两种方式实现最小栈
minstack.c 引用 stcak_seq.h(详见栈的实现文章)https://blog.youkuaiyun.com/Alsnoa/article/details/80216882
#include<stdio.h>
#include"stack_seq.h"
#define TEST_HEADER printf("==================%s==================\n",__FUNCTION__)
//Question:return min value as O(1)time stack (two way).
//1.Fisrt insert two value.
typedef struct MinStack
{
SeqStack stack;
}MinStack;
void MinStackInit(MinStack* minstack)
{
if(minstack==NULL)
{
return;
}
InitSeqStack(&minstack->stack);
}
void DestoryMinStack(MinStack* minstack)
{
if(minstack==NULL)
{
return;
}
DestoryStack(&minstack->stack);
}
void MinStackPush(MinStack* minstack,TypeStack value)
{
if(minstack==NULL)
{
return;
}
int i;
TypeStack tmp;
TypeStack min=value;
i=SeqStackTop(&minstack->stack,&tmp);
if(i>0 && tmp<min)
{
min=tmp;
}
SeqStackPush(&minstack->stack,value);
SeqStackPush(&minstack->stack,min);
}
void MinStackPop(MinStack* minstack)
{
if(minstack==NULL)
{
return;
}
SeqStackPop(&minstack->stack);
SeqStackPop(&minstack->stack);
}
int MinStackTop(MinStack* minstack,TypeStack* value)
{
if(minstack==NULL)
{
return 0;
}
return SeqStackTop(&minstack->stack, value);
}
void Test()
{
TEST_HEADER;
MinStack min;
TypeStack value;
int i;
MinStackInit(&min);
MinStackPush(&min,'a');
MinStackPush(&min,'b');
MinStackPush(&min,'c');
MinStackPush(&min,'d');
MinStackPush(&min,'e');
i=MinStackTop(&min,&value);
printf("Ret expect:1 actully:%d \n",i);
printf("Top expect:a actully:%c \n",value);
MinStackPop(&min);
i=MinStackTop(&min,&value);
printf("Ret expect:1 actully:%d \n",i);
printf("Top expect:a actully:%c \n",value);
DestoryMinStack(&min);
i=MinStackTop(&min,&value);
printf("Ret expect:0 actully:%d \n",i);
printf("Top expect: actully:%c \n",value);
}
//2.Use two stack to complete.
typedef struct TwoStack{
SeqStack first;
SeqStack second;
}TwoStack;
void TwoStackInit(TwoStack* min)
{
if(min==NULL)
{
return;
}
InitSeqStack(&min->first);
InitSeqStack(&min->second);
}
void DestoryTwoStack(TwoStack* min)
{
if(min==NULL)
{
return;
}
DestoryStack(&min->first);
DestoryStack(&min->second);
}
void TwoStackPush(TwoStack* min,TypeStack value)
{
if(min==NULL)
{
return;
}
TypeStack minvalue;
int i;
i=SeqStackTop(&min->second,&minvalue);
if(i=0)
{//first intert.
minvalue=value;
}
else if(i>0)
{
if(value<minvalue)
{
minvalue=value;
}
}
SeqStackPush(&min->first,value);
SeqStackPush(&min->second,minvalue);
}
void TwoStackPop(TwoStack* min)
{
if(min==NULL)
{
return;
}
SeqStackPop(&min->first);
SeqStackPop(&min->second);
}
int TwoStackTop(TwoStack* min,TypeStack *value1,TypeStack* value2)
{
if(min==NULL|| value1==NULL|| value2==NULL)
{
return 0;
}
SeqStackTop(&min->first,value1);
SeqStackTop(&min->second,value2);
return 1;
}
void Test2()
{
TEST_HEADER;
int i;
TwoStack min;
TypeStack tmp1;
TypeStack tmp2;
TwoStackInit(&min);
TwoStackPush(&min,'a');
TwoStackPush(&min,'b');
TwoStackPush(&min,'c');
TwoStackPush(&min,'d');
TwoStackPush(&min,'e');
TwoStackPop(&min);
i=TwoStackTop(&min,&tmp1,&tmp2);
printf("i except: 1 ,actully:%d \n",i);
printf("tmp1 expect:d ,actully:%c \n",tmp1);
printf("tmp2 expect:a ,acurlly:%c \n",tmp2);
DestoryTwoStack(&min);
i=TwoStackTop(&min,&tmp1,&tmp2);
printf("i except: 0 ,actully:%d \n",i);
printf("tmp1 expect: ,actully:%c \n",tmp1);
printf("tmp2 expect: ,acurlly:%c \n",tmp2);
}
int main()
{
Test();
Test2();
return 0;
}
两个栈实现一个队列 queue_by_two_stack.c
引用 stcak_seq.h(详见栈的实现文章)https://blog.youkuaiyun.com/Alsnoa/article/details/80216882
#include<stdio.h>
#include"stack_seq.h"
#define TEST_HEADER printf("================%s===============\n",__FUNCTION__)
//1.two stack to complete a queue.
