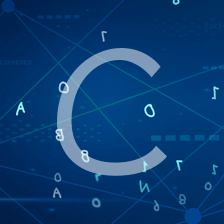
leetcode
zsjmfy
这个作者很懒,什么都没留下…
展开
-
105. Construct Binary Tree from Preorder and Inorder Traversal
Given preorder and inorder traversal of a tree, construct the binary tree. Note: You may assume that duplicates do not exist in the tree.解题思路:给出前序和中序遍历,重建二叉树,根据前序找到跟节点,然后将中序分为两部分,分别在两部分中递归找到左右孩子节点。非递原创 2016-12-14 14:29:54 · 200 阅读 · 0 评论 -
106. Construct Binary Tree from Inorder and Postorder Traversal
Given inorder and postorder traversal of a tree, construct the binary tree. Note: You may assume that duplicates do not exist in the tree.解题思路:利用后序和中序结果重建二叉树,思路和105那道题一样,既可以递归也可以非递归。 一刷ac 递归方法。/**原创 2016-12-14 15:40:27 · 182 阅读 · 0 评论 -
260. Single Number III
Given an array of numbers nums, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. For example: Given nums =原创 2016-12-18 21:30:49 · 208 阅读 · 0 评论 -
leetcode,79. Word Search
Given a 2D board and a word, find if the word exists in the grid. The word can be constructed from letters of sequentially adjacent cell, where “adjacent” cells are those horizontally or vertically ne原创 2016-12-27 19:46:51 · 184 阅读 · 0 评论 -
6. ZigZag Conversion
The string “PAYPALISHIRING” is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility) P A H N A P L S I I原创 2016-12-29 14:12:30 · 219 阅读 · 0 评论 -
9. Palindrome Number
Determine whether an integer is a palindrome. Do this without extra space.一刷ac 解题思路:数学题,要求不适用额外空间,可以将x切分为两部分比较是否相同或者相差一位。或者每次对比首尾两个数字。public class Solution { public boolean isPalindrome(int x) {原创 2016-12-29 14:56:59 · 215 阅读 · 0 评论 -
8. String to Integer (atoi)
Implement atoi to convert a string to an integer.public class Solution { public int myAtoi(String str) { str = str.trim(); if(str == null || str.length() == 0) return 0; int原创 2016-12-29 15:13:01 · 185 阅读 · 0 评论 -
62. Unique Paths
A robot is located at the top-left corner of a m x n grid (marked ‘Start’ in the diagram below). The robot can only move either down or right at any point in time. The robot is trying to reach the bot原创 2016-12-29 22:11:51 · 155 阅读 · 0 评论 -
17. Letter Combinations of a Phone Number
Given a digit string, return all possible letter combinations that the number could represent. A mapping of digit to letters (just like on the telephone buttons) is given below. Input:Digit string “2原创 2016-12-30 16:03:08 · 252 阅读 · 0 评论 -
18. 4Sum
Given an array S of n integers, are there elements a, b, c, and d in S such that a + b + c + d = target? Find all unique quadruplets in the array which gives the sum of target. Note: The solution set原创 2016-12-30 17:05:21 · 183 阅读 · 0 评论 -
24. Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head. For example, Given 1->2->3->4, you should return the list as 2->1->4->3. Your algorithm should use only constant space. You ma原创 2016-12-30 18:36:56 · 177 阅读 · 0 评论 -
25. Reverse Nodes in k-Group
Given a linked list, reverse the nodes of a linked list k at a time and return its modified list. If the number of nodes is not a multiple of k then left-out nodes in the end should remain as it is.原创 2016-12-31 00:36:28 · 176 阅读 · 0 评论 -
28. Implement strStr()
Implement strStr(). Returns the index of the first occurrence of needle in haystack, or -1 if needle is not part of haystack.一刷没ac 解题思路:kmppublic class Solution { public int strStr(String haystac原创 2016-12-31 10:38:42 · 200 阅读 · 0 评论 -
30. Substring with Concatenation of All Words
