1. MATLAB 数据(变量)
1.1 类型
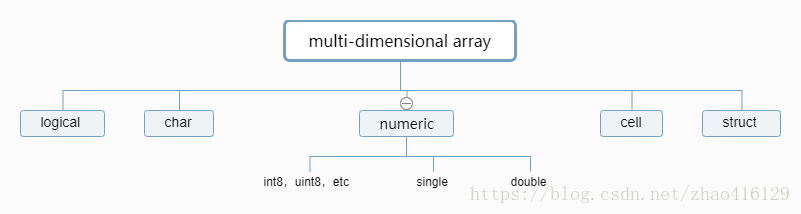
1.2 变量(数据)类型的转换
% 函数列表:
double( )
single( )
int8( ) int16( ) int32( ) int64( )
uint8( ) uint16( ) uint32( ) uint64( )
EX:
>> A=20
A =
20
>> B=int8(A)
B =
20
>> whos
Name Size Bytes Class Attributes
A 1x1 8 double
B 1x1 1 int8
ans 1x3 24 double
1.3 字符或字符串 < char or string>
1.3.1 字符
- 字符在 ASCII 中用 0 到 255 之间的数字代码表示
>> s1 = 'h'
whos
uint16(s1)
s1 =
h
Name Size Bytes Class Attributes
s1 1x1 2 char
ans =
104
- ASCII对照表:
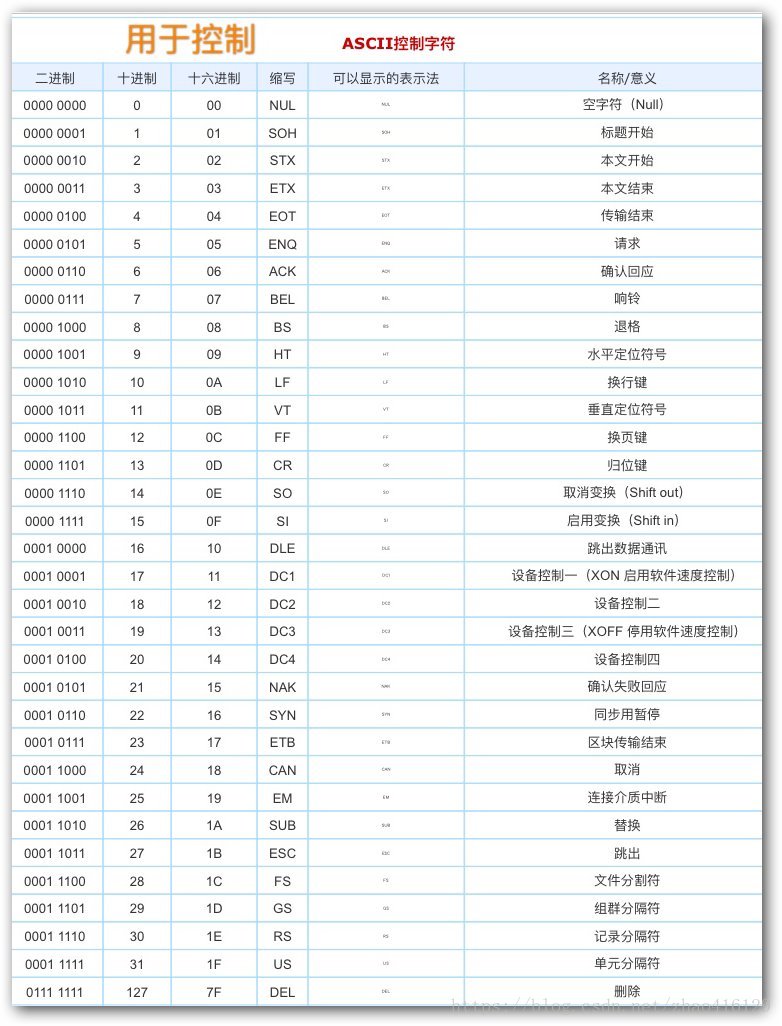
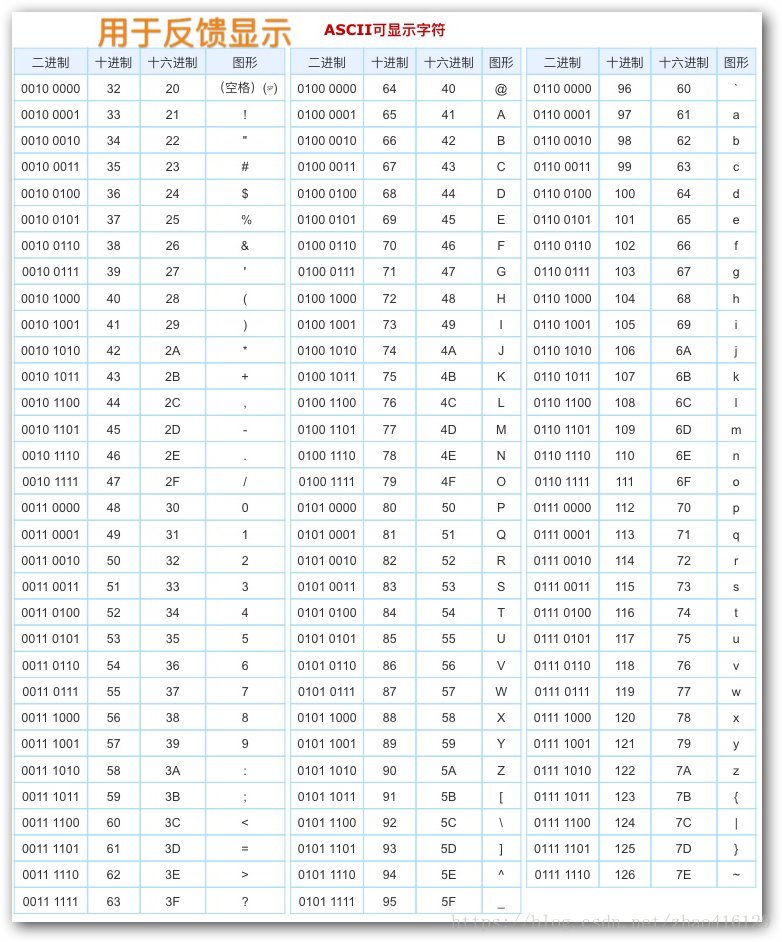
1.3.2 字符串
>> s1 = 'Example';
s2 = 'String';
>> s3 = [s1 s2]
s3 =
ExampleString
>> s4 = [s1; s2]
错误使用 vertcat % 此处s1与s2字母数量不一致,故报错
串联的矩阵的维度不一致。
>> str = 'aardvark';
'a' == str
ans =
1 1 0 0 0 1 0 0
>> str(str == 'a') = 'Z'