typedef struct Queue_by_two_stack{
SeqStack input;
SeqStack output;
}Queue;
void QueueInit(Queue* que)
{
if(que==NULL)
{
return;
}
InitSeqStack(&que->input);
InitSeqStack(&que->output);
}
void DestoryQueue(Queue* que)
{
if(que==NULL)
{
return;
}
DestoryStack(&que->input);
DestoryStack(&que->output);
}
void QueuePush(Queue* que,TypeStack value)
{
if(que==NULL)
{
return;
}
while(1)
{
int i;
TypeStack tmp;
i=SeqStackTop(&que->output,&tmp);
if(i==0)
{
break;
}
else if(i>0)
{
SeqStackPush(&que->input,tmp);
}
SeqStackPop(&que->output);
}
SeqStackPush(&que->input,value);
}
void QueuePop(Queue* que)
{
if(que==NULL)
{
return;
}
while(1)
{
int i;
TypeStack tmp;
i=SeqStackTop(&que->input,&tmp);
if(i==0)
{
break;
}
else if(i>0)
{
SeqStackPush(&que->output,tmp);
}
SeqStackPop(&que->input);
}
SeqStackPop(&que->output);
}
int QueueTop(Queue* que,TypeStack *value)
{
if(que==NULL)
{
return;
}
while(1)
{
int i;
TypeStack tmp;
i=SeqStackTop(&que->input,&tmp);
if(i==0)
{
break;
}
else if(i>0)
{
SeqStackPush(&que->output,tmp);
}
SeqStackPop(&que->input);
}
return SeqStackTop(&que->output,value);
}
void Test()
{
TEST_HEADER;
Queue que;
int i;
TypeStack tmp;
QueueInit(&que);
QueuePush(&que,'a');
QueuePush(&que,'b');
QueuePush(&que,'c');
QueuePush(&que,'d');
QueuePush(&que,'e');
QueuePop(&que);
ShowStruct(&(&que)->output);
i=QueueTop(&que,&tmp);
printf("i expect:1 actully:%d \n",i);
printf("top exptct:b actully:%c \n",tmp);
DestoryQueue(&que);
i=QueueTop(&que,&tmp);
printf("i expect:0 actully:%d \n",i);
printf("top exptct: actully:%c \n",tmp);
}
int main()
{
Test();
return 0;
}
stack_by_two_queue.c 引用queue_seq.h 原文链接https://blog.youkuaiyun.com/Alsnoa/article/details/80217742
#include<stdio.h>
#include"queue_seq.h"
#define TEST_HEADER printf("=============%s============\n",__FUNCTION__)
typedef struct Stack_By_Two_Queeue{
SeqQueue queue1;
SeqQueue queue2;
}Stack;
void StackInit(Stack* stack)
{
if(stack==NULL)
{
return;
}
SeqQueueInit(&stack->queue1);
SeqQueueInit(&stack->queue2);
}
void StackPush(Stack *stack ,TypeQueue value)
{
if(stack==NULL)
{
return;
}
//fisrt insert in queue2
if(stack->queue1.size==0)
{
SeqQueuePush(&stack->queue2,value);
}
else
{
SeqQueuePush(&stack->queue1,value);
}
}
void StackPop(Stack* stack)
{
if(stack==NULL)
{
return;
}
if(stack->queue1.size==0 && stack->queue2.size==0)
