一、判断Path指向目录还是文件
- 在
net.xxr.hdfs
包里创建PathToFileOrDir
类
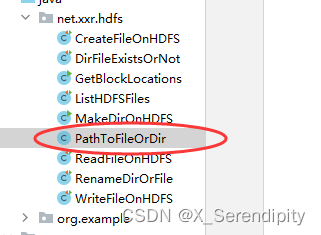
package net.xxr.hdfs;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import java.net.URI;
public class PathToFileOrDir {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
conf.set("dfs.client.use.datanode.hostname", "true");
String uri = "hdfs://master:9000";
FileSystem fs = FileSystem.get(new URI(uri), conf, "root");
Path path1 = new Path("/ied01");
if (fs.isDirectory(path1)) {
System.out.println("[" + path1 + "]指向的是目录!");
} else {
System.out.println("[" + path1 + "]指向的是文件!");
}
Path path2 = new Path("/lzy01/test2.txt");
if (fs.isFile(path2)) {
System.out.println("[" + path2 + "]指向的是文件!");
} else {
System.out.println("[" + path2 + "]指向的是目录!");
}
}
}
- 结果
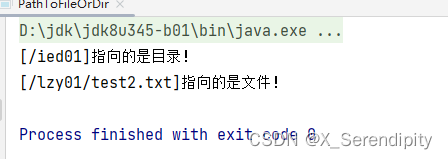
二、删除目录或文件
- 在
net.xxr.hdfs
包里创建DeleteFileOrDir
类
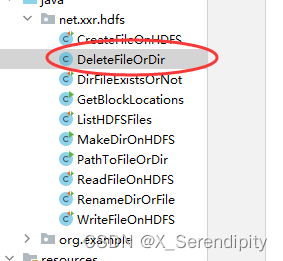
1、删除文件
- 删除
/lzy/hello.txt
文件 - 编写
deleteFile()
方法
package net.xxr.hdfs;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.junit.Test;
import java.net.URI;
public class DeleteFileOrDir {
@Test
public void deleteFile() throws Exception {
Configuration conf = new Configuration();
conf.set("dfs.client.use.datanode.hostname", "true");
String uri = "hdfs://master:9000";
FileSystem fs = FileSystem.get(new URI(uri), conf, "root");
Path path = new Path(uri + "/lzy01/hello.txt");
boolean result = fs.delete(path, false);
if (result) {
System.out.println("文件[" + path + "]删除成功!");
} else {
System.out.println("文件[" + path + "]删除失败!");
}
}
}
- 结果
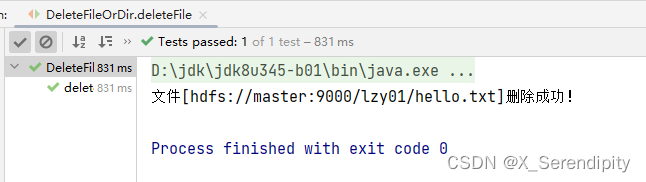
- 利用Hadoop WebUI界面查看
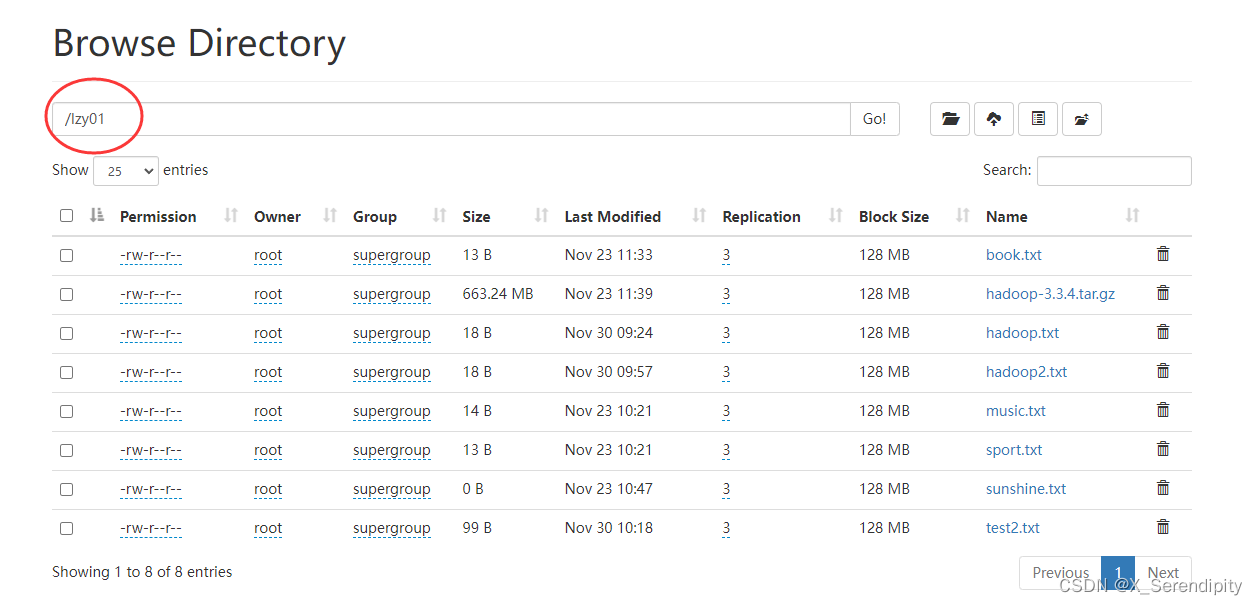
- 再运行
deleteFile()
测试方法,查看结果
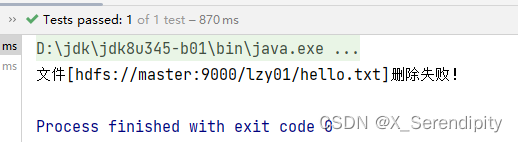
- 可以在删除文件之前,判断文件是否存在,需要修改代码
package net.xxr.hdfs;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.junit.Test;
import java.net.URI;
public class DeleteFileOrDir {
@Test
public void deleteFile() throws Exception {
Configuration conf = new Configuration();
