连接过程
public class Test1 {
static String url = "jdbc:mysql://localhost:3306/day17";
static String user = "root";
static String password = "1123";
public static void main(String[] args) throws SQLException {
Driver driver = new Driver();
DriverManager.deregisterDriver(driver);
Connection conn = DriverManager.getConnection(url, user, password);
Statement stt = conn.createStatement();
String sql = "select * from user";
ResultSet resultSet = stt.executeQuery(sql);
while(resultSet.next()){
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
String address = resultSet.getString("address");
System.out.println("id:"+id+" name:"+name+" address:"+address);
}
resultSet.close();
stt.close();
conn.close();
}
}
先编译SQL后传参
public class Test2 {
static String url = "jdbc:mysql://localhost:3306/day17";
static String user = "root";
static String password = "1123";
public static void main(String[] args) {
Connection conn = null;
PreparedStatement ps = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(url, user, password);
String sql = "insert into user(id,name,gender,age,address) value(?,?,?,?,?)";
ps = conn.prepareStatement(sql);
ps.setInt(1,55);
ps.setString(2,"张长旭");
ps.setString(3,"男");
ps.setInt(4,18);
ps.setString(5,"背景");
ps.execute();
} catch (Exception e){
e.printStackTrace();
}finally {
try {
ps.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
JDBC
概念
- Java DataBase Connectivity Java 数据库连接,java语言操作数据库
- 本质:是sun公司(官方)定义的一套所有关系型数据库的规则,即接口。各个数据库厂商,去实现这套接口提供数据库驱动jar包,我们使用这套接口编程,真正执行的代码是jar包中的实现类。
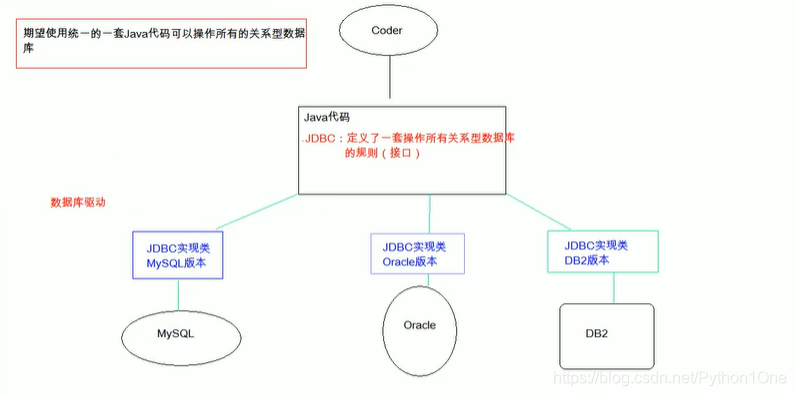
快速入门
步骤
- 导入驱动jar包
1. 复制jar包带项目的libs目录下
2. 项目右键—>add as library - 注册驱动
Class.forName("com.mysql.jdbc.Driver");
- 获取数据库连接对象
Connection
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/day17", "root", "1123");
- 定义sql
String sql = "update user set age = 50 where id = 1";
- 获取执行sql语句的对象