You are given a string, s, and a list of words, words, that are all of the same length. Find all starting indices of substring(s) in s that is a concatenation of each word in words exactly once and wit原创 2016-12-31 19:03:31 · 173 阅读 · 0 评论 -
76. Minimum Window Substring
Given a string S and a string T, find the minimum window in S which will contain all the characters in T in complexity O(n). For example, S = “ADOBECODEBANC” T = “ABC” Minimum window is “BANC”. No原创 2016-12-31 19:05:08 · 198 阅读 · 0 评论 -
31. Next Permutation
Implement next permutation, which rearranges numbers into the lexicographically next greater permutation of numbers. If such arrangement is not possible, it must rearrange it as the lowest possible or原创 2016-12-31 20:59:38 · 216 阅读 · 0 评论 -
34. Search for a Range
Given a sorted array of integers, find the starting and ending position of a given target value. Your algorithm’s runtime complexity must be in the order of O(log n). If the target is not found in th原创 2016-12-31 21:27:36 · 184 阅读 · 0 评论 -
39. Combination Sum
Given a set of candidate numbers (C) (without duplicates) and a target number (T), find all unique combinations in C where the candidate numbers sums to T. The same repeated number may be chosen from原创 2016-12-31 22:20:22 · 191 阅读 · 0 评论 -
41. First Missing Positive
Given an unsorted integer array, find the first missing positive integer. For example, Given [1,2,0] return 3, and [3,4,-1,1] return 2. Your algorithm should run in O(n) time and uses constant spac原创 2017-01-01 09:59:23 · 199 阅读 · 0 评论 -
46. Permutations
Given a collection of distinct numbers, return all possible permutations. For example, [1,2,3] have the following permutations: [ [1,2,3], [1,3,2], [2,1,3], [2,3,1], [3,1,2], [3,2,原创 2017-01-01 10:54:43 · 227 阅读 · 0 评论 -
47. Permutations II
Given a collection of numbers that might contain duplicates, return all possible unique permutations. For example, [1,1,2] have the following unique permutations: [ [1,1,2], [1,2,1], [2,1,1原创 2017-01-01 11:16:02 · 177 阅读 · 0 评论 -
48. Rotate Image
You are given an n x n 2D matrix representing an image. Rotate the image by 90 degrees (clockwise). Follow up: Could you do this in-place?一刷没ac 解题思路:反转两次。public class Solution { public void rot原创 2017-01-01 11:40:14 · 176 阅读 · 0 评论 -
49. Group Anagrams
Given an array of strings, group anagrams together. For example, given: [“eat”, “tea”, “tan”, “ate”, “nat”, “bat”], Return: [ [“ate”, “eat”,”tea”], [“nat”,”tan”], [“bat”] ]public class So原创 2017-01-01 12:56:06 · 230 阅读 · 0 评论 -
100. Same Tree
Given two binary trees, write a function to check if they are equal or not. Two binary trees are considered equal if they are structurally identical and the nodes have the same value.一刷ac 递归/** * De原创 2017-01-01 13:31:49 · 158 阅读 · 0 评论 -
88. Merge Sorted Array
Given two sorted integer arrays nums1 and nums2, merge nums2 into nums1 as one sorted array. Note: You may assume that nums1 has enough space (size that is greater or equal to m + n) to hold addition原创 2017-01-01 13:38:16 · 196 阅读 · 0 评论 -
83. Remove Duplicates from Sorted List
Given a sorted linked list, delete all duplicates such that each element appear only once. For example, Given 1->1->2, return 1->2. Given 1->1->2->3->3, return 1->2->3./** * Definition for singly-l原创 2017-01-01 13:51:03 · 204 阅读 · 0 评论 -
55. Jump Game
Given an array of non-negative integers, you are initially positioned at the first index of the array. Each element in the array represents your maximum jump length at that position. Determine if you原创 2017-01-01 14:54:33 · 165 阅读 · 0 评论 -
