框架图
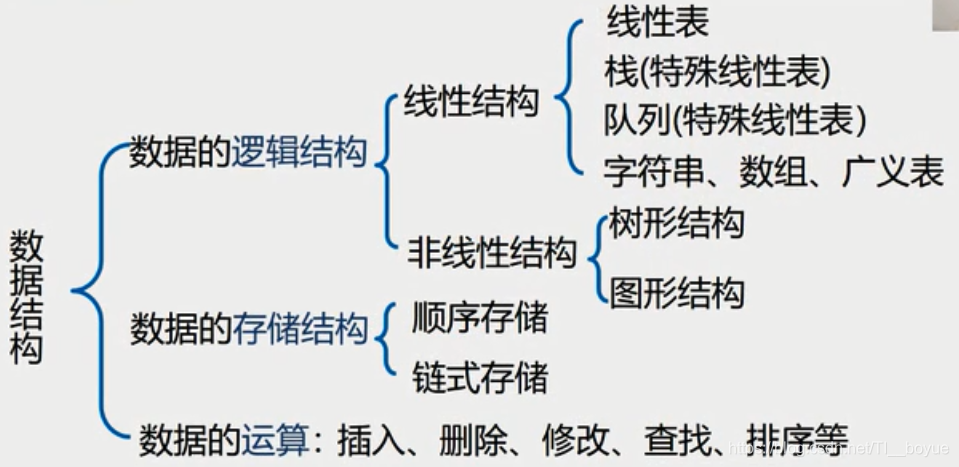
线性表
顺序结构
#include<iostream>
using namespace std;
#define maxsize 100
typedef int elemtype;
#define ok 1
#define error 0
typedef int status;
typedef struct List{
elemtype data[maxsize];
int length;
}list;
void create_list(list* sqlist);
void out_elem(list sqlist);
status get_elem(list sqlist,int i,elemtype *e);
status insert(list& sqlist,int i,elemtype e);
status delete1(list& sqlist,int i,elemtype *e);
int main(){
list sqlist;
create_list(&sqlist);
out_elem(sqlist);
cout<<endl;
cout<<"获取第i个元素"<<endl;
elemtype e;
cout<<get_elem(sqlist,2,&e)<<endl;
cout<<e<<endl;
cout<<"插入元素"<<endl;
cout<<insert(sqlist,3,9)<<endl;
out_elem(sqlist);
cout<<endl;
cout<<"删除元素"<<endl;
cout<<delete1(sqlist,4,&e)<<endl;
out_elem(sqlist);
cout<<endl;
return 0;
}
创建线性表
void create_list(list* sqlist){
elemtype temp[] = {1,2,3,4,5};
int length = sizeof(temp)/sizeof(temp[0]);
for(int i=0;i<length;i++){
sqlist->data[i] = temp[i];
}
sqlist->length = length;
}
输出验证
void out_elem(list sqlist){
for(int i=0;i<sqlist.length;i++){
cout<<sqlist.data[i]<<' ';
}
}
获取第i个元素
status get_elem(list sqlist,int i,elemtype *e){
if(sqlist.length == 0 || i<1 || i>sqlist.length)
return error;
*e = sqlist.data[i-1];
return ok;
}
插入元素到特定位置
status insert(list& sqlist,int i,elemtype e){
if(sqlist.length == maxsize){
cout<<"空间已满"<<endl;
return error;
}
if(i<1 ||i>sqlist.length+1){
cout<<"i不在范围"<<endl;
return error;
}
int k = sqlist.length;
if(i!=k+1){
while(i<=k){
sqlist.data[k]=sqlist.data[k-1];
k--;
}
}
sqlist.data[k] = e;
sqlist.length++;
return ok;
}
删除特定位置元素
status delete1(list& sqlist,int i,elemtype *e){
if(sqlist.length == 0 || i<1 || i>sqlist.length)
return error;
*e = sqlist.data[i-1];
if(i!=sqlist.length){
while(sqlist.length > i){
sqlist.data[i-1] = sqlist.data[i];
i++;
}
}
sqlist.length --;
return ok;
}
整体代码
#include<iostream>
using namespace std;
#define maxsize 100
typedef int elemtype;
#define ok 1
#define error 0
typedef int status;
typedef struct List{
elemtype data[maxsize];
int length;
}list;
void create_list(list* sqlist);
void out_elem(list sqlist);
status get_elem(list sqlist,int i,elemtype *e);
status insert(list& sqlist,int i,elemtype e);
status delete1(list& sqlist,int i,elemtype *e);
int main(){
list sqlist;
create_list(&sqlist);
out_elem(sqlist);
cout<<endl;
cout<<"获取第i个元素"<<endl;
elemtype e;
cout<<get_elem(sqlist,2,&e)<<endl;
cout<<e<<endl;
cout<<"插入元素"<<endl;
cout<<insert(sqlist,3,9)<<endl;
out_elem(sqlist);
cout<<endl;
cout<<"删除元素"<<endl;
cout<<delete1(sqlist,4,&e)<<endl;
out_elem(sqlist);
cout<<endl;
return 0;
}
void create_list(list* sqlist){
elemtype temp[] = {1,2,3,4,5};
int length = sizeof(temp)/sizeof(temp[0]);
for(int i=0;i<length;i++){
sqlist->data[i] = temp[i];
}
sqlist->length = length;
}
void out_elem(list sqlist){
for(int i=0;i<sqlist.length;i++){
cout<<sqlist.data[i]<<' ';
}
}
status get_elem(list sqlist,int i,elemtype *e){
if(sqlist.length == 0 || i<1 || i>sqlist.length)
return error;
*e = sqlist.data[i-1];
return ok;
}
status insert(list& sqlist,int i,elemtype e){
if(sqlist.length == maxsize){
cout<<"空间已满"<<endl;
return error;
}
if(i<1 ||i>sqlist.length+1){
cout<<"i不在范围"<<endl;
return error;
}
int k = sqlist.length;
if(i!=k+1){
while(i<=k){
sqlist.data[k]=sqlist.data[k-1];
k--;
}
}
sqlist.data[k] = e;
sqlist.length++;
return ok;
}
status delete1(list& sqlist,int i,elemtype *e){
if(sqlist.length == 0 || i<1 || i>sqlist.length)