Statement
Statement stmt = conn.createStatement();
- 执行SQL,接收返回值
int count = stmt.executeUpdate(sql);
- 处理返回值(结果)
System.out.println(count);
- 释放资源
stmt.close(); conn.close();
public class JdbcDemo1 {
public static void main(String[] args) throws Exception {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/day17", "root", "1123");
String sql = "update user set age = 50 where id = 1";
Statement stmt = conn.createStatement();
int count = stmt.executeUpdate(sql);
System.out.println(count);
stmt.close();
conn.close();
}
}
详解各个对象
DriverManager:驱动管理对象
注册驱动:告诉程序使用哪一个数据库驱动jar。
- 源码中存在静态代码块。执行
Class.forName("com.mysql.jdbc.Driver");
会注册驱动 - mysql 5之后的驱动jar包可以省略注册步骤。
获取数据库连接:
- 方法:static Connection getConnection(String url,String user, String password);
- 参数:
- url:指定连接的路径
- 语法:
jdbc:mysql://ip地址(域名):端口号/数据库名称
- 例子:
jdbc:mysql://localhost:3306/day17
- 细节:本地服务器,端口为3306,url可以简写为
jdbc:mysql:///数据库名称
- user:用户名
- password:密码
Connection :数据库连接对象
1.获取执行SQL的对象
StatementcreateStatement()
PreparedStatement prepareStatement(String sql)
2.管理事务:
- 开启事务:
void setAutoCommit(boolean autoCommit)
:调用该方法设置参数为false,即开启事务 - 提交事务:
void commit()
- 回滚事务:
void rollback()
Statement :执行SQL的对象
执行SQL
boolean execute(String sql)
:可以执行任意的SQLint executeUpdate(String sql)
:执行DML(insert, update, delete)语句(常用)、DDL(create,alter,drop)语句(不常用)
- 返回值:影响的行数,通过影响行数,判断DML语句是否执行成功,返回值>0则执行成功
ResultSet executeQuery(String sql)
:执行DQL(select)语句
案例
- 插入一条数据
public class JdbcDemo2 {
public static void main(String[] args) {
Statement stmt = null;
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String sql = "insert into user(id,name,gender) values(60,'zcx','nv')";
conn = DriverManager.getConnection("jdbc:mysql:///day17", "root", "1123");
stmt = conn.createStatement();
int count = stmt.executeUpdate(sql);
System.out.println(count);
if(count > 0){
System.out.println("添加成功");
