基于JDK1.8
构造方法
- ArrayList()默认长度为10
- ArrayList(Colletion<? extend E> c) 将集合初始化为list元素
- ArrayList(int len)指定长度为len的list
常用方法
- add(E e) 添加元素 ,返回boolean 类型
- add(int index, E e) 在index索引位置,添加元素; void 方法
- addAll(Collection<? extend E> c) 将集合C中的所有元素添加到List的末尾处,返回boolean类型
- addAll(int index, Collection<? extend E> c) 将集合C中的所有元素添加到List的指定索引处,返回boolean类型
- clear() 清空List中的元素, void 类型
- clone() 生成List的一个副本
- contains(Object o)是否包含元素o, boolean类型
- get(int index) 获取索引处的元素
- indexOf(Object o)返回元素o首次出现的位置, 若没有,返回-1;
- isEmpty() 是否为空,boolean
- Iterator() 迭代使用
- lastIndexOf(Object o) 逆向首次出现的元素位置,若没有,返回-1;
- remove(int index) 删除索引处的元素,boolean
- removeAll(Collection<?> c) 删除所有在集合c中出现的元素
- removeRange(int i,int j) 移除I,j范围之间的元素
- retainAll(Collection<?> c) 若集合c中有元素在list中出现,返回true
- set(int index, E element) 将索引index处,设置为元素element
- size() 返回长度
- sublist(int I,int j) 返回I,j之间的子元素list
- toArray() 返回数组
- 实践代码中还包含集中list常用的迭代方式
下面是实践代码
public static void main(String[] args){
//构造方法
ArrayList<String> a = new ArrayList<>();//默认长度为10
ArrayList<String> b = new ArrayList<>(20);//可以指定容量
HashSet<String> hs = new HashSet<String>();
hs.add("a");
hs.add("b");
hs.add("c");
ArrayList<String> c = new ArrayList<>(hs);//可以将一个实现Collection接口的类初始化
System.out.println("默认构造函数长度:"+a.size());
System.out.println("指定初始容量长度:"+b.size());
System.out.println("Collection初始化为ArrayList:"+c);
a.add("a1");
a.add(0,"a2");//该方法只能在a的长度以内插入,否则抛出异常报错
System.out.println("添加元素"+a);
a.addAll(hs);//添加Collection元素
System.out.println("添加元素"+a);
a.addAll(0, hs);//在指定位置添加Collection元素
