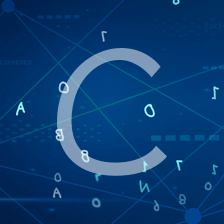
leetcode
文章平均质量分 68
673556617-real
leetcode java angular golang 分布式
展开
-
Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head.For example,Given 1->2->3->4, you should return the list as 2->1->4->3.Your algorithm should use only constant space. Y原创 2015-07-28 20:53:46 · 396 阅读 · 0 评论 -
Remove Duplicates from Sorted Array
Given a sorted array, remove the duplicates in place such that each element appear only once and return the new length.Do not allocate extra space for another array, you must do this in place with原创 2015-07-28 21:41:05 · 437 阅读 · 0 评论 -
Multiply Strings
参考了网上的一种解题思路,原网址找不到了。。 思路的话仔细看看会明白的,当时举了一个例子按照这个流程跑了下。这个思路还是很清晰的。代码:package codes;public class MultiplyStrings { public static void main(String[] args) { String str1 = "9"; String str2 =原创 2015-08-25 12:23:57 · 421 阅读 · 0 评论 -
Rotate Image
You are given an n x n 2D matrix representing an image.Rotate the image by 90 degrees (clockwise).Follow up:Could you do this in-place?用个临时的数组,存下转换的位置再复制回去就行。代码:package codes;p原创 2015-08-26 04:40:31 · 396 阅读 · 0 评论 -
Palindrome Number
Determine whether an integer is a palindrome. Do this without extra space.实现代码:public boolean isPalindrome02(int x) { String num= Integer.toString(x); if(num.length()>11 || num.charAt(0)=='原创 2015-07-19 11:19:03 · 386 阅读 · 0 评论 -
Container With Most Water
Given n non-negative integers a1, a2, ..., an, where each represents a point at coordinate (i, ai). n vertical lines are drawn such that the two endpoints of line i is at (i, ai) and (i, 0). Fin原创 2015-07-19 12:28:32 · 437 阅读 · 0 评论 -
String to Integer (atoi)
Implement atoi to convert a string to an integer.Hint: Carefully consider all possible input cases. If you want a challenge, please do not see below and ask yourself what are the possible input ca原创 2015-07-19 00:32:04 · 471 阅读 · 0 评论 -
Longest Palindromic Substring
提述:Given a string S, find the longest palindromic substring in S. You may assume that the maximum length of S is 1000, and there exists one unique longest palindromic substring.写了一个算法,但是原创 2015-07-15 13:57:05 · 434 阅读 · 0 评论 -
Reverse Integer
Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321这道题其实不难,重要的是对一些特殊值进行判断。如果输入数字为超过int范围的值该如何处理。例如:1534236469,反过来会超范围,但是在计算的过程中不会提示你已经越界了。我用的是一个Long原创 2015-07-18 22:45:15 · 367 阅读 · 0 评论 -
ZigZag Conversion
The string "PAYPALISHIRING" is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)P A H NA P L S原创 2015-07-18 10:30:42 · 492 阅读 · 0 评论 -
Longest Substring Without Repeating Characters
实现思路参考了网上的内容。一开始的想法是通过一个嵌套循环计算每一位的最长子串,时间复杂度是O(n3)。在嵌套的第二个循环内,使用 map.containKey来判断当前是否存在值,存在的话跳出循环,不存在的话存入key。这个思路最大的问题是复杂度比较高。网上提供了 一种线性时间的解决方法,核心思想是当遇到重复的字符时,起点更新为重复字符的上一个位置。需要借助一个数组来保存上一个位置的信息。原创 2015-07-14 12:59:50 · 514 阅读 · 0 评论 -
Median of Two Sorted Arrays
leetecode 上的一道题:There are two sorted arrays nums1 and nums2 of size m and n respectively. Find the median of the two sorted arrays. The overall run time complexity should be O(log (m+n)).实现代码:原创 2015-07-13 23:09:47 · 539 阅读 · 0 评论 -
leetcode难度及面试频率
转自:http://blog.youkuaiyun.com/yutianzuijin/article/details/11477603 1Two Sum25arraysort转载 2015-07-19 21:49:18 · 530 阅读 · 0 评论 -
Permutations
