ajax简介
- Ajax :“Asynchronous Javascript And XML”(异步 JavaScript 和 XML)
ajax的优点
- 无需重新加载整个网页的情况下,能够更新部分网页内容的技术
- 允许根据用户事件来更新部分网页内容
ajax的缺点
- 没有浏览历史,不能回退
- 存在跨域问题,只能访问同源网站内容(解决办法:jsonp/cors设置请求头)
- SEO不友好
ajax的使用方法
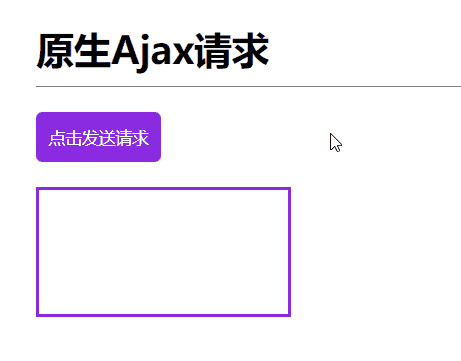
原生ajax
- 创建对象:
const xhr = new XMLHttpRequest();
- 设置请求方法:
xhr.open('GET', 'http://localhost:8000/server');
- 发送请求:
xhr.send();
- 处理服务端返回的结果
const btn = document.getElementsByTagName('button');
<script>
btn[0].onclick = function () {
const xhr = new XMLHttpRequest();
xhr.open('GET', 'http://localhost:8000/server');
xhr.send();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
if (xhr.status >= 200 && xhr.readyState <= 300) {
console.log(xhr.status);
console.log(xhr.statusText);
console.log(xhr.getAllResponseHeaders);
console.log(xhr.response);
result.innerHTML = xhr.response;
} else {
}
}
}
}
</script>
<script>
btn[0].onclick = function(){
const xhr = new XMLHttpRequest();
xhr.open('POST', 'http://localhost:8000/server');
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
xhr.send('a=100&&b=200&&c=300');
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
if(xhr.status >= 200 && xhr.status<300){
result.innerHTML = xhr.response;
}
}
};
};
</script>
xhr.timeout = 2000;
xhr.ontimeout = function (){
alert('网络异常,超时');
};
xhr.onerror = function(){
alert('网络异常');
};
btns[1].onclick = function(){
xhr.abort()};
jquery-ajax
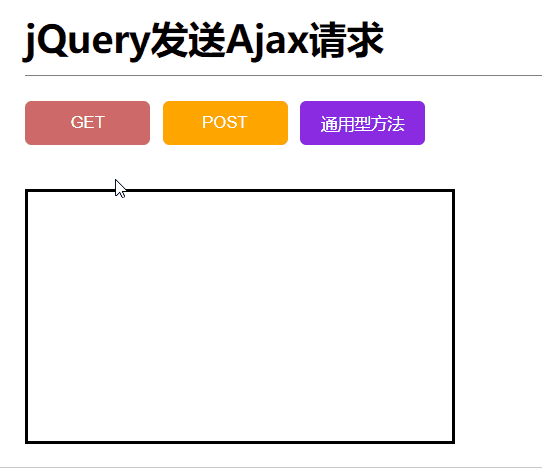
<script>
$('button').eq(0).click(function () {
$.get('http://localhost:8000/jquery-server', { a: 100, b: 200 }, function (data) {
console.log(data);
}, 'json')
});
$('button').eq(1).click(function () {
$.post('http://localhost:8000/jquery-server', { a: 100, b: 200 }, function (data) {
console.log(data);
})
});
$('button').eq(2).click(function () {
$.ajax({
url: 'http://localhost:8000/jquery-server',
data: { a: 100, b: 200 },
type: 'GET',
dataType: 'json',
success: function (data) {
console.log(data);
},
timeout: 2000,
error: function () {
console.log('出错了');
},
headers: {
c: 300,
d: 400
}
})
})
</script>
axios-ajax
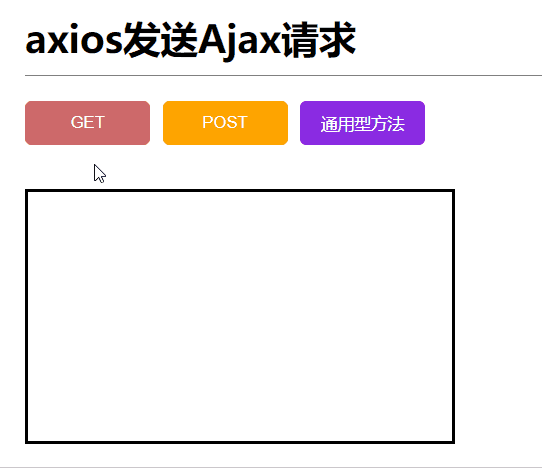
<script>
const btns = document.querySelectorAll('button')
btns[0].onclick = function () {
axios.get('http://localhost:8000/axios-server', {
params: {
id: 1000,
vip: 10
},
headers: {
name: 'hanser',
age: '10'
}
}).then(value => {
console.log(value);
})
}
btns[1].onclick = function () {
axios.post('http://localhost:8000/axios-server', {
username: 'admin',
password: '123'
}, {
params: {
id: 1,
vip: 123
},
headers: {
name: 'younghd',
age: '22'
},
...