59. Spiral Matrix II
Given an integer n, generate a square matrix filled with elements from 1 to n2 in spiral order. For example, Given n = 3, You should return the following matrix: [ [ 1, 2, 3 ], [ 8, 9, 4 ], [原创 2017-01-01 16:01:40 · 181 阅读 · 0 评论 -
60. Permutation Sequence
The set [1,2,3,…,n] contains a total of n! unique permutations. By listing and labeling all of the permutations in order, We get the following sequence (ie, for n = 3): “123” “132” “213” “231” “原创 2017-01-01 16:43:13 · 167 阅读 · 0 评论 -
61. Rotate List
Given a list, rotate the list to the right by k places, where k is non-negative. For example: Given 1->2->3->4->5->NULL and k = 2, return 4->5->1->2->3->NULL./** * Definition for singly-linked list原创 2017-01-01 17:21:48 · 162 阅读 · 0 评论 -
187. Repeated DNA Sequences
All DNA is composed of a series of nucleotides abbreviated as A, C, G, and T, for example: “ACGAATTCCG”. When studying DNA, it is sometimes useful to identify repeated sequences within the DNA. Write原创 2017-02-07 14:17:11 · 150 阅读 · 0 评论 -
188. Best Time to Buy and Sell Stock IV
Say you have an array for which the ith element is the price of a given stock on day i. Design an algorithm to find the maximum profit. You may complete at most k transactions. Note: You may not eng原创 2017-02-07 15:59:23 · 229 阅读 · 0 评论 -
287. Find the Duplicate Number
Given an array nums containing n + 1 integers where each integer is between 1 and n (inclusive), prove that at least one duplicate number must exist. Assume that there is only one duplicate number, fin原创 2017-02-27 23:44:06 · 156 阅读 · 0 评论 -
289. Game of Life
According to the Wikipedia’s article: “The Game of Life, also known simply as Life, is a cellular automaton devised by the British mathematician John Horton Conway in 1970.”Given a board with m by n ce原创 2017-02-28 00:09:01 · 192 阅读 · 0 评论 -
290. Word Pattern
Given a pattern and a string str, find if str follows the same pattern.Here follow means a full match, such that there is a bijection between a letter in pattern and a non-empty word in str.Examples:原创 2017-02-28 00:24:15 · 160 阅读 · 0 评论 -
207. Course Schedule
There are a total of n courses you have to take, labeled from 0 to n - 1. Some courses may have prerequisites, for example to take course 0 you have to first take course 1, which is expressed as a pai原创 2017-02-18 20:46:24 · 171 阅读 · 0 评论 -
210. Course Schedule II
There are a total of n courses you have to take, labeled from 0 to n - 1. Some courses may have prerequisites, for example to take course 0 you have to first take course 1, which is expressed as a pai原创 2017-02-18 21:11:49 · 185 阅读 · 0 评论 -
300. Longest Increasing Subsequence
Given an unsorted array of integers, find the length of longest increasing subsequence.For example, Given [10, 9, 2, 5, 3, 7, 101, 18], The longest increasing subsequence is [2, 3, 7, 101], therefore原创 2017-02-28 10:39:13 · 163 阅读 · 0 评论 -
209. Minimum Size Subarray Sum
Given an array of n positive integers and a positive integer s, find the minimal length of a contiguous subarray of which the sum ≥ s. If there isn’t one, return 0 instead. For example, given the arra原创 2017-02-19 20:12:49 · 160 阅读 · 0 评论 -
208. Implement Trie (Prefix Tree)
Implement a trie with insert, search, and startsWith methods. Note: You may assume that all inputs are consist of lowercase letters a-z.class TrieNode { boolean isWord = false; TrieNode[] chi原创 2017-02-19 21:17:56 · 211 阅读 · 0 评论