Given a collection of numbers, return all possible permutations.For example,[1,2,3] have the following permutations:[1,2,3], [1,3,2], [2,1,3], [2,3,1], [3,1,2], and [3,2,1].实现的思路是使用递原创 2015-08-27 00:24:42 · 426 阅读 · 0 评论 -
Longest Common Prefix
Write a function to find the longest common prefix string amongst an array of strings.总之不难,但是脑袋要清醒..代码:public class Solution { public String longestCommonPrefix(String[] strs) {原创 2015-07-21 14:31:48 · 501 阅读 · 0 评论 -
Two Sum
Given an array of integers, find two numbers such that they add up to a specific target number.The function twoSum should return indices of the two numbers such that they add up to the target, whe原创 2015-07-20 17:08:37 · 409 阅读 · 0 评论 -
Integer to Roman
Given an integer, convert it to a roman numeral.Input is guaranteed to be within the range from 1 to 3999.这道题直接上来硬写的,感觉有点没动脑筋。想起当时用java课上一道关于扑克牌的课后作业,应该写的灵活些。像本题,如果还需要写出后续的数字这种写法就不妥当了。我想原创 2015-07-21 00:45:12 · 429 阅读 · 0 评论 -
4Sum
实现的时候参考了http://blog.sina.com.cn/s/blog_71d59f9a01018dh3.html 的思路。Given an array S of n integers, are there elements a, b, c, and d in S such that a + b + c + d = target? Find all unique quadru原创 2015-07-20 15:52:08 · 437 阅读 · 0 评论 -
Roman to Integer
Given a roman numeral, convert it to an integer.Input is guaranteed to be within the range from 1 to 3999.搞了挺久一直没搞出来。看了网上的参考后知道是推导的公式写错了。如果是从高位往地位扫(也就是一次循环用s.charAt(i)由高往低),那么相邻元素之原创 2015-07-21 12:07:28 · 460 阅读 · 0 评论 -
Pow(x, n)
参考:http://blog.youkuaiyun.com/linhuanmars/article/details/20092829参考了这上面的二分解法。自己写了一个最简单的发现会超时报错。关于递归方面一直不是很好,这学期要加强下算法这方面的知识。 关于代码中的几种情况用三个例子进行说明。代码:package codes;public class Pow {原创 2015-08-28 02:31:25 · 427 阅读 · 0 评论 -
Jump Game
原网址:http://blog.youkuaiyun.com/linhuanmars/article/details/21354751发现递归跟动规的知识都还给老师了。。最近要大补一下。Given an array of non-negative integers, you are initially positioned at the first index of the a原创 2015-08-28 06:17:34 · 318 阅读 · 0 评论 -
Remove Nth Node From End of List
Given a linked list, remove the nth node from the end of list and return its head.For example, Given linked list: 1->2->3->4->5, and n = 2. After removing the second node from the end, the原创 2015-07-21 19:43:34 · 554 阅读 · 0 评论 -
Letter Combinations of a Phone Number
Given a digit string, return all possible letter combinations that the number could represent.A mapping of digit to letters (just like on the telephone buttons) is given below.Input:Digit st原创 2015-07-21 22:49:21 · 551 阅读 · 0 评论 -
Merge Two Sorted Lists
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists.改了好久,可是明明不难的啊。代码:package leetcode;import java.uti原创 2015-07-22 13:40:53 · 384 阅读 · 0 评论 -
3Sum
Given an array S of n integers, are there elements a, b, c in S such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.Note:Elements in a triplet (a,b,c原创 2015-07-22 18:40:39 · 423 阅读 · 0 评论 -
Valid Parentheses
Given a string containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.The brackets must close in the correct order, "()" and "()[]{}" are all va原创 2015-07-22 17:51:19 · 506 阅读 · 0 评论 -
Search for a Range
Given a sorted array of integers, find the starting and ending position of a given target value.Your algorithm's runtime complexity must be in the order of O(log n).If the target is not found原创 2015-08-04 15:20:56 · 490 阅读 · 0 评论 -