str =
ZZrdvZrk
1.3.3 练习
1.4 Structure
1.4.1 EX:
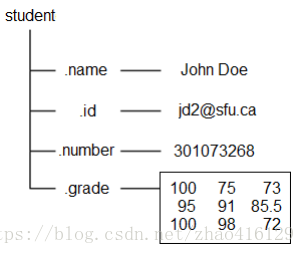
>> student.name = 'John Doe';
student.id = 'jdo2@sfu.ca';
student.number = 301073268;
student.grade = [100, 75, 73; ...
95, 91, 85.5; ...
100, 98, 72];
student
student =
name: 'John Doe'
id: 'jdo2@sfu.ca'
number: 301073268
grade: [3x3 double]
student(2).name = 'Ann Lane';
student(2).id = 'aln4@sfu.ca';
student(2).number = 301078853;
student(2).grade = [95 100 90; 95 82 97; 100 85 100];
1.4.2 结构函数
函数名 | 功能 |
---|
cell2struct | |
fieldnames | |
getfield | |
isfield | |
isstruct | |
orderfields | |
rmfield | |
setfield | |
struct | |
struct2cell | |
structfun | |
1.4.3 嵌套结构
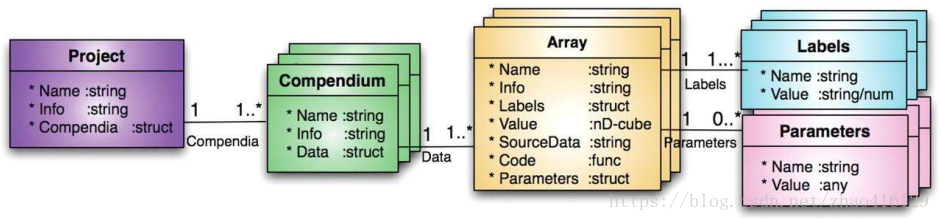
>> A = struct('data', [3 4 7; 8 0 1], 'nest', ...
struct('testnum', 'Test 1', ...
