颜色的配置可以通过以下几个方法:
● QQ截图
● 在线色表+颜色进制转换
http://tools.jb51.net/static/colorpicker/
● 色彩搭配
颜色的color:rgb(197,146,163);可以替代为十六进制color:c592a3;
dialog.h
#ifndef DIALOG_H
#define DIALOG_H
#include <QDialog>
#include <QDebug>
#include <QPushButton>
//通过宏定义设置按钮不同状态下的颜色
#define QPushButton_STYTLE (QString("\
/*按钮普通态*/\
QPushButton\
{\
font-family:Microsoft Yahei;\
/*字体大小为20点*/\
font-size:20pt;\
/*字体颜色为深色*/\
color:#272643;\
/*背景颜色*/\
background-color:#e3f6f5;\
/*边框圆角半径为8像素*/\
border-radius:8px;\
}\
/*按钮悬停态*/\
QPushButton:hover\
{\
/*背景颜色*/\
background-color:#bae8e8;\
}\
/*按钮按下态*/\
QPushButton:pressed\
{\
/*背景颜色*/\
background-color:rgb(44 , 105 , 141);\
/*左内边距为3像素,让按下时字向右移动3像素*/\
padding-left:3px;\
/*上内边距为3像素,让按下时字向下移动3像素*/\
padding-top:3px;\
}"))
class Dialog : public QDialog
{
Q_OBJECT
public:
Dialog(QWidget *parent = 0);
~Dialog();
private:
QPushButton* west;
QPushButton* east;
QPushButton* north;
QPushButton* south;
QPushButton* middle;
//私有槽函数
private slots:
//声明自定义槽函数
void mySlot1();
void mySlot2();
void mySlot3();
void mySlot4();
void mySlot0();
};
#endif // DIALOG_H
dialog.cpp
#include "dialog.h"
Dialog::Dialog(QWidget *parent)
: QDialog(parent)
{
resize(500,500);//重定义宽高
east = new QPushButton("东",this);//实例化按钮
east->setStyleSheet(QPushButton_STYTLE);//设置按钮不同状态下的颜色
east->resize(150,100);//定义按钮的宽高
east->move(325,200);//相对框图内的坐标移动
connect(east,SIGNAL(clicked()),this,SLOT(mySlot1()));
//connect,用于连接信号槽之间的因果关系
//参数1:发射者,通信的对象,此对象是信号槽触发的来源,例如:按钮对象(n.)
//参数2:信号函数,使用SIGNAL()包裹,表示发射者触发的效果,例如:点击(v.)
//参数3:接收者,通信对象,此对象是执行结果代码的主体(n.)
//参数4:槽函数,使用SLOT()包裹,表示接收者要执行的函数(v.)
west = new QPushButton("西",this);
west->setStyleSheet(QPushButton_STYTLE);
west->resize(150,100);
west->move(25,200);
connect(west,SIGNAL(clicked()),this,SLOT(mySlot2()));
south = new QPushButton("南",this);
south->setStyleSheet(QPushButton_STYTLE);
south->resize(150,100);
south->move(175,325);
connect(south,SIGNAL(clicked()),this,SLOT(mySlot3()));
north = new QPushButton("北",this);
north->setStyleSheet(QPushButton_STYTLE);
north->resize(150,100);
north->move(175,75);
connect(north,SIGNAL(clicked()),this,SLOT(mySlot4()));
middle = new QPushButton("中",this);
middle->setStyleSheet(QPushButton_STYTLE);
middle->resize(150,150);
middle->move(175,175);
connect(middle,SIGNAL(clicked()),this,SLOT(mySlot0()));
}
void Dialog::mySlot1()
{
int x = this->x();//获取框图在以屏幕左上角为坐标轴原点下的横坐标
int y = this->y();//获取框图在以屏幕左上角为坐标轴原点下的横坐标
move(x+28,y);//在指定的坐标下移动框图位置
qDebug() << "列阵在东,向东挺进28像素";//输出内容,并自动换行
}
void Dialog::mySlot2()
{
int x = this->x();
int y = this->y();
move(x-28,y);
qDebug() << "列阵在西,向西挺进28像素";
}
void Dialog::mySlot3()
{
int x = this->x();
int y = this->y();
move(x,y+28);
qDebug() << "列阵在南,向南挺进28像素";
}
void Dialog::mySlot4()
{
int x = this->x();
int y = this->y();
move(x,y-28);
qDebug() << "列阵在北,向北挺进28像素";
}
void Dialog::mySlot0()
{
qDebug() << "众将听令!回守中原!";
move(1170,562);
}
//析构函数
Dialog::~Dialog()
{
delete west;
delete east;
delete north;
delete south;
delete middle;
}
效果图如下:
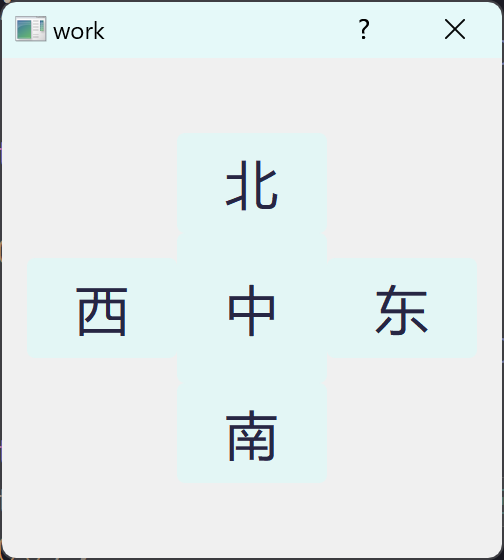
图中的文字按钮点击都可以有相应的效果,能向特定方向进行移动并输出内容