conf.set("dfs.client.use.datanode.hostname", "true");
String uri = "hdfs://master:9000";
FileSystem fs = FileSystem.get(new URI(uri), conf, "root");
Path path = new Path(uri + "/lzy01/hi.txt");
if (fs.exists(path)) {
boolean result = fs.delete(path, false);
if (result) {
System.out.println("文件[" + path + "]删除成功!");
} else {
System.out.println("文件[" + path + "]删除失败!");
}
} else {
System.out.println("文件[" + path + "]不存在!");
}
}
}
- 结果
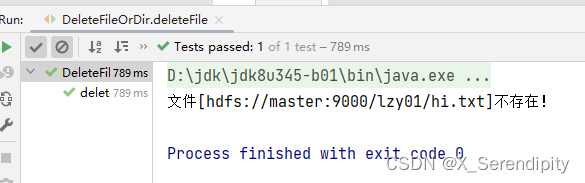
2、删除目录
- 删除
/ied01
目录 - 编写
deleteDir()
方法
@Test
public void deleteDir() throws Exception {
Configuration conf = new Configuration();
conf.set("dfs.client.use.datanode.hostname", "true");
String uri = "hdfs://master:9000";
FileSystem fs = FileSystem.get(new URI(uri), conf, "root");
Path path = new Path(uri + "/ied01");
if (fs.exists(path)) {
boolean result = fs.delete(path, true);
if (result) {
System.out.println("目录[" + path + "]删除成功!");
} else {
System.out.println("目录[" + path + "]删除失败!");
}
} else {
System.out.println("目录[" + path + "]不存在!");
}
}
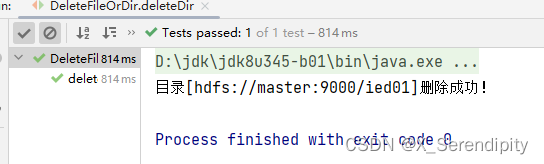
- 再运行
deleteDir()
方法,查看结果
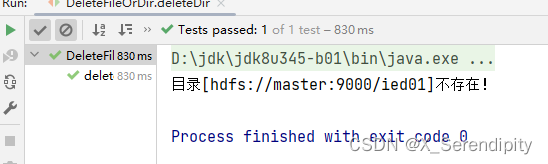
3、删除目录或文件
- 进行三个层面的判断:判断类型(目录或文件)、判断是否存在、判断删除是否成功
- 删除
/ied03/exam.txt
文件和/ied02
目录 - 编写
delete()
方法
@Test
public void delete() throws Exception {
Configuration conf = new Configuration();
conf.set("dfs.client.use.datanode.hostname", "true");
String uri = "hdfs://master:9000";
FileSystem fs = FileSystem.get(new URI(uri), conf, "root");
Random random = new Random();
int choice = random.nextInt(100) % 2;
String[] strPath = {"/ied03/exam.txt", "/ied02"};
Path path = new Path(uri + strPath[choice]);
String type = "";
if (fs.isDirectory(path)) {
type = "目录";
} else {
type = "文件";
}
if (fs.exists(path)) {
boolean result = fs.delete(path, true);
if (result) {
System.out.println(type + "[" + path + "]删除成功!");
} else {
System.out.println(type + "[" + path + "]删除失败!");
}
} else {
System.out.println(type + "[" + path + "]不存在!");
}
}