})
}
btns[2].onclick = function () {
axios({
method: 'POST',
url: 'http://localhost:8000/axios-server',
params: {
vip: 10,
id: 123
},
headers: {
a: 100,
b: 200
},
data: {
name: 'younghd',
age: '22'
}
}).then(response => {
console.log(response);
})
}
</script>
fetch()-ajax
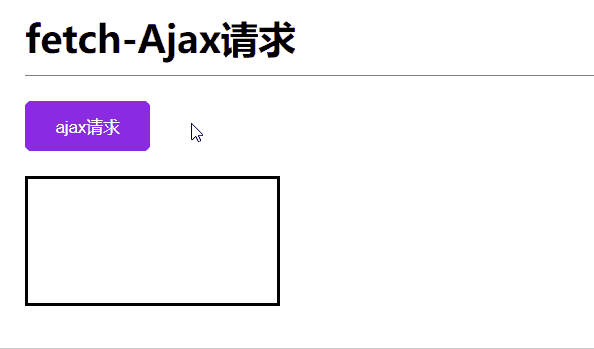
<script>
const btn = document.getElementsByTagName('button');
btn[0].onclick = function () {
fetch('http://localhost:8000/fetch-server', {
method: 'POST',
headers: {
name: 'YoungHD'
},
body: 'name=admin&pd=password'
}).then(Response => {
console.log(Response);
return Response.text();
}).then(Response => {
console.log(Response);
})
}
</script>
服务器端:node.js-express
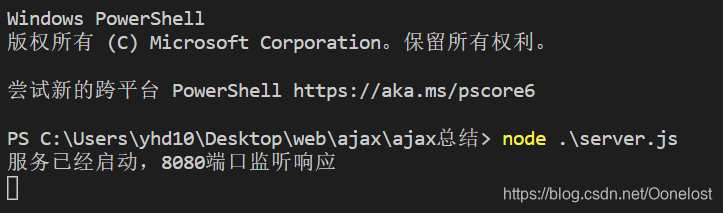
const express = require('express');
const app = express();
app.get('/server', (requrest, response)=>{
response.setHeader('Access-Control-Allow-Origin', '*')
response.send('这是原生ajax');
});
app.all('/jquery-server', (requrest, response)=>{
response.setHeader('Access-Control-Allow-Origin', '*')
response.setHeader('Access-Control-Allow-Headers', '*')
const data = {name:'jquery-ajax'}
response.send(JSON.stringify(data))
});
app.all('/axios-server', (requrest, response)=>{
response.setHeader('Access-Control-Allow-Origin', '*')
response.setHeader('Access-Control-Allow-Headers', '*')
const data = {name:'axios-ajax'};
response.send(JSON.stringify(data));
});
app.all('/fetch-server', (requrest, response)=>{
response.setHeader('Access-Control-Allow-Origin', '*')
response.setHeader('Access-Control-Allow-Headers', '*')
const data = {name:'fetch-ajax'};
response.send(JSON.stringify(data));
});
app.listen(8000,()=>{
console.log("服务已经启动,8080端口监听响应");
});