return error;
*e = sqlist.data[i-1];
if(i!=sqlist.length){
while(sqlist.length > i){
sqlist.data[i-1] = sqlist.data[i];
i++;
}
}
sqlist.length --;
return ok;
}
链式结构
#include<iostream>
using namespace std;
typedef int elemtype;
typedef struct node {
elemtype data;
struct node *next;
} node, *link_node;
typedef int status;
#define ok 1
#define error 0
void create_node_list(link_node head);
void out_elem(node head_node);
status get_elem(link_node head, int i, elemtype *e);
status insert_node(link_node head, int i, elemtype e);
status delete_node(link_node head, int i, elemtype *e);
status delete_list(link_node head);
int main() {
node head_node;
cout << "创建链表" << endl;
create_node_list(&head_node);
out_elem(head_node);
cout << "获取第i个元素" << endl;
elemtype e;
cout << get_elem(&head_node, 10, &e) << endl;
cout << e << endl;
cout << "插入元素" << endl;
cout << insert_node(&head_node, 5, 12) << endl;
out_elem(head_node);
cout << "删除元素" << endl;
cout << delete_node(&head_node, 5, &e) << endl;
cout << e << endl;
out_elem(head_node);
cout<<"删除整表"<<endl;
delete_list(&head_node);
out_elem(head_node);
return 0;
}
创建链表
void create_node_list(link_node head) {
link_node p, q;
elemtype array[] = { 1,2,3,4,5,6,7,8,9,10 };
int length = sizeof(array) / sizeof(array[0]);
q = head;
head->data = length;
head->next = NULL;
for (int i = 0; i < length; i++) {
p = new node;
p->data = array[i];
p->next = NULL;
q->next = p;
q = p;
}
}
输出验证
void out_elem(node head_node) {
link_node p = head_node.next;
while (p) {
cout << p->data << ' ';
p = p->next;
}
cout << endl;
}
获取第i个元素
status get_elem(link_node head, int i, elemtype *e) {
if (head->data == 0 || i<1 || i>head->data)
return error;
int count = 1;
link_node p = head->next;
while (p && count < i) {
p = p->next;
count++;
}
*e = p->data;
return ok;
}
插入元素
status insert_node(link_node head, int i, elemtype e) {
if (i<1 || i>head->data + 1) return error;
link_node q, p = head;
int count = 1;
while (p && count < i) {
p = p->next;
count++;
}
q = new node;
q->data = e;
q->next = p->next;
p->next = q;
head->data++;
return ok;
}
删除元素
status delete_node(link_node head, int i, elemtype *e) {
if (head->data ==0 || i<1 || i>head->data)
return error;
int count = 1;
link_node q, p = head;
while (p && count < i) {
p = p->next;
count++;
}
q = p->next;
*e = q->data;
p->next = q->next;
delete q;
head->data--;
return ok;
}
删除整表
status delete_list(link_node head){
link_node q,p=head->next;
while(p){
q=p;
p=q->next;
delete q;
head->data--;
}
head->next=NULL;
return ok;
}
完整代码
#include<iostream>
using namespace std;
typedef int elemtype;
typedef struct node {
elemtype data;
struct node *next;
} node, *link_node;
typedef int status;
#define ok 1
#define error 0
void create_node_list(link_node head);
void out_elem(node head_node);
status get_elem(link_node head, int i, elemtype *e);
status insert_node(link_node head, int i, elemtype e);
status delete_node(link_node head, int i, elemtype *e);
status delete_list(link_node head);
int main() {
node head_node;
cout << "创建链表" << endl;
create_node_list(&head_node);
out_elem(head_node);
cout << "获取第i个元素" << endl;
elemtype e;
cout << get_elem(&head_node, 10, &e) << endl;
cout << e << endl;
cout << "插入元素" << endl;
cout << insert_node(&head_node, 5, 12) << endl;
out_elem(head_node);
cout << "删除元素" << endl;
cout << delete_node(&head_node, 5, &e) << endl;
cout << e << endl;
