- function:用cv2 读取、显示、保存 图像
- c2.read->按照bgr读取->show->可以正常显示bgr->write->把bgr转成rgb才会写入
- plot 显示的是rgb
- https://www.qianbo.com.cn/Tool/Rgba/ rgb对应颜色查询
import cv2
import matplotlib.pyplot as plt
import numpy as np
def show_img_cv2(rgb,window_name = 'bgr'):
cv2.namedWindow(window_name, cv2.WINDOW_AUTOSIZE)
cv2.imshow(window_name,rgb),cv2.waitKey()
def get_img_cv2(img_path):
bgr = cv2.imread(img_path)
if bgr is None:
print('cv2 get ', img_path.split('\\')[-1], ' failed! ',end='')
raise Exception('maybe there are something wrong with path!')
return bgr
def save_img_cv2(path,bgr):
try:
succ = cv2.imwrite(path, bgr)
if succ == False:
print('check the path!',end=' ');
raise NameError()
except Exception:
print('save img failed!')
def plt_img(img):
plt.figure()
plt.imshow(img)
plt.show()
path = r'C:\Users\13361\Pictures\Camera Roll\mac.jpg'
bgr = get_img_cv2(path)
1. 用cv2读取出来的数组是bgr格式的,使用cv2.imshow显示
show_img_cv2(bgr)
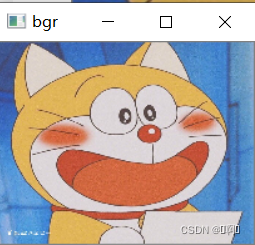
2. pyplot是用rgb格式显示的,直接显示在cv2得到的图像会出错
plt_img(bgr)
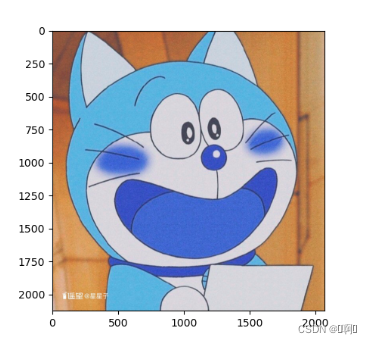
3. 把bgr格式转化为rgb
rgb = cv2.cvtColor(bgr,cv2.COLOR_BGR2RGB)
show_img_cv2(rgb,'rgb')
plt_img(rgb)
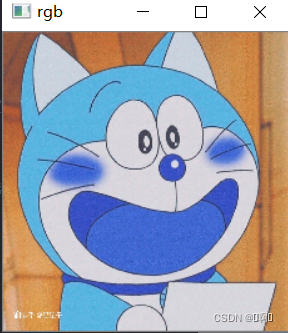
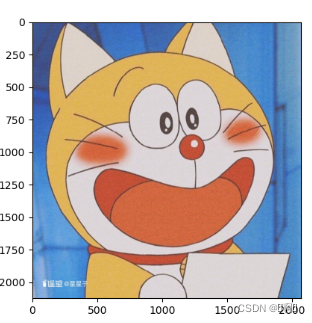
4. cv2.imwrite(img), img必须是bgr格式,否则图片会出错
save_img_cv2(r'..\data\rgb.png',rgb)
save_img_cv2(r'..\data\bgr.png',bgr)
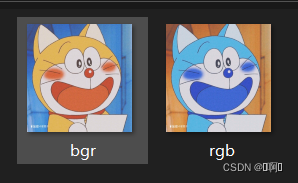
5. PIL包处理图像,Image.open(path)读取出来的是一个图像对象,而不是数组
def get_img_PIL(path):
from PIL import Image
try:
img_obj = Image.open(path)
return img_obj
except Exception:
print('PIL op failed!')
img_obj = get_img_PIL(path)
img_obj.show()
img = np.asarray(img_obj)
img.shape
(2124, 2070, 3)
img_obj = Image.fromarray(np.uint8(img))
<PIL.Image.Image image mode=RGB size=2070x2124 at 0x21A1521F7B8>
img_obj.save(path)
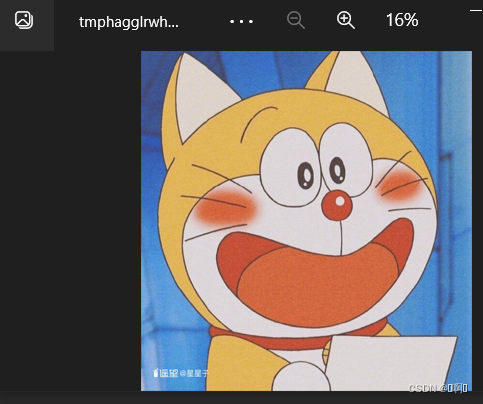
6. subplot
def subpolt(img_dict,row = 1,column = 3):
plt.figure()
for k,(name,img) in enumerate(img_dict.items()):
subplot = plt.subplot(row, column, k+1)
plt.imshow(img)
subplot.set_title(name)
plt.xticks([])
plt.yticks([])
plt.show()
img_dict = {'bgr(read from cv2)':bgr,'rgb':rgb, 'Image from PIL':img_obj,}
subpolt(img_dict,row = 1,column = 3)
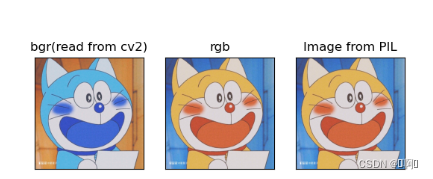