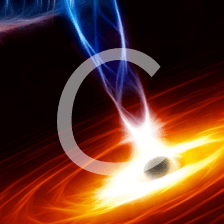
leetcode
文章平均质量分 56
AkibaTakuya
这个作者很懒,什么都没留下…
展开
-
leetcode - Integer to Roman
Given an integer, convert it to a roman numeral.Input is guaranteed to be within the range from 1 to 3999.原创 2014-09-10 17:21:27 · 405 阅读 · 0 评论 -
leetcode - Insertion Sort List
Sort a linked list using insertion sort.原创 2014-09-14 13:49:07 · 342 阅读 · 0 评论 -
leetcode - Palindrome Number
Determine whether an integer is a palindrome. Do this without extra space.Some hints:Could negative integers be palindromes? (ie, -1)If you are thinking of converting the integer to string, no原创 2014-09-11 12:41:34 · 421 阅读 · 0 评论 -
leetcode - Binary Tree Preorder Traversal
Given a binary tree, return the preorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [1,2,3].Note: Recursive soluti原创 2014-09-19 16:53:00 · 539 阅读 · 0 评论 -
leetcode - Reverse Words in a String
Given an input string, reverse the string word by word.For example,Given s = "the sky is blue",return "blue is sky the".click to show clarification.Clarification:What constitutes原创 2014-09-10 15:12:11 · 467 阅读 · 0 评论 -
leetcode - Max Points on a Line
Given n points on a 2D plane, find the maximum number of points that lie on the same straight line.原创 2014-09-13 12:34:13 · 416 阅读 · 0 评论 -
leetcode - Next Permutation
Implement next permutation, which rearranges numbers into the lexicographically next greater permutation of numbers.If such arrangement is not possible, it must rearrange it as the lowest possible原创 2014-09-12 21:06:37 · 503 阅读 · 0 评论 -
leetcode - Sort List
Sort a linked list in O(n log n) time using constant space complexity.原创 2014-09-14 02:51:39 · 382 阅读 · 0 评论 -
leetcode - Pow(x, n)
class Solution {public: double pow(double x, int n) { double res = 1; if(x == 1) return 1; if(x == -1) { if(n % 2) return -1; else return 1; } bool flag = false; if(n < 0)原创 2014-09-19 21:03:54 · 383 阅读 · 0 评论 -
leetcode - Binary Tree Postorder Traversal
Given a binary tree, return the postorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [3,2,1].Note: Recursive solut原创 2014-09-19 16:33:36 · 684 阅读 · 0 评论 -
leetcode - LRU Cache
Design and implement a data structure for Least Recently Used (LRU) cache. It should support the following operations: get and set.get(key) - Get the value (will always be positive) of the key if原创 2014-09-18 22:55:04 · 594 阅读 · 0 评论 -
leetcode - 3Sum
Given an array S of n integers, are there elements a, b, c in S such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.Note:Elements in a triplet (a,b,c原创 2014-09-10 16:56:14 · 378 阅读 · 0 评论 -
leetcode - Linked List Cycle II
Given a linked list, return the node where the cycle begins. If there is no cycle, return null.Follow up:Can you solve it without using extra space?/** * Definition for singly-linked list.原创 2014-09-21 14:56:12 · 495 阅读 · 0 评论 -
leetcode - Sqrt(x)
Implement int sqrt(int x).Compute and return the square root of x.原创 2014-09-19 21:11:22 · 279 阅读 · 0 评论 -
leetcode - Two Sum
Given an array of integers, find two numbers such that they add up to a specific target number.The function twoSum should return indices of the two numbers such that they add up to the target, whe原创 2014-09-10 15:37:47 · 393 阅读 · 0 评论 -
leetcode - Reorder List
Given a singly linked list L: L0→L1→…→Ln-1→Ln,reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→…You must do this in-place without altering the nodes' values.For example,Given {1,2,3,4}, reorder it to原创 2014-09-19 22:20:36 · 481 阅读 · 0 评论 -
leetcode - Roman to Integer
Given a roman numeral, convert it to an integer.Input is guaranteed to be within the range from 1 to 3999.原创 2014-09-13 09:18:39 · 482 阅读 · 0 评论 -
leetcode - Evaluate Reverse Polish Notation
Evaluate the value of an arithmetic expression in Reverse Polish Notation.Valid operators are +, -, *, /. Each operand may be an integer or another expression.Some examples: ["2", "1",原创 2014-09-13 10:39:52 · 312 阅读 · 0 评论 -
leetcode - Single Number
Given an array of integers, every element appears twice except for one. Find that single one.Note:Your algorithm should have a linear runtime complexity. Could you implement it without using ext原创 2014-09-19 21:10:01 · 361 阅读 · 0 评论 -
leetcode - Divide Two Integers
Divide two integers without using multiplication, division and mod operator.原创 2014-09-10 17:14:16 · 414 阅读 · 0 评论 -
leetcode - Reverse Integer
Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321click to show spoilers.Have you thought about this?Here are some good questions to ask before c原创 2014-09-19 21:06:47 · 314 阅读 · 0 评论 -