out_elem(head_node);
cout<<"删除整表"<<endl;
delete_list(&head_node);
out_elem(head_node);
return 0;
}
void create_node_list(link_node head) {
link_node p, q;
elemtype array[] = { 1,2,3,4,5,6,7,8,9,10 };
int length = sizeof(array) / sizeof(array[0]);
q = head;
head->data = length;
head->next = NULL;
for (int i = 0; i < length; i++) {
p = new node;
p->data = array[i];
p->next = NULL;
q->next = p;
q = p;
}
}
void out_elem(node head_node) {
link_node p = head_node.next;
while (p) {
cout << p->data << ' ';
p = p->next;
}
cout << endl;
}
status get_elem(link_node head, int i, elemtype *e) {
if (head->data == 0 || i<1 || i>head->data)
return error;
int count = 1;
link_node p = head->next;
while (p && count < i) {
p = p->next;
count++;
}
*e = p->data;
return ok;
}
status insert_node(link_node head, int i, elemtype e) {
if (i<1 || i>head->data + 1) return error;
link_node q, p = head;
int count = 1;
while (p && count < i) {
p = p->next;
count++;
}
q = new node;
q->data = e;
q->next = p->next;
p->next = q;
head->data++;
return ok;
}
status delete_node(link_node head, int i, elemtype *e) {
if (head->data ==0 || i<1 || i>head->data)
return error;
int count = 1;
link_node q, p = head;
while (p && count < i) {
p = p->next;
count++;
}
q = p->next;
*e = q->data;
p->next = q->next;
delete q;
head->data--;
return ok;
}
status delete_list(link_node head){
link_node q,p=head->next;
while(p){
q=p;
p=q->next;
delete q;
head->data--;
}
head->next=NULL;
return ok;
}
链表翻转
迭代反转法
void turn_iteration_list(link_node head){
if(head->next == NULL || head->next->next == NULL) return;
link_node p,q,s;
q=NULL;
p=head->next;
s=p->next;
while(1){
p->next =q;
if(s == NULL) break;
q=p;
p=s;s=p->next;
}
head->next = p;
}
递归翻转法
link_node turn_recursive_list(link_node first){
if(first == NULL || first->next == NULL) return first;
link_node new_head = turn_recursive_list(first->next);
first->next->next = first;
first->next = NULL;
return new_head;
}
头插法翻转
void turn_insert_head_list(link_node head){
if(head->next == NULL || head->next->next == NULL) return;
link_node end = head;
while(end->next){
end = end->next;
}
link_node p,q;
p=head->next;
while(p!=end){
q=p->next;
p->next = end->next;
end->next = p;
p = q;
}
head->next = end;
}
就地逆置法翻转
void turn_local_list(link_node head){
if(head->next == NULL || head->next->next == NULL) return;
link_node p,q;
q=head->next;
p=q->next;
while(p){
q->next = p->next;
p->next = head->next;
head->next = p;
p=q->next;
}
}
完整代码
#include<iostream>
using namespace std;
typedef int elemtype;
typedef struct node{
elemtype data;
struct node *next;
}node,*link_node;
void create_node_list(link_node head) {
link_node p, q;
elemtype array[] = {1,2,3,4,5,6,7,8,9,10};
int length = sizeof(array) / sizeof(array[0]);
q = head;
head->data = length;
head->next = NULL;
for (int i = 0; i < length; i++) {
p = new node;
p->data = array[i];
p->next = NULL;
q->next = p;
q = p;
}
}
void out_elem(node head_node) {
link_node p = head_node.next;
while (p) {
cout << p->data << ' ';
p = p->next;
}
cout << endl;
}
void turn_iteration_list(link_node head);
link_node turn_recursive_list(link_node first);
void turn_insert_head_list(link_node head);
void turn_local_list(link_node head);
int main(){
node head_node;
create_node_list(&head_node);
out_elem(head_node);
cout<<"迭代翻转法:"<<endl;
turn_iteration_list(&head_node);
out_elem(head_node);
cout<<"迭代翻转法"<<endl;
head_node.next = turn_recursive_list(head_node.next);
