1、停止动画
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button onclick="stopAnimate()">停止动画</button>
<script>
let num = 0;
let timer = null;
function frame() {
num++;
if (num > 100) {
return;
}
console.log(num)
timer = requestAnimationFrame(frame);
}
frame();
function stopAnimate() {
cancelAnimationFrame(timer);
}
</script>
</body>
</html>
2、执行动画
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box {
width: 100px;
height: 100px;
position: absolute;
background-color: #ff2d51;
}
</style>
</head>
<body>
<button onclick="startAnimate()">Start-动画</button>
<button onclick="stopAnimate()">End-动画</button>
<div id="box"></div>
<script>
let box = document.getElementById("box");
let sum = 500;
let start = 0;
let timer = null;
function frame() {
start++;
if (start > 500) {
return;
}
box.style.left = start + "px"
timer = requestAnimationFrame(frame)
console.log(timer);
}
function startAnimate() {
frame();
}
function stopAnimate() {
cancelAnimationFrame(timer)
}
</script>
</body>
</html>
3、改造动画
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<!-- 1、导入openlayers的依赖 -->
<link rel="stylesheet" href="https://lib.baomitu.com/ol3/4.6.5/ol.css">
<script src="https://lib.baomitu.com/ol3/4.6.5/ol.js"></script>
<script src="./utils/index.js"></script>
<script src="https://lib.baomitu.com/Turf.js/latest/turf.min.js"></script>
<style>
* {
margin: 0;
padding: 0;
}
#map {
width: 100vw;
height: 100vh;
}
</style>
</head>
<body>
<!-- 2、设置地图容器的挂载点 -->
<div id="map">
</div>
<script>
const gaode = new ol.layer.Tile({
title: "高德地图",
source: new ol.source.XYZ({
url: 'http://wprd0{1-4}.is.autonavi.com/appmaptile?lang=zh_cn&size=1&style=7&x={x}&y={y}&z={z}',
wrapX: false
})
});
const map = new ol.Map({
target: "map",
layers: [gaode],
view: new ol.View({
center: [114.30, 30.50],
zoom: 12,
projection: "EPSG:4326"
})
})
let data = {
"type": "Feature",
"properties": {},
"geometry": {
"type": "LineString",
"coordinates": [
[114.302214, 30.532323],
[114.362718, 30.529691]
]
}
}
let marker = new ol.Feature({
geometry: new ol.geom.Point([114.302214, 30.532323])
})
const marker_layer = new ol.layer.Vector({
source: new ol.source.Vector({
features: [marker]
}),
style: setMarkerStyle("./images/定位.png")
})
map.addLayer(marker_layer)
let feature = new ol.format.GeoJSON().readFeature(data);
const source = new ol.source.Vector({
features: [feature]
})
const layer = new ol.layer.Vector({
source,
style: setLineStyle({
color: "#ff2d5180",
width: 8
})
})
map.addLayer(layer);
let sum = 200;
let distance = turf.lineDistance(data);
let path = turf.along(data, distance / sum)
let line_path = turf.lineString([[114.302214, 30.532323], path.geometry.coordinates])
const feature_animate = new ol.format.GeoJSON().readFeature(line_path);
const layer_animate = new ol.layer.Vector({
source: new ol.source.Vector({
features: [feature_animate]
}),
style: setLineStyle({
width: 4
})
})
map.addLayer(layer_animate)
let start = 1;
function frame() {
start++;
if (start > sum) {
start = 0;
feature_animate.getGeometry().setCoordinates([])
return;
}
let along_path = turf.along(data, distance * (start / sum));
feature_animate.getGeometry().appendCoordinate(along_path.geometry.coordinates);
marker.getGeometry().setCoordinates(along_path.geometry.coordinates)
requestAnimationFrame(frame)
}
map.on("click", () => {
frame();
})
</script>
</body>
</html>
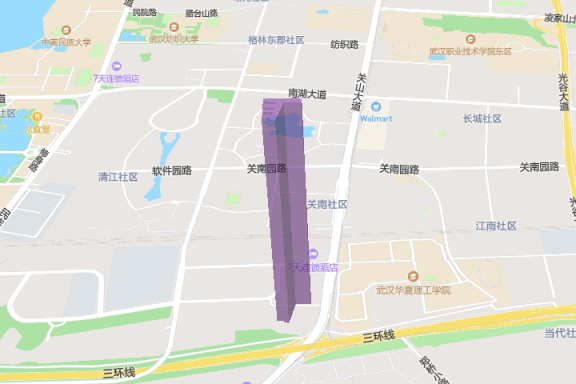