文章目录
一. 开发环境搭建
1. 安装 mingw-w64 编译器 (GCC for Windows 64 & 32 bits)、Cmake 工具(可选)
- mingw-w64 下载链接
- Cmake 下载链接
- 解压到自己想要的目录下后,添加系统环境变量
- 测试环境变量是否设置成功,cmd 中分别输入
g++ --verison
以及cmake --version
是否正确输出版本信息
2. vscode 插件安装
- C/C++
- cmake(可选)
- cmake tools(可选)
二. 简单编译示例
1. 在终端执行 g++ 命令 生成可执行文件
-
g++ 编译单文件,生成带调试信息的可执行文件
g++ -g main.cpp -o single_file_exec
main.cpp
单文件#include <iostream> using namespace std; int add(int a, int b) { return (a + b); } int main() { cout << "Hello world" << endl; return 0; }
-
g++ 编译多文件,生成带调试信息的可执行文件
g++ -g main.cpp add.cpp -o multi_file_exec
main.cpp
#include <iostream> int add(int a, int b); using namespace std; int main() { int a = 5; int b = 10; cout << "a + b = " << add(a, b) << endl; return 0; }
add.cpp
int add(int a, int b) { return (a + b); }
2. 使用 make 生成可执行文件 (多文件)
-
当前目录结构如下:
│ Makefile │ ├───include │ add.h │ └───src add.cpp main.cpp
main.cpp
#include <iostream> #include "add.h" using namespace std; int main() { int a = 5; int b = 10; cout << "a + b = " << add(a, b) << endl; return 0; }
add.cpp
#include "add.h" int add(int a, int b) { return (a + b); }
add.cpp
#ifndef ADD_H #define ADD_H int add(int a, int b); #endif
Makefile
cpp_src := src/main.cpp src/add.cpp include_path := include debug : @echo $(cpp_src) exec : $(cpp_src) @g++ -g $^ -o $@ -I$(include_path) clean : @del \Q exec .PHONY : debug exec
-
在当前目录的终端下执行以下命令生成可执行文件:
mingw32-make.exe exec
-
当前目录结构如下:
│ exec.exe │ Makefile │ ├───include │ add.h │ └───src add.cpp main.cpp
3. 用 CMake 生成可执行文件
-
当前目录结构如下:
│ CMakeLists.txt │ ├───include │ add.h │ └───src add.cpp main.cpp
CMakeLists.txt
project(MYADD) # 项目名称 include_directories (include) # 头文件目录路径 add_executable(exec src/main.cpp src/add.cpp)
-
vscode命令栏中执行CMake: Configure (第一次需选择Kit for cmake,选择本地安装的GCC mingw即可)生成build目录以及其内的文件
这一步其实相当于以下命令
mkdir build cd build cmake .. # 如果电脑本身装了 VS,第一次可能需运行 cmake -G "MingW Makefiles" ..
当前目录结构如下:
├─build │ ├─.cmake │ │ └─api │ │ └─v1 │ │ ├─query │ │ │ └─client-vscode │ │ └─reply │ ├─CMakeFiles │ │ ├─3.26.1 │ │ │ ├─CompilerIdC │ │ │ │ └─tmp │ │ │ └─CompilerIdCXX │ │ │ └─tmp │ │ ├─CMakeScratch │ │ ├─objs │ │ │ └─exec.dir │ │ │ └─src │ │ └─pkgRedirects │ └─objs ├─include └─src
-
在build目录的终端下执行以下命令生成可执行文件(在 build 里):
mingw32-make.exe
4. 使用 vscode 中的 C/C++插件 生成可执行文件并 debug (推荐)
-
单个文件
当前目录结构如下:
└───src # 用于存放源代码 main.cpp
-
选择 main.cpp 文件,左侧工具栏 Run and Debug -> create a launch.json file;
-
选择 C++(GDB/LLDB);
-
选择 g++.exe;
-
在.vscode 中生成配置文件
launch.json
tasks.json
;tasks.json
用于生成可执行文件,本质也是用 g++命令生成可执行文件launch.json
用于 debug 可执行文件
此时目录结构如下:
├───.vscode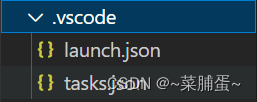 │ launch.json │ tasks.json └───src main.cpp main.exe
-
此时就可以使用
launch.json
文件进行 debug 了
-
-
多个文件
当前目录结构如下:
├───include # 头文件目录 │ add.h │ ├───objs # 目标文件目录 └───src # 源文件目录 add.cpp main.cpp
-
按上述单个文件的步骤在.vscode 中生成配置文件
launch.json
tasks.json
,会报错,需要根据具体情况修改这两个配置文件; -
按需修改配置文件
launch.json
{ // Use IntelliSense to learn about possible attributes. // Hover to view descriptions of existing attributes. // For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387 "version": "0.2.0", "configurations": [ { "name": "g++.exe - 生成和调试活动文件", "type": "cppdbg", "request": "launch", // "program": "${fileDirname}\\${fileBasenameNoExtension}.exe", "program": "${fileDirname}\\..\\objs\\${fileBasenameNoExtension}.exe", // modified 指定要debug的可执行文件的路径 "args": [], "stopAtEntry": false, "cwd": "C:\\Program Files (x86)\\mingw64\\bin", "environment": [], "console": "externalTerminal", "MIMode": "gdb", "miDebuggerPath": "C:\\Program Files (x86)\\mingw64\\bin\\gdb.exe", "setupCommands": [ { "description": "为 gdb 启用整齐打印", "text": "-enable-pretty-printing", "ignoreFailures": true } ], "preLaunchTask": "C/C++: g++.exe 生成活动文件" // 调用tasks.json,生成可执行文件,如果采用上述的三个方法生成可执行文件,则可把这句注释掉,然后debug } ] }
tasks.json
{ "tasks": [ { "type": "cppbuild", "label": "C/C++: g++.exe 生成活动文件", "command": "C:\\Program Files (x86)\\mingw64\\bin\\g++.exe", "args": [ "-g", // "${file}", "${fileDirname}\\*.cpp", // modified "-o", // "${fileDirname}\\${fileBasenameNoExtension}.exe", "${fileDirname}\\..\\objs\\${fileBasenameNoExtension}.exe", // modified "-I${fileDirname}\\..\\include" // modified ], "options": { "cwd": "C:\\Program Files (x86)\\mingw64\\bin" }, "problemMatcher": ["$gcc"], "group": { "kind": "build", "isDefault": true }, "detail": "调试器生成的任务。" } ], "version": "2.0.0" }
-
此时可以正常使用
launch.json
文件进行 debug
当前目录结构如下:
├───.vscode │ launch.json │ tasks.json │ ├───include │ add.h │ ├───objs │ main.exe │ └───src add.cpp main.cpp
-