out_elem(head_node);
cout<<"头插法翻转"<<endl;
turn_insert_head_list(&head_node);
out_elem(head_node);
cout<<"就地翻转"<<endl;
turn_local_list(&head_node);
out_elem(head_node);
return 0;
}
void turn_iteration_list(link_node head){
if(head->next == NULL || head->next->next == NULL) return;
link_node p,q,s;
q=NULL;
p=head->next;
s=p->next;
while(1){
p->next =q;
if(s == NULL) break;
q=p;
p=s;s=p->next;
}
head->next = p;
}
link_node turn_recursive_list(link_node first){
if(first == NULL || first->next == NULL) return first;
link_node new_head = turn_recursive_list(first->next);
first->next->next = first;
first->next = NULL;
return new_head;
}
void turn_insert_head_list(link_node head){
if(head->next == NULL || head->next->next == NULL) return;
link_node end = head;
while(end->next){
end = end->next;
}
link_node p,q;
p=head->next;
while(p!=end){
q=p->next;
p->next = end->next;
end->next = p;
p = q;
}
head->next = end;
}
void turn_local_list(link_node head){
if(head->next == NULL || head->next->next == NULL) return;
link_node p,q;
q=head->next;
p=q->next;
while(p){
q->next = p->next;
p->next = head->next;
head->next = p;
p=q->next;
}
}
合并链表
#include<iostream>
using namespace std;
typedef int elemtype;
typedef struct node{
elemtype data;
struct node *next;
}node,*link_node;
void create_node_list(link_node head,elemtype array[],int length);
void out_elem(node head_node);
void combine_node_list(link_node head_combin,node head_node1,node head_node2);
void combin_node_list_no(link_node head1,link_node head2);
int main(){
node head_node1,head_node2,head_node_combine;
elemtype array1[]={0,1,3,5,7,9};
elemtype array2[]={1,2,4,6,8,10,11,12};
cout<<"head_node1"<<endl;
create_node_list(&head_node1,array1,sizeof(array1)/sizeof(array1[0]));
out_elem(head_node1);
cout<<"head_node2"<<endl;
create_node_list(&head_node2,array2,sizeof(array2)/sizeof(array2[0]));
out_elem(head_node2);
cout<<"合并后新建链表"<<' ';
combine_node_list(&head_node_combine,head_node1,head_node2);
cout<<"长度:"<<head_node_combine.data<<endl;
out_elem(head_node_combine);
cout<<"原空间-以head_node1为主";
combin_node_list_no(&head_node1,&head_node2);
cout<<" 长度:"<<head_node1.data<<endl;
out_elem(head_node1);
return 0;
}
void create_node_list(link_node head,elemtype array[],int length){
head->data=length;
head->next=NULL;
link_node q,p;
p=head;
for(int i=0;i<length;i++){
q = new node;
q->data = array[i];
q->next = NULL;
p->next = q;
p=q;
}
}
void out_elem(node head_node){
if(head_node.next == NULL) return;
link_node p=head_node.next;
while(p){
cout<<p->data<<" ";
p=p->next;
}
cout<<endl;
}
void combine_node_list(link_node head_combin,node head_node1,node head_node2){
link_node p,q,s,m;
elemtype temp;
head_combin->next=NULL;
head_combin->data=0;
p=head_combin;
q=head_node1.next;
s=head_node2.next;
while(q&&s){
if(q->data<=s->data){
temp=q->data;
q=q->next;
}else {
temp = s->data;
s=s->next;
}
m = new node;
m->data = temp;
m->next = NULL;
p->next=m;
p=m;
}
if(q){
p->next=q;
}
if(s){
p->next=s;
}
head_combin->data = head_node1.data+head_node2.data;
}
void combin_node_list_no(link_node head1,link_node head2){
link_node p,q,s;
s=head1;
p=head1->next;q=head2->next;
while( p && q){
if(p->data<=q->data){
s->next = p;
s=p;
p=p->next;
}else {
s->next = q;
s=q;
q=q->next;
}
}
if(p) s->next = p;
if(q) s->next = q;
head1->data=head1->data+head2->data;
}
传送门
青岛大学-王卓-数据结构
单链表反转详解(4种算法实现)
合并两个单链表(链表方式)