{
return;
}
if(stack->queue1.size==0)
{
TypeQueue value;
while(1)
{
if(stack->queue2.size==1)
{
break;
}
SeqQueueTop(&stack->queue2,&value);
SeqQueuePush(&stack->queue1,value);
SeqQueuePop(&stack->queue2);
}
SeqQueuePop(&stack->queue2);
}
else
{
TypeQueue value;
while(1)
{
SeqQueueTop(&stack->queue1,&value);
if(stack->queue1.size==1)
{
break;
}
SeqQueuePush(&stack->queue2,value);
SeqQueuePop(&stack->queue1);
}
SeqQueuePop(&stack->queue1);
}
}
int StackTop(Stack*stack ,TypeQueue* value)
{
if(stack==NULL || value==NULL)
{
return 0;
}
if(stack->queue1.size==0 && stack->queue2.size==0)
{
return 0;
}
if(stack->queue1.size==0)
{
while(1)
{
if(stack->queue2.size==0)
{
break;
}
SeqQueueTop(&stack->queue2,value);
SeqQueuePush(&stack->queue1,*value);
SeqQueuePop(&stack->queue2);
}
}
else
{
while(1)
{
if(stack->queue1.size==0)
{
break;
}
SeqQueueTop(&stack->queue1,value);
SeqQueuePush(&stack->queue2,*value);
SeqQueuePop(&stack->queue1);
}
}
return 1;
}
void Test()
{
TEST_HEADER;
Stack stack;
TypeQueue tmp;
size_t a;
StackInit(&stack);
StackPush(&stack,'a');
StackPush(&stack,'b');
StackPush(&stack,'c');
StackPush(&stack,'d');
StackPush(&stack,'e');
StackPush(&stack,'f');
StackTop(&stack,&tmp);
printf("expect:f, acutlly:%c \n",tmp);
StructShow(&stack.queue1);
StructShow(&stack.queue2);
StackPop(&stack);
StackPop(&stack);
StackPop(&stack);
StackPop(&stack);
StackTop(&stack,&tmp);
printf("expect:b, acutlly:%c \n",tmp);
StructShow(&stack.queue1);
StructShow(&stack.queue2);
StackPop(&stack);
StackPop(&stack);
StackPop(&stack);
StackPop(&stack);
StructShow(&stack.queue1);
StructShow(&stack.queue2);
}
int main()
{
Test();
return 0;
}
=============Test============
expect:f, acutlly:f
Seq->top:a
Seq->head:0
Seq->tail:5
Seq->size:6
Seq->top:f
Seq->head:5
Seq->tail:5
Seq->size:0
expect:b, acutlly:b
Seq->top:b
Seq->head:9
Seq->tail:9
Seq->size:0
Seq->top:a
Seq->head:11
Seq->tail:12
Seq->size:2
Seq->top:a
Seq->head:9
Seq->tail:9
Seq->size:0
Seq->top:b
Seq->head:12
Seq->tail:12
Seq->size:0
if_number_from_stack.c 引用stack_seq.h https://blog.youkuaiyun.com/Alsnoa/article/details/80216882
#include<stdio.h>
#include<string.h>
#include"stack_seq.h"
#define TEST_HEADER printf("\n=============%s=============\n",__FUNCTION__)
//1.to judge if the string was accounding to the stackpush and stack pop.
