c语言之链表基础操作
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int count = 0;
struct node
{
int data;
struct node *pnext;
};
struct node *create_node(int dat)
{
struct node *p = (struct node *)malloc(sizeof(struct node *));
if(NULL == p)
{
printf("malloc error\n");
}
memset(p,'\0',sizeof(struct node));
p->data = dat;
p->pnext = NULL;
return p;
}
void Insert_Tail(struct node *ph,struct node *new)
{
struct node *p = ph;
while(NULL != p->pnext)
{
p = p->pnext;
count++;
}
p->pnext = new;
ph->data = count+1;
}
void Insert_Head(struct node *ph,struct node *new)
{
new->pnext = ph->pnext;
ph->pnext = new;
ph->data = ++count;
}
int Delete_node(struct node *ph,int data)
{
struct node *temp = ph;
struct node *tempre = NULL;
while(NULL != temp->pnext)
{
tempre = temp;
temp = temp ->pnext;
if(temp->data == data)
{
if(NULL == temp->pnext)
{
tempre->pnext = NULL;
free(temp);
}
else
{
tempre->pnext = temp->pnext;
}
ph->data = count - 1;
printf("节点 %d 删除成功\n",data);
return 0;
}
}
}
struct node * Search_Node(struct node *ph,int dat)
{
struct node *temp = ph;
while(NULL != temp->pnext)
{
temp = temp->pnext;
if(temp->data == dat)
{
printf("node value:%d find success\n",temp->data);
return temp;
}
}
printf("not find node \n");
return NULL;
}
void mod_node(struct node *ph,int data,int new_data)
{
struct node *temp = ph;
struct node *p = NULL;
p = Search_Node(temp,data);
if(NULL != p)
{
p->data = new_data;
printf("节点修改成功\n");
}
else
{
printf("节点修改失败\n");
}
}
void Print_Node(struct node *ph)
{
int i = 0;
struct node *temp = ph;
printf("--------------遍历节点--------------\n");
printf("node num %d\n",ph->data);
while(NULL != temp->pnext)
{
i++;
printf("node %d = %d\n",i,temp->pnext->data);
temp = temp->pnext;
}
printf("--------------遍历完成---------------\n");
}
int main(void)
{
struct node *phead = create_node(0);
Insert_Head(phead,create_node(1));
Insert_Head(phead,create_node(2));
Insert_Head(phead,create_node(3));
Insert_Head(phead,create_node(4));
Insert_Head(phead,create_node(5));
Insert_Head(phead,create_node(6));
Print_Node(phead);
Delete_node(phead,3);
Print_Node(phead);
mod_node(phead,5,10);
Print_Node(phead);
Search_Node(phead,6);
return 0;
}
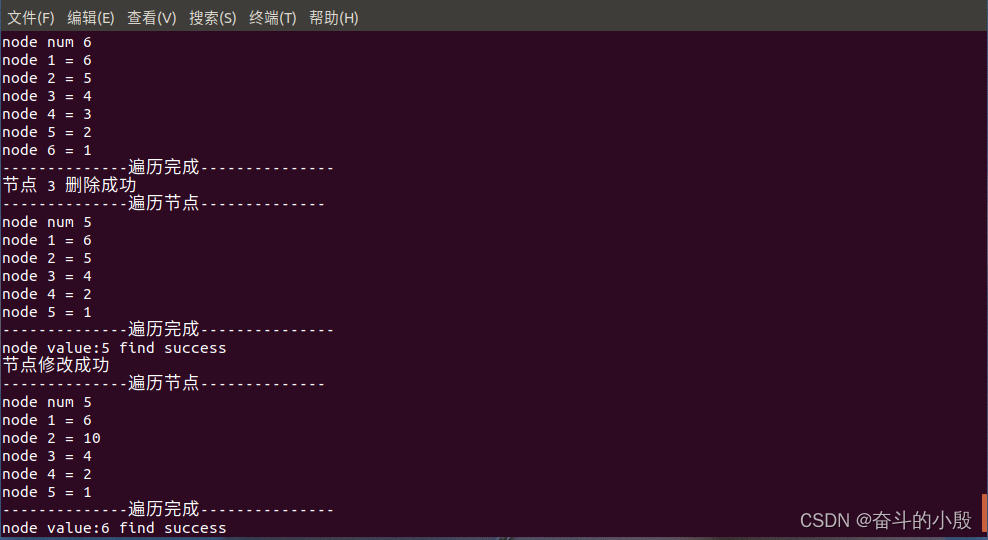