1.什么是图?
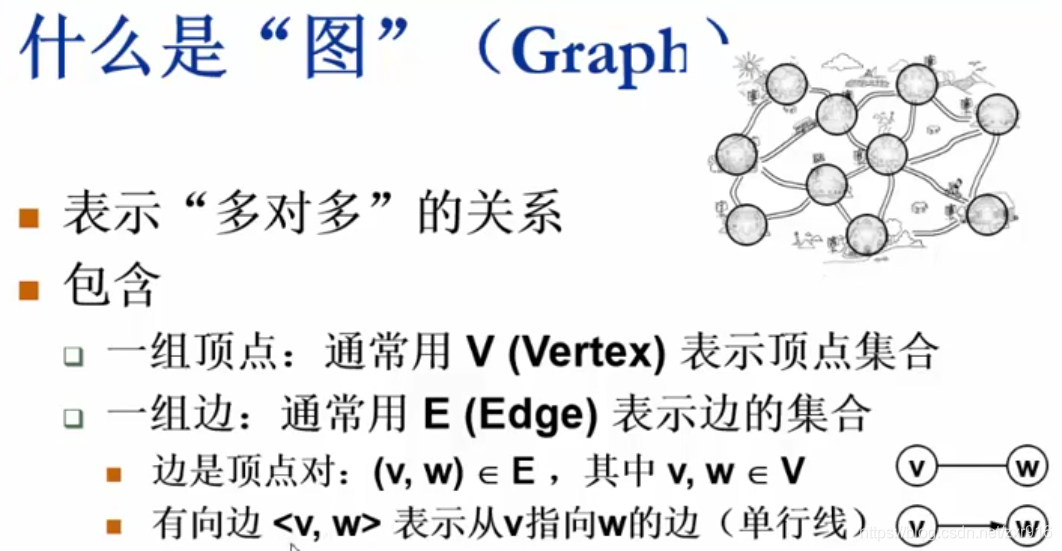
2.图的抽象数据结构
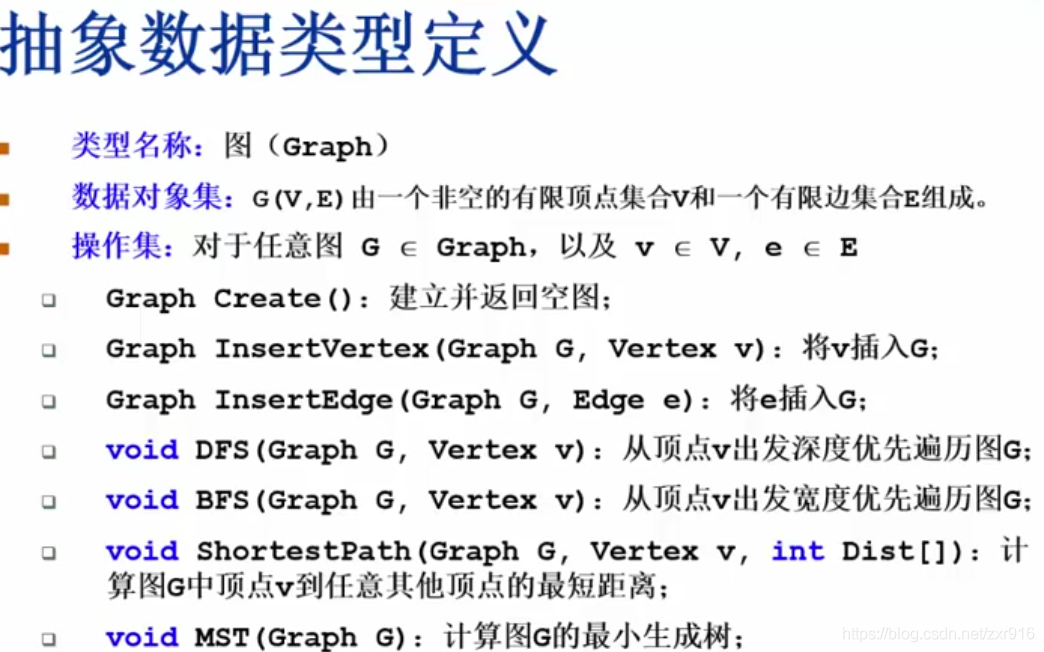
3.如何在程序中表示一个图?