}else {
System.out.println("添加失败");
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if(stmt != null) {
try {
stmt.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn != null) {
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
}
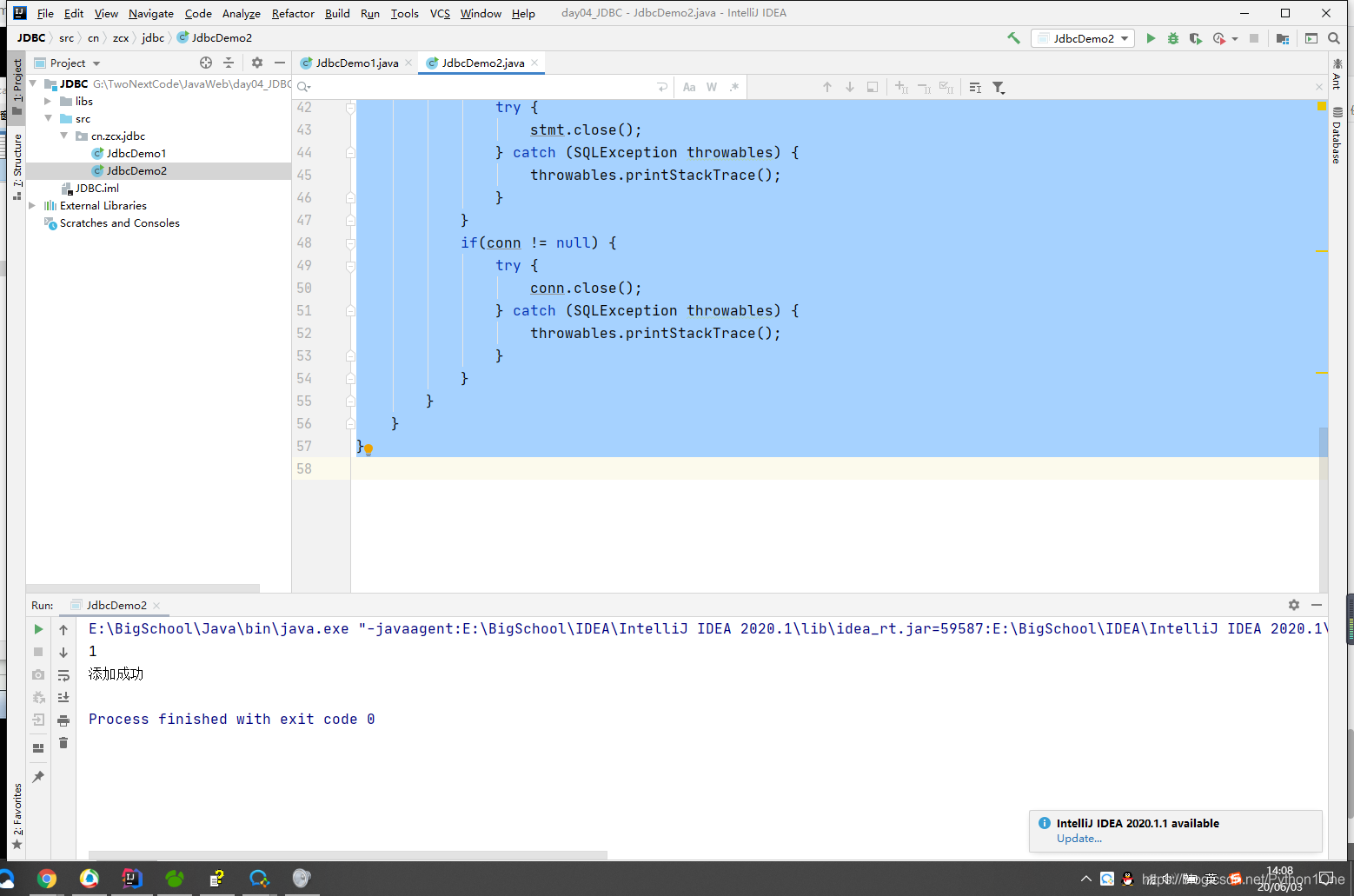
- 修改一条数据
public class JdbcDemo3 {
public static void main(String[] args) {
Statement stmt = null;
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String sql = "update user set age=60 where id = 1";
conn = DriverManager.getConnection("jdbc:mysql:///day17", "root", "1123");
stmt = conn.createStatement();
int count = stmt.executeUpdate(sql);
System.out.println(count);
if(count > 0){
System.out.println("修改成功");
}else {
System.out.println("修改失败");
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if(stmt != null) {
try {
stmt.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn != null) {
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
}
- 删除一条数据
public class JdbcDemo4 {
public static void main(String[] args) {
Statement stmt = null;
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String sql = "delete from user where id = 60";
conn = DriverManager.getConnection("jdbc:mysql:///day17", "root", "1123");
stmt = conn.createStatement();
int count = stmt.executeUpdate(sql);
System.out.println(count);
if(count > 0){
System.out.println("删除成功");
}else {
System.out.println("删除失败");
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if(stmt != null) {
try {
stmt.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn != null) {
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
}
- 执行DDL创建表
public class JdbcDemo5 {
public static void main(String[] args) {
Statement stmt = null;
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String sql = "create table student(id int,name varchar(20) ,age int)";