Search Insert Position
Given a sorted array and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.You may assume no duplicates in the array.原创 2015-08-04 15:32:58 · 447 阅读 · 0 评论 -
Rectangle Area
Find the total area covered by two rectilinear rectangles in a 2D plane.Each rectangle is defined by its bottom left corner and top right corner as shown in the figure.Assume that the tota原创 2015-07-24 14:18:30 · 511 阅读 · 0 评论 -
Generate Parentheses
Given n pairs of parentheses, write a function to generate all combinations of well-formed parentheses.For example, given n = 3, a solution set is:"((()))", "(()())", "(())()", "()(())", "()()原创 2015-07-24 14:54:19 · 470 阅读 · 0 评论 -
MongoDB启动方法
最近在做个人博客,打算尝试下mongodb做数据库。记录下如何开启数据库的。1. 首先开启一个控制台,进入mongodb的安装目录的bin文件夹下,如图2.命令mongod --dbpath=数据库路径 路径自己选定就可以3.开启后会提示等待请求接入。可以用localhost:27017来查看状态这样就连上了。因为之前用的是My原创 2015-07-24 19:07:59 · 552 阅读 · 0 评论 -
Valid Sudoku
Determine if a Sudoku is valid, according to: Sudoku Puzzles - The Rules.The Sudoku board could be partially filled, where empty cells are filled with the character '.'.A partially fille原创 2015-08-22 14:41:09 · 440 阅读 · 0 评论 -
Count and Say
参考:http://blog.youkuaiyun.com/linhuanmars/article/details/20679963The count-and-say sequence is the sequence of integers beginning as follows:1, 11, 21, 1211, 111221, ...1 is read off as "one原创 2015-08-23 12:50:05 · 494 阅读 · 0 评论 -
Wiggle Sort
leetcode 上的一道题[LeetCode] Wiggle Sort Wiggle SortGiven an unsorted array nums, reorder it in-place such that nums[0] = nums[2] .For example, given nums = [3, 5, 2, 1, 6, 4], one pos原创 2016-05-22 17:00:33 · 412 阅读 · 0 评论 -
Permutations
Given a collection of distinct numbers, return all possible permutations.For example,[1,2,3] have the following permutations:[ [1,2,3], [1,3,2], [2,1,3], [2,3,1], [3,1,2], [3,2,1原创 2016-09-06 06:04:16 · 510 阅读 · 0 评论 -
Sort Colors
Given an array with n objects colored red, white or blue, sort them so that objects of the same color are adjacent, with the colors in the order red, white and blue.Here, we will use the integers原创 2016-11-06 11:09:00 · 306 阅读 · 0 评论 -
Combinations
Given two integers n and k, return all possible combinations of k numbers out of 1 ... n.For example,If n = 4 and k = 2, a solution is:[ [2,4], [3,4], [2,3], [1,2], [1,3], [1,4],]原创 2016-11-08 08:34:45 · 265 阅读 · 0 评论 -
Number of Boomerangs
Given n points in the plane that are all pairwise distinct, a "boomerang" is a tuple of points (i, j, k) such that the distance between i and j equals the distance between i and k (the order of原创 2016-11-08 13:51:53 · 1520 阅读 · 0 评论 -
Decode Ways
A message containing letters from A-Z is being encoded to numbers using the following mapping:‘A’ -> 1 ‘B’ -> 2 … ‘Z’ -> 26 Given an encoded message containing digits, determine the total number of原创 2016-10-28 02:23:45 · 252 阅读 · 0 评论 -
Arranging Coins
You have a total of n coins that you want to form in a staircase shape, where every k-th row must have exactly k coins.Given n, find the total number of full staircase rows that can be formed.原创 2016-11-09 00:00:22 · 324 阅读 · 0 评论