leetcode - Copy List with Random Pointer
A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null.Return a deep copy of the list./** * Definition for singly-l原创 2014-09-26 11:27:17 · 786 阅读 · 0 评论 -
leetcode - Clone Graph
Clone an undirected graph. Each node in the graph contains a label and a list of its neighbors.OJ's undirected graph serialization:Nodes are labeled uniquely.We use # as a separator for ea原创 2014-09-27 12:58:32 · 712 阅读 · 0 评论 -
leetcode - Word Break II
Given a string s and a dictionary of words dict, add spaces in s to construct a sentence where each word is a valid dictionary word.Return all such possible sentences.For example, givens = "原创 2014-09-25 19:13:31 · 394 阅读 · 0 评论 -
leetcode - Word Break
Given a string s and a dictionary of words dict, determine if s can be segmented into a space-separated sequence of one or more dictionary words.For example, givens = "leetcode",dict = ["leet"原创 2014-09-22 00:00:07 · 522 阅读 · 0 评论 -
leetcode - Linked List Cycle
/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; */struct ListNode{ int val; ListNode *ne原创 2014-09-20 14:09:48 · 653 阅读 · 0 评论 -
leetcode - Single Number II
Given an array of integers, every element appears three times except for one. Find that single one.Note:Your algorithm should have a linear runtime complexity. Could you implement it without usi原创 2014-09-26 18:36:01 · 359 阅读 · 0 评论 -
leetcode - String to Integer (atoi)
Implement atoi to convert a string to an integer.Hint: Carefully consider all possible input cases. If you want a challenge, please do not see below and ask yourself what are the possible input ca原创 2014-09-19 21:14:27 · 391 阅读 · 0 评论 -
leetcode - Gas Station
//假设sum为总的耗油量,max为起始点a到终点b的耗油量,如果,当到达b的时候,//max < 0,那么,出发点肯定不能从a开始,这个时候将max = 0,然后,pos = i+1,选择pos为起始点,然后继续遍历。//如果,最后的sum = 0,则返回pos.class Solution {public: int canCompleteCircuit(std::vector原创 2014-09-27 10:41:45 · 463 阅读 · 0 评论 -
leetcode - 3Sum Closest
Given an array S of n integers, find three integers in S such that the sum is closest to a given number, target. Return the sum of the three integers. You may assume that each input would have exact原创 2014-09-28 09:57:31 · 305 阅读 · 0 评论 -
leetcode - Palindrome Partitioning II
Given a string s, partition s such that every substring of the partition is a palindrome.Return the minimum cuts needed for a palindrome partitioning of s.For example, given s = "aab",Return原创 2014-09-27 18:42:03 · 324 阅读 · 0 评论 -
leetcode - Sum Root to Leaf Numbers
Given a binary tree containing digits from 0-9 only, each root-to-leaf path could represent a number.An example is the root-to-leaf path 1->2->3 which represents the number 123.Find the tota原创 2014-09-28 17:00:42 · 534 阅读 · 0 评论 -
leetcode - Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head.For example,Given 1->2->3->4, you should return the list as 2->1->4->3.Your algorithm should use only constant space. Y原创 2014-09-28 12:40:50 · 694 阅读 · 0 评论 -
leetcode - Longest Common Prefix
Write a function to find the longest common prefix string amongst an array of strings.原创 2014-09-28 10:21:30 · 553 阅读 · 0 评论 -
leetcode - Candy
class Solution {public: int candy(std::vector &ratings) { std::vector ans(ratings.size(),0); ans[0] = 1; int sz = ratings.size(); for (int i = 1; i < sz; i++) { if(ratings[i-1] < rat原创 2014-09-26 19:27:06 · 416 阅读 · 0 评论 -
leetcode - Symmetric Tree
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).For example, this binary tree is symmetric: 1 / \ 2 2 / \ / \3 4 4 3But the f原创 2014-09-28 17:24:04 · 641 阅读 · 0 评论 -
leetcode - Add Two Numbers
You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a link原创 2014-09-28 10:09:29 · 346 阅读 · 0 评论 -
leetcode - Palindrome Partitioning
Given a string s, partition s such that every substring of the partition is a palindrome.Return all possible palindrome partitioning of s.For example, given s = "aab",Return [ ["aa","原创 2014-09-27 17:17:33 · 625 阅读 · 0 评论 -
leetcode - Construct Binary Tree from Preorder and Inorder Traversal
Given preorder and inorder traversal of a tree, construct the binary tree.Note:You may assume that duplicates do not exist in the tree./** * Definition for binary tree * struct TreeNode {原创 2014-10-06 11:38:01 · 621 阅读 · 0 评论 -
leetcode - Binary Tree Level Order Traversal II
Given a binary tree, return the bottom-up level order traversal of its nodes' values. (ie, from left to right, level by level from leaf to root).For example:Given binary tree {3,9,20,#,#,15,7},原创 2014-10-03 16:30:19 · 553 阅读 · 0 评论