'xdata', [4 2 8],'ydata', [7 1 6]));
A(2).data = [9 3 2; 7 6 5];
A(2).nest.testnum = 'Test 2';
A(2).nest.xdata = [3 4 2];
A(2).nest.ydata = [5 0 9];
A.nest
ans =
testnum: 'Test 1'
xdata: [4 2 8]
ydata: [7 1 6]
ans =
testnum: 'Test 2'
xdata: [3 4 2]
ydata: [5 0 9]
1.5 元胞数组 Cell Array
1.5.1 元胞数组
- 与矩阵类似,但每个条目包含不同类型的数据;
- 使用
{}
来声明;
>> A(1,1)={[1 4 3; 0 5 8; 7 2 9]};
A(1,2)={'Anne Smith'};
A(2,1)={3+7i};
A(2,2)={-pi:pi:pi};
>> A
A =
[3x3 double] 'Anne Smith'
[3.0000 + 7.0000i] [1x3 double]
或者:
A{1,1}=[1 4 3; 0 5 8; 7 2 9];
A{1,2}='Anne Smith';
A{2,1}=3+7i;
A{2,2}=-pi:pi:pi;
1.5.2 访问元胞数组
>> A{1,1}
ans =
1 4 3
0 5 8
7 2 9
>> A{1,1}(1,1)
ans =
1
>>A{1,1}(2,2)
ans =
5
1.5.3 元胞数组函数
函数 | 功能 |
---|
cell | |
cell2mat | |
cell2struct | |
celldisp | |
cellfun | |
cellplot | |
cellstr | |
iscell | |
mat2cell | |
num2cell | |
struct2cell | |
EX:num2cell()
and mat2cell()
>> a = magic(3)
b = num2cell(a)
c = mat2cell(a, [1 1 1], 3)
a =
8 1 6
3 5 7
4 9 2
b =
[8] [1] [6]
[3] [5] [7]
[4] [9] [2]
c =
[1x3 double]
[1x3 double]
[1x3 double]
1.6 多维数组 Multi-dimensional Array
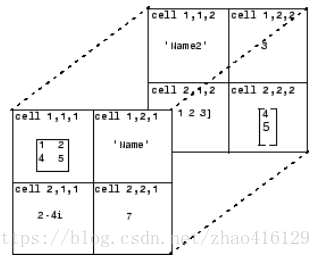
1.6.1 方法一:
>>A{1,1,1} = [1 2;4 5];
A{1,2,1} = 'Name';
A{2,1,1} = 2-4i;
A{2,1,1} = 7;
A{1,1,2} = 'Name2';
A{1,2,2} = 3;
A{2,1,2} = 0:1:3;
A{2,2,2} = [4 5]';
1.6.2 方法二:cat()
-
数组连接:
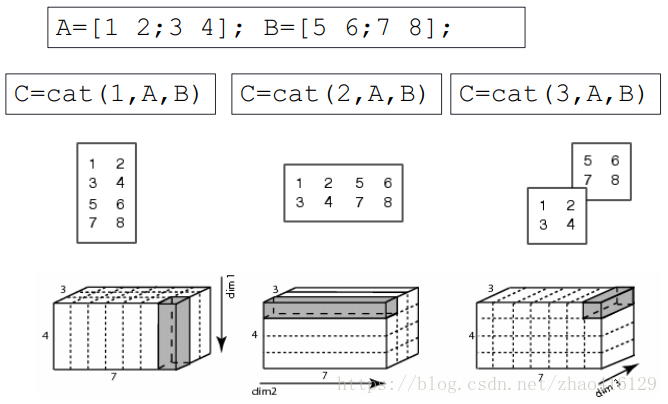
-
多维数组:
A{1,1} = [1 2;4 5];
A{1,2} = 'Name';
A{2,1} = 2-4i;
A{2,2} = 7;
B{1,1} = 'Name2';
B{1,2} = 3;
B{2,1} = 0:1:3;
B{2,2} = [4 5]';
C = cat(3, A, B)
1.6.3 reshape()
>> A = {'James Bond', [1 2;3 4;5 6]; pi, magic(5)}
C = reshape(A,1,4)
A =
'James Bond' [3x2 double]
[ 3.1416] [5x5 double]
C =
'James Bond' [3.1416] [3x2 double] [5x5 double]
1.7 检查变量和变量状态
函数 | 功能 |
---|
isinteger | |
islogical | |
isnan | |
isnumeric | |
isprime | |
isreal | |
iscell | |
ischar | |
isempty | |
isequal | |
isfloat | |
isglobal | |
isinf | |
2. 数据存取 Data Access
2.1 文件存取
2.1.1 save() 和 load()
- 保存workspace中所有的数据到
.mat
文件中;
clear; a = magic(4);
save mydata1.mat