3.1 邻接矩阵
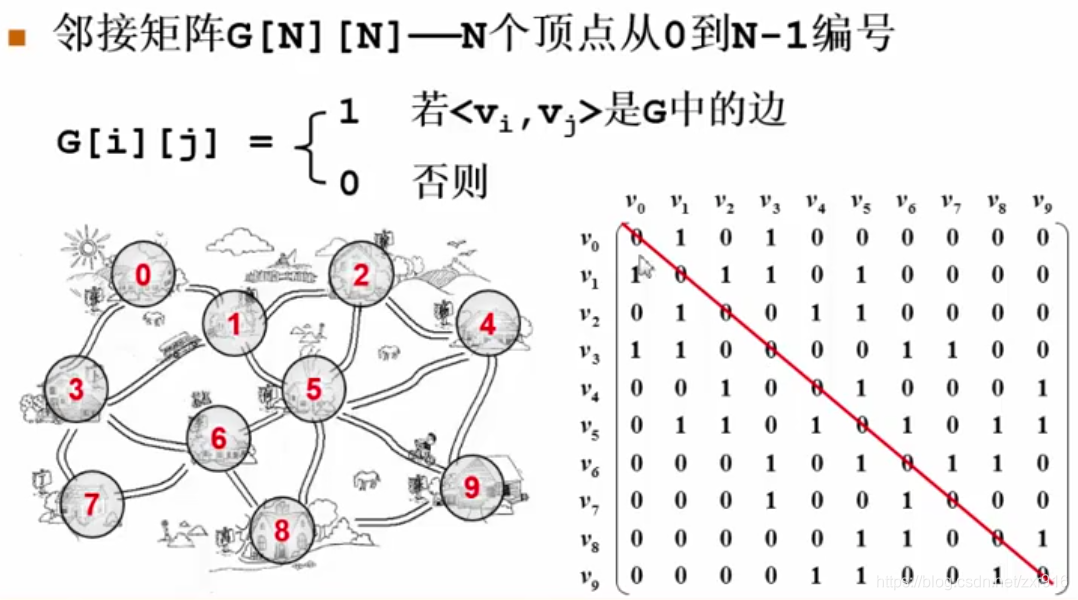
3.2 邻接表
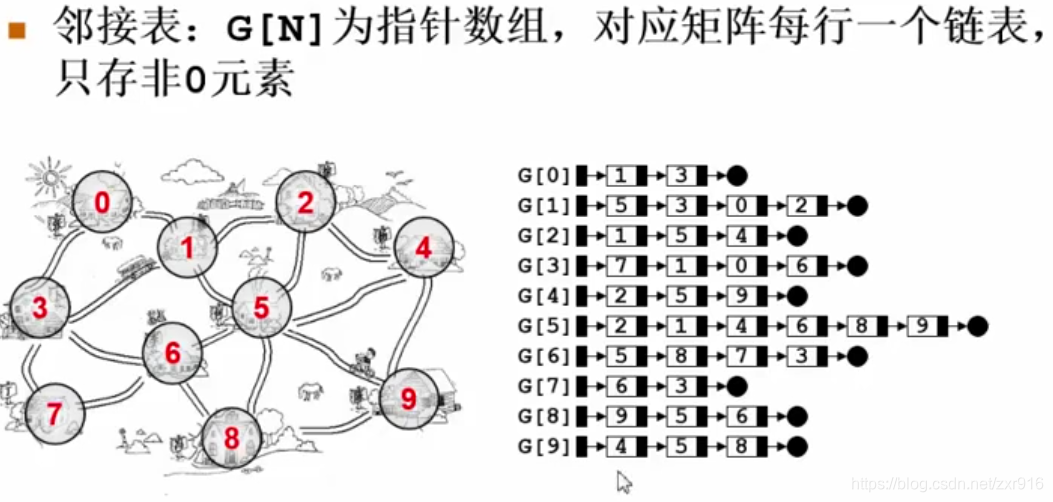
4.图的遍历
4.1 深度优先搜索
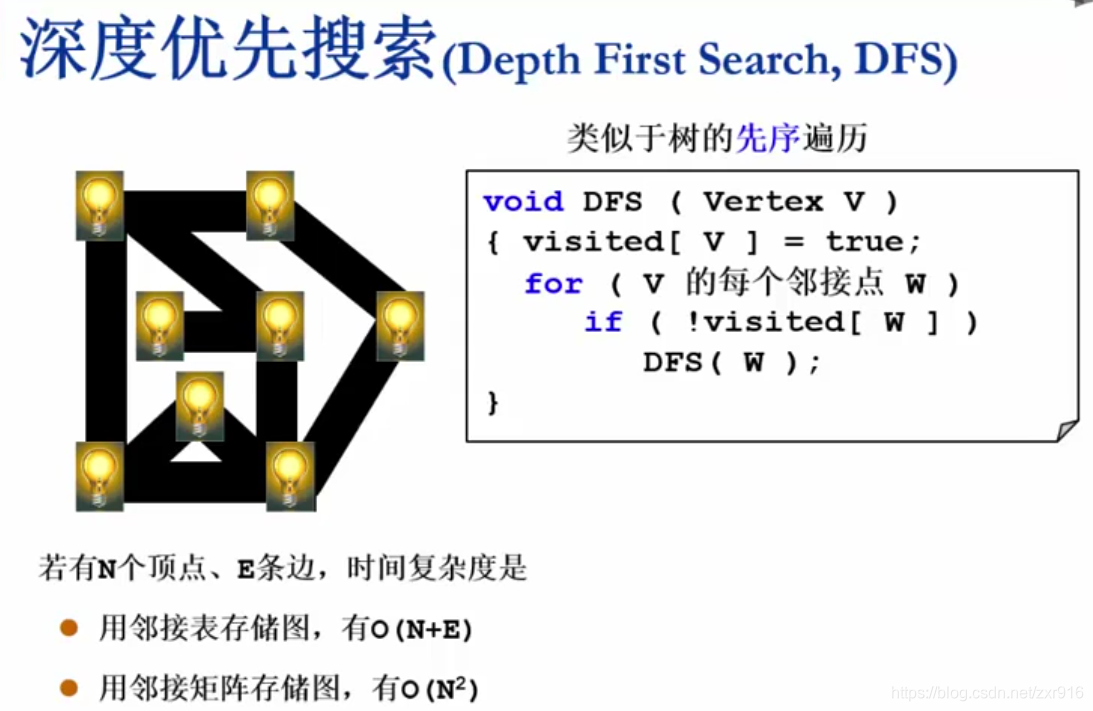
4.2 广度优先搜索
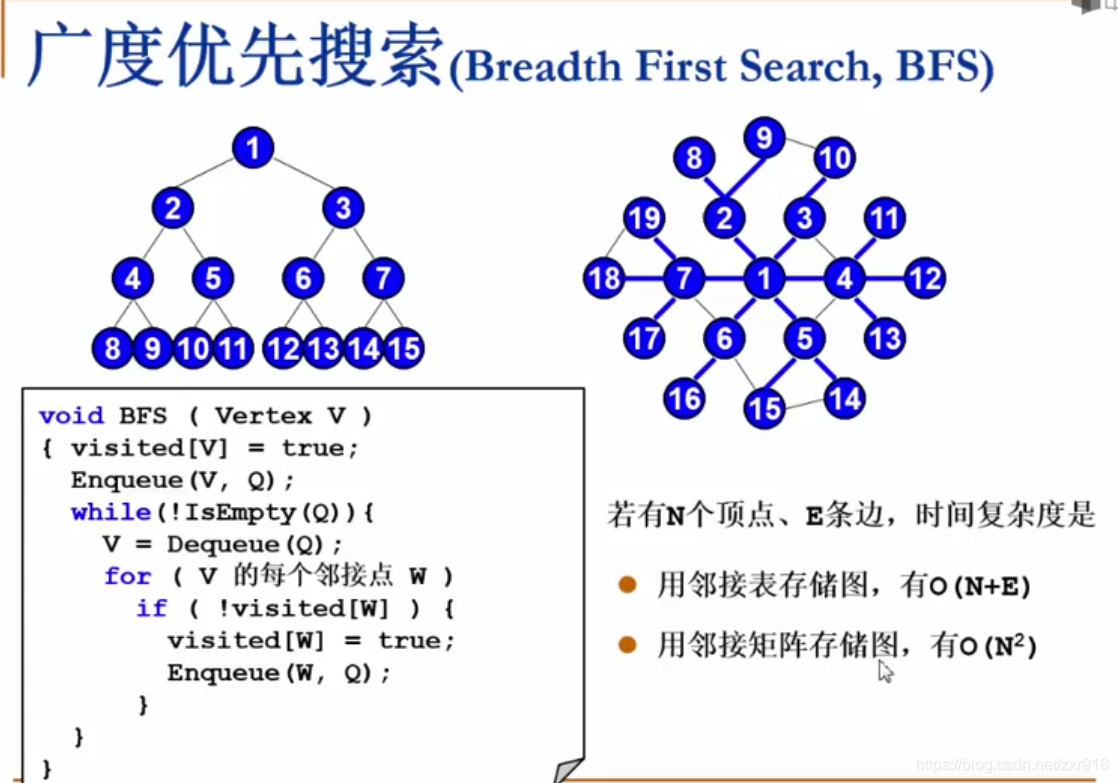
5.图的C语言实现
#include<stdio.h>
#include<stdlib.h>
#define WeightType int
#define MaxVertexNum 3
typedef struct GNode *PtrToGNode;
typedef PtrToGNode MGraph;
typedef int Vertex;
typedef struct ENode *PtrToENode;
typedef PtrToENode Edge;
struct GNode{
int Nv;
int Ne;