int If_number_from_stack_pop(char arr_in[],size_t size_in,\
char arr_out[], size_t size_out)
{
if(size_in==0||size_out==0)
{
return 0;
}
//a b c d e f 6 d e f c b a 6
size_t input=0;
size_t output=0;
SeqStack tmp;
InitSeqStack(&tmp);
for(input=0;input<size_in;input++)
{
SeqStackPush(&tmp,arr_in[input]);
while(1)
{
size_t i;
TypeStack value;
i=SeqStackTop(&tmp,&value);
if(i==0)
{
break;
}
if(value==arr_out[output])
{
SeqStackPop(&tmp);
output++;
printf("%c ",value);
}
else
{
break;
}
}
}
printf("\n");
if(output==size_out)
{
return 1;
}
return 0;
}
void Test()
{
TEST_HEADER;
char arr_in[6]="abcdef";
char arr_out1[6]="defcba";
char arr_out2[6]="defcab";
int i;
i=If_number_from_stack_pop(arr_in,6,arr_out1,6);
printf("i:1 actully:%d \n",i);
i=If_number_from_stack_pop(arr_in,6,arr_out2,6);
printf("i:0 actully:%d \n",i);
}
int main()
{
Test();
return 0;
}
shared_stack.c
#include<stdio.h>
#define TEST_HEADER printf("=============%s==============\n",__FUNCTION__)
#define MaxSize 1024
typedef struct SharedStack{
//[0,top1) [top2,MaxSize)
TypeStack data[MaxSize];
size_t top1;
size_t top2;
}SharedStack;
void SharedStackInit(SharedStack* stack)
{
if(stack==NULL)
{
return;
}
stack->data[0]=0;
stack->top1=0;
stack->top2=MaxSize;
}
void DestorySharedStack(SharedStack* stack)
{
if(stack==NULL)
{
return;
}
stack->data[0]=0;
stack->top1=0;
stack->top2=MaxSize;
}
void Stack1Push(SharedStack* stack,TypeStack value)
{
if(stack==NULL)
{
return;
}
if(stack->top1>=stack->top2)
{
printf("The SharedStack is full!\n");
return;
}
stack->data[stack->top1]=value;
stack->top1++;
}
void Stack2Push(SharedStack* stack, TypeStack value)
{
if(stack==NULL)
{
return;
}
if(stack->top1>=stack->top2)
{
printf("The SharedStack is full!\n");
return;
}
stack->data[stack->top2-1]=value;
stack->top2--;
}
void Stack1Pop(SharedStack* stack)
{
if(stack==NULL)
{
return;
}
stack->top1--;
}
void Stack2Pop(SharedStack* stack)
{
if(stack==NULL)
{
return;
}
stack->top2++;
}
int StackTop1(SharedStack* stack,TypeStack* value)
{
if(stack==NULL)
{
return;
}
if(stack->top1==0)
{
return 0;
}
*value=stack->data[stack->top1-1];
return 1;
}
int StackTop2(SharedStack* stack,TypeStack* value)
{
if(stack==NULL)
{
return;
}
if(stack->top2==MaxSize)
{
*value='0';
return 0;
}
*value=stack->data[stack->top2];
return 1;
}
void Test()
{
TEST_HEADER;
SharedStack share;
int i;
TypeStack value;
SharedStackInit(&share);
Stack1Push(&share,'a');
Stack1Push(&share,'b');
Stack1Push(&share,'c');
Stack1Push(&share,'d');
Stack1Push(&share,'e');
Stack1Pop(&share);
Stack1Pop(&share);
Stack1Pop(&share);
Stack1Pop(&share);
i=StackTop1(&share,&value);
printf("\n----------stack1_test-----------\n");
printf("value: a actully :%c \n",value);
Stack2Push(&share,'a');
Stack2Push(&share,'b');
Stack2Push(&share,'c');
Stack2Push(&share,'d');
Stack2Pop(&share);
i=StackTop2(&share,&value);
printf("\n----------stack2_test-----------\n");
printf("value: c actully :%c \n",value);
for(i=0;i<1020;i++)
{
Stack2Push(&share,'b');
}
printf("\n----------stack_only_full_test-----------\n");
i=StackTop2(&share,&value);
printf("value: b actully :%c \n",value);
printf("\n----------stack_over_full_test-----------\n");
Stack1Push(&share,'c');
i=StackTop1(&share,&value);
printf("value: c actully :%c \n",value);
}
int main()
{
Test();
return 0;
}
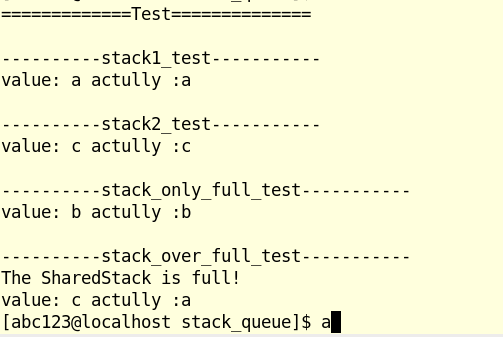