有三个字符串 S,S1,S2,其中,S 长度不超过 300,S1和 S2的长度不超过 10。
现在,我们想要检测 S1 和 S2是否同时在 S中出现,且 S1 位于 S2的左边,并在 S中互不交叉(即,S1 的右边界点在 S2 的左边界点的左侧)。
计算满足上述条件的最大跨距(即,最大间隔距离:最右边的 S2 的起始点与最左边的 S1 的终止点之间的字符数目)。
如果没有满足条件的 S1,S2 存在,则输出 −1。
例如,S= abcd123ab888efghij45ef67kl, S1=S1= ab, S2=S2= ef,其中,S1S1 在 SS 中出现了 2 次,S2 也在 S 中出现了 2 次,最大跨距为:18。
输入格式
输入共一行,包含三个字符串 S,S1,S2,字符串之间用逗号隔开。
数据保证三个字符串中不含空格和逗号。
输出格式
输出一个整数,表示最大跨距。
如果没有满足条件的 S1 和 S2存在,则输出 −1。
输入样例:
abcd123ab888efghij45ef67kl,ab,ef
输出样例:
18
一、介绍string.find()函数:
返回字符串s1在s中的位置,如果没有找到,则返回-1
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
string s="what are you dong";
string s1="are";
int position;
position=s.find(s1);
if(position==-1)
cout<<"not find"<<endl;
else
cout<<"position= "<<position<<endl;
return 0;
}
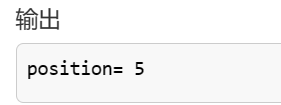
返回任意字符s1在s中第一次出现的位置,s1为字符, 'a' "a"都可以
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
string s="hahahaha";
string s1="a";
//char s1='a';
int position;
position=s.find_first_of(s1);
cout<<position<<endl;
return 0;
}
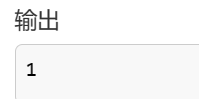
从字符串s下标为a开始查找字符串s1,返回起始位置 s.find(s1,a); 查找不到返回-1
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
string s="have a good time";
string s1="good";
int position;
position=s.find(s1,3);
cout<<position<<endl;
position=s.find(s1,12);
cout<<position<<endl;
return 0;
}
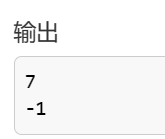
查找字符s1在s中出现的所有起始位置
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
string s="abb abb abb";
string s1="a"; //char s1='a';
int position=0;
int i=0;
while((position=s.find_first_of(s1,position))!=string::npos)
{
cout<<position<<endl;
position++;
i++;//i为出现的总次数
}
cout<<"total="<<i<<endl;
return 0;
}
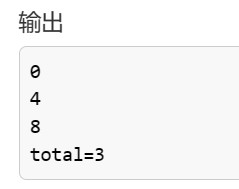
反向查找,从字符串右侧开始匹配s1,并返回在字符串中的位置(下标)
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
string s="aaabcd";
string s1="abc";
int position;
position=s.rfind(s1);
cout<<position<<endl;
return 0;
}
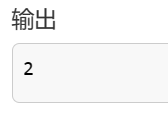
二、解决方案:
1.不使用find函数
#include<iostream>
using namespace std;
int main(){
string s,s1,s2;
int n=0;
char a;
while(cin>>a)
{
if(a!=',')
{
if(n==0) s+=a;
else if(n==1) s1+=a;
else s2+=a;
}
else n++;
}
//cout<<s<<endl<<s1<<endl<<s2;
//cout<<s.size()<<endl;
int count1=0,count2=0,result=0;
for(int i=0;i<=s.size()-s1.size();i++){
int j=0;
for(;j<s1.size();j++){
if(s[i+j] !=s1[j]) break;
//cout<<i<<' '<<j<<endl;
//else cout<<j<<endl;
}
//cout<<j<<endl;
if(j==s1.size()){
count1=i+j;//s1最右边
//cout<<i<<" "<<j;
//cout<<count1<<endl;
break;
}
// else{
// cout<<"-1";
// return 0;
// }
}
int max=0;
for(int i=count1;i<=s.size()-s2.size();i++){
int k=0;
for(;k<s2.size();k++){
if(s[i+k] !=s2[k]) break;
}
//cout<<i<<endl;
if(k==s2.size()){
count2=i;//s2最左边
if(count2>max) max=count2;
}
// else{
// cout<<"-1";
// return 0;
// }
//cout<<max<<endl;
}
result=max-count1;
if(count1>0 && max>0) cout<<result;
else cout<<"-1";
return 0;
}
2.使用find函数
#include<iostream>
#include<string>
using namespace std;
int main()
{
string S,S1,S2;
int i=0;
char a;
while(cin>>a)
{
if(a!=',')
{
if(i==0) S+=a;
else if(i==1) S1+=a;
else S2+=a;
}
else i++;
}
int s,s1,s2;
s1=S.find(S1);
s2=S.rfind(S2);
if(s1!=-1&&s2!=-1&&s1+S1.length()-1<s2){
cout<<s2-s1-S1.length()<<endl;
}
else cout<<"-1"<<endl;
}