conn = DriverManager.getConnection("jdbc:mysql:///day17", "root", "1123");
stmt = conn.createStatement();
int count = stmt.executeUpdate(sql);
System.out.println(count);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if(stmt != null) {
try {
stmt.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn != null) {
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
}
ResultSet:结果集对象,来封装结果
boolean next()
:游标向下移动一行,判断当前行是否是最后一行末尾(是否有数据),如果是末尾(无数据)则返回falsegetXxx(参数)
:获取数据
- Xxx代表数据类型
int getInt() || String getString()
- 参数:
- Int:列编号,从1开始。如
getString("age")
- String:列名
public class JdbcDemo6 {
public static void main(String[] args) {
Statement stmt = null;
Connection conn = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String sql = "select * from user";
conn = DriverManager.getConnection("jdbc:mysql:///day17", "root", "1123");
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
while( rs.next() ){
int id = rs.getInt("id");
String name = rs.getString("name");
System.out.println("id:" + id + "--name:" + name);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if(rs != null) {
try {
rs.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(stmt != null) {
try {
stmt.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn != null) {
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
}
- 练习:将表中数据封装为对象,打印输出
- 定义User类
- 定义方法
public List<User> findAll(){}
- 实现方法
select * from user
PrepareStatement:执行SQL的对象
JDBC工具类:JDBCUtils
- 目的:简化书写
- 分析:
- 注册驱动抽取
- 抽取一个方法获取连接
- 要求:不传参,保证工具类的通用性
- 方法:通过配置文件
- 抽取一个方法释放资源
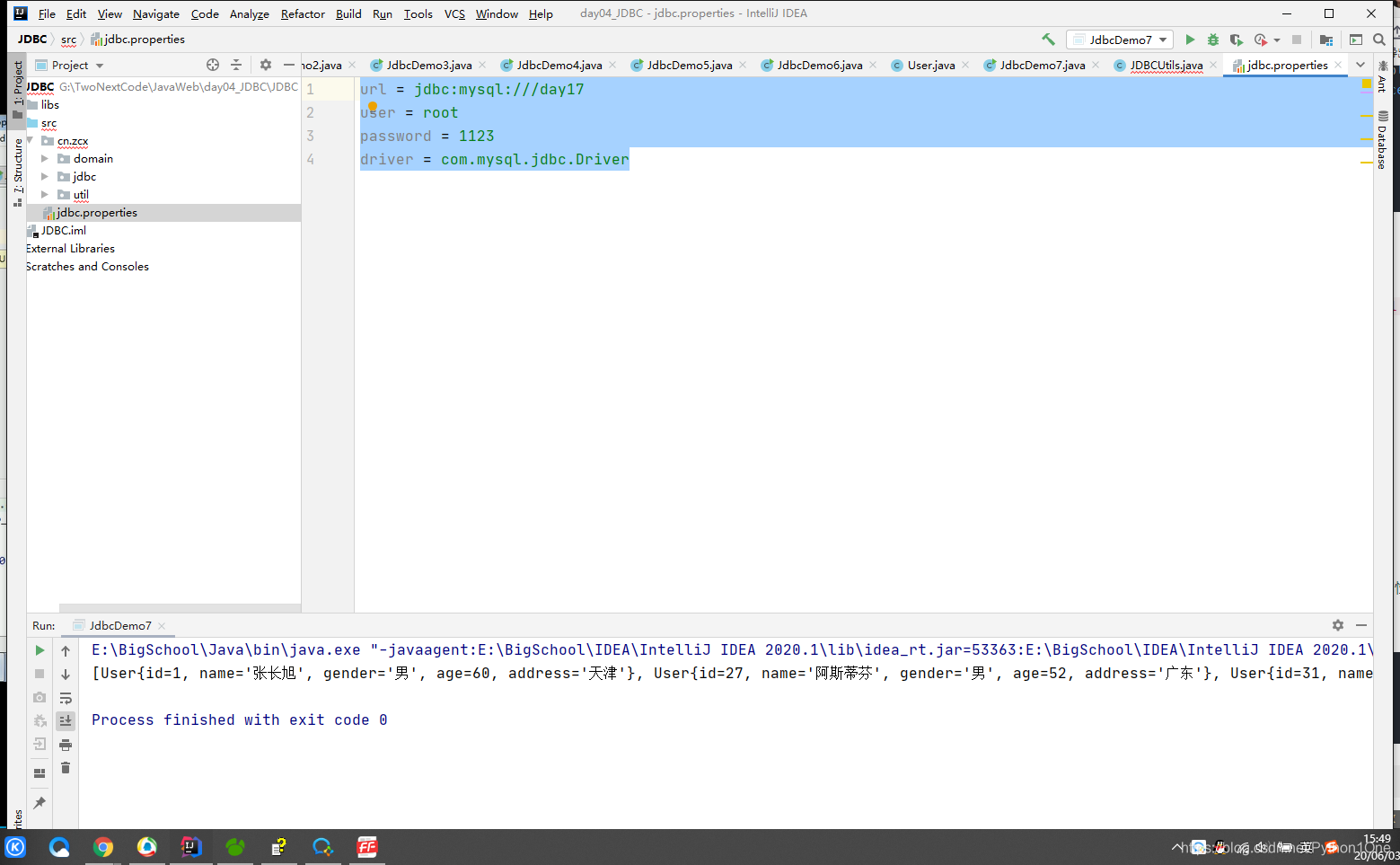
url = jdbc:mysql:///day17
user = root
password = 1123
driver = com.mysql.jdbc.Driver
public class JDBCUtils {
private static String url;
private static String user;
private static String password;
private static String driver;
static {
try {
Properties pro = new Properties();
