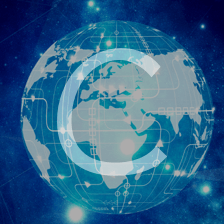
Leetcode
a1eafall
这个作者很懒,什么都没留下…
展开
-
151. Reverse Words in a String 字符串
题目地址使用string str来暂存每次得到的单词,使用vector<string> vs来记录所有单词,对题目提供的字符串进行一次扫描,遇到非空格就追加到str中, 遇到空格就添加新的单词,并将现有str清空,防止重复添加。class Solution {public: void reverseWords(string &s) { string str;原创 2016-10-16 10:45:59 · 389 阅读 · 0 评论 -
357. Count Numbers with Unique Digits 排列组合
题目地址简单的排列组合问题,数字一共10个,所以不重复的数最多占10位,也就是0<=x<10^10,只需要考虑n<=10的情况,从n位数到1一位数依次遍历,统计。 最后一个0单独考虑。class Solution {public: int countNumbersWithUniqueDigits(int n) { int ans = 0; if (n >原创 2017-03-30 16:40:52 · 557 阅读 · 0 评论 -
109. Convert Sorted List to Binary Search Tree
题目地址 和上题108类似,关键在于分割当前链表,记得将左子链表尾部置NULL。class Solution {public: TreeNode *sortedListToBST(ListNode *head) { if (!head) { return NULL; } int size = 0; L原创 2017-01-29 15:15:38 · 475 阅读 · 0 评论 -
108. Convert Sorted Array to Binary Search Tree 有序数组转换为平衡二叉搜索树
题目地址 要求二叉搜索树,则是左大右小,平衡的话,左右子树高度差不超过1,首先考虑大小为1,2,3……的数组,中间的为头结点,左边为左子树,右边为右子树,然后扩展到一般的数组,左右子数的构建用递归就可以了。class Solution {public: TreeNode *sortedArrayToBST(vector<int> &nums) { if (nums.emp原创 2017-01-29 15:09:12 · 331 阅读 · 0 评论 -
66. Plus One
题目地址注意当输入为空时应该输出1.class Solution {public: vector<int> plusOne(vector<int> &digits) { reverse(digits.begin(), digits.end()); vector<int> ans; int carry = 0; for (int原创 2016-10-06 23:07:50 · 364 阅读 · 0 评论 -
67. Add Binary
题目地址二级制的大数运算,注意输入为0的情况。class Solution {public: string addBinary(string a, string b) { reverse(a.begin(), a.end()); reverse(b.begin(), b.end()); string ans; int i, c原创 2016-10-06 23:10:15 · 310 阅读 · 0 评论 -
206. Reverse Linked List 链表
题目地址头插法。/** * Definition for singly-linked list. * */struct ListNode { int val; ListNode *next; ListNode(int x) : val(x), next(NULL) {}};class Solution {public: ListNode *reverseLis原创 2016-10-06 23:12:33 · 358 阅读 · 0 评论 -
43. Multiply Strings 大数
题目地址把较大的数作为被乘数,与乘数的每一位相乘,然后加到最后的结果上。 使用数组存储最后的结果,注意相乘后的位数。class Solution {public: string multiply(string num1, string num2) { string ans; const int maxn = num1.size() + num2.size(原创 2016-10-06 23:05:50 · 235 阅读 · 0 评论 -
236. Lowest Common Ancestor of a Binary Tree
题目地址/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */c原创 2016-10-19 16:56:45 · 268 阅读 · 0 评论 -
19. Remove Nth Node From End of List 链表遍历
题目地址设置两个指针,一个先走n步,然后两个同步走,这样当前一个走到表尾的时候,后一个就走到了倒数第n个。/** * Definition for singly-linked list. * */struct ListNode { int val; ListNode *next; ListNode(int x) : val(x), next(NULL) {}};clas原创 2016-10-06 22:57:58 · 262 阅读 · 0 评论 -
14. Longest Common Prefix
题目地址dd注意判空class Solution {public: string longestCommonPrefix(vector<string> &strs) { string ans = ""; if (strs.size() == 0) return ans; int len = strs[0].size(); fo原创 2016-10-06 22:50:26 · 262 阅读 · 0 评论 -
2. Add Two Numbers 大数运算
题目地址注意长度不等时的情况class Solution {public: ListNode *addTwoNumbers(ListNode *l1, ListNode *l2) { vector<int> v1, v2, v3; while (l1 != NULL) { v1.push_back(l1->val);原创 2016-10-06 22:43:45 · 321 阅读 · 0 评论 -
1. Two Sum
题目地址直接枚举,复杂度O(n^2).class Solution {public: vector<int> twoSum(vector<int> &nums, int target) { vector<int> ans; for (int i = 0; i < nums.size(); ++i) { for (int j = i +原创 2016-10-06 22:37:52 · 197 阅读 · 0 评论 -
146. LRU Cache hash+链表
题目地址使用了一个hash表和一个链表,每次访问元素(get)或是添加元素都将元素置于链表的头部,尾部的自然就是最久未使用的。 hash表存储元素在链表中的位置,链表储存key-value,以方便超出容量时从hash表移除旧元素。class LRUCache {public: unordered_map<int, list<pair<int,int>>::iterator> ump;原创 2017-03-16 22:48:25 · 681 阅读 · 0 评论