网页访问
- 网页是特殊的网络服务(HTTP,Hypertext Transfer Protocol)
– 在浏览器输入URL地址
– 浏览器将连接到远程服务器上(IP+80Port)
– 请求下载一个HTML文件下来,放在本地的临时文件夹中
– 在浏览器显示出来
HTTP
- 超文本传输协议(HyperText Transfer Protocol)
- 用于从万维网(www/World Wide Web)服务器传输超文本到本地浏览器的传输协议
- 资源文件采用HTML编写,以URL形式对外提供
HTML
- 超文本标记语言(HyperText Markup Language)
- 表单概念(form),网页上可以输入的地方
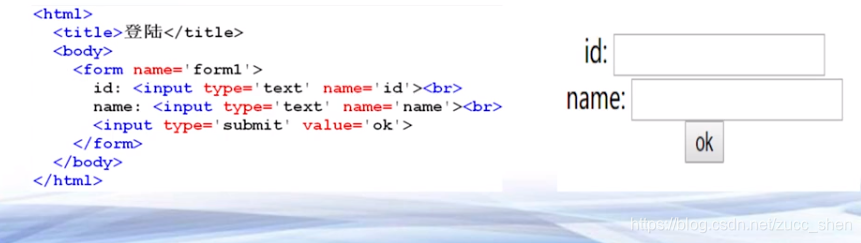
- 访问方式
– Get:从服务器获取资源到客户端
– Post:从客户端向服务器发送数据
– Put:上传文件
– Delete:删除文件
–Head:报文头部
–Options:询问支持的方法
–Trace:追踪路径
– Connect:用隧道协议连接代理
JAVA HTTP编程
- Java HTTP 编程(java.net包)
- 支持模拟成浏览器的方式去访问网页
- URL,代表一个资源
– http://www.zucc.edu.cn/index.html?a=1&b=2&c=3
–第一个代表什么协议、网站域名、目录资源文件名字、提供给资源文件的参数 - URLConnection
– 获取资源的连接器
– 根据URL的openConnection()方法获取URLConnection
–connet方法,建立和资源的联系通道
–getInputStream方法,获取资源的内容
public class URLConnectionGetTest
{
public static void main(String[] args)
{
try
{
String urlName = "http://www.baidu.com";
URL url = new URL(urlName);
URLConnection connection = url.openConnection();
connection.connect();
Map<String, List<String>> headers = connection.getHeaderFields();
for (Map.Entry<String, List<String>> entry : headers.entrySet())
{
String key = entry.getKey();
for (String value : entry.getValue())
System.out.println(key + ": " + value);
}
System.out.println("----------");
System.out.println("getContentType: " + connection.getContentType());
System.out.println("getContentLength: " + connection.getContentLength());
System.out.println("getContentEncoding: " + connection.getContentEncoding());
System.out.println("getDate: " + connection.getDate());
System.out.println("getExpiration: " + connection.getExpiration());
System.out.println("getLastModifed: " + connection.getLastModified());
System.out.println("----------");
BufferedReader br = new BufferedReader(new InputStreamReader(connection.getInputStream(), "UTF-8"));
String line = "";
while((line=br.readLine()) != null)
{
System.out.println(line);
}
br.close();
}
catch (IOException e)
{
e.printStackTrace();
}
}
}
public class URLConnectionPostTest
{
public static void main(String[] args) throws IOException
{
String urlString = "https://tools.usps.com/go/ZipLookupAction.action";
Object userAgent = "HTTPie/0.9.2";
Object redirects = "1";
CookieHandler.setDefault(new CookieManager(null, CookiePolicy.ACCEPT_ALL));
Map<String, String> params = new HashMap<String, String>();
params.put("tAddress", "1 Market Street");
params.put("tCity", "San Francisco");
params.put("sState", "CA");
String result = doPost(new URL(urlString), params,
userAgent == null ? null : userAgent.toString(),
redirects == null ? -1 : Integer.parseInt(redirects.toString()));
System.out.println(result);
}
public static String doPost(URL url, Map<String, String> nameValuePairs, String userAgent, int redirects)
throws IOException
{
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
if (userAgent != null)
connection.setRequestProperty("User-Agent", userAgent);
if (redirects >= 0)
connection.setInstanceFollowRedirects(false);
connection.setDoOutput(true);
try (PrintWriter out = new PrintWriter(connection.getOutputStream()))
{
boolean first = true;
for (Map.Entry<String, String> pair : nameValuePairs.entrySet())
{
if (first)
{
first = false;
}
else
{
out.print('&');
}
String name = pair.getKey();
String value = pair.getValue();
out.print(name);
out.print('=');
out.print(URLEncoder.encode(value, "UTF-8"));
}
}
String encoding = connection.getContentEncoding();
if (encoding == null)
{
encoding = "UTF-8";
}
if (redirects > 0)
{
int responseCode = connection.getResponseCode();
System.out.println("responseCode: " + responseCode);
if (responseCode == HttpURLConnection.HTTP_MOVED_PERM
|| responseCode == HttpURLConnection.HTTP_MOVED_TEMP
|| responseCode == HttpURLConnection.HTTP_SEE_OTHER)
{
String location = connection.getHeaderField("Location");
if (location != null)
{
URL base = connection.getURL();
connection.disconnect();
return doPost(new URL(base, location), nameValuePairs, userAgent, redirects - 1);
}
}
}
else if (redirects == 0)
{
throw new IOException("Too many redirects");
}