save mydata2.mat -ascii % 使mydata1.mat文件在其他程序中打开不乱码;
load('mydata1.mat')
load('mydata2.mat','-ascii')
2.1.2 读取Excel文件:xlsread()
score = xlsread('04score.xlsx')
score = xlsread('04score.xlsx', 'B2:D4') % 读取注明单元格的内容;
2.1.3 写入Excel文件:xlswrite()
M = mean(score')'; % mean()函数是column 运算,而score中每个学生的成绩是row,故先转置 ' ,求得平均数,在转置回来;
xlswrite('04score.xlsx', M, 1, 'E2:E4'); % xlswrite('filename', 'variable', 'sheet(页)', 'location');
xlswrite('04score.xlsx', {'Mean'}, 1, 'E1'); % 写进表头{'Mean'};
std(A,a) % a=0时为无偏估计,分母为n-1;a=1时为有偏估计,分母为n。默认形式:std(A,0,1);
std(A,a,b) % 增加的形参b是维数,若A是二维矩阵,则b=1表示按列分,b=2表示按行分;若为三维以上,b=i就是增多的一维维数;
>> N = std(Score, 1, 2)
N =
0.8165
0.8165
0.8165
>> N = std(Score, 1, 1)
N =
2.6247 2.4495 2.6247
% 将N及表头写入Excel表格中;
>> xlswrite('04score.xlsx', N, 1, 'F2:F4');
>> xlswrite('04score.xlsx', {'std'}, 1, 'F1');
2.1.4 在Excel中获得文本
>> [Score Header] = xlsread('04score.xlsx')
Score =
1.0000 2.0000 3.0000 2.0000 0.8165
6.0000 5.0000 4.0000 5.0000 0.8165
7.0000 8.0000 9.0000 8.0000 0.8165
Header =
'' 'text1' 'text2' 'text3' 'Mean' 'std'
'a' '' '' '' '' ''
'ab' '' '' '' '' ''
'abc' '' '' '' '' ''
2.2 低阶文件输入/输出
- 以字节或字符级别读写文件;
- 具有
fid
; - 文件中的位置由可以移动的指针
pointer
指定;
2.2.1 功能函数
函数 | 功能 |
---|
fopen | |
fclose | |
fscanf | 读数据 |
fprintf | 写数据 |
feof | |
fid = fopen('[filename]', '[permission]');
status = fclose(fid);
permission
包括'r'
'r+'
'w'
'w+'
'a'
'a+'
2.2.2 举例:将正弦值写入文件
x = 0:pi/10:pi;
y = sin(x);
fid = fopen('sinx.txt','w');
for i=1:11
fprintf(fid,'%5.3f %8.4f\n', x(i), y(i));
end
fclose(fid);
type sinx.txt
2.2.3 通过格式化I/O进行读写
- Read:
A = fscanf(fid, format, size);
- Write:
fprintf(fid, format, x, y, ...);
A
:读取的数据;format
:格式说明符;size
:读取的数据量;x, y, ...
:写入的数据;- 格式说明符:
%-12.5e
;-12.5
表示宽度和精度;
2.2.4 从文件中读取
fid = fopen('04asciiData.txt','r'); i = 1;
while ~feof(fid)
name(i,:) = fscanf(fid,'%5c',1);
year(i) = fscanf(fid,'%d',1);
no1(i) = fscanf(fid,'%d',1);
no2(i) = fscanf(fid,'%d',1);
no3(i) = fscanf(fid,'%g',1);
no4(i) = fscanf(fid,'%g\n');
i=i+1;
end
fclose(fid);