torch.gather()
import torch
a = torch.tensor([
[ 0, 1, 2, 3, 4],
[ 5, 6, 7, 8, 9],
[10, 11, 12, 13, 14]])
b = torch.tensor(
[[1, 0, 0, 0, 0],
[0, 0, 1, 0, 0],
[0, 0, 0, 0, 0]])
c = a.gather(0, b)
d = a.gather(1, b)
print(c)
print(d)
'''
tensor([[5, 1, 2, 3, 4],
[0, 1, 7, 3, 4],
[0, 1, 2, 3, 4]])
tensor([[ 1, 0, 0, 0, 0],
[ 5, 5, 6, 5, 5],
[10, 10, 10, 10, 10]])
'''
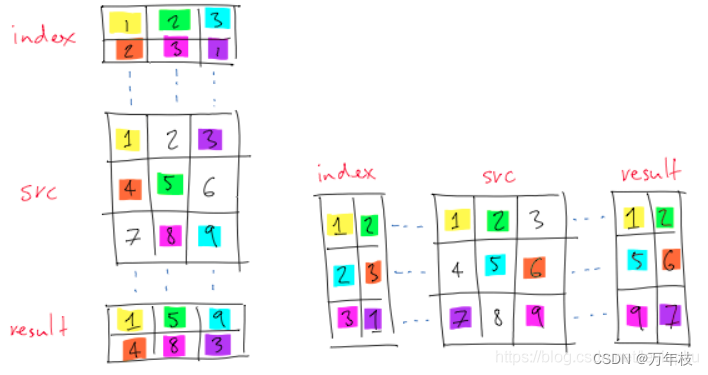
topK()
import numpy as np
import torch
import torch.utils.data.dataset as Dataset
from torch.utils.data import Dataset,DataLoader
tensor1=torch.tensor([[10,1,2,1,1,1,1,1,1,1,10],
[3,4,5,1,1,1,1,1,1,1,1],
[7,8,9,1,1,1,1,1,1,1,1],
[1,4,7,1,1,1,1,1,1,1,1]],dtype=torch.float32)
print(torch.topk(tensor1,k=3,dim=1,largest=True))
print('-'*40)
print(torch.topk(tensor1,k=3,dim=0,largest=True))
'''
torch.return_types.topk(
values=tensor([[10., 10., 2.],
[ 5., 4., 3.],
[ 9., 8., 7.],
[ 7., 4., 1.]]),
indices=tensor([[10, 0, 2],
[ 2, 1, 0],
[ 2, 1, 0],
[ 2, 1, 7]]))
----------------------------------------
torch.return_types.topk(
values=tensor([[10., 8., 9., 1., 1., 1., 1., 1., 1., 1., 10.],
[ 7., 4., 7., 1., 1., 1., 1., 1., 1., 1., 1.],
[ 3., 4., 5., 1., 1., 1., 1., 1., 1., 1., 1.]]),
indices=tensor([[0, 2, 2, 2, 2, 2, 2, 2, 2, 2, 0],
[2, 3, 3, 3, 3, 3, 3, 3, 3, 3, 2],
[1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 3]]))
'''
mean()
import torch
x = torch.tensor([
[[1,2,3,4],[5,6,7,8],[9,10,11,12]],
[[13,14,15,16],[17,18,19,20],[21,22,23,24]]
]).float()
print(x.size())
print(x.mean(dim=0,keepdim=True))
print(x.mean(dim=1,keepdim=True))
print(x.mean(dim=2,keepdim=True))
'''
torch.Size([2, 3, 4])
tensor([[[ 7., 8., 9., 10.],
[11., 12., 13., 14.],
[15., 16., 17., 18.]]])
tensor([[[ 5., 6., 7., 8.]],
[[17., 18., 19., 20.]]])
tensor([[[ 2.5000],
[ 6.5000],
[10.5000]],
[[14.5000],
[18.5000],
[22.5000]]])
'''