StringBuilder response = new StringBuilder();
try (Scanner in = new Scanner(connection.getInputStream(), encoding))
{
while (in.hasNextLine())
{
response.append(in.nextLine());
response.append("\n");
}
}
catch (IOException e)
{
InputStream err = connection.getErrorStream();
if (err == null) throw e;
try (Scanner in = new Scanner(err))
{
response.append(in.nextLine());
response.append("\n");
}
}
return response.toString();
}
}
HttpClient 的HTTP编程
- JDK HTTP Client (JDK自带,从9开始)
– JDK11正式发布
– java.net.http包
– 取代URLConnection
–支持HTTP1.1和HTTP/2
– 实现大部分HTTP方法
– 主要类 - HttpClient - HttpRequest(提交参数给服务器) - HttpResponse(服务器反馈给我的信息)
public static void doGet() {
try{
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder(URI.create("http://www.baidu.com")).build();
HttpResponse response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
}
catch(Exception e) {
e.printStackTrace();
}
}
}
public static void doPost() {
try {
HttpClient client = HttpClient.newBuilder().build();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://tools.usps.com/go/ZipLookupAction.action"))
.header("User-Agent", "HTTPie/0.9.2")
.header("Content-Type","application/x-www-form-urlencoded;charset=utf-8")
.POST(HttpRequest.BodyPublishers.ofString("tAddress="
+ URLEncoder.encode("1 Market Street", "UTF-8")
+ "&tCity=" + URLEncoder.encode("San Francisco", "UTF-8") + "&sState=CA"))
.build();
HttpResponse response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.statusCode());
System.out.println(response.headers());
System.out.println(response.body().toString());
}
catch(Exception e) {
e.printStackTrace();
}
}
- Apache HttpComponents 的HttpClient(Apache出品)
– 从HttpClien进化而来
– 是一个集成的Java HTTP 工具包
– 实现所有HTTO方法:get/post/put/delete
– 支持自动转向
–支持https协议
–支持代理服务器等
public class HttpComponentsGetTest {
public final static void main(String[] args) throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
RequestConfig requestConfig = RequestConfig.custom()
.setConnectTimeout(5000)
.setConnectionRequestTimeout(5000)
.setSocketTimeout(5000)
.setRedirectsEnabled(true)
.build();
HttpGet httpGet = new HttpGet("http://www.baidu.com");
httpGet.setConfig(requestConfig);
String srtResult = "";
try {
HttpResponse httpResponse = httpClient.execute(httpGet);
if(httpResponse.getStatusLine().getStatusCode() == 200){
srtResult = EntityUtils.toString(httpResponse.getEntity(), "UTF-8");
System.out.println(srtResult);
}else
{
}
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
httpClient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public class HttpComponentsPostTest {
public final static void main(String[] args) throws Exception {
CloseableHttpClient httpClient = HttpClientBuilder.create().setRedirectStrategy(new LaxRedirectStrategy()).build();
RequestConfig requestConfig = RequestConfig.custom().
setConnectTimeout(10000).setConnectionRequestTimeout(10000)
.setSocketTimeout(10000).setRedirectsEnabled(false).build();
HttpPost httpPost = new HttpPost("https://tools.usps.com/go/ZipLookupAction.action");
httpPost.setConfig(requestConfig);
List<BasicNameValuePair> list = new ArrayList<BasicNameValuePair>();
list.add(new BasicNameValuePair("tAddress", URLEncoder.encode("1 Market Street", "UTF-8")));
list.add(new BasicNameValuePair("tCity", URLEncoder.encode("San Francisco", "UTF-8")));
list.add(new BasicNameValuePair("sState", "CA"));
try {
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(list,"UTF-8");
httpPost.setEntity(entity);
httpPost.setHeader("User-Agent", "HTTPie/0.9.2");
HttpResponse httpResponse = httpClient.execute(httpPost);
String strResult = "";
if(httpResponse != null){
System.out.println(httpResponse.getStatusLine().getStatusCode());
if (httpResponse.getStatusLine().getStatusCode() == 200) {
strResult = EntityUtils.toString(httpResponse.getEntity());
}
else {
strResult = "Error Response: " + httpResponse.getStatusLine().toString();
}
}else{
}
System.out.println(strResult);
} catch (Exception e) {
e.printStackTrace();
}finally {
try {
if(httpClient != null){
httpClient.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}