WeightType G[MaxVertexNum][MaxVertexNum];
};
struct ENode{
Vertex V1,V2;
WeightType Weight;
};
MGraph CreateGraph(int VertexNum)
{
Vertex V,W;
MGraph Graph;
Graph=(MGraph)malloc(sizeof(struct GNode));
Graph->Nv=VertexNum;
Graph->Ne=0;
for(V=0;V<Graph->Nv;V++){
for(W=0;W<Graph->Nv;W++){
Graph->G[V][W]=0;
}
}
return Graph;
}
void InsertEdge(MGraph Graph,Edge E)
{
Graph->G[E->V1][E->V2]=E->Weight;
Graph->G[E->V2][E->V1]=E->Weight;
}
int main()
{
int i,j;
MGraph Graph;
Edge E[3];
Graph=CreateGraph(3);
for(i=0;i<3;i++){
for(j=0;j<3;j++){
printf("G[%d][%d]=%d ",i,j,Graph->G[i][j]);
}
}
printf("\n");
for(i=0;i<3;i++){
E[i]=(Edge)malloc(sizeof(struct ENode));
}
E[0]->V1=0;
E[0]->V2=1;
E[0]->Weight=2;
E[1]->V1=1;
E[1]->V2=2;
E[1]->Weight=3;
E[2]->V1=0;
E[2]->V2=2;
E[2]->Weight=6;
for(i=0;i<3;i++){
InsertEdge(Graph,E[i]);
}
for(i=0;i<3;i++){
for(j=0;j<3;j++){
printf("G[%d][%d]=%d ",i,j,Graph->G[i][j]);
}
}
printf("\n");
return 0;
}