System.out.println("添加元素"+a);
//ArrayList<String> d = ArrayList<String>a.clone();
ArrayList<String> d = new ArrayList<String>(a);
d.clear();
System.out.println("clear后的结果:"+d);
System.out.println("是否包含某一个元素:"+a.contains("a"));
System.out.println("通过索引获取某一个元素:"+a.get(0));
System.out.println("元素首次出现的索引位置:"+a.indexOf("a"));
System.out.println("判断是否为空:"+a.isEmpty());
System.out.println("移除索引位置上的元素:"+a.remove(0));
System.out.println(a);
System.out.println("移除首次出现的元素:"+a.remove("a1"));//返回boolean
System.out.println(a);
//System.out.println("移除区间内元素:"+aremoveRange(0,2));
System.out.println("设置索引上的元素为指定元素"+a.set(0,"fg")+a);
String[] s = (String[])a.toArray(new String[a.size()]);
System.out.println(s);
ArrayList<String> e = new ArrayList<>(a.subList(0,3));
System.out.println("list截取:"+s);
// 循环迭代
for(String str: e){
System.out.print(str+" ");
}
System.out.println();
//Iterator 迭代
Iterator it = e.iterator();
while(it.hasNext()){
System.out.print(it.next()+" ");
}
System.out.println();
//forEach 迭代循环, java1.8中含有的
e.forEach(show->{
System.out.print(show+" ");
});
System.out.println();
ListIterator it1 = e.listIterator();
while(it1.hasNext()){
System.out.print(it1.next()+" ");
}
System.out.println();
e.replaceAll(x->x+"abc");//将e中的元素全部加上"abc"后缀,并替换原来的元素
e.forEach(show->{
System.out.print(show + " ");
});
System.out.println();
ArrayList<String> ay = new ArrayList<String>();
ay.add("fgabc");
ay.add("gh");
System.out.println("是否包含集合ay中的元素,只要含有一个就返回true"+e.retainAll(ay));
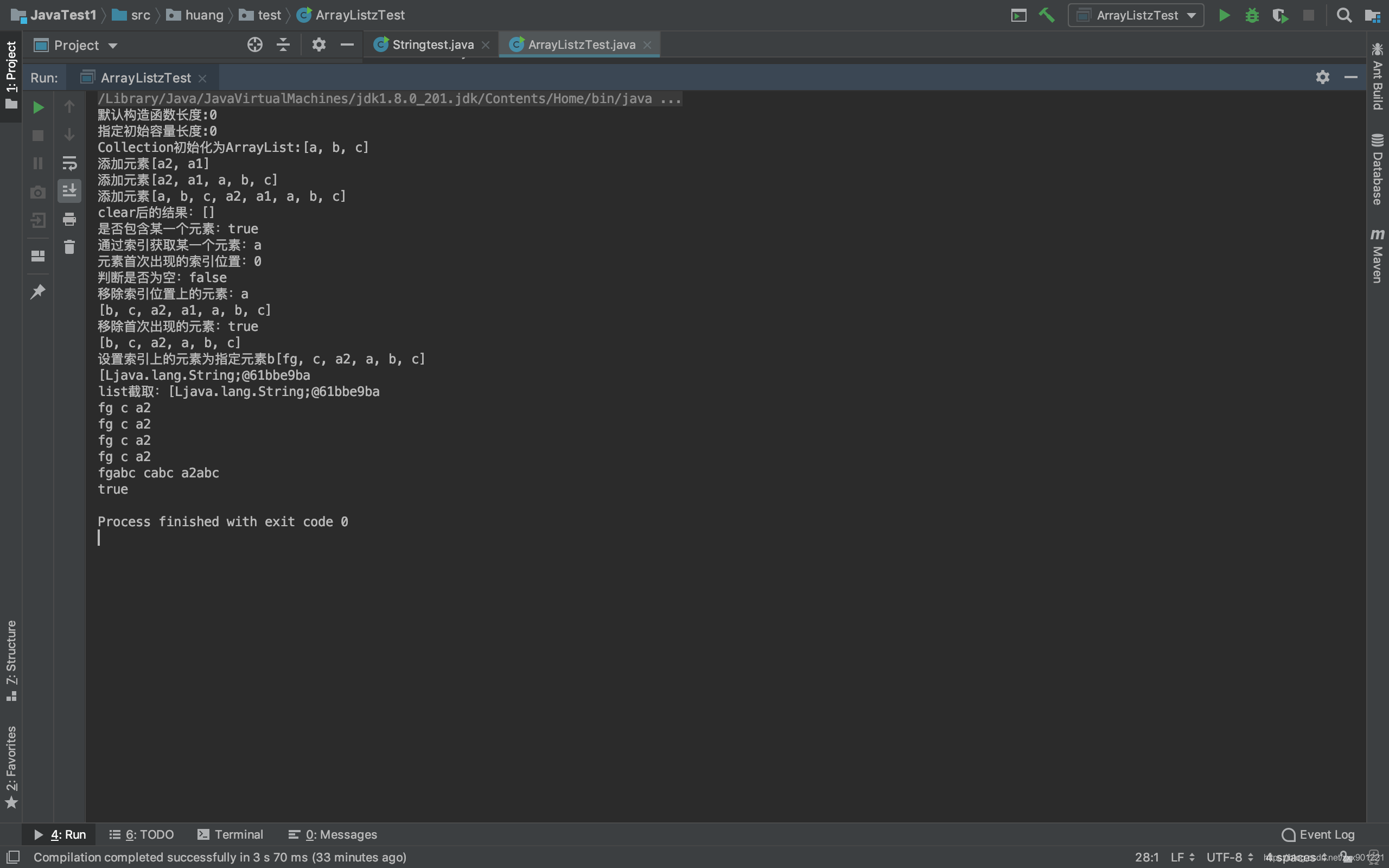
}