ClassLoader classLoader = JDBCUtils.class.getClassLoader();
URL res = classLoader.getResource("jdbc.properties");
String path = res.getPath();
System.out.println(path);
pro.load(new FileReader(path));
url = pro.getProperty("url");
user = pro.getProperty("user");
password = pro.getProperty("password");
driver = pro.getProperty("driver");
Class.forName(driver);
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(url,user,password);
}
public static void close(Statement stmt,Connection conn){
if (stmt != null){
try {
stmt.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if (conn != null){
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
public static void close(ResultSet rs, Statement stmt, Connection conn){
if (rs != null){
try {
rs.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if (stmt != null){
try {
stmt.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if (conn != null){
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
案例–登录案例
步骤
- 创建数据库表
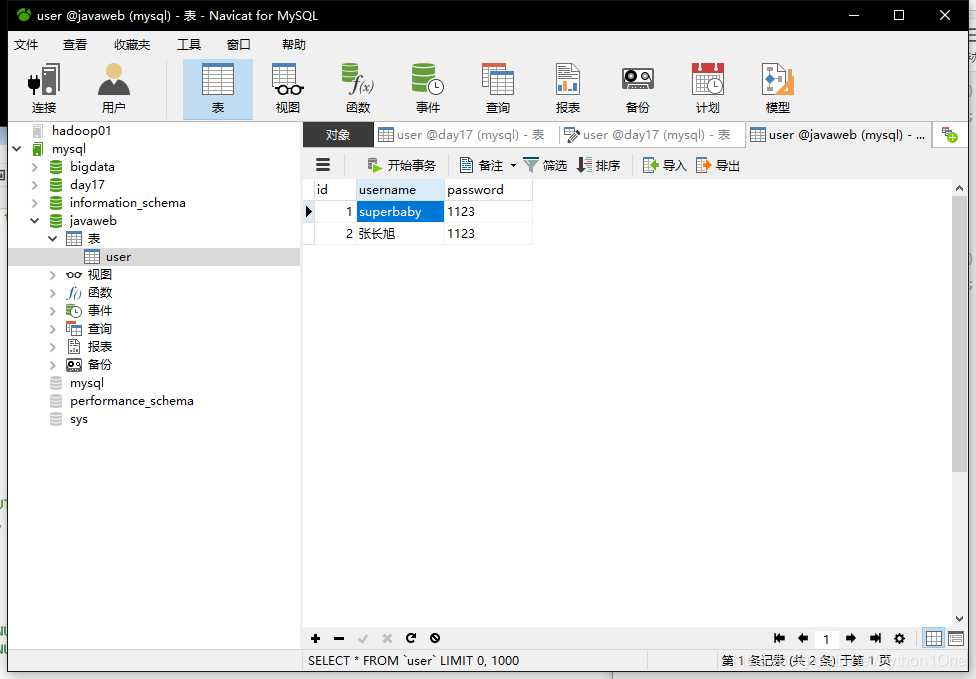
- 编写代码
public class JDBCDemo9_Login {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("输入用户名:");
String username = sc.nextLine();
System.out.print("输入密码:");
String password = sc.nextLine();
boolean flag = new JDBCDemo9_Login().login(username, password);
if (flag){
System.out.println("登录成功");
}else {
System.out.println("登录失败");
}
}
public boolean login(String username,String password){
if(username == null || password == null){
return false;
}
Connection conn = null;
Statement stmt = null;
ResultSet res = null;
try {
conn = JDBCUtils.getConnection();
String sql = "select * from user where username = '"+username+"' and password = '"+password+"'";
stmt = conn.createStatement();
res = stmt.executeQuery(sql);
return res.next();
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtils.close(res,stmt,conn);
}
return false;
}
}