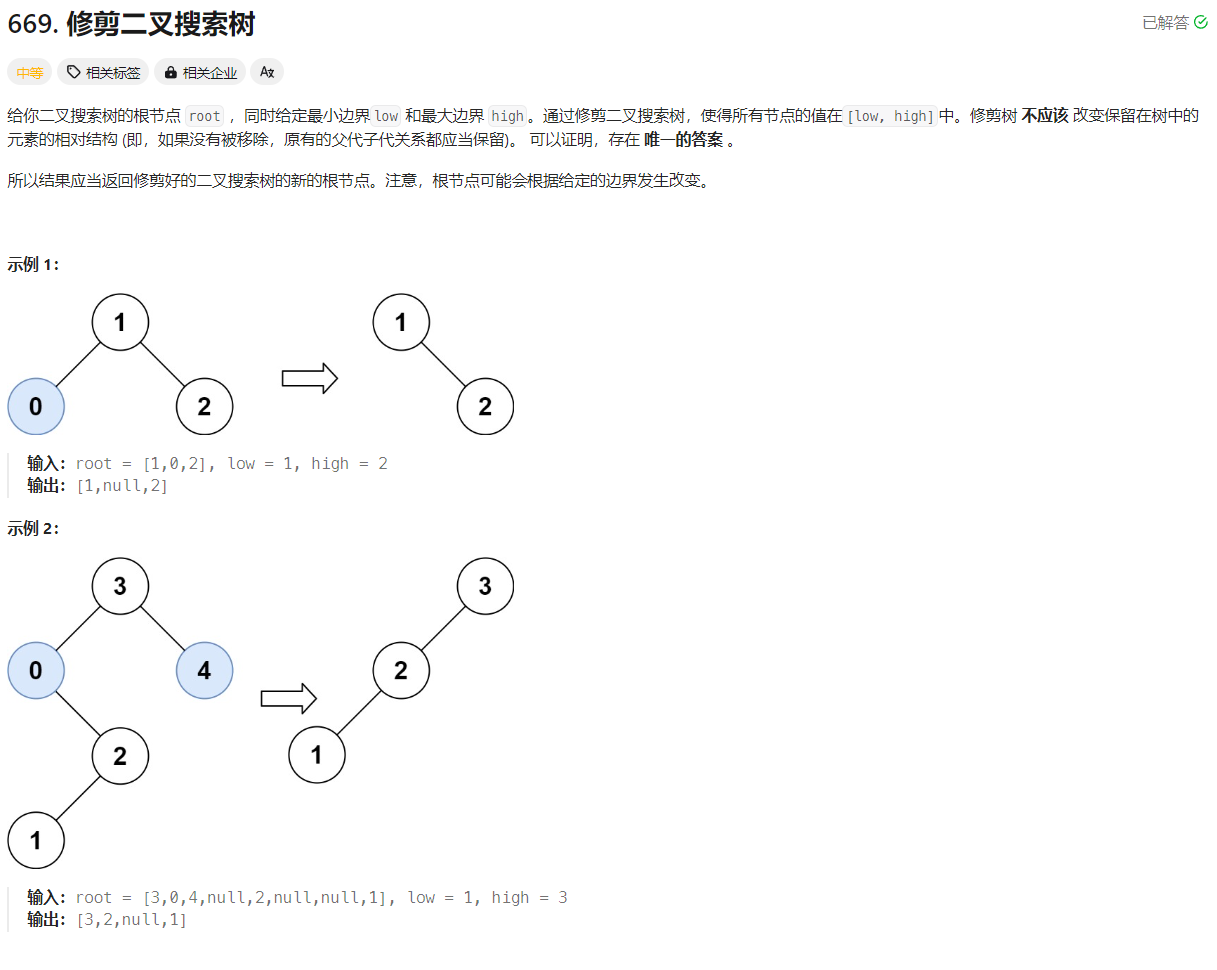
解题思路
- 此题需要采用后序遍历解决,因为可能删除多个节点,如果先序遍历,可能头节点符合删除要求,就直接停止遍历了。
- 此树为二叉搜索树,搜索树节点的左子树均小于该节点,右子树均大于该节点。因此roo.val < key 遍历右子树, 反之左子树。
- 删除节点需要以下三种情况
- 该节点为叶子节点,返回null即可
- 该节点的左子节点不为空,右子节点为空,返回其左子节点。反之返回右子节点。
- 该节点左右节点均不为空,则将该节点的左子树作为该节点其右子树最左节点的左子树,返回该节点右子节点。
- 删除该节点的做法就是直接将该节点的父母节点指向该节点的左/右节点。
具体代码
if(root == null) return root;
root.left = trimBST(root.left, low, high);
root.right = trimBST(root.right, low, high);
if(root.val > high || root.val < low){
if(root.left == null && root.right == null) return null;
else if(root.left != null && root.right == null) return root.left;
else if(root.left == null && root.right != null) return root.right;
else{
TreeNode tmp = root.right;
while(tmp.left != null) tmp = tmp.left;
tmp.left = root.left;
